You can quickly test a SQL Server connection in PowerShell using the `System.Data.SqlClient.SqlConnection` class to ensure your application can connect to the database.
$connectionString = "Server=YOUR_SERVER_NAME;Database=YOUR_DATABASE_NAME;User Id=YOUR_USERNAME;Password=YOUR_PASSWORD;"
$connection = New-Object System.Data.SqlClient.SqlConnection $connectionString
try {
$connection.Open()
Write-Host "Connection successful!"
} catch {
Write-Host "Connection failed: $_"
} finally {
$connection.Close()
}
Understanding SQL Connections
What is an SQL Connection?
An SQL connection is a bridge between an application and a database that enables the application to execute SQL queries to manipulate and retrieve data. SQL connections are essential for various databases, including SQL Server, MySQL, and PostgreSQL. Establishing a reliable SQL connection is crucial for the performance and stability of applications that depend on database interactions.
Why Test SQL Connections?
Testing SQL connections is vital for several reasons:
- Performance: A successful connection ensures that your application can interact with the database efficiently.
- Troubleshooting: Diagnosing connection issues can save time during the development process and enhance user experience.
By regularly testing SQL connections, you can preemptively catch issues that could lead to application downtime or data access problems.
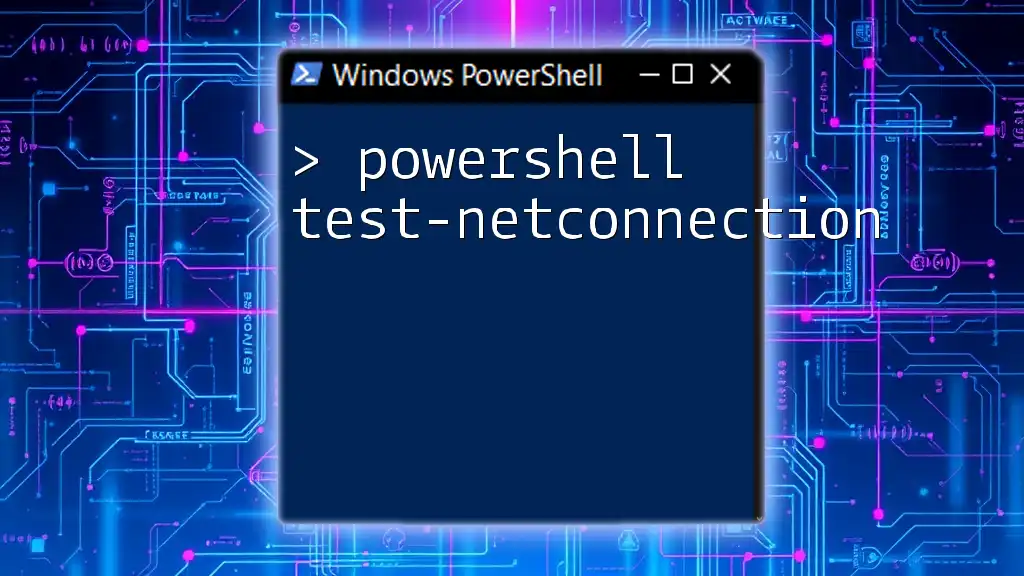
Preparing PowerShell for SQL Connection Testing
Required Modules and Tools
Before you can test SQL connections using PowerShell, you need to ensure that the necessary modules are installed. The most commonly used module is `SqlServer`, which provides various cmdlets for managing SQL Server instances.
To install this module, you can use the following command:
Install-Module SqlServer
Once installed, you can leverage its commands to interact with SQL databases effectively.
Permissions and Credentials
Proper permissions are essential when testing SQL connections. Ensure your user account has been granted appropriate roles on the SQL server, such as `db_datareader` or `db_datawriter`.
Additionally, managing credentials securely in PowerShell is critical. You can store credentials using `Get-Credential`, which prevents hardcoding sensitive information directly in your scripts.
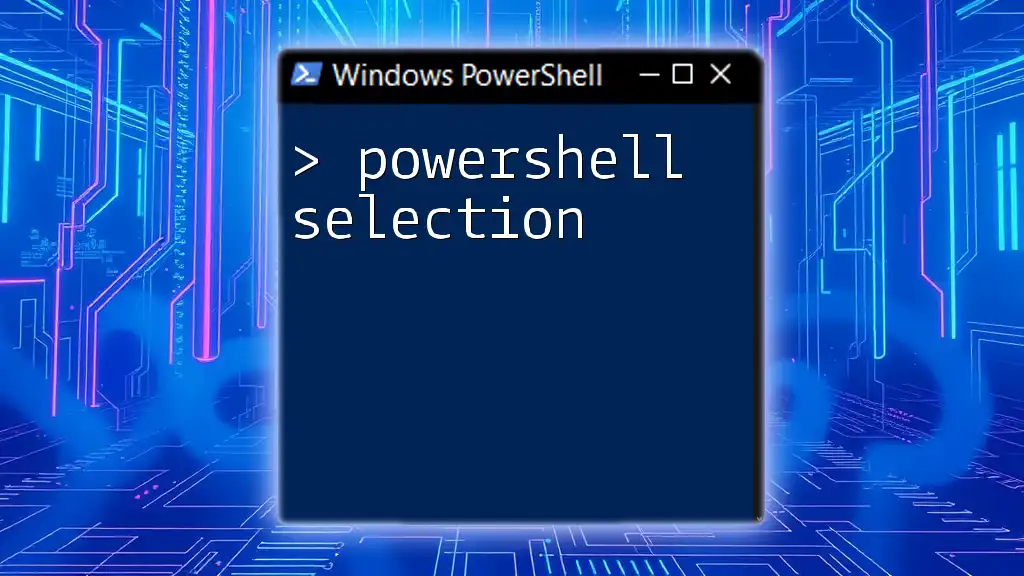
Methods to Test SQL Connection in PowerShell
Using `System.Data.SqlClient.SqlConnection`
One of the most straightforward methods to test an SQL connection is by using the .NET class `System.Data.SqlClient.SqlConnection`. This approach allows for fine control over the connection process.
Code Example
$connectionString = "Server=YourServer;Database=YourDatabase;User Id=YourUser;Password=YourPassword;"
$connection = New-Object System.Data.SqlClient.SqlConnection
$connection.ConnectionString = $connectionString
try {
$connection.Open()
Write-Host "Connection Successful" -ForegroundColor Green
} catch {
Write-Host "Connection Failed: $_" -ForegroundColor Red
} finally {
$connection.Close()
}
Here’s what each part does:
- $connectionString: Defines the connection string that contains vital information such as server address, database name, and credentials.
- New-Object: Creates a new instance of the `SqlConnection` class.
- $connection.Open(): Attempts to open the connection. If it's successful, you will receive a confirmation message.
By wrapping connection attempts in a `try-catch` block, you can handle exceptions gracefully and diagnose issues directly.
Using `Invoke-Sqlcmd`
Another powerful method is using `Invoke-Sqlcmd`, a cmdlet that runs T-SQL commands directly from PowerShell.
Code Example
Invoke-Sqlcmd -Query "SELECT 1" -ServerInstance "YourServer" -Database "YourDatabase" -Username "YourUser" -Password "YourPassword"
This command runs a simple SQL query that checks the connection to your SQL Server instance. If the connection is successful, it will return the query result.
Importance of Exception Handling
Error handling is critical when running scripts that connect to databases. Always wrap your code in `try-catch` blocks to manage exceptions appropriately. For example:
try {
Invoke-Sqlcmd -Query "SELECT 1" -ServerInstance "YourServer" -Database "YourDatabase" -Username "YourUser" -Password "YourPassword"
Write-Host "Connection Successful" -ForegroundColor Green
} catch {
Write-Host "An error occurred: $_" -ForegroundColor Red
}
This enhancement provides visibility into what went wrong if a connection attempt fails.
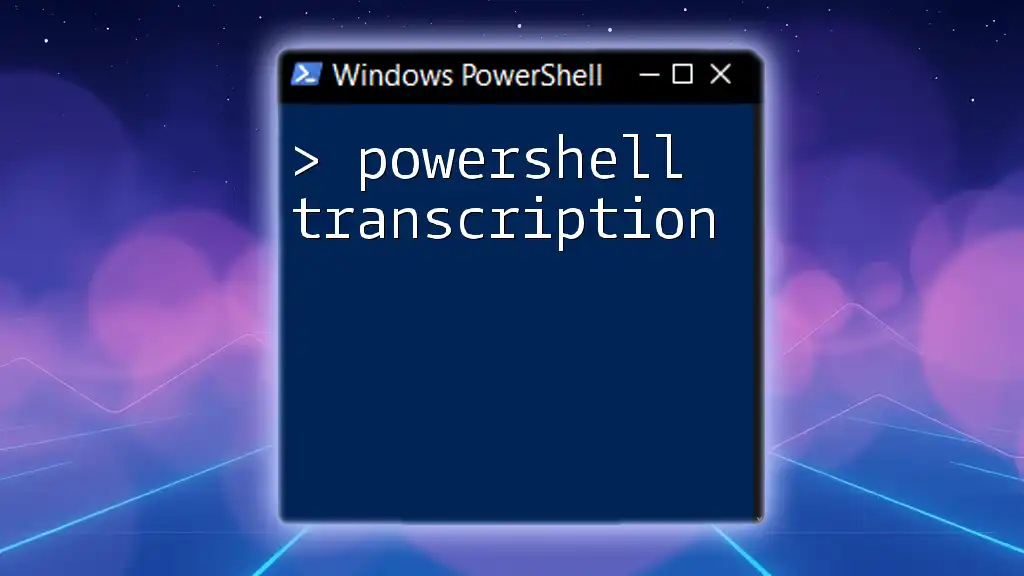
Verifying SQL Connection
Checking Connection Using Ping
It's also wise to verify that the SQL server is reachable over the network before testing the SQL connection itself. You can use the `Test-NetConnection` cmdlet for this purpose.
Code Example
Test-NetConnection -ComputerName "YourServer" -Port 1433 # Default SQL Server port
This command checks if the SQL Server is reachable and if the appropriate port is open. Confirming that the server is online can help eliminate potential problems early in the troubleshooting process.
Monitoring Performance
Monitoring the performance of SQL connections is essential, especially in production environments. Consider leveraging PowerShell's scheduled tasks to run connection tests at regular intervals. This proactive approach can help identify connectivity issues before they impact users.
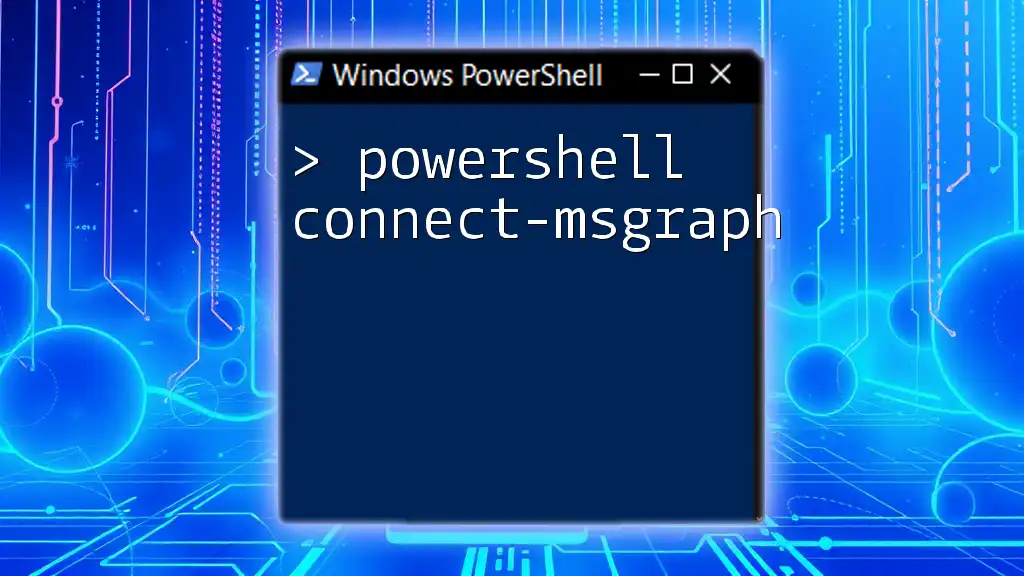
Common Issues and Troubleshooting
Connection Issues
Some common connection problems include:
- Incorrect Server Name: Ensure that the server name specified in your connection string is accurate.
- Authentication Errors: Double-check your usernames and passwords.
- Network Problems: Firewalls or network configurations can block database connections.
Network Configuration Problems
It's crucial to review firewall settings that might prevent communication with the SQL server. Sometimes, SQL Server may be configured to listen on a different port than the default (1433), which can lead to connectivity issues.
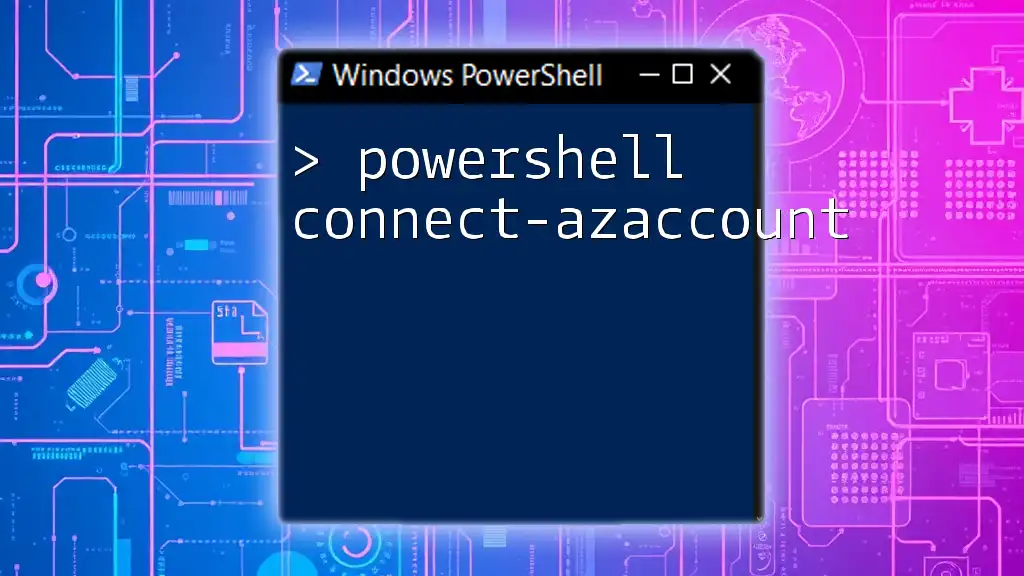
Best Practices for Testing SQL Connections
Writing Readable Code
Script maintainability is essential. Write comments to describe what your code does and keep your code organized to enhance readability. This approach makes it easier for you and others to revisit your scripts in the future.
Utilizing Variables for Ease of Use
Using variables makes your scripts more maintainable and gives you flexibility to change settings quickly. For example:
$server = "YourServer"
$database = "YourDatabase"
$username = "YourUser"
$password = "YourPassword"
# Connection Test Code Here
By defining your connection variables upfront, you can easily adjust them as needed.
Regular Testing
Integrate SQL connection testing into your routine tasks. Setting up a scheduled task in Windows or a cron job in Linux to run these tests can help ensure that your connections remain stable over time.
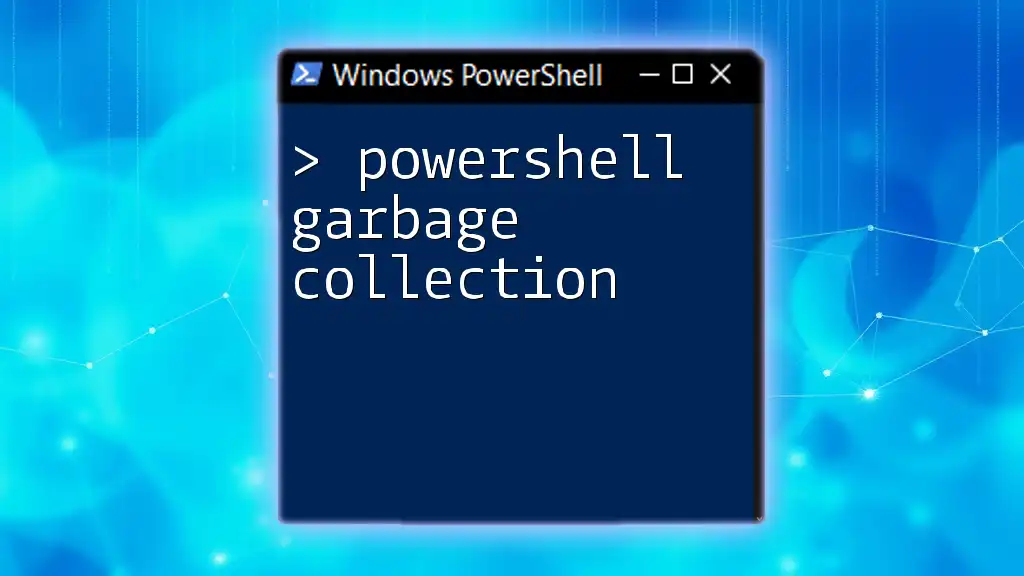
Conclusion
Testing SQL connections using PowerShell is not just essential for ensuring application performance but also vital for maintaining a reliable database environment. By using the methods outlined in this guide, you can fully leverage PowerShell’s capabilities to automate and simplify database management tasks. Regularly implementing these practices will help you catch potential issues before they affect your operations.
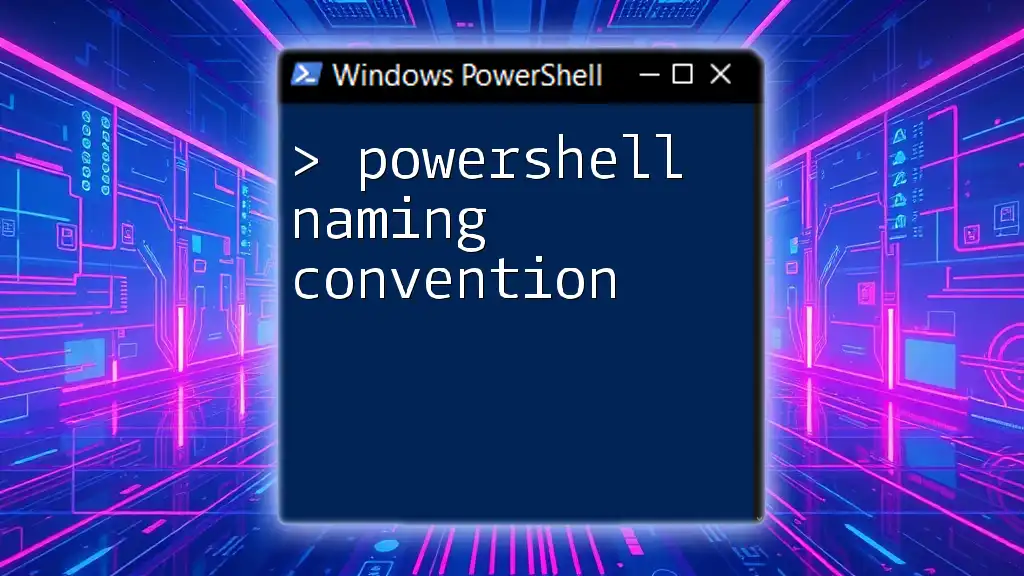
Additional Resources
For further reading, consider exploring official Microsoft documentation, PowerShell forums, and SQL Server community resources. Engaging with such materials can deepen your understanding and skills in managing SQL databases through PowerShell.
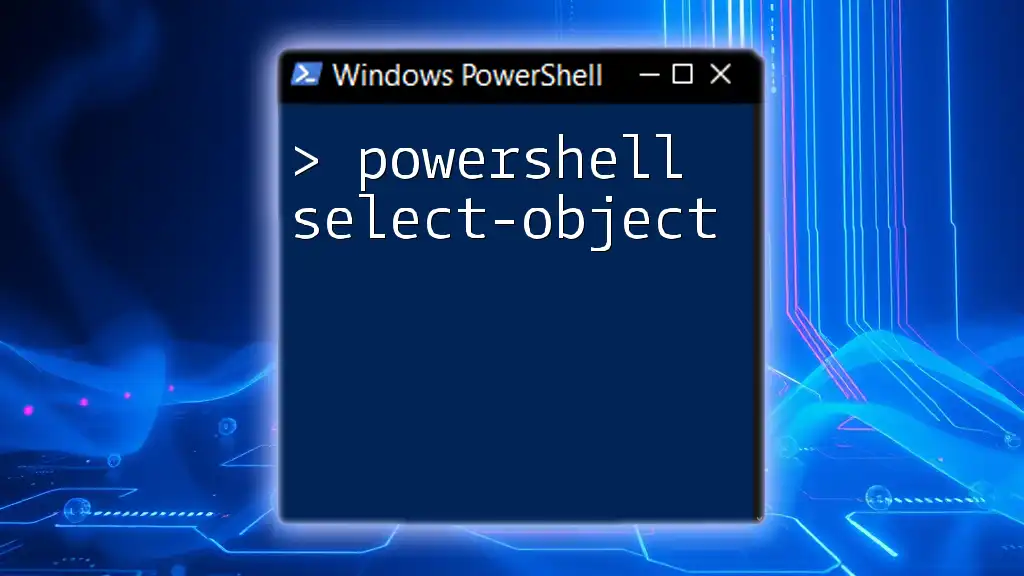
FAQs
Can I test SQL connection without a password?
Yes, if you are using Windows Authentication, you can omitting the username and password in the connection string.
How do I handle SQL connection strings in a secure manner?
Use secure methods such as PowerShell SecureString for managing credentials and avoid hardcoding sensitive information in your scripts.
What is the best command to test a SQL Server connection in PowerShell?
Both `System.Data.SqlClient.SqlConnection` and `Invoke-Sqlcmd` are excellent choices, but the method you choose should depend on your needs and the context. For a quick check, `Invoke-Sqlcmd` can be very efficient.