To locate a specific string within files or variables using PowerShell, you can utilize the `Select-String` cmdlet, as shown in the example below:
Select-String -Path "C:\path\to\your\file.txt" -Pattern "yourString"
Understanding Strings in PowerShell
What is a String?
A string in PowerShell is a sequence of characters enclosed in single or double quotes. Strings can be used to represent text, paths, user inputs, and much more. Understanding how to effectively manipulate strings is crucial for any PowerShell user.
For example:
$greeting = "Hello, World!"
Common Use Cases
Strings are used in a wide variety of scenarios within PowerShell:
- Script generation: Dynamically creating scripts with designated values.
- Text processing: Modifying and analyzing content from files or user inputs.
- Configurations and parameters: Specifying settings or parameters in PowerShell modules.
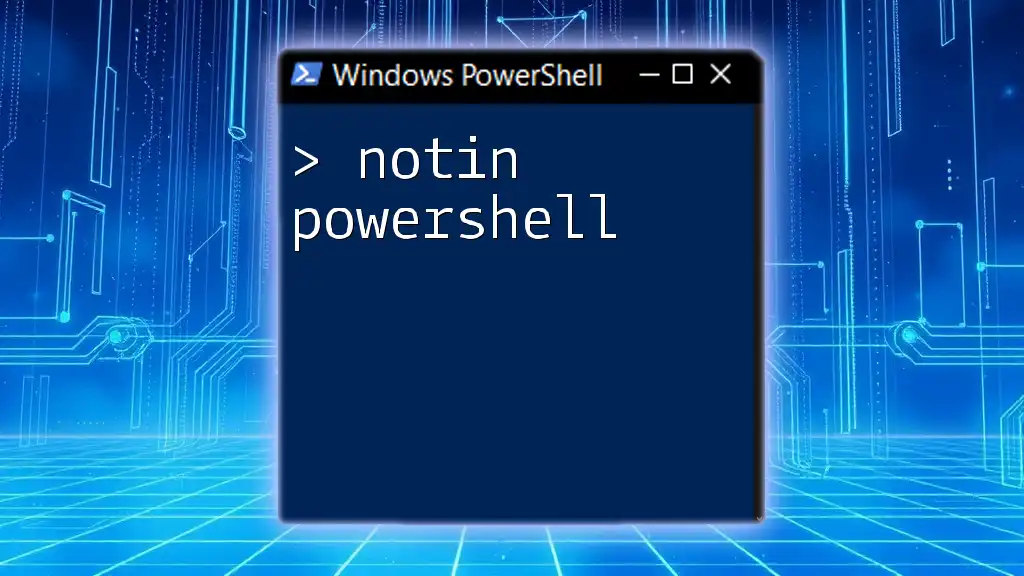
Finding Strings in PowerShell
Basic String Search with `-like` Operator
The `-like` operator is one of the simplest ways to search for a string pattern within another string. It works with wildcards, where the asterisk (*) represents zero or more characters.
Example:
$text = "Hello, World!"
$result = $text -like "*World*"
Write-Output $result # Output: True
In this example, the output is `True`, indicating that the substring "World" exists within `$text`.
Using the `-match` Operator for Regex Search
For more complex string searches, the `-match` operator leverages regular expressions (regex). Regular expressions are powerful tools for pattern matching and can be used to find strings that fit specific patterns.
Example of Using `-match`:
$text = "Hello, PowerShell"
if ($text -match "Power.*") {
Write-Output "Match Found!"
}
In this example, the regex pattern `"Power.*"` successfully matches "PowerShell".
Using Capture Groups in Regex: Capture groups in regex allow you to extract specific portions of a matched string.
Here’s how to do it:
if ($text -match "Power(.*)") {
$matchedString = $matches[1]
Write-Output "Matched Part: $matchedString"
}
In this case, `$matches[1]` stores "Shell," which is retrieved from the matched string.
`Select-String` Cmdlet for File and Input Search
The `Select-String` cmdlet is a versatile command for searching through files and streams of text. It can handle file content and pipeline input efficiently.
What is `Select-String`?
- It can search through each line of a file and return lines that match a specific pattern.
Basic Usage Example:
Get-Content "sample.txt" | Select-String -Pattern "Example"
This command retrieves all lines from `sample.txt` containing the word "Example".
Advanced Usage with Flags: You can modify the behavior of `Select-String` using various flags, such as making the search case-sensitive or searching for whole words.
Example:
Get-Content "sample.txt" | Select-String -Pattern "Example" -CaseSensitive
In this example, only lines containing "Example" with the exact casing will be matched.
Searching Multiple Files and Directories
To search through several files in a directory, you can use `Select-String` with wildcard patterns.
Example:
Select-String -Path "C:\MyFolder\*" -Pattern "SearchTerm"
This command searches for "SearchTerm" in all files located in the folder "C:\MyFolder".
Filtering Results: Sometimes, you may want to refine search results. You can pipe the output to `Where-Object` for further filtering.
Example:
Select-String -Path "C:\MyFolder\*" -Pattern "SearchTerm" | Where-Object { $_.Line -like "*specific condition*" }
With this command, you can further filter the results based on additional conditions.
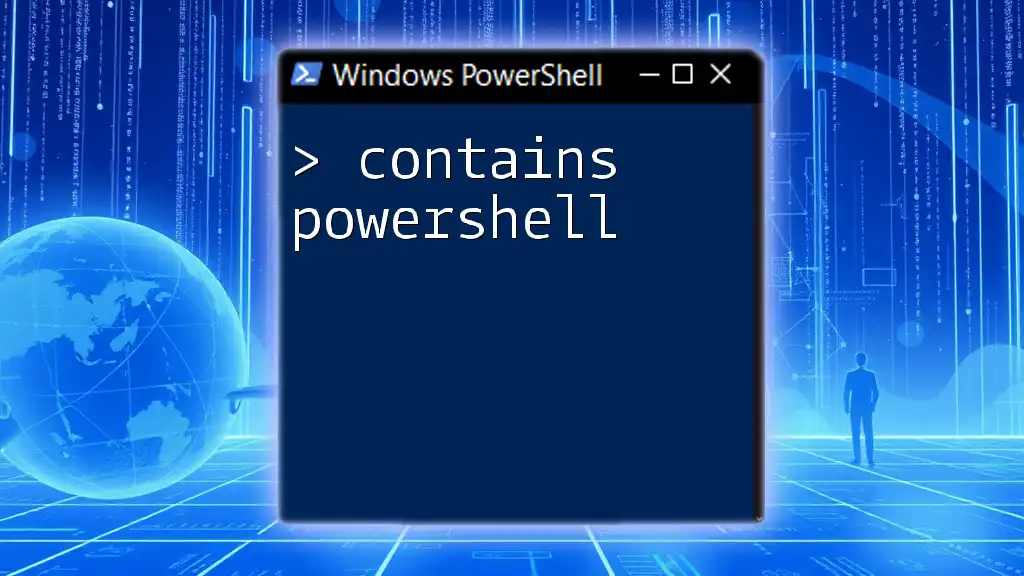
String Replacement Techniques
Using `-replace` Operator
The `-replace` operator allows you to replace occurrences of a substring with a new string. This operator also uses regular expressions, making it quite powerful.
Example of Replacing Strings:
$text = "This is a test."
$newText = $text -replace "test", "string"
Write-Output $newText # Output: "This is a string."
In this case, "test" is replaced with "string," demonstrating how easy it is to modify strings in PowerShell.
Using `Select-String` for Incremental Replacements
You can also utilize `Select-String` for string replacements by combining it with a loop structure.
Example:
Get-Content "sample.txt" |
ForEach-Object { $_ -replace "oldString", "newString" } |
Set-Content "updated_sample.txt"
This command reads `sample.txt`, replaces every instance of "oldString" with "newString," and saves the modified content to `updated_sample.txt`.
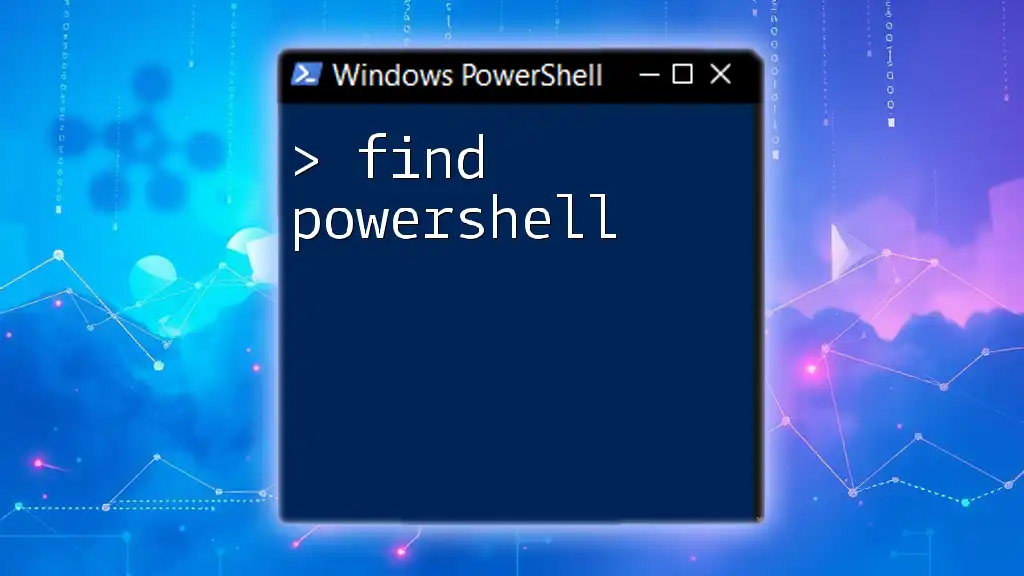
Performance Considerations
Understanding the performance implications of different string search techniques is key. Using operators like `-like` and `-match` can be efficient for smaller datasets, while `Select-String` shines when dealing with large files or multiple files due to its pipeline support.
When to Choose Each Method
- Use `-like` for simple pattern matches requiring wildcards.
- Use `-match` for complex regex needs.
- Use `Select-String` for searching through texts in files or outputs from other commands.
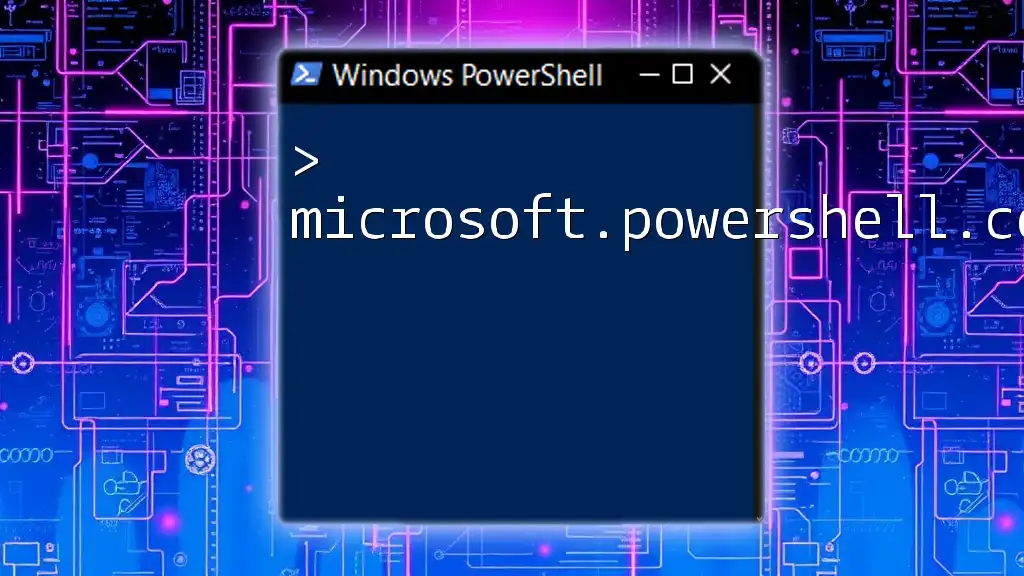
Conclusion
In this guide, we explored the various ways to find strings in PowerShell using different operators and cmdlets. By now, you should have a comprehensive understanding of string searching techniques, allowing you to manipulate text efficiently in your scripts.
Remember to practice these techniques in your own projects, and don’t hesitate to share your experiences or questions about string manipulation in PowerShell! Happy scripting!
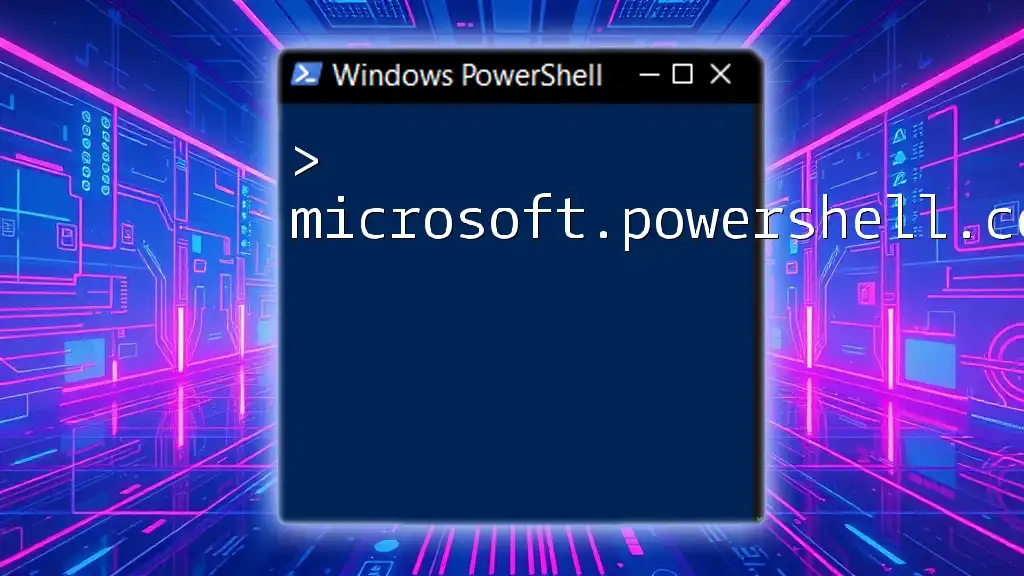
Additional Resources
For further learning:
- Explore the [PowerShell documentation](https://docs.microsoft.com/en-us/powershell/)
- Check out forums and communities for shared knowledge and support
- Consider delving into advanced PowerShell courses for deeper expertise in string manipulation and beyond.