To run a SQL query in PowerShell, you can use the `Invoke-Sqlcmd` cmdlet to execute your SQL command against a SQL Server database.
Invoke-Sqlcmd -Query "SELECT * FROM YourTable" -ServerInstance "YourServerName" -Database "YourDatabaseName"
What is PowerShell?
PowerShell is a powerful task automation and configuration management framework, consisting of a command-line shell and associated scripting language. It enables the manipulation of data and the management of system settings. Beyond its standard capabilities, PowerShell allows users to execute commands called cmdlets, which can carry out a vast array of tasks, from simple file operations to complex networking commands.
One of the standout features of PowerShell is its ability to interact with various data sources, including relational databases such as SQL Server. This gives IT professionals and database administrators a flexible tool for database management. You can not only read and manipulate data but also automate repetitive database tasks using scripts.
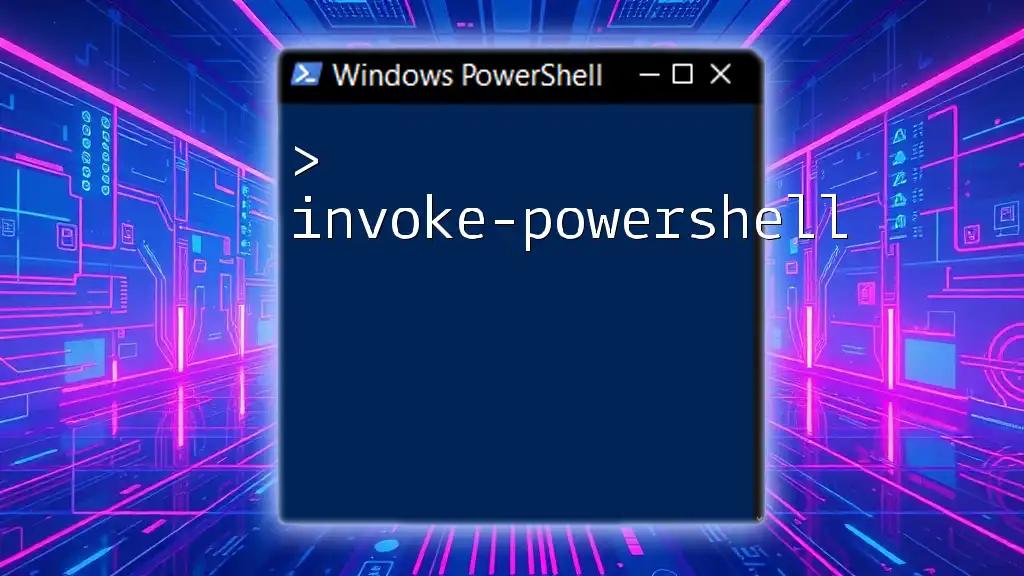
Setting Up Your Environment
Prerequisites for Running SQL Queries
Before you can run SQL queries in PowerShell, it's essential to ensure that you have the necessary environment set up correctly.
Install the SQL Server Module: PowerShell can enhance its capabilities by leveraging specific modules. In this case, you need the SQL Server module, which provides cmdlets specifically designed for SQL interactions. Install the module by executing the following command:
Install-Module -Name SqlServer
Connecting to SQL Server
One of the first steps in running SQL queries is establishing a connection to your SQL Server.
Using Integrated Security vs SQL Authentication: You have two primary methods to establish connections: Integrated Security, which uses the credentials of the currently logged-in Windows user, and SQL Authentication, which requires a username and password. Depending on your organizational policy, you will choose the most suitable method.
Sample Code Snippet for Connecting to SQL Server:
Here's an example of how to connect to a SQL Server using Integrated Security:
$connectionString = "Server=your_server;Database=your_database;Integrated Security=True;"
$connection = New-Object System.Data.SqlClient.SqlConnection($connectionString)
In the above command, replace `your_server` and `your_database` with your actual server name and database name.
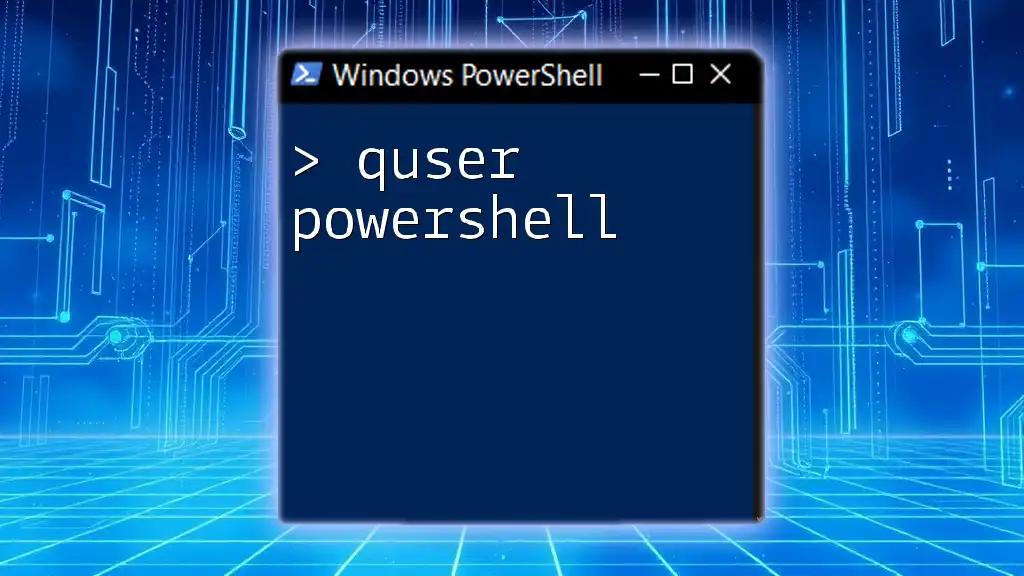
Constructing and Running SQL Queries in PowerShell
Running a Simple SQL Query
Once you have successfully connected to your SQL Server, you can begin executing SQL queries.
Example of a Select Query: The `SELECT` statement is one of the most common SQL commands used to fetch data from a database. Here's how you can execute a simple `SELECT` query to retrieve all records from a specified table:
$command = $connection.CreateCommand()
$command.CommandText = "SELECT * FROM your_table"
$connection.Open()
$reader = $command.ExecuteReader()
while ($reader.Read()) {
Write-Host $reader["ColumnName"]
}
$connection.Close()
In this code snippet, replace `your_table` with the name of your table and `ColumnName` with a column you wish to display. This example shows how to read each record from the returned data and display it in the console.
Running More Complex Queries
As you gain confidence, you can start working with complex SQL queries, including data manipulation.
Inserting, Updating, and Deleting Records: These operations are often necessary during database management, and they can be accomplished using straightforward SQL commands. Below are examples of how to perform these operations:
# Insert
$command.CommandText = "INSERT INTO your_table (Column1, Column2) VALUES ('Value1', 'Value2')"
$command.ExecuteNonQuery()
# Update
$command.CommandText = "UPDATE your_table SET Column1 = 'NewValue' WHERE ConditionColumn = 'ConditionValue'"
$command.ExecuteNonQuery()
# Delete
$command.CommandText = "DELETE FROM your_table WHERE ConditionColumn = 'ConditionValue'"
$command.ExecuteNonQuery()
These snippets illustrate how to insert a new row, update existing records, and delete records from the specified table. Be sure to adjust the SQL queries to fit your specific requirements.

Handling SQL Script Files
Running SQL Scripts from PowerShell
Introduction to SQL Scripts: SQL scripts often contain multiple SQL statements within a single file, allowing complex manipulations and batch processing of commands. Running an entire script can save you the time of executing individual commands.
Example Code to Run SQL Script File:
If you have a SQL script saved at a specified file path, you can easily execute it in PowerShell:
$sqlFilePath = "C:\Path\To\Your\Script.sql"
$sqlScript = Get-Content $sqlFilePath -Raw
$command.CommandText = $sqlScript
$command.ExecuteNonQuery()
This code reads the SQL script file into PowerShell and then executes the contained commands. Ensure that the file path is correct and accessible to PowerShell.
Best Practices for Running SQL Queries
To ensure the security and efficiency of your SQL queries, following best practices is advisable.
Using Parameterized Queries: One of the most crucial aspects of SQL programming is to avoid SQL injection— a prevalent form of attack where malicious users can manipulate queries by injecting harmful SQL commands. Parameterized queries help mitigate this risk:
$command.CommandText = "SELECT * FROM your_table WHERE Column1 = @Value"
$command.Parameters.Add((New-Object Data.SqlClient.SqlParameter("@Value", "SomeValue")))
In this example, `@Value` serves as a parameter placeholder. The value to be queried is then safely passed through `Parameters.Add`, which safeguards against unwanted SQL injection attacks.
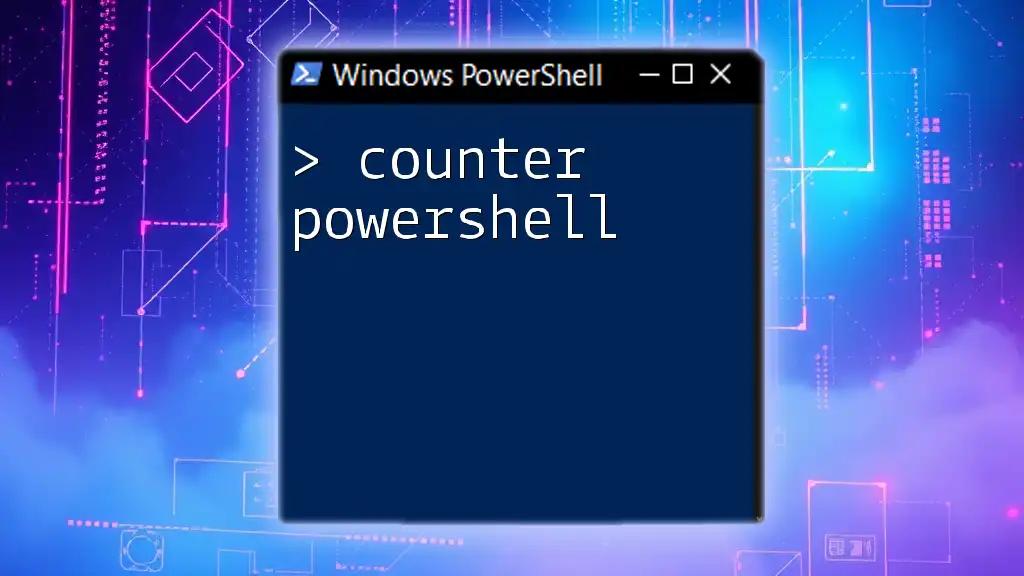
Troubleshooting Common Errors
Connection Issues
Connection errors are common stumbling blocks when attempting to connect to SQL Server from PowerShell. Verify that your SQL Server instance is running and accessible. Also, check firewall settings that might be blocking the connection to your database server.
Syntax Errors in Queries
When running SQL commands, it's not uncommon to encounter syntax errors. Always ensure that your SQL statements are correctly structured and that you're using the proper table and column names. Pay close attention to error messages, as they can provide insight into what went wrong in your query.
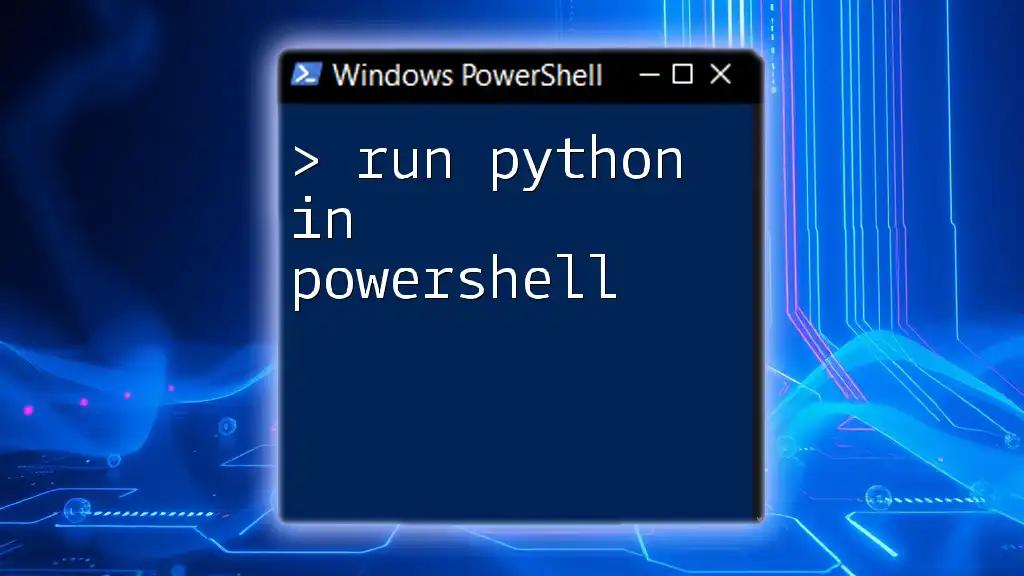
Conclusion
This guide demonstrated how to effectively run SQL queries in PowerShell, from connecting to a SQL Server instance to executing various commands, including SELECT, INSERT, UPDATE, and DELETE operations. Equipped with this knowledge, you can automate database tasks efficiently and securely.
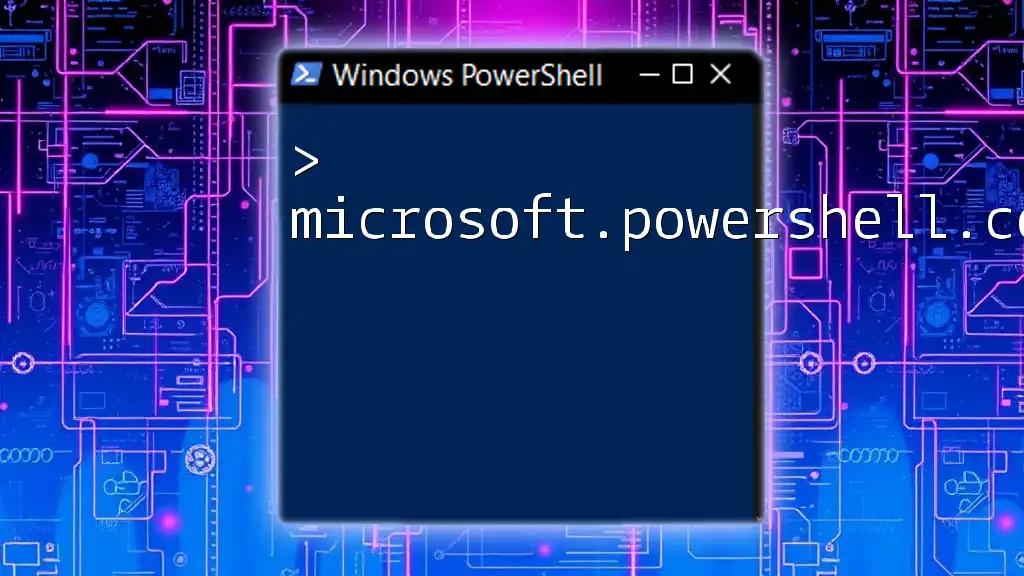
Additional Resources
For further exploration, consider checking out the official PowerShell documentation, which provides in-depth guidance on cmdlets and scripting techniques. Additionally, several online courses offer valuable insights for mastering SQL Server and PowerShell.
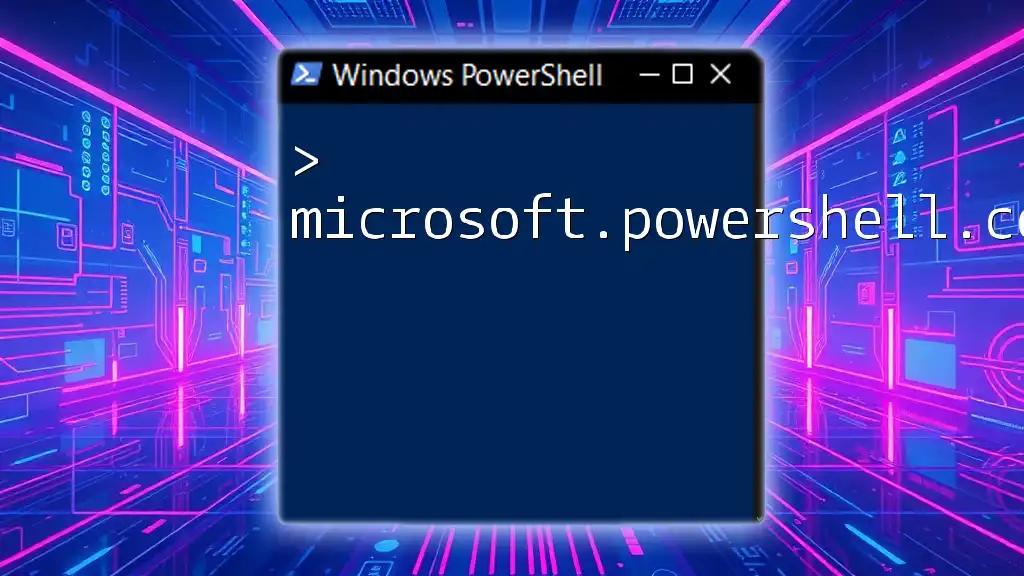
Call to Action
We welcome your feedback and any questions you may have as you embark on your journey with PowerShell and SQL. For more tips and tricks on using PowerShell, subscribe to our newsletter or leave a comment below!