In PowerShell, you can determine the length of a string by using the `.Length` property, as shown in the following code snippet:
$string = "Hello, World!"
$length = $string.Length
Write-Host "The length of the string is $length"
Understanding String Length in PowerShell
What is a String in PowerShell?
A string in PowerShell is a sequence of characters used to represent text. Strings can be enclosed in single quotes (`'`) for literal strings or double quotes (`"`) for strings that allow variable interpolation. Understanding strings is fundamental for any programming or scripting because they are one of the primary data types you will work with.
Why is String Length Important?
String length refers to the number of characters present in a string. This measurement is crucial for various programming tasks. For instance, you might need to validate user input, format output, or manipulate text. Knowing how to check the length of a string effectively can save time and prevent errors in your scripts and applications.
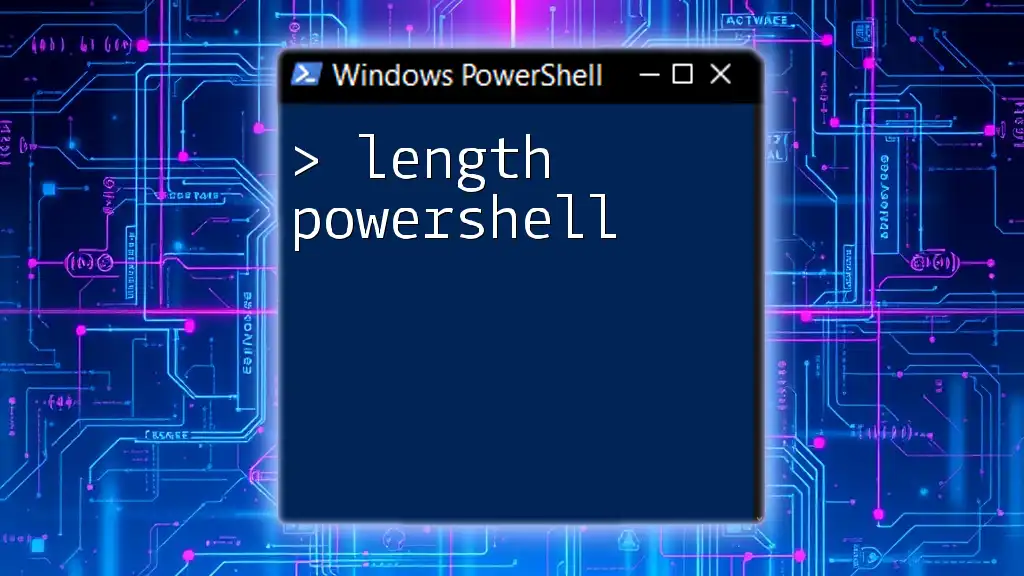
Basic Concepts of String Length in PowerShell
Introduction to PowerShell String Length
PowerShell string length is an inherent property of string objects. This property allows you to determine how many characters are in a string, which can come in handy in numerous scenarios, such as input validation and data processing.
Retrieving String Length in PowerShell
You can retrieve the string length in PowerShell in a couple of straightforward ways:
Using the `.Length` Property The simplest way to check string length is by using the `.Length` property. This property is directly accessible on any string variable.
$myString = "Hello, World!"
$length = $myString.Length
Write-Host "The length of the string is: $length"
In this example, the output will confirm that the string “Hello, World!” is 13 characters long. This method is efficient and quick, making it the go-to for checking string lengths.
Utilizing the `Measure-Object` Cmdlet Another way to obtain the length of a string is by using the `Measure-Object` cmdlet. This method can be particularly useful when chaining commands or processing pipeline data.
$myString = "PowerShell"
$length = ($myString | Measure-Object -Character).Characters
Write-Host "The length of the string is: $length"
This code snippet also outputs the length of the string, albeit using a more convoluted method than accessing the `.Length` property directly. While `Measure-Object` is versatile, it is not as efficient for simply retrieving string length, so use this method as needed.
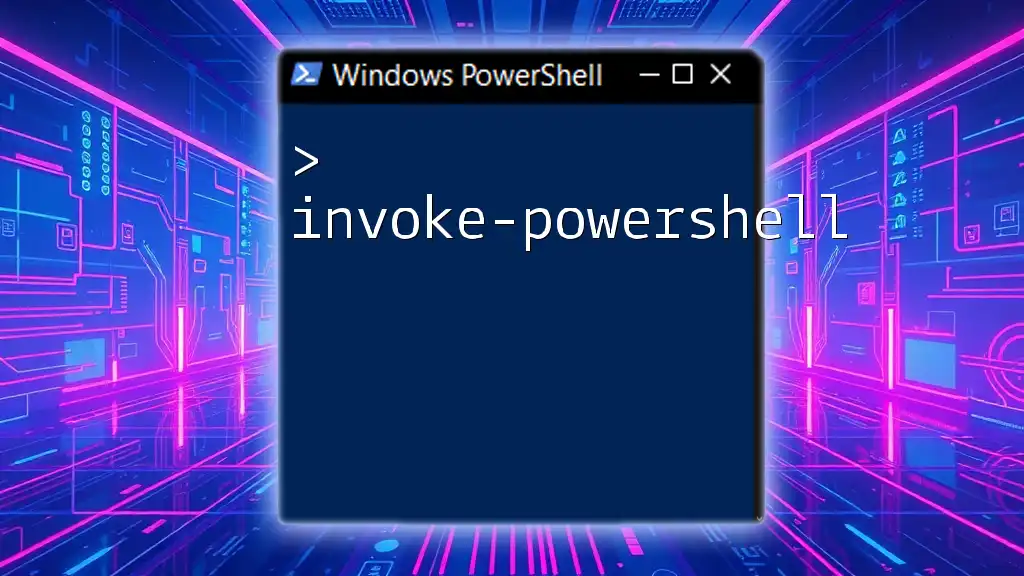
String Length in Action: Examples and Use Cases
Validating Input Length
Input validation is a critical aspect of user experience and security. Ensuring that string inputs meet specific criteria can prevent errors and potential attacks (e.g., SQL Injection).
param (
[string]$username
)
if ($username.Length -lt 5) {
Write-Host "Username must be at least 5 characters long."
} else {
Write-Host "Username accepted."
}
In this example, the PowerShell script checks the length of the username provided. If it is less than five characters, it prompts the user for a valid username, demonstrating the importance of string length validation in your scripts.
Manipulating Strings Based on Length
You can manipulate strings based on their lengths to enhance functionality. For instance, if a string is longer than a specified length, you might want to trim it for display purposes.
$myString = "This is a string"
if ($myString.Length -gt 10) {
$myString = $myString.Substring(0, 10) + "..."
}
Write-Host $myString
In this snippet, if the string exceeds ten characters, it gets truncated and appended with ellipses. This strategy is effective for maintaining a clean and concise interface in your outputs.
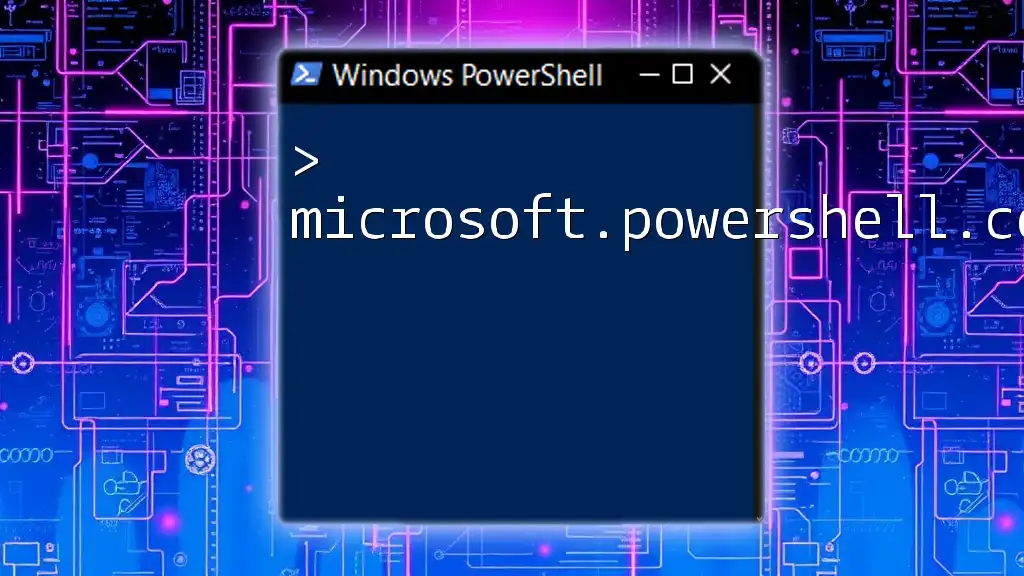
Advanced Techniques
String Length in Arrays
Sometimes you may need to calculate the total length of multiple strings stored in an array. Understanding how to manage string lengths in this manner can provide insight into data analytics or summarize information efficiently.
$stringsArray = @("One", "Two", "Three", "Four")
$totalLength = ($stringsArray | Measure-Object -Character).Characters
Write-Host "Total length of all strings in the array: $totalLength"
This snippet demonstrates how to calculate the cumulative length of strings in an array by using `Measure-Object`, reinforcing the power of PowerShell in handling collections.
Performance Considerations
While checking string length is generally fast, performance can become an issue when handling very large strings or large arrays of strings. Always assess whether you are using the most efficient method for your specific case—especially in scripts that require high performance or run frequently.
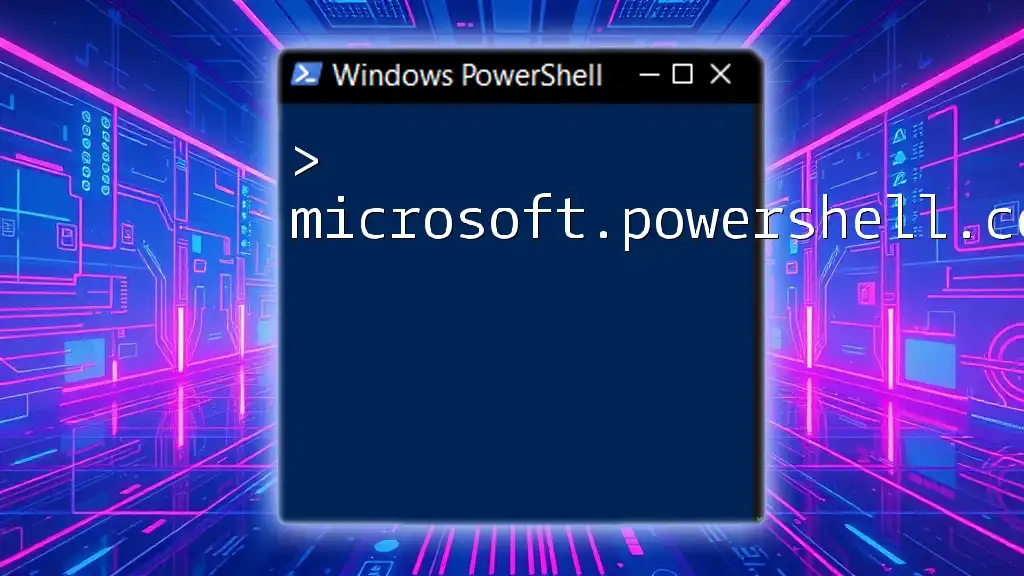
Conclusion
Summary of PowerShell String Length Techniques
Understanding how to work with string length in PowerShell is vital for effective scripting and programming. From basic properties like `.Length` to using cmdlets like `Measure-Object`, you have powerful tools at your disposal. Validating and manipulating string lengths enables you to create more robust, user-friendly scripts.
Call to Action
Practice these techniques by applying them in small projects. The more comfortable you are with these concepts, the more confident you'll become in scripting with PowerShell. Check out additional resources and communities for further exploration of these concepts!
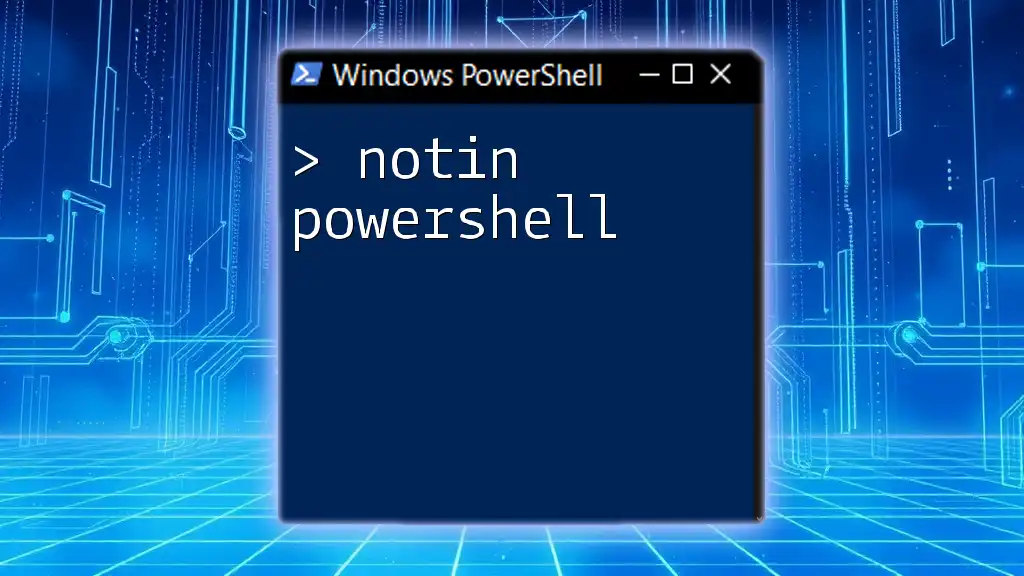
Additional Resources
Recommended Reading
Visit the official PowerShell documentation for in-depth explanations of string handling and properties.
Practice Exercises
Engage with exercises designed to reinforce your grasp of counting and manipulating string lengths in various scenarios. The best way to learn is through practice!