To add a domain user to the local administrator group using PowerShell, you can execute the following command:
Add-LocalGroupMember -Group "Administrators" -Member "DOMAIN\UserName"
Replace "DOMAIN\UserName" with the actual domain and username of the user you want to add.
Understanding Local Admin Groups in Windows
What is a Local Administrator Group?
A Local Administrator Group is a group assigned specific permissions on a local computer. Members of this group have heightened levels of control, allowing them to install software, change system settings, and manage user accounts, among other tasks. The group plays a critical role in system administration, ensuring that trusted users can perform essential functions without compromising the system.
Why Add Domain Users to Local Admin Group?
Granting domain users access to the Local Administrator Group can be crucial in various situations, such as IT support scenarios where remote management is required, or when users need to run applications that demand administrative privileges.
By using PowerShell to manage this process, administrators benefit from streamlined scripting capabilities and greater automation potential, which is invaluable in a professional environment.
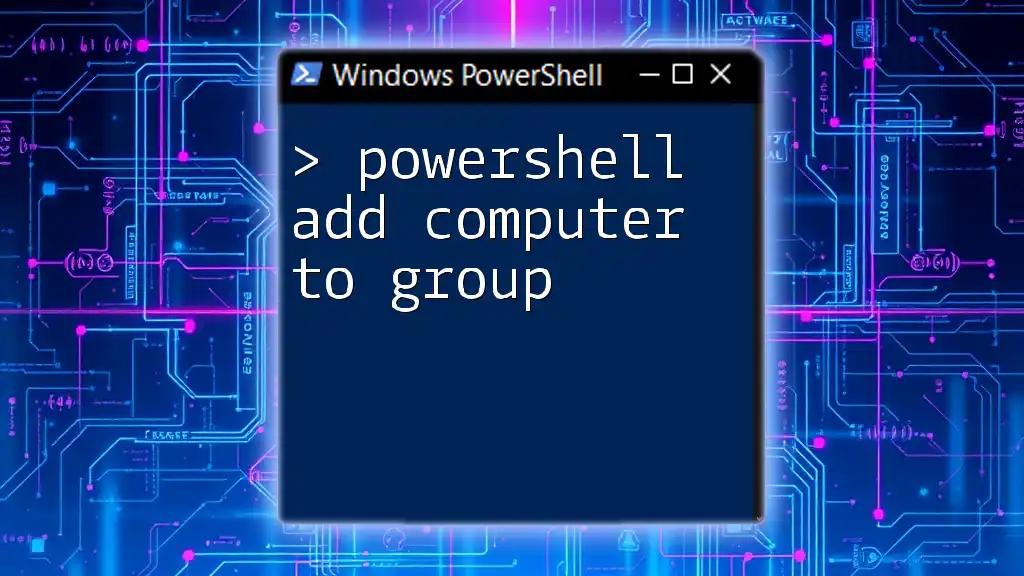
Prerequisites
Basic PowerShell Knowledge
Before proceeding with the commands, a foundational understanding of PowerShell cmdlets and basic scripting is necessary. Familiarity with concepts like objects, parameters, and cmdlet syntax will help ensure a smoother learning experience.
Administrative Privileges Required
To execute the commands that add users to the local admin group, administrative permissions on the target machine are necessary. Ensure that you have permission to make these changes; otherwise, you may encounter access errors.
Environment Setup
It's essential to have a system configured for executing both PowerShell and Active Directory commands. Make sure your PowerShell version supports the required features, ideally PowerShell 5.1 or later.
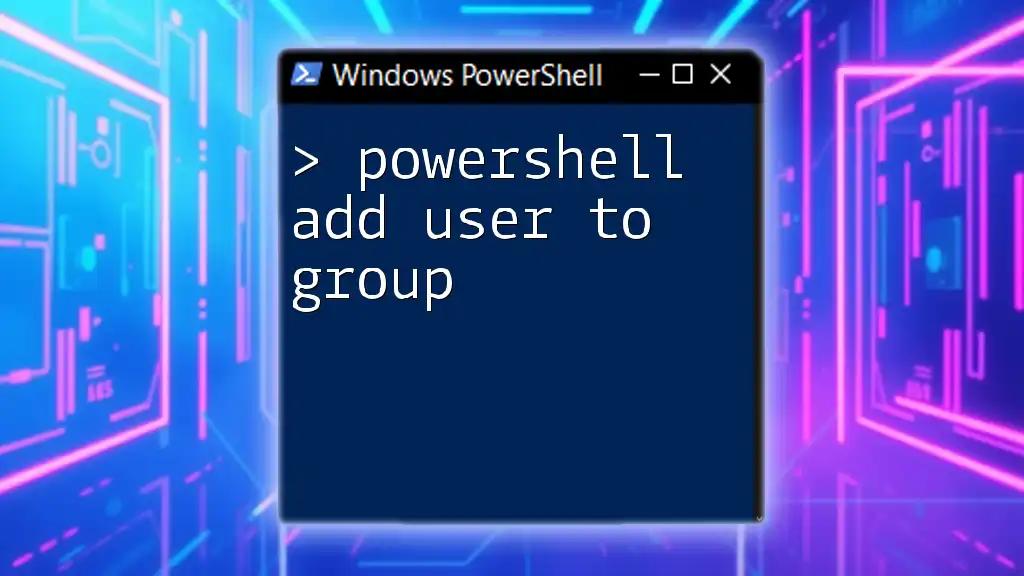
PowerShell Commands to Add Domain Users to Local Admin Group
Using the `Add-LocalGroupMember` Cmdlet
One of the most straightforward methods to add a domain user to the Local Administrator Group is through the `Add-LocalGroupMember` cmdlet. This cmdlet is part of the Microsoft.PowerShell.LocalAccounts module and allows for adding users with specific roles easily.
Syntax and Example:
The basic syntax for the `Add-LocalGroupMember` cmdlet is as follows:
Add-LocalGroupMember -Group "Administrators" -Member "DOMAIN\Username"
- `-Group` specifies which group you want to add the user to.
- `-Member` is the user you're adding. Make sure to format it correctly as `DOMAIN\Username`.
Using the cmdlet, you could effectively add a user called John from the domain named "MyDomain" with the following command:
Add-LocalGroupMember -Group "Administrators" -Member "MyDomain\John"
Using `net localgroup` Command
For those familiar with traditional command-line methods, you can opt for the `net localgroup` command. This approach can be useful when scripting or in environments where PowerShell is not available.
Syntax and Example:
Here’s how you would use `net localgroup` to achieve the same result:
net localgroup Administrators DOMAIN\Username /add
While both methods accomplish the same goal, `Add-LocalGroupMember` is generally preferred for its ease of use within PowerShell scripts.
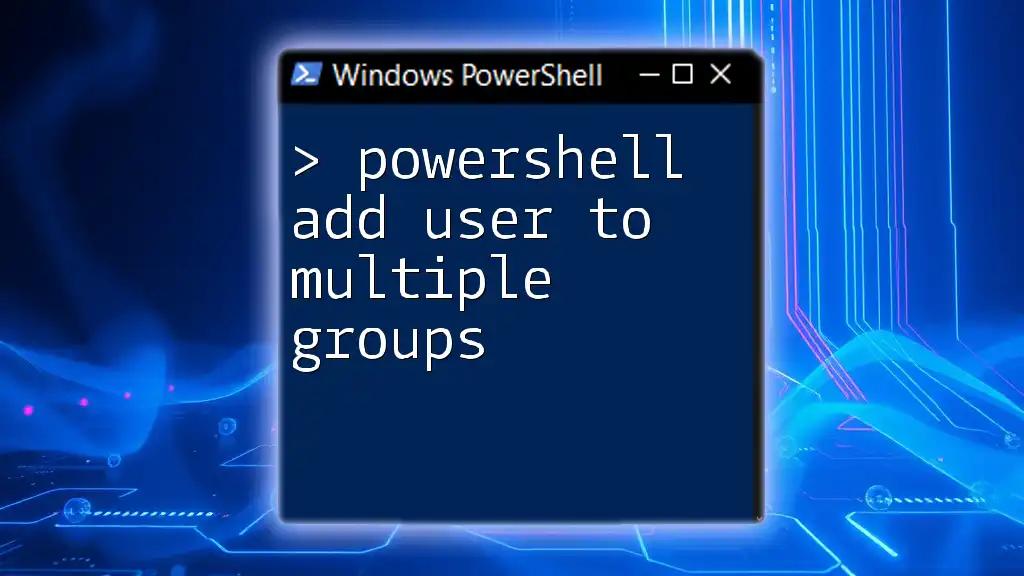
Verifying the Changes
How to Check Local Group Membership
After adding a user to the Local Administrator Group, it's essential to verify that the addition was successful. You can utilize the `Get-LocalGroupMember` cmdlet to view the members of the local group.
Example:
To check if your user John was added successfully, use the following command:
Get-LocalGroupMember -Group "Administrators"
This command will return a list of all users in the Administrators group. If John is listed, then the user was added correctly.

Troubleshooting Common Issues
Insufficient Permissions Error
If you encounter an error indicating insufficient permissions, ensure that you are executing the PowerShell with administrative privileges. Running PowerShell as an administrator will provide the necessary permissions.
User Not Found Error
If you receive a message stating that the user was not found, double-check the username and domain. Verify that the user account indeed exists in Active Directory, and confirm that the format is correct.
Local Group Limitations
Be aware of limitations on the number of users that can be added to the local group. While not commonly encountered, some systems might impose restrictions that could hinder your ability to add users.

Additional Tips for PowerShell Users
Batch Adding Users
In scenarios where multiple users need local admin privileges, you can automate the process. Here’s how you can batch add several users at once:
$users = "DOMAIN\User1", "DOMAIN\User2"
foreach ($user in $users) {
Add-LocalGroupMember -Group "Administrators" -Member $user
}
This approach ensures efficiency by processing the membership additions in one go, reducing manual efforts.
Scripting for Automation
Creating reusable scripts can save valuable time in a busy IT environment. Structure your PowerShell script to allow easy modifications as organizational needs change. An example structure could be:
$administrators = @("DOMAIN\User3", "DOMAIN\User4")
foreach ($admin in $administrators) {
if (-not (Get-LocalGroupMember -Group "Administrators" | Where-Object { $_.Name -eq $admin })) {
Add-LocalGroupMember -Group "Administrators" -Member $admin
}
}
This script checks if the users are already part of the group before attempting to add them, thus minimizing errors.
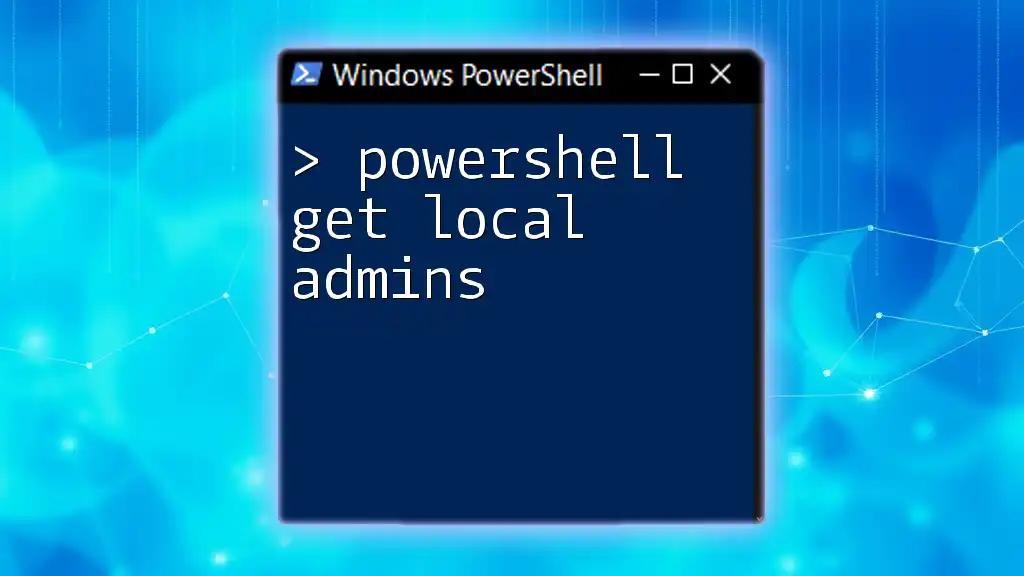
Conclusion
Managing local admin privileges through PowerShell is a powerful method to ensure streamlined operations and enhanced security. By utilizing the `Add-LocalGroupMember` cmdlet or `net localgroup`, system administrators can efficiently assign necessary permissions while avoiding the pitfalls of manual user management.
The skills learned here will serve as a foundation for further exploration into PowerShell, unlocking a wealth of automation and productivity opportunities.

Call to Action
Now that you have insight into how to use PowerShell to add domain users to the local admin group, consider signing up for our newsletters or courses to deepen your knowledge and explore more PowerShell functionalities. Happy scripting!