To create a self-signed certificate in PowerShell, you can use the `New-SelfSignedCertificate` cmdlet, which allows you to easily generate a certificate for testing or development purposes.
New-SelfSignedCertificate -DnsName "www.example.com" -CertStoreLocation "cert:\LocalMachine\My"
Understanding Self-Signed Certificates
A self-signed certificate is a digital certificate that is signed by the person or organization creating it, rather than a Certificate Authority (CA). These certificates are essential in scenarios where secure communications are needed within controlled environments. They are commonly used in development and testing environments, for example, to enable SSL/TLS on local servers or for securing API communications.
Self-signed certificates differ significantly from those issued by CAs. While CA-issued certificates are trusted because they are validated by a recognized authority, self-signed certificates inherently lack this level of trust. Thus, they are useful for internal purposes where trust can be managed directly.
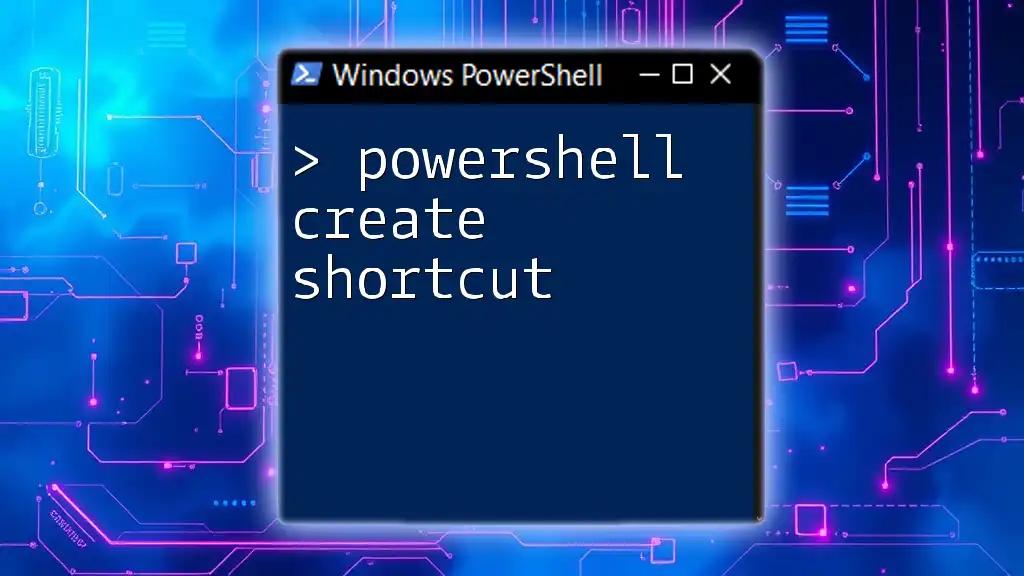
Setting Up PowerShell for Certificate Management
Before diving into creating a self-signed certificate, ensure your PowerShell is set up correctly. The `New-SelfSignedCertificate` cmdlet is available in PowerShell 5.0 and later, which comes pre-installed on modern versions of Windows.
You might need to verify your environment's settings, particularly around script execution. To allow scripts to run, execute the following command:
Set-ExecutionPolicy RemoteSigned
This command will enable your PowerShell session to run local scripts that you create while allowing downloaded scripts to require validation.
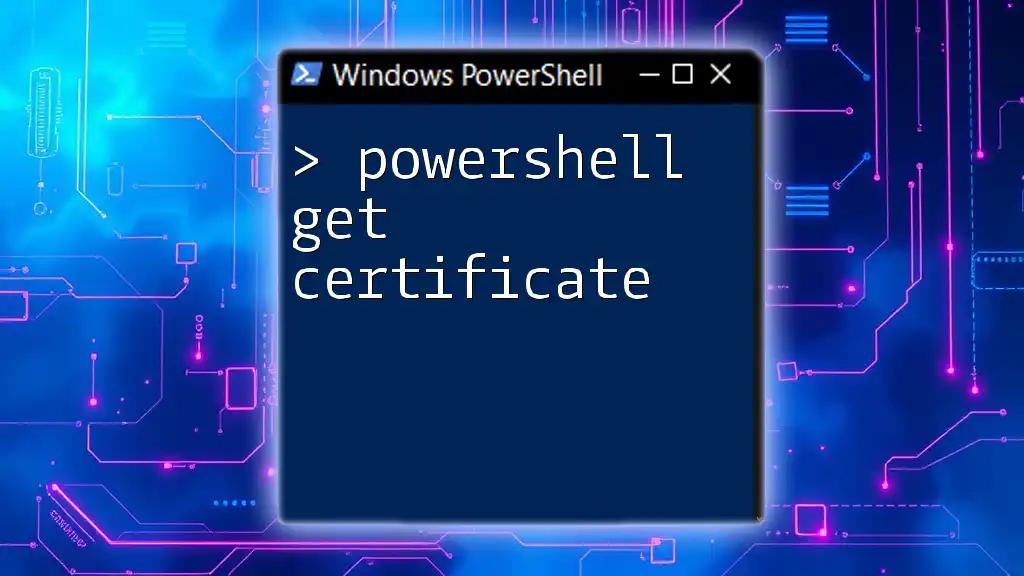
Creating a Self-Signed Certificate with PowerShell
Introduction to the `New-SelfSignedCertificate` Cmdlet
The `New-SelfSignedCertificate` cmdlet is a straightforward way to create self-signed certificates. It provides a variety of options to customize the certificate based on your needs, making it an essential command for system administrators and developers alike.
Basic Syntax and Parameters
The general syntax for the cmdlet is as follows:
New-SelfSignedCertificate -DnsName "<DNS Name>" -CertStoreLocation "<Store Location>"
Key parameters include:
- `-DnsName`: Specifies the DNS name for the certificate. This could be a domain name or IP address.
- `-CertStoreLocation`: Indicates where to store the certificate in your system, such as "Cert:\LocalMachine\My".
- `-KeyLength`: Defines the strength of the encryption used in the certificate. Common values include 2048 or 4096 bits.
- `-NotAfter`: Sets the expiration date for the certificate, which is crucial for security reasons.
Example 1: Basic Self-Signed Certificate
To create a basic self-signed certificate, you can use the following command:
$cert = New-SelfSignedCertificate -DnsName "example.com" -CertStoreLocation "Cert:\LocalMachine\My"
In this example, a self-signed certificate is created with the DNS name "example.com," and it is stored in the local machine's certificate store under the "My" location. After executing this command, you can verify the certificate's presence in the certificate manager.
Example 2: Self-Signed Certificate with Custom Parameters
You can customize the certificate further by adding additional parameters like key length and expiration:
$cert = New-SelfSignedCertificate -DnsName "test.local" -CertStoreLocation "Cert:\LocalMachine\My" -KeyLength 2048 -NotAfter (Get-Date).AddYears(5)
In this example:
- The `-KeyLength 2048` specifies that the certificate will use 2048-bit encryption.
- The `-NotAfter (Get-Date).AddYears(5)` indicates that the certificate will expire five years from its creation date. Customizing the expiration helps to manage security policies effectively, especially when certificates must be renewed regularly.
Example 3: Creating a Self-Signed Certificate for Code Signing
In scenarios where you need to sign scripts or executables, you can create a self-signed certificate specifically intended for code signing:
$cert = New-SelfSignedCertificate -DnsName "code.signing" -CertStoreLocation "Cert:\LocalMachine\My" -KeyUsage DigitalSignature
Here, the `-KeyUsage DigitalSignature` parameter is critical. This specifies that the certificate is for digital signatures, which helps enhance trust when signing software or scripts.
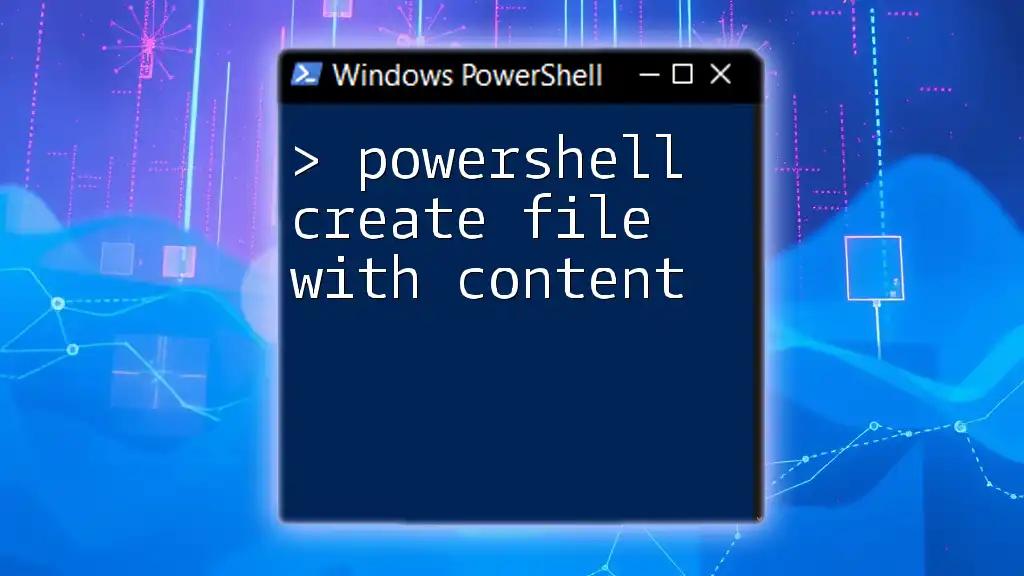
Verifying the Self-Signed Certificate
Once you have created a self-signed certificate, it’s important to verify its existence and details. You can do so by executing the following command:
Get-ChildItem -Path Cert:\LocalMachine\My
This command lists all the certificates in the specified certificate store. You can search the output for your newly created certificate, ensuring it has the intended properties and is stored correctly.
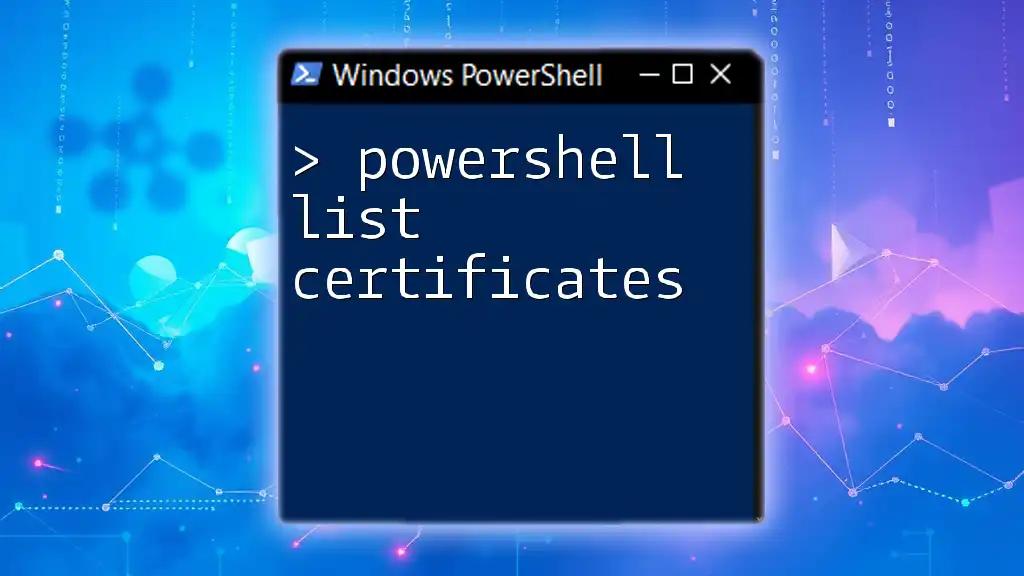
Exporting the Self-Signed Certificate
After creating and verifying your self-signed certificate, you may want to export it for use on other systems or within applications. Here is how you can export the certificate to a `.pfx` file:
Export-PfxCertificate -Cert $cert -FilePath "C:\Path\To\Your\Certificate.pfx" -Password (ConvertTo-SecureString -String "yourpassword" -Force -AsPlainText)
In this command:
- The `-FilePath` specifies where to save the exported certificate.
- The `-Password` parameter ensures that the exported certificate is protected with a password, which is essential for maintaining security.
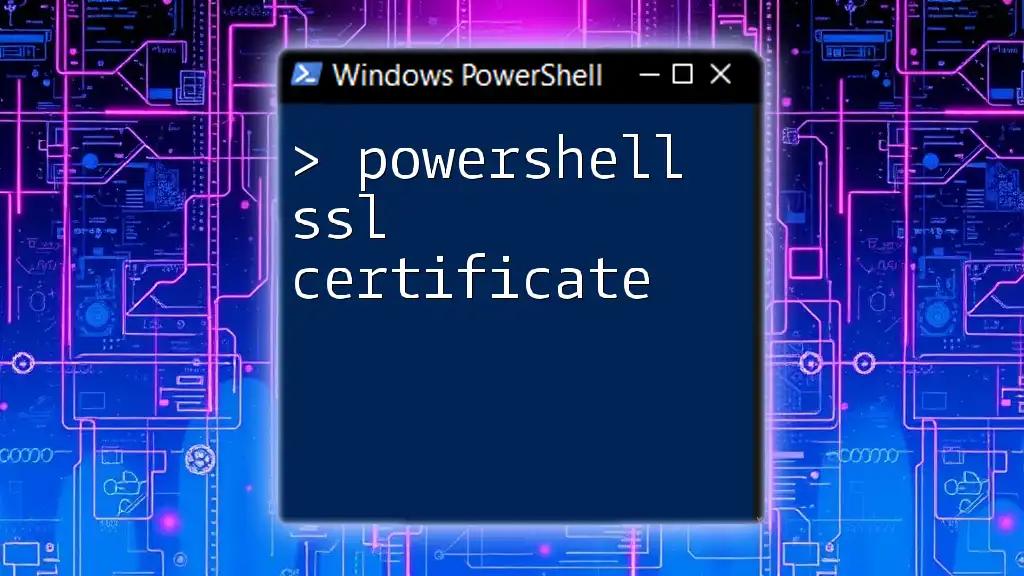
Using Self-Signed Certificates
Once you have your self-signed certificate ready, you may need to install it on client machines or configure applications to use it. To manage trusted certificates on client machines, you can use Microsoft Management Console (MMC) to add the certificate to the 'Trusted Root Certification Authorities' store.
For configurations, applications such as IIS, Apache, or any API service can be set up to utilize the self-signed certificate for secure communication.
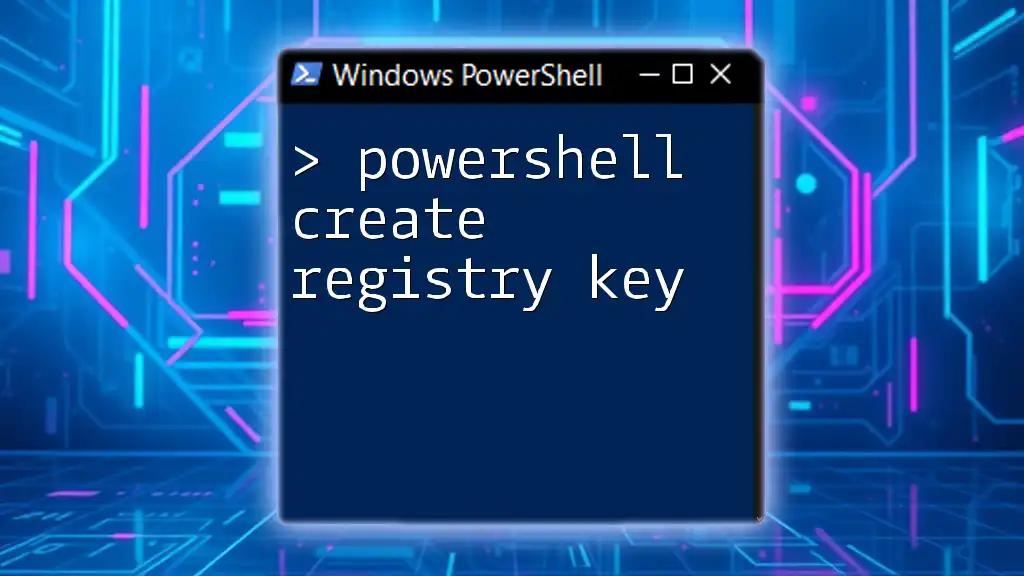
Best Practices for Using Self-Signed Certificates
While self-signed certificates are helpful, they come with certain limitations and considerations:
- Security Limitations: Because these certificates are not validated by a reputable CA, relying on them in a production environment poses security risks. Always ensure to monitor their use rigorously.
- Renewals: Regularly check the expiration dates on your self-signed certificates and establish a process for renewing them to avoid service disruptions.
- Use in Testing Only: It's recommended to reserve self-signed certificates for development or internal testing. For production environments, always opt for CA-issued certificates to ensure user trust.
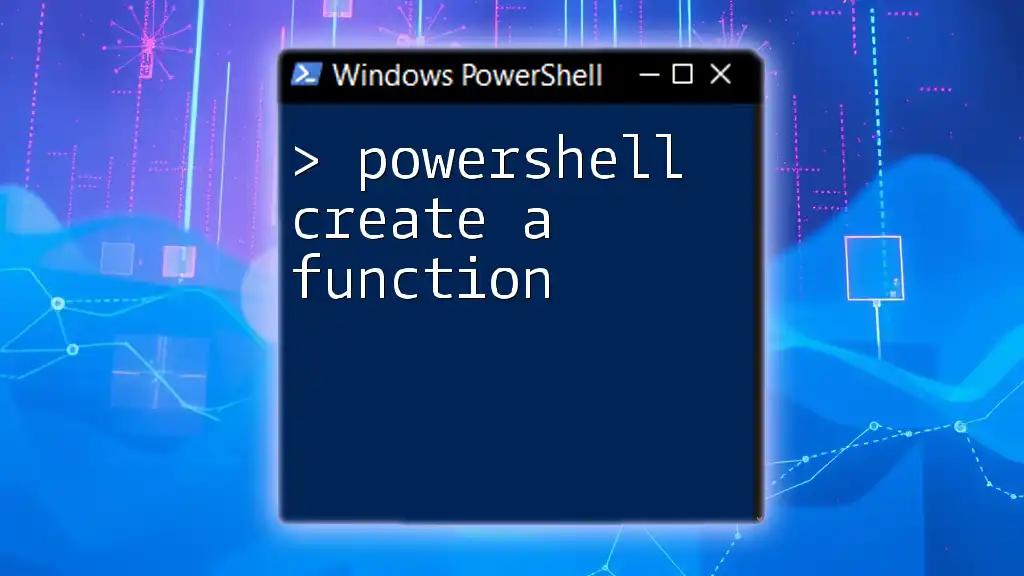
Conclusion
Creating a self-signed certificate in PowerShell is an invaluable skill for developers and IT professionals. With the `New-SelfSignedCertificate` cmdlet, you can efficiently generate certificates for various uses, enhancing your systems' security and functionality. Practice and explore the capabilities provided by PowerShell, and consider delving deeper into certificate management for better overall system security.
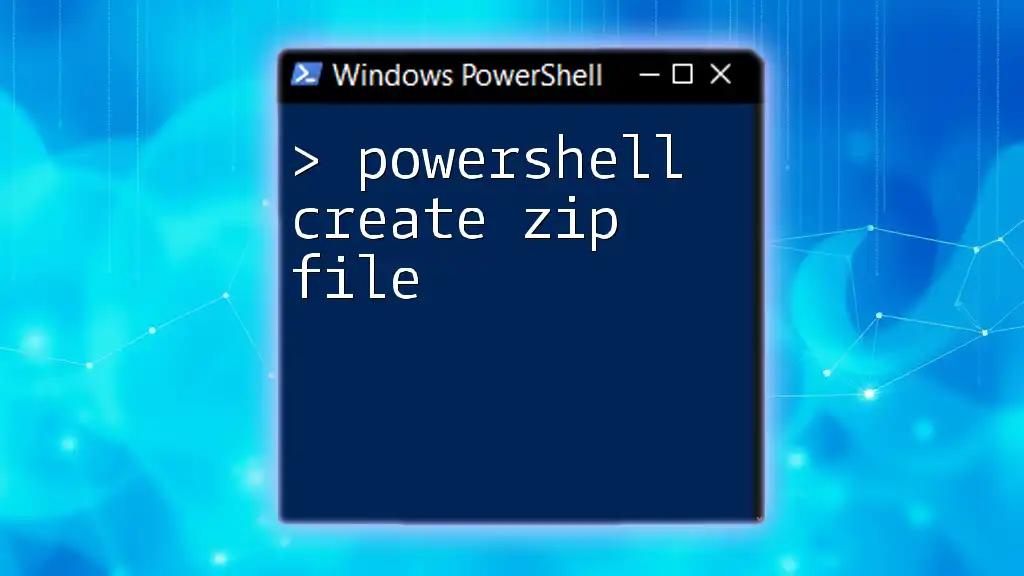
Further Resources
For more advanced implementation and management, consider referring to the official Microsoft documentation on `New-SelfSignedCertificate`. Additionally, numerous tutorials and informative articles are available online to expand your knowledge of PowerShell scripting and certificate practices.
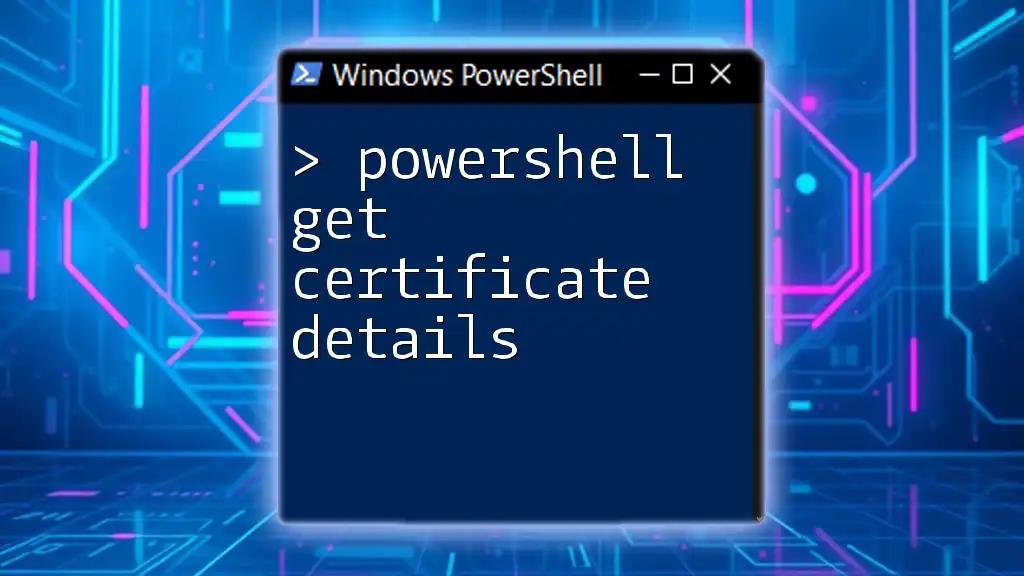
FAQ Section
Are self-signed certificates secure?
While self-signed certificates can encrypt data and provide a level of security, they lack the trusted validation from a CA, making them less reliable in public-facing scenarios.
When should I use a self-signed certificate vs. an official CA-issued certificate?
Self-signed certificates are best suited for testing and internal use where external trust isn't required. For production systems, always choose CA-issued certificates.
How do I delete a self-signed certificate?
To remove a self-signed certificate, locate it in the certificate store using `Get-ChildItem`, then use `Remove-Item` to delete it. Execute a command like this:
Remove-Item -Path "Cert:\LocalMachine\My\<Thumbprint>"
Just replace `<Thumbprint>` with the actual thumbprint of the certificate you wish to delete.