To print a statement in PowerShell, you can use the `Write-Host` cmdlet to display messages in the console.
Write-Host 'Hello, World!'
Understanding the Print Statement in PowerShell
A print statement is a fundamental component of any programming language, allowing users to output text or values to the console. In PowerShell, utilizing print statements is essential for displaying information, debugging, and providing feedback to users as scripts execute. While the concept of printing in PowerShell is similar to other languages, specific commands and methodologies set it apart.
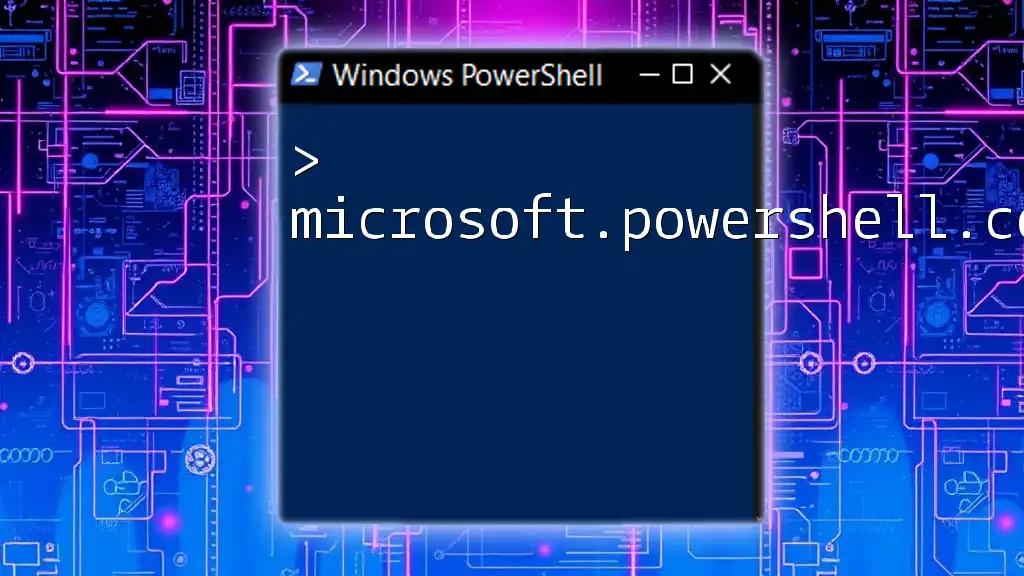
Basic Print Statement: `Write-Host`
What is Write-Host?
`Write-Host` is a command used to display messages directly to the console. It's primarily used for outputting text that is informational and doesn't need further processing in the pipeline.
Syntax:
Write-Host "Your message here"
Example Usage
Here’s a simple usage example that displays a message:
Write-Host "Hello, World!"
When executed, this command will output Hello, World! to the console.
Formatting Output
One of the powerful features of `Write-Host` is its ability to format output. You can customize the appearance of text using parameters for color and background.
For instance:
Write-Host "Success!" -ForegroundColor Green -BackgroundColor Black
In this example, the text Success! is displayed in green on a black background, enhancing visibility and making messages more engaging.
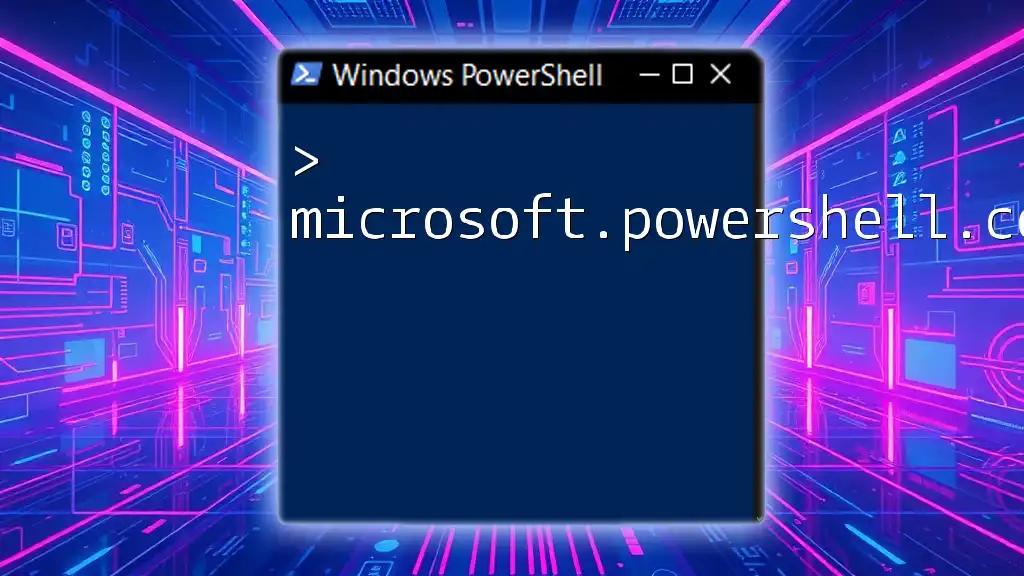
Alternative Methods to Display Output
Write-Output
Another commonly used command in PowerShell for printing is `Write-Output`. This command outputs data to the console and is specifically designed to work with the pipeline.
Syntax:
Write-Output "Your message here"
This command behaves similarly to `Write-Host`, but its key difference is that it can send output down a pipeline to other commands, making it valuable for script integration.
Example Usage
To illustrate its use:
Write-Output "This is output text."
This command simply prints This is output text. to the console. However, because it can function with the pipeline, this output can be further processed or directed.
Echo vs Write-Output
PowerShell also allows shorthand for `Write-Output` through the `echo` command. This command serves the same purpose as `Write-Output`.
For example:
echo "This is echoed text."
In this case, This is echoed text. will be displayed just as it would with `Write-Output`.
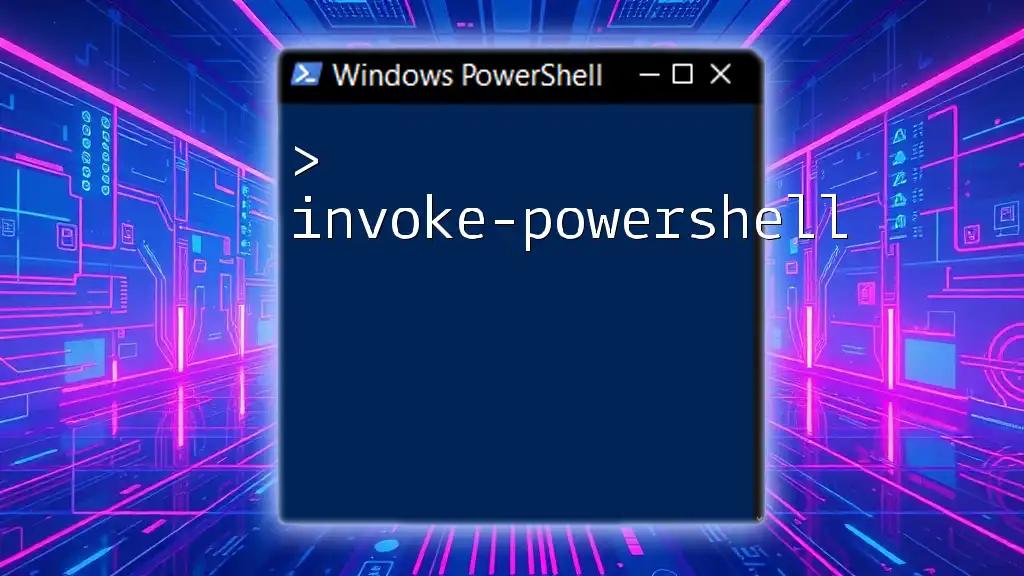
Advanced Print Statement Techniques
Using Variables in Print Statements
You can leverage print statements to display values stored within variables. This approach allows for dynamic message creation that can cater to various scenarios.
For illustration:
$name = "John"
Write-Host "My name is $name"
The resulting output will be My name is John, demonstrating the flexibility of variable use in print statements.
Formatting Strings with Interpolation
String interpolation in PowerShell is a powerful feature that enables you to insert other variables or expressions directly within strings.
For example:
$num1 = 10
$num2 = 20
Write-Host "The sum of $num1 and $num2 is $(($num1 + $num2))"
Here, the command prints The sum of 10 and 20 is 30, showcasing how calculations can also be integrated seamlessly.
Combining Multiple Outputs
To combine multiple print outputs into a single statement, you can concatenate different variables and strings easily.
An example would be:
$greeting = "Hello"
$name = "Sarah"
Write-Host "$greeting, $name! Welcome to PowerShell."
This outputs Hello, Sarah! Welcome to PowerShell., giving clarity and context to your messages.
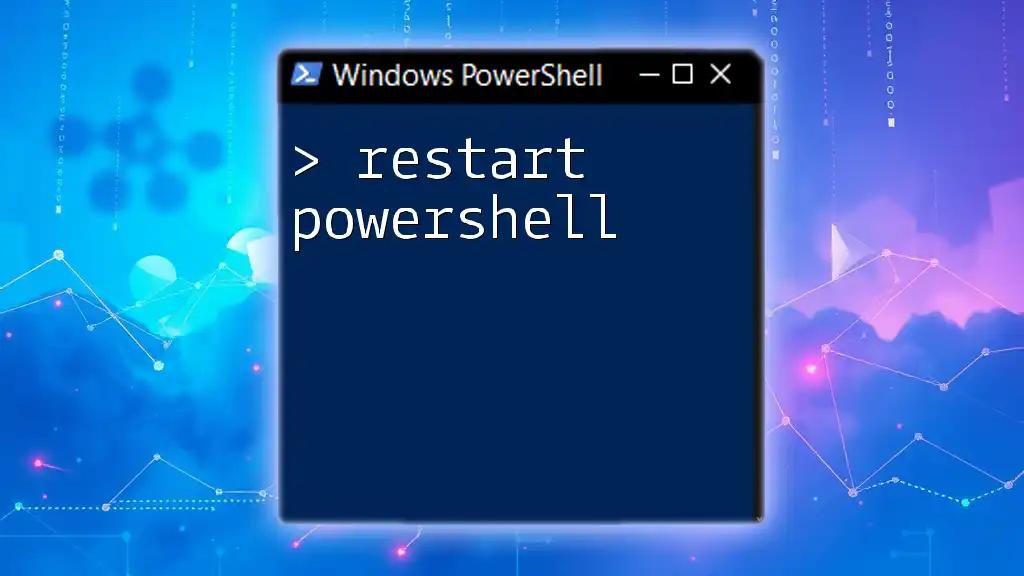
Debugging with Print Statements
Using Print Statements for Troubleshooting
Print statements are not only for display; they serve as crucial tools for debugging. By placing `Write-Host` at various points within your code, you can track the flow of execution and check variable values.
Consider the following example:
if ($age -lt 18) {
Write-Host "You are a minor."
} else {
Write-Host "You are an adult."
}
In this case, depending on the value of the `$age` variable, the corresponding message will be printed, making it easy to pinpoint the current state of execution.
Logging Information for Monitoring
Print statements can also be used for logging purposes. By adding timestamps to your outputs, you can keep track of when specific actions occur in your script.
For example:
Write-Host "$(Get-Date): Starting the script..."
This will output the current date and time followed by Starting the script..., allowing you to monitor script progression effectively.
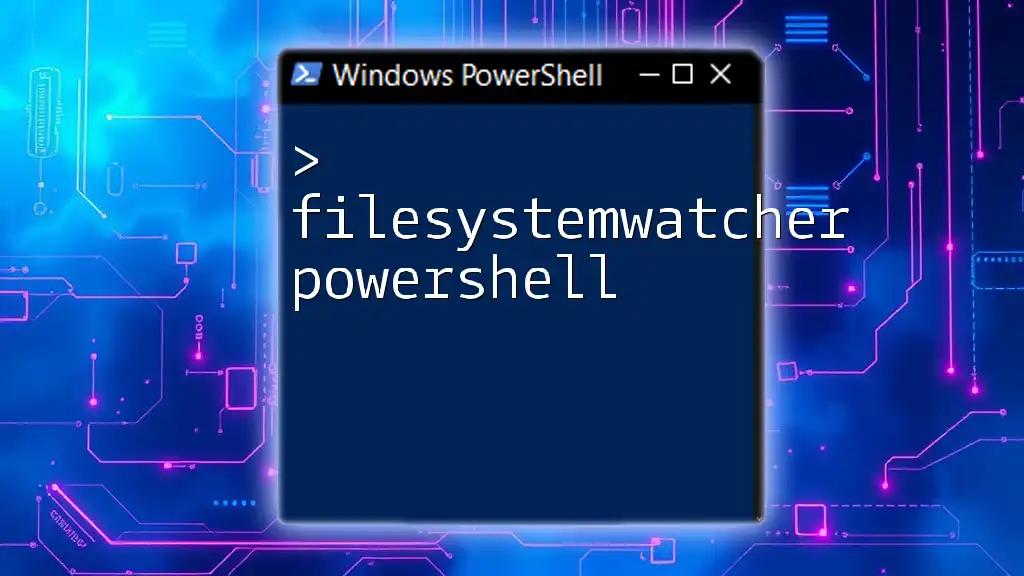
Best Practices for Using Print Statements
Choosing the Right Output Method
It's essential to understand when to use specific print commands. Use `Write-Host` for output meant solely for user information, while `Write-Output` is more suited for messages that you want to be used within the pipeline.
Keep Output Concise and Meaningful
Clear, concise messages enhance readability and make debugging easier. Avoid verbose output that can clutter the console and obfuscate important information.

Conclusion
In PowerShell, effectively utilizing print statements is vital for both user interaction and debugging. With commands like `Write-Host`, `Write-Output`, and the ability to incorporate variables and formatting, you can enhance your scripts' interactivity and functionality. Embrace these print statements in your workflows, and you'll find them invaluable in your PowerShell scripting journey. Explore more PowerShell tutorials to deepen your knowledge and skills!
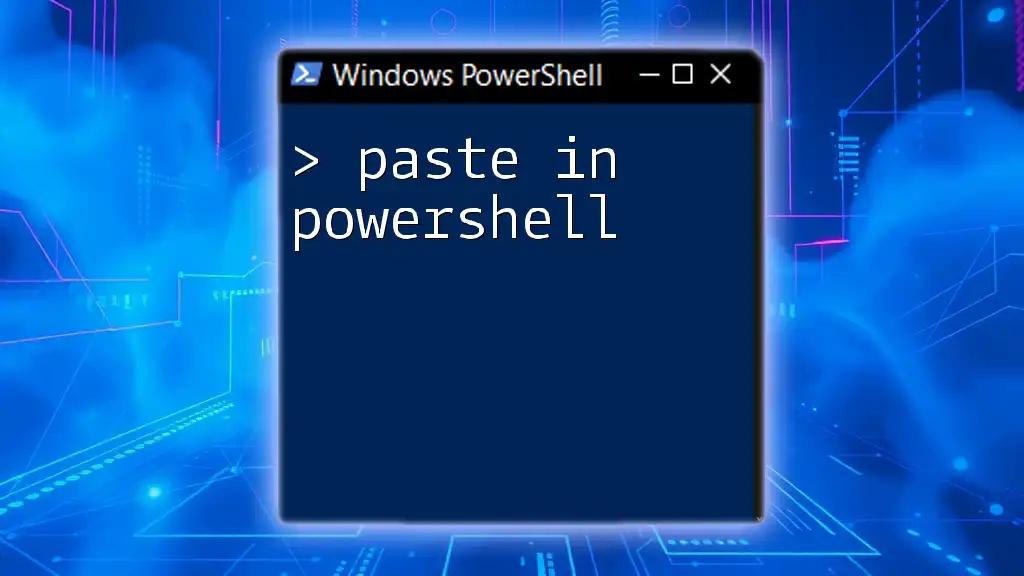
Additional Resources
For further study, refer to the official PowerShell documentation, PowerShell community forums, and explore additional articles on related topics to continue your learning path in scripting with PowerShell.