You can execute a SQL script from PowerShell by utilizing the `Invoke-Sqlcmd` cmdlet, which allows you to run Transact-SQL commands directly against a SQL Server instance.
Here's a code snippet to demonstrate how to run a SQL script:
Invoke-Sqlcmd -ServerInstance "YourServerName" -Database "YourDatabaseName" -InputFile "C:\Path\To\Your\Script.sql"
Prerequisites for Running SQL Scripts Using PowerShell
Understanding SQL Server and PowerShell
PowerShell is a powerful command-line shell and scripting language that lets you automate tasks and manage configurations across Windows systems. When combined with SQL Server, it allows users to manipulate databases and execute SQL commands effortlessly. Understanding how both tools can work together is essential for effective database management and automation.
Required Software and Tools
To successfully run a SQL script from PowerShell, you need a few tools and libraries:
- SQL Server Management Studio (SSMS): This is the primary tool for managing SQL Server databases and will help in craft SQL scripts.
- SQL Server PowerShell Module: This module provides cmdlets that simplify database management tasks.
Make sure you have the necessary versions installed that match your SQL Server setup.
Access and Permissions
Executing SQL scripts requires appropriate permissions on the target database. Always check if your user role can execute the commands contained in the SQL script. If you encounter issues, consult with your database administrator to ensure you have sufficient permissions.
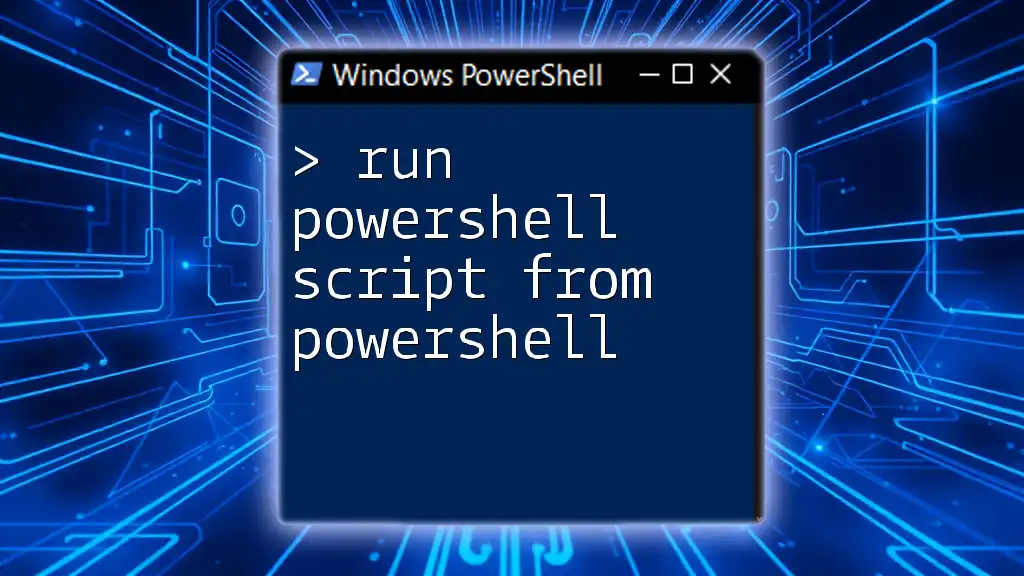
Setting Up Your PowerShell Environment for SQL Script Execution
Installing SQL Server PowerShell Module
The first step to run a SQL script from PowerShell is to ensure that you have the SQL Server PowerShell module installed. You can quickly set it up through PowerShell itself by using the following command:
Install-Module -Name SqlServer -AllowClobber -Force
This command will install the SQL Server module, allowing you to access its cmdlets.
Loading the SQL Server Module
Once the module is installed, you need to load it into your PowerShell session before running SQL-related commands. You can do this by executing the following command:
Import-Module SqlServer
Now you’re ready to connect to SQL Server!
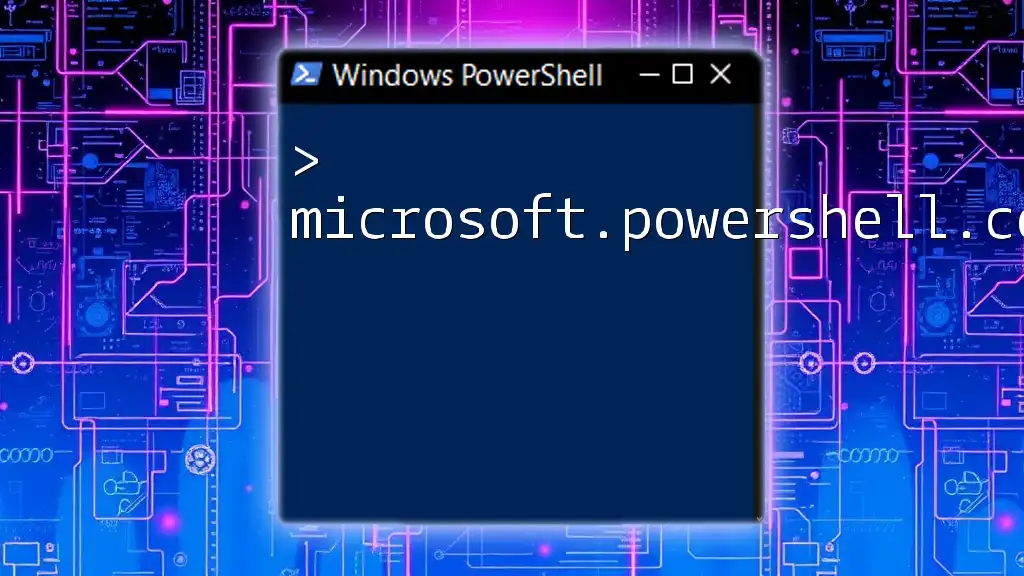
How to Execute an SQL Script from PowerShell
Connecting to the SQL Server
To begin executing SQL scripts, you need to establish a connection to your SQL Server instance. This is typically done using a connection string. Here’s a sample of how to set up the connection string in PowerShell:
$connectionString = "Server=your_server;Database=your_database;Integrated Security=True;"
$connection = New-Object System.Data.SqlClient.SqlConnection($connectionString)
Replace `your_server` and `your_database` with the appropriate server name and database name. If you are using SQL authentication, you may add `User Id` and `Password` to the connection string.
Running the SQL Script
Once you have a connection established, the next step is to read your SQL script from a file. This can be done with the following snippet:
$sqlScript = Get-Content -Path "C:\path\to\your\script.sql" -Raw
Make sure the path to the SQL script is correct.
Now that you have the script stored in a variable, you can execute it against the database by creating a command object:
$command = $connection.CreateCommand()
$command.CommandText = $sqlScript
Finally, you can open the connection and execute the SQL command:
$connection.Open()
$command.ExecuteNonQuery()
$connection.Close()
Example Demonstration
Here’s how everything fits together in a complete PowerShell script for running a SQL script:
# Load SQL Server Module
Import-Module SqlServer
# Connection string
$connectionString = "Server=your_server;Database=your_database;Integrated Security=True;"
$connection = New-Object System.Data.SqlClient.SqlConnection($connectionString)
# SQL Script
$sqlScript = Get-Content -Path "C:\path\to\your\script.sql" -Raw
# Execute the SQL Script
$command = $connection.CreateCommand()
$command.CommandText = $sqlScript
$connection.Open()
$command.ExecuteNonQuery()
$connection.Close()
Write-Host "SQL Script executed successfully."
This complete script is easy to adapt. Just change the server and database details and specify your SQL script file's correct path.
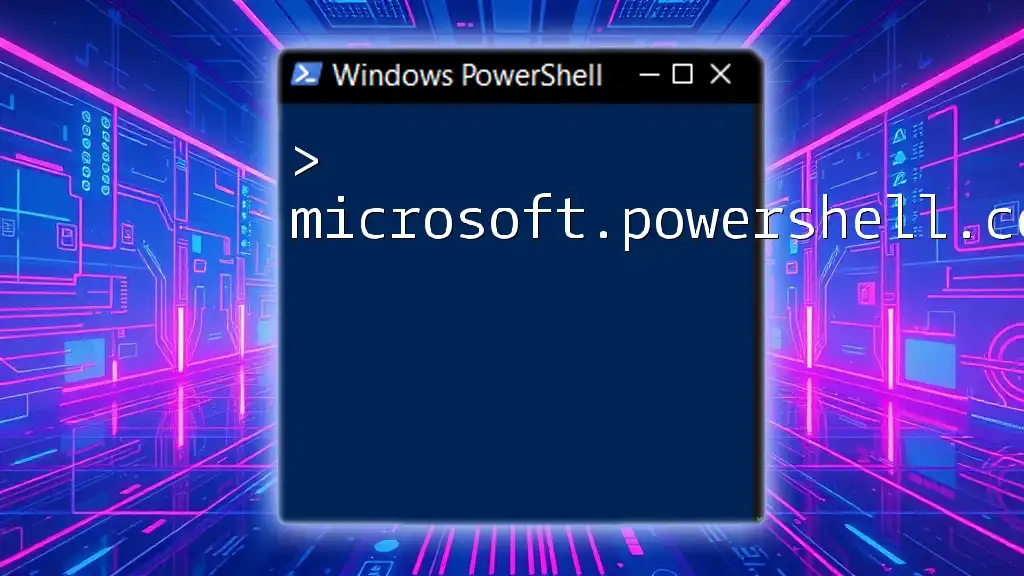
Troubleshooting Common Issues
Connection Failures
If you run into issues when attempting to connect to SQL Server, verify the connection string's accuracy. Common errors often arise from using the wrong server name, database name, or incorrect authentication credentials. A simple check against your SQL Server Configuration Manager may help clarify the correct details.
Script Execution Errors
When executing SQL scripts, you might see various errors related to the SQL syntax or permissions. Review the error messages carefully as they provide direction on what went wrong. If necessary, test the SQL commands directly within SQL Server Management Studio to identify and troubleshoot the issues.
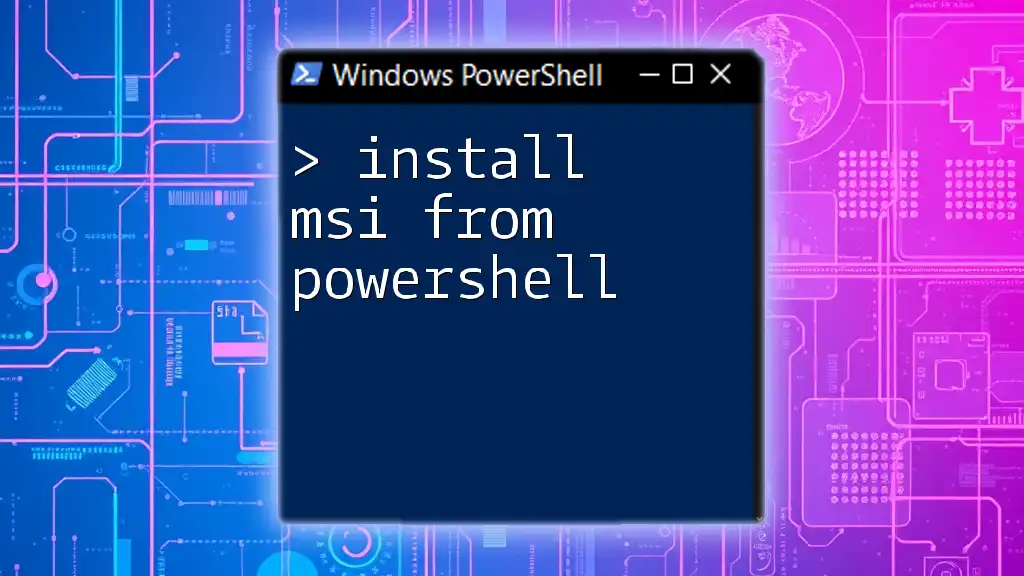
Best Practices for Running SQL Scripts from PowerShell
Using Try-Catch for Error Handling
To improve reliability, it’s good practice to implement error handling. By using a try-catch block, you can manage errors gracefully and understand failures better. Here’s an example:
try {
$connection.Open()
$command.ExecuteNonQuery()
Write-Host "SQL Script executed successfully."
} catch {
Write-Host "Error: $_"
} finally {
$connection.Close()
}
Logging Execution Results
Logging is essential to track what has been executed and any issues that have arisen during the process. You may log messages to the console or write them to a file, allowing for easier investigation later.
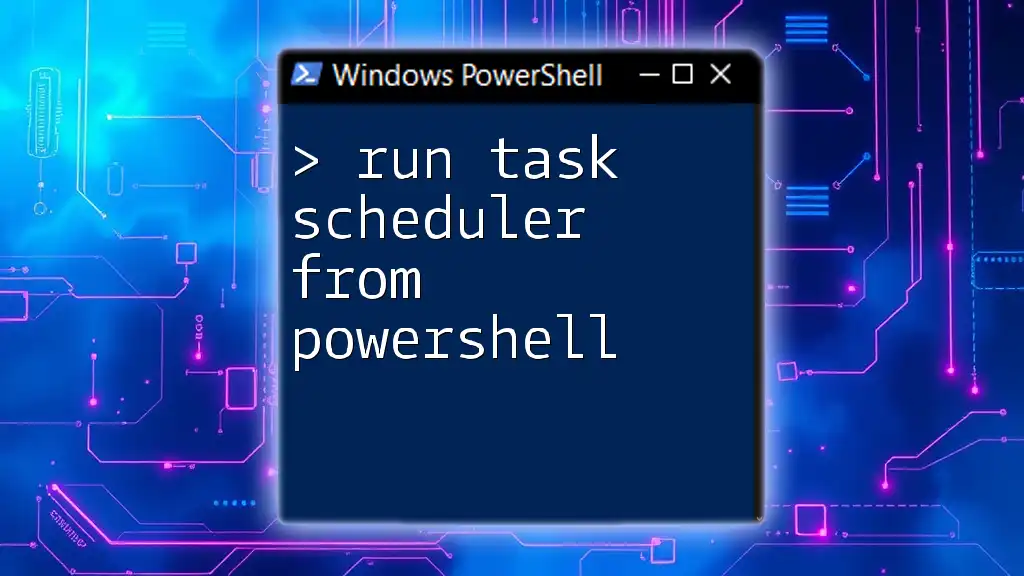
Conclusion
In this guide, we’ve covered the essential steps you need to run a SQL script from PowerShell. By utilizing the power of PowerShell, you can streamline SQL operations, improve efficiency, and automate repetitive tasks. With practice, proficiency will come, making SQL script execution via PowerShell a powerful tool in your skillset.
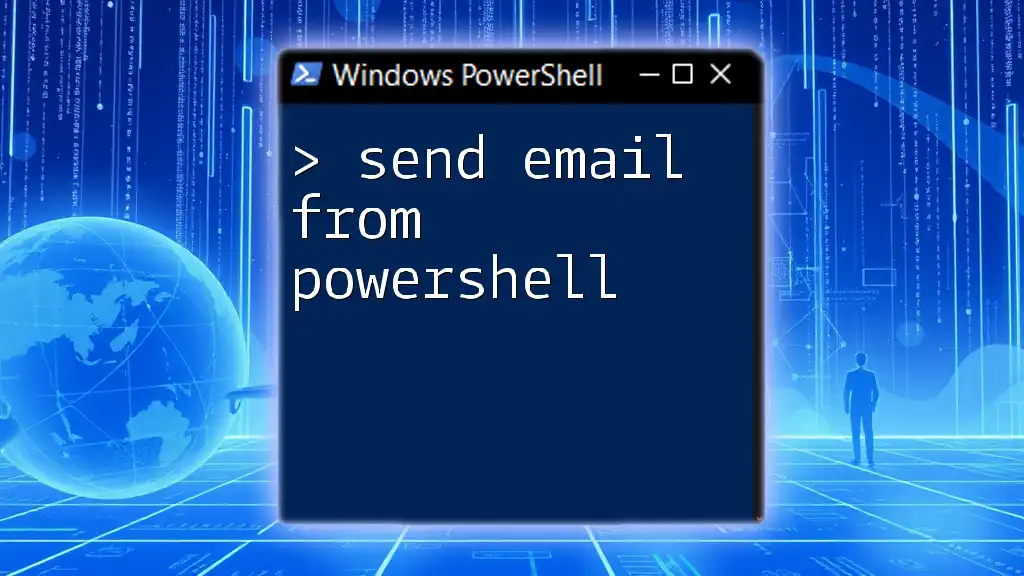
Additional Resources
For further reference, Microsoft provides extensive documentation on the SQL Server PowerShell Module. Engage with learning platforms that offer courses tailored to PowerShell scripting and SQL Server management, which can further enhance your skills.