In PowerShell, you can create an empty array by using the following syntax:
$array = @()
What is an Array in PowerShell?
An array in PowerShell is a data structure that allows you to store multiple items in a single variable. This is particularly important for scripting and automation tasks where you need to manage collections of data efficiently. Arrays can hold data of varying types, including strings, integers, and even other arrays, making them a versatile choice for developers.
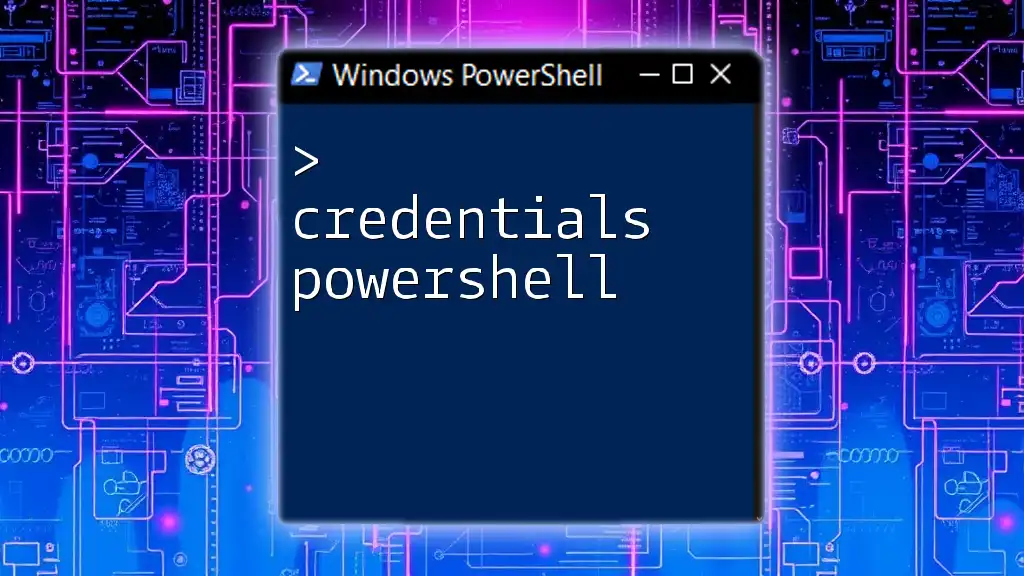
Why Use an Empty Array?
Empty arrays are valuable tools for flexibility in data storage. When you create an empty array, you're setting up a placeholder that can dynamically expand as you add items. This is especially useful when the size of data is not known upfront, allowing your scripts to adapt and respond to different data inputs seamlessly.
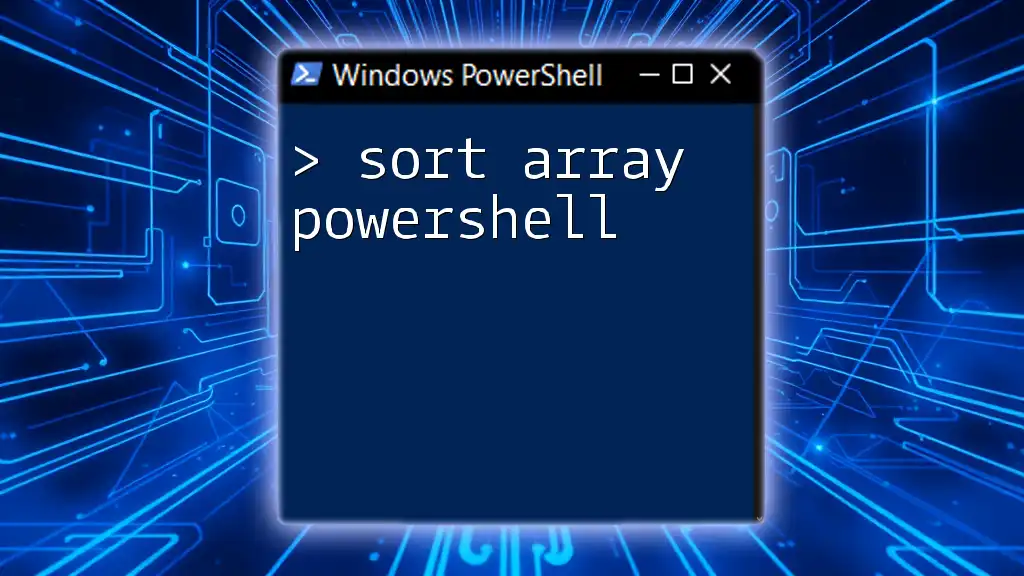
Creating an Empty Array in PowerShell
Syntax for Creating an Empty Array
To create an empty array in PowerShell, the most common syntax is the following:
$myArray = @()
This line initializes a new variable, `$myArray`, as an empty array. This approach is straightforward and widely used among PowerShell users.
Different Methods for Initializing an Empty Array
Using the Array Subexpression
You can also create an empty array using the array subexpression method:
$myAnotherArray = @( )
This method produces a similar result to the previous one, but it can be particularly useful in expressions where explicit array creation is needed.
Using the Array Constructor
A more formal method involves using the array constructor:
[array]$myThirdArray = @()
This method creates an empty array while explicitly declaring its type as an `[array]`. It can help clarify intent in scripts where understanding the data type is vital.
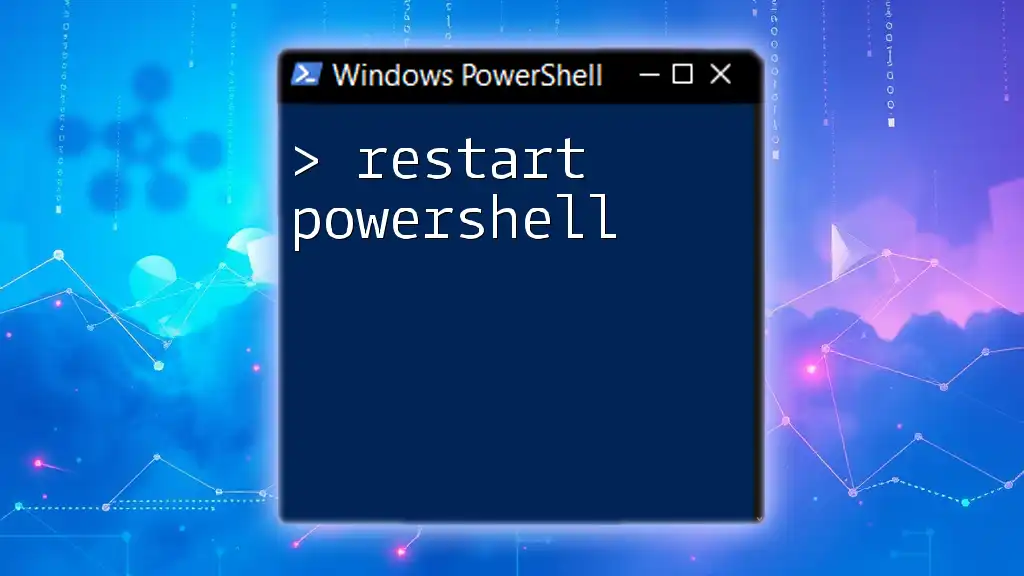
Adding Items to an Empty Array
Dynamic Array Resizing
One of the most powerful features of arrays in PowerShell is their dynamic resizing capability. When you add items to an empty array, PowerShell automatically handles resizing behind the scenes. For example:
$myArray += "First Item"
$myArray += "Second Item"
After executing this code, `$myArray` now contains two items, showcasing how you can build up your array iteratively.
Common Pitfalls When Adding Items
While adding items to an array is straightforward, it’s essential to be aware of potential pitfalls. For example, if you try to manipulate an array with incompatible types or use incorrect addition syntax, you may encounter issues. Always ensure you are using the `+=` operator to append items correctly.
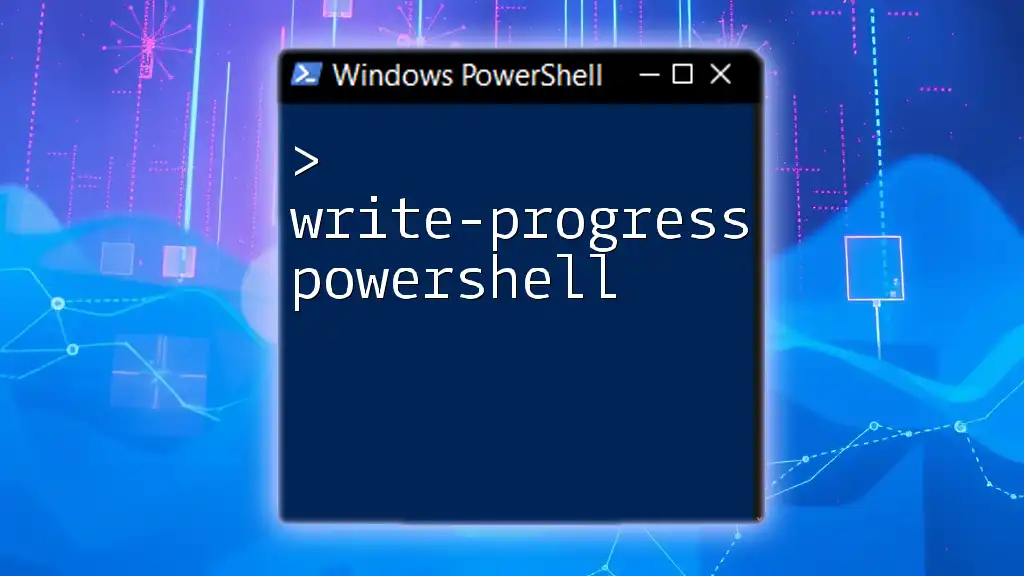
Accessing Array Elements
Zero-Based Indexing
In PowerShell, arrays use zero-based indexing, meaning the first item of an array is located at index `0`. For example:
$firstElement = $myArray[0]
This line will retrieve the first item from `$myArray`. Understanding this indexing scheme is fundamental when working with arrays to avoid off-by-one errors.
Looping Through an Array
The `foreach` loop is an intuitive way to iterate through all the elements in an array. Here’s how you can do it:
foreach ($item in $myArray) {
Write-Host $item
}
This loop will output each item contained in the array, making it easy to process all elements sequentially.
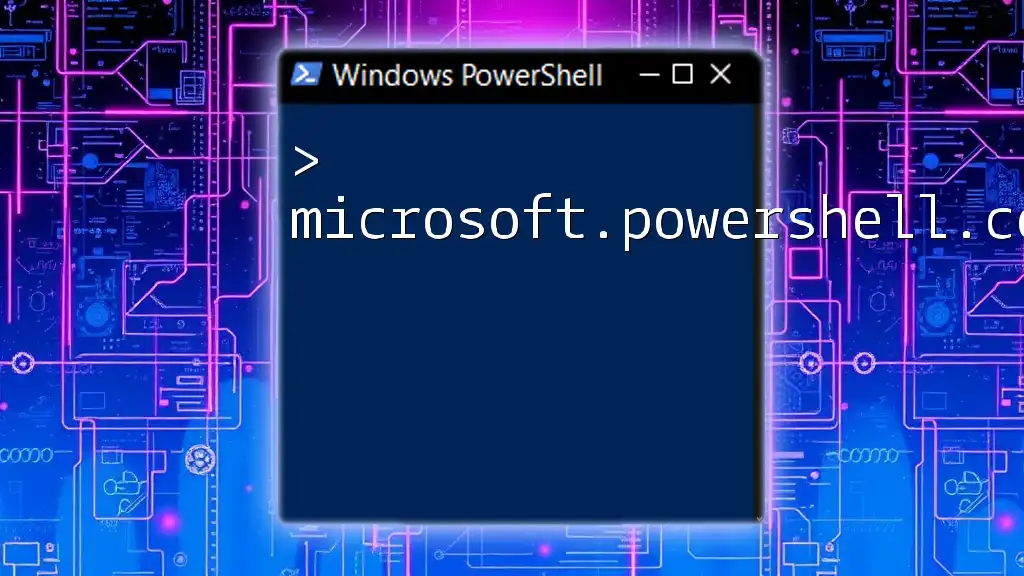
Manipulating Empty Arrays
Clearing an Array
At times, you might want to clear an array without affecting its initial variable assignment. One way to achieve this is by calling the `Clear` method:
$myArray.Clear()
After this command, `$myArray` will remain an array but will no longer contain any elements.
Checking for Emptiness
To verify if an array is empty, you can use the following approach:
if ($myArray.Count -eq 0) {
Write-Host "Array is empty"
}
This simple check ensures you know the state of your array before proceeding with any operations.
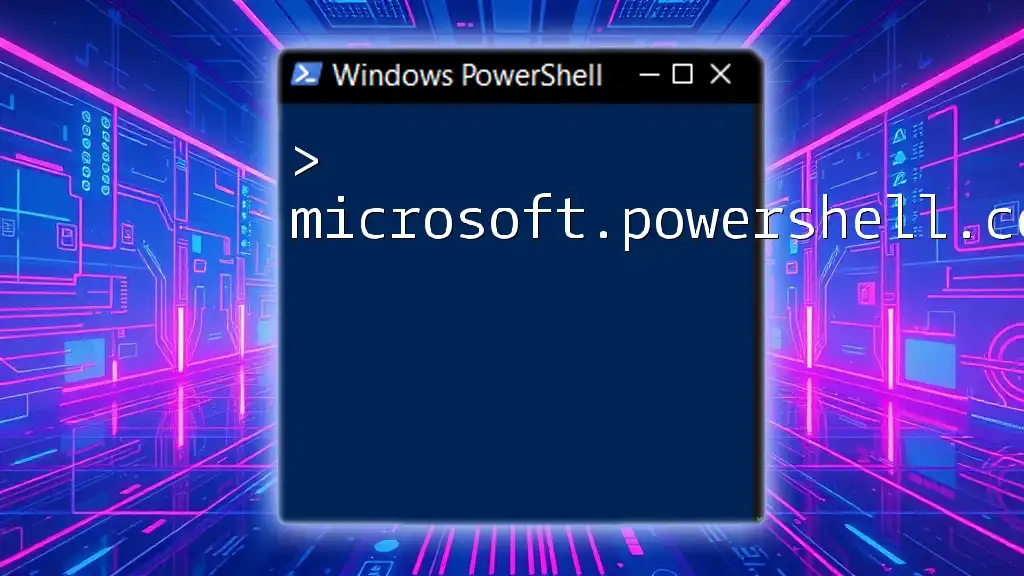
Use Cases for Empty Arrays in PowerShell Scripts
Data Collection
Using empty arrays is vital in scenarios where you need to collect data from various sources. For instance, if you're processing multiple files and want to gather their contents into a single collection, initializing an empty array allows you to dynamically store and manipulate that data.
Temporary Storage
Empty arrays can be handy for temporary storage, especially during script execution that requires intermediate results. By initializing an empty array, you can subsequently fill it with specific data points that help you accomplish tasks in more complex scripts.
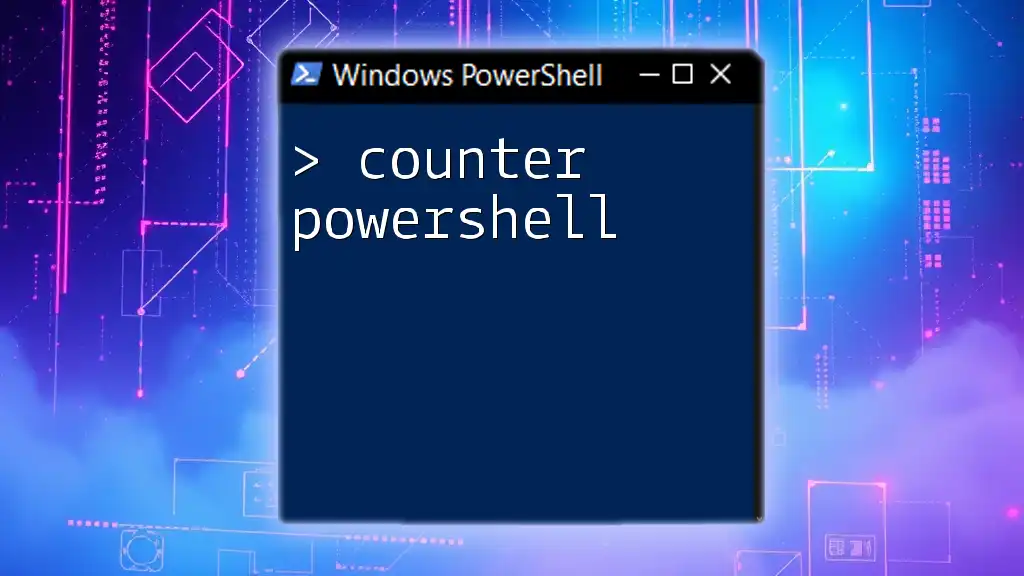
Best Practices for Working with Empty Arrays
Keeping Arrays Clean and Manageable
Maintaining the cleanliness of your arrays is crucial. Use clear naming conventions and comments within your scripts to enhance readability. This practice ensures future maintenance is more manageable for you or anyone else working on your scripts.
Performance Considerations
While arrays in PowerShell are robust, consider their performance implications in extensive scripting. For example, certain operations, such as repeatedly resizing an array by adding items, can become less efficient as the size grows. If performance becomes an issue, consider using alternative data structures, such as `ArrayList` or `Dictionary`, depending on your needs.
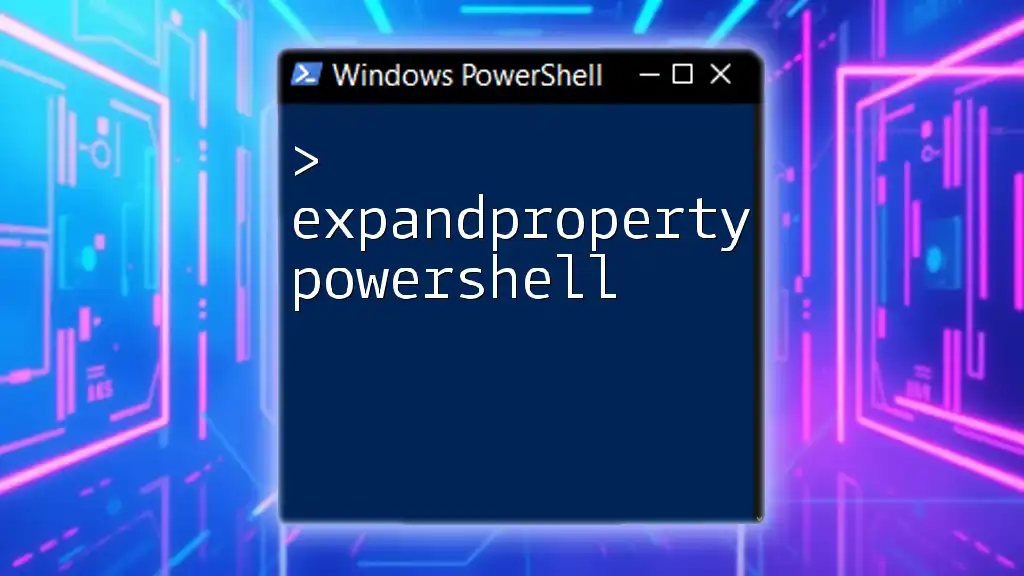
Conclusion
In PowerShell, the ability to create empty arrays and manage them efficiently puts powerful tools at your disposal. They allow for dynamic data management, facilitating efficient scripting. By understanding the methods of creation, manipulation, and best practices for arrays, PowerShell users can enhance their script functionality and reliability. Whether for data collection or temporary storage, mastering empty arrays will significantly aid your automation tasks.
Further Reading and Resources
For more in-depth information, you can refer to official Microsoft documentation and additional PowerShell learning resources. Staying updated with the latest in PowerShell will only enhance your scripting capabilities.