To create a shortcut with a custom icon using PowerShell, you can use the following code snippet:
$Shortcut = New-Object -ComObject WScript.Shell
$ShortcutPath = "$env:USERPROFILE\Desktop\MyShortcut.lnk"
$TargetPath = "C:\Path\To\Your\Application.exe"
$IconPath = "C:\Path\To\Your\Icon.ico"
$Shortcut = $Shortcut.CreateShortcut($ShortcutPath)
$Shortcut.TargetPath = $TargetPath
$Shortcut.IconLocation = $IconPath
$Shortcut.Save()
This script creates a desktop shortcut named "MyShortcut.lnk" for the specified application with a custom icon.
Understanding Shortcuts in Windows
What is a Shortcut?
A shortcut in Windows is a link that allows a user to quickly access a file, folder, or application without navigating through the file system. It typically appears as a small icon, often with an arrow in the corner, indicating that it links to another location. Shortcuts streamline workflow by placing frequently used items at your fingertips.
Why Use PowerShell for Creating Shortcuts?
Using PowerShell to create shortcuts provides several advantages. Automation is a significant benefit, especially for users managing multiple shortcuts or setting up new environments. PowerShell scripts can be run as batch processes, allowing for the creation of numerous shortcuts with minimal effort. Additionally, for system administrators and advanced users, PowerShell enables precise customization that can be cumbersome to achieve through the graphical user interface.
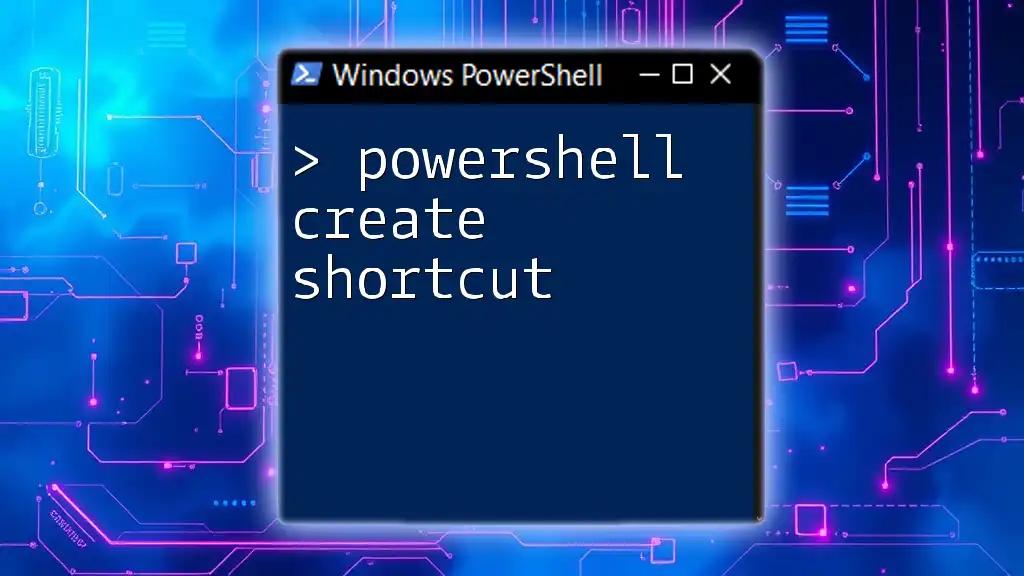
Getting Started with PowerShell
Opening PowerShell
To create a shortcut with PowerShell, the first step is to open PowerShell. You can do this by searching "PowerShell" in the Start menu or using the following paths depending on your Windows version:
- Windows 10 and 11: Right-click on the Start button and select "Windows PowerShell" or "Windows Terminal."
Setting Permissions
Before running scripts, it’s crucial to ensure you have the appropriate permissions. You can check your execution policy to make sure it allows script execution by running:
Get-ExecutionPolicy
If the policy is set to "Restricted," you may want to change it using the command:
Set-ExecutionPolicy RemoteSigned
This change allows local scripts to run, while required scripts from the internet must be signed.
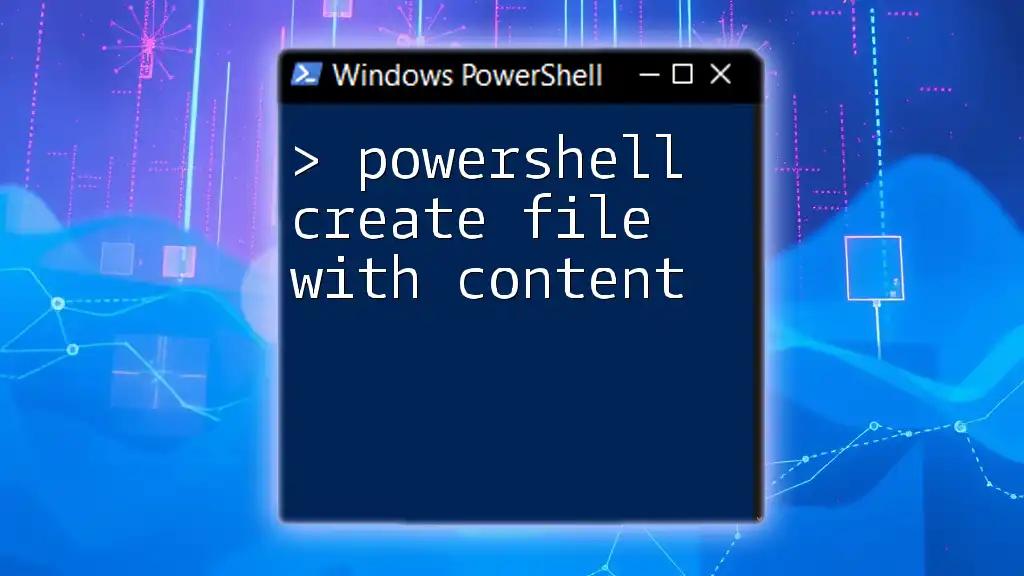
Creating a Shortcut with PowerShell
Basic Structure of a Shortcut
When creating a shortcut, it’s essential to understand its basic components. The following are the key attributes of a shortcut:
- Target Path: The file or application that the shortcut will point to.
- Working Directory: The folder from which the program runs. This is particularly important for applications that require relative file paths.
- Icon Location: The file path for the icon that will represent the shortcut.
- Shortcut Name: The name under which the shortcut will appear.
PowerShell Commands for Creating a Shortcut
PowerShell provides access to the `WScript.Shell` COM object, which handles shortcut creation. Here’s a basic example of creating a shortcut without an icon:
$ShortcutPath = "$env:USERPROFILE\Desktop\MyShortcut.lnk"
$TargetPath = "C:\Path\To\Your\Target.exe"
$WshShell = New-Object -ComObject WScript.Shell
$Shortcut = $WshShell.CreateShortcut($ShortcutPath)
$Shortcut.TargetPath = $TargetPath
$Shortcut.Save()
In this snippet, `MyShortcut.lnk` will be created on your desktop, pointing to the specified executable.
Adding an Icon to the Shortcut
Adding a custom icon to your shortcut is straightforward. Once you've initiated the shortcut object, you can specify an icon file by using the `IconLocation` property. Here’s how you can modify the previous example to include an icon:
$IconPath = "C:\Path\To\Your\Icon.ico"
$Shortcut.IconLocation = $IconPath
$Shortcut.Save()
By running this code, the newly created shortcut will now display the specified icon, enhancing the visual organization of your desktop or folder.
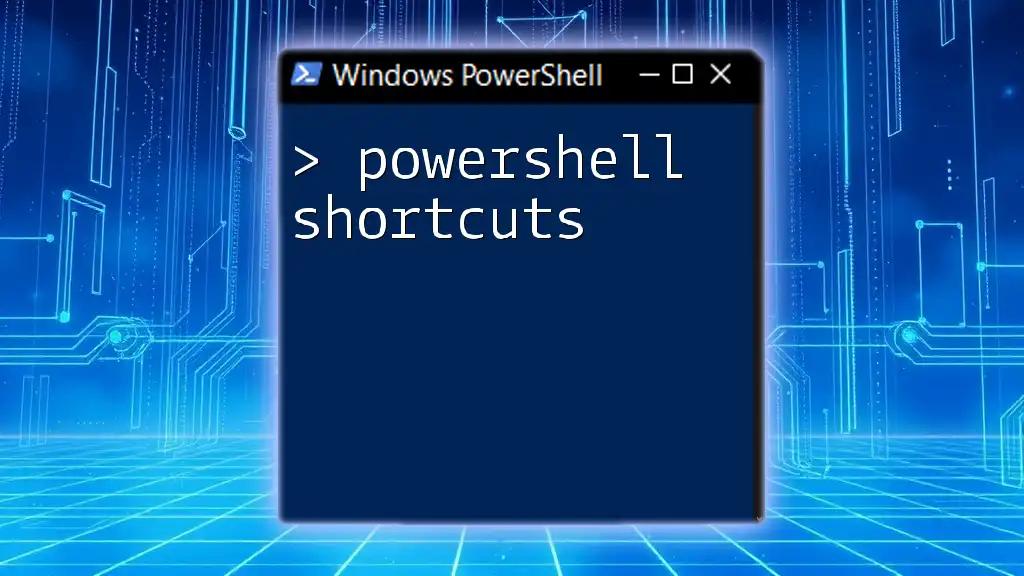
Advanced Shortcut Options
Setting Shortcut Properties
You can further customize your shortcuts by adjusting additional parameters like hotkeys and window styles. For example:
$Shortcut.Hotkey = "CTRL+ALT+M"
$Shortcut.WindowStyle = 1 # 1: Normal window, 2: Minimized, 3: Maximized
Here, the shortcut will launch with the specified hotkey combination and window style, providing an extra layer of functionality tailored to your preferences.
Exploring Parameters
Taking customization further, you can define where your program starts using the `StartIn` property. This allows the program to resolve relative paths correctly.
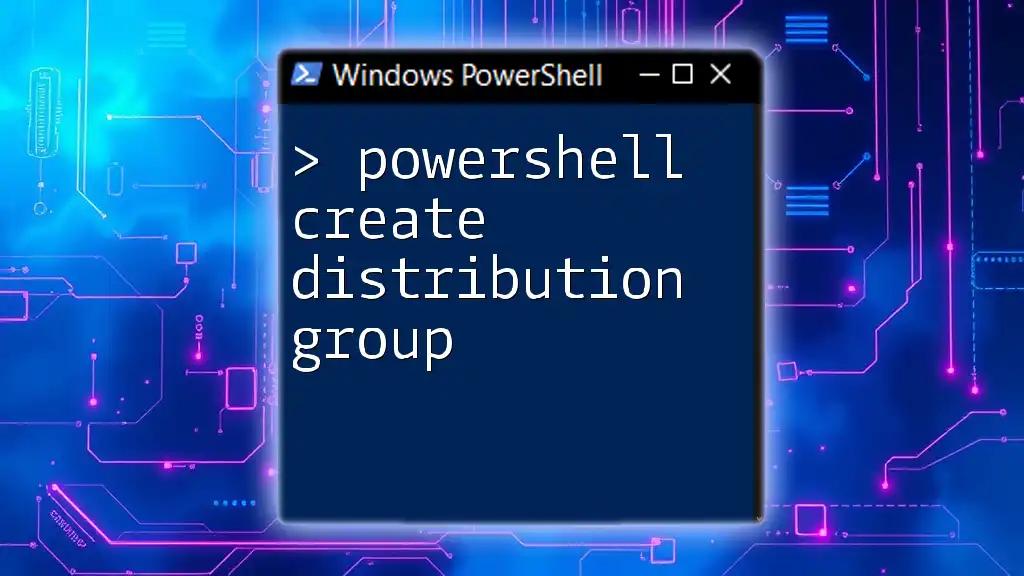
Error Handling and Troubleshooting
Common Issues When Creating Shortcuts
When creating shortcuts with PowerShell, you may encounter a few common issues, especially regarding permissions. If you receive an "Access Denied" error, ensure that your user account has permission to write to the target directory.
Debugging PowerShell Scripts
Debugging your PowerShell scripts can save time and frustration. Using `Try-Catch` blocks is a valuable technique for handling errors gracefully. For instance:
Try {
# Code to create shortcut
$Shortcut.Save()
} Catch {
Write-Host "An error occurred: $_"
}
This way, if an error occurs, your script provides a clear error message without terminating unexpectedly.
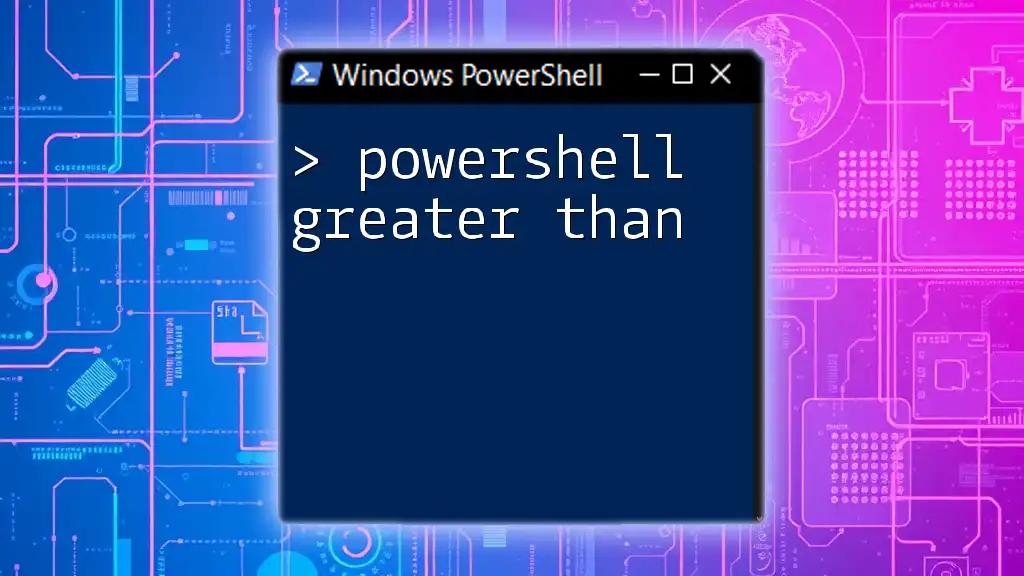
Practical Use Cases
Automating Shortcut Creation for Teams
One potent application of the `powershell create shortcut with icon` command is to streamline the setup for teams. By creating a single script that generates shortcuts for all team members, you ensure consistency and save time.
Batch Shortcut Creation for Multiple Files
If you need to create several shortcuts at once, a loop can automate the process efficiently. Consider the following example, where multiple destination files are targeted:
$Files = @("C:\Path\File1.exe", "C:\Path\File2.exe")
foreach ($File in $Files) {
$ShortcutPath = "$env:USERPROFILE\Desktop\" + [System.IO.Path]::GetFileName($File) + ".lnk"
$WshShell = New-Object -ComObject WScript.Shell
$Shortcut = $WshShell.CreateShortcut($ShortcutPath)
$Shortcut.TargetPath = $File
$Shortcut.Save()
}
This loop creates a shortcut for each file listed, placing them directly on the desktop.
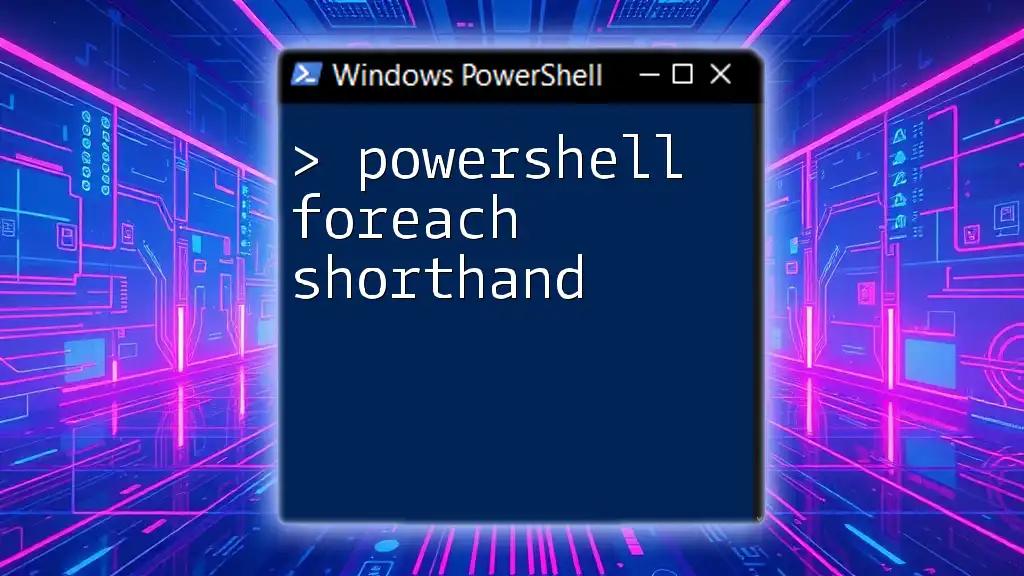
Conclusion
Creating shortcuts with PowerShell not only simplifies access to frequently used applications and files but also empowers users with automation capabilities that boost productivity. As you delve deeper into PowerShell, take the time to experiment with different commands and properties.
By mastering the art of automation, you’ll find that creating customized and functional shortcuts enhances your workflow significantly. Don’t hesitate to subscribe to our tutorials for more insights into leveraging PowerShell to its fullest potential!
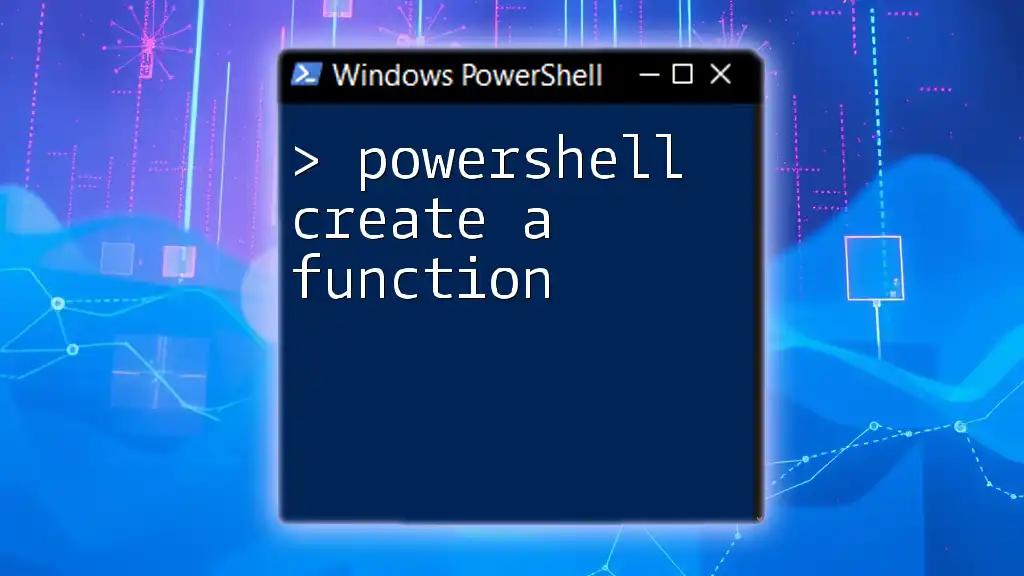
Additional Resources
For further learning, consider exploring the official Microsoft PowerShell documentation. Engaging with online PowerShell forums and communities can also provide valuable knowledge to enhance your skills.
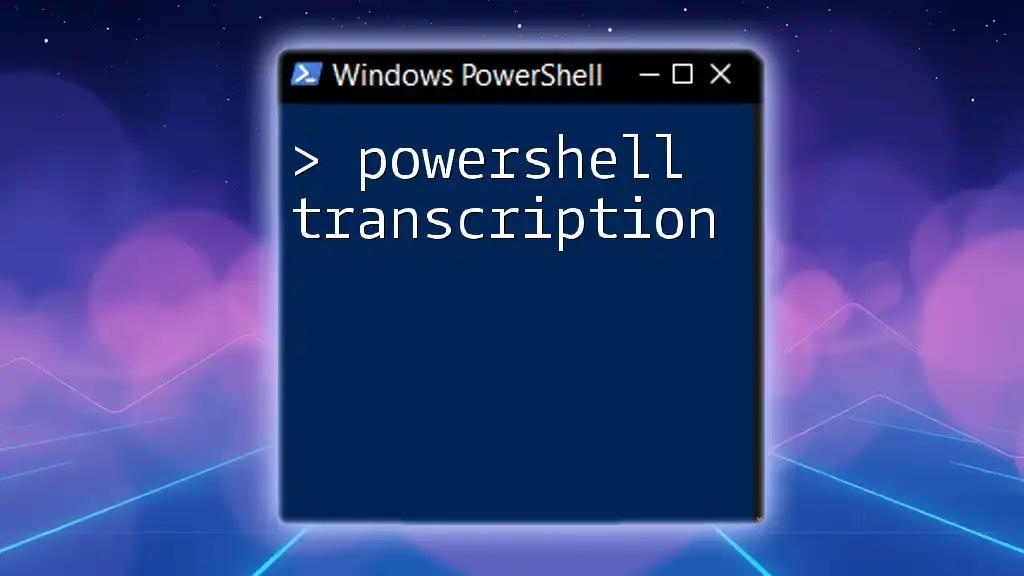
FAQs
Can I create shortcuts on other drives using PowerShell?
Yes, you can create shortcuts on any drive. Just specify the desired path accordingly, such as `D:\Shortcuts\MyShortcut.lnk`.
What types of files can be targeted by shortcuts?
Shortcuts can point to various file types, including executables (.exe), documents (.docx), folders, and even URLs for web shortcuts.
Can I create a shortcut for a website?
Absolutely! You can create a shortcut to a web URL by setting the `TargetPath` to the link:
$TargetPath = "https://www.example.com"
This will create a web shortcut that opens the specified URL in your default browser.