In PowerShell, a hash table is a collection of key-value pairs that can be created using the `@{}` syntax for efficient data organization and retrieval. Here's how you can create a hash table:
$hashTable = @{
Key1 = 'Value1'
Key2 = 'Value2'
Key3 = 'Value3'
}
What is a Hash Table?
Definition
A hash table is a data structure that uses key-value pairs to store data. In PowerShell, hash tables provide a convenient way to organize related information. They are especially useful for storing data that can easily be referenced by unique keys. Hash tables allow for fast lookup, insertion, and deletion, making them an efficient choice for scenarios where performance is key.
Use Cases
Hash tables are widely used in various scenarios, including but not limited to:
- Storing configuration settings for applications
- Caching results of expensive operations
- Grouping related information, such as user details or application settings
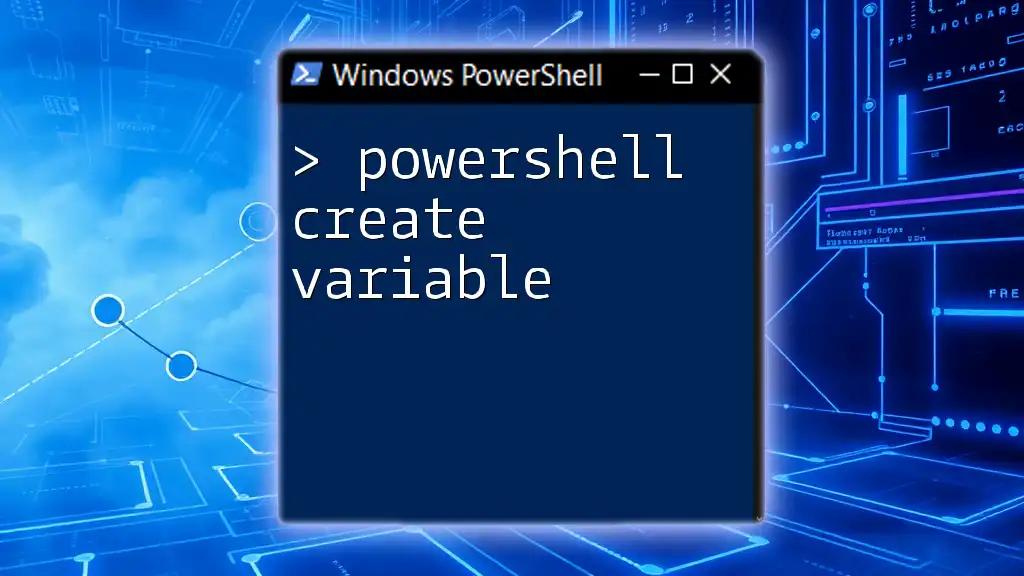
Creating a Hash Table in PowerShell
Basic Syntax
To create a hash table in PowerShell, you can use the following syntax:
$myHashTable = @{}
This initializes an empty hash table. You can think of it as a container ready to store your key-value pairs.
Adding Key-Value Pairs
To add elements to a hash table, you can assign a value to a specific key as follows:
$myHashTable['Key1'] = 'Value1'
$myHashTable['Key2'] = 'Value2'
Each key must be unique within a hash table. Attempting to use a duplicate key will overwrite the existing value, which could lead to data loss if not handled properly.
Initializing a Hash Table with Pairs
You can also create a hash table with predefined key-value pairs right from the start. Here’s how:
$myHashTable = @{
'Key1' = 'Value1'
'Key2' = 'Value2'
}
This method not only initializes the hash table but also fills it with values, making it more efficient, especially for larger datasets.
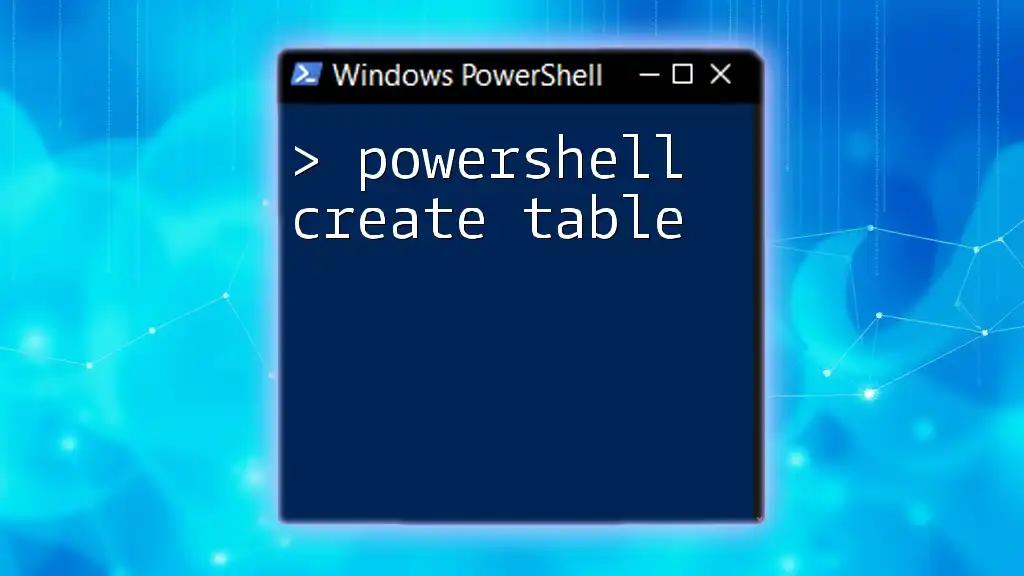
Accessing Hash Table Values
Retrieving Values
To retrieve values from a hash table, simply use the key as follows:
$value = $myHashTable['Key1']
This command fetches the value associated with `Key1`. If the key doesn’t exist, it will return `null`, so it’s crucial to ensure that the key being accessed exists.
Checking for Keys
To avoid retrieving a `null` value or causing an error, you can check whether a key exists in the hash table before accessing it:
if ($myHashTable.ContainsKey('Key1')) {
Write-Output "Key1 exists!"
}
This ensures that your code does not produce unexpected results and enhances its robustness.

Modifying and Removing Hash Table Entries
Updating Values
Modifying an existing value in a hash table is straightforward. Simply assign a new value to the existing key:
$myHashTable['Key1'] = 'NewValue1'
This command updates the value for `Key1` to `NewValue1`, allowing for dynamic data management.
Removing Key-Value Pairs
If you need to remove an entry, you can do so with this command:
$myHashTable.Remove('Key1')
This will delete the key-value pair associated with `Key1`. Keep in mind that attempting to remove a key that does not exist will not cause an error, but it will not change the hash table.

Iterating Through Hash Tables
Looping Methods
To iterate through a hash table, you can use a `foreach` loop:
foreach ($key in $myHashTable.Keys) {
Write-Output "$key = $($myHashTable[$key])"
}
This loop goes through each key in `Keys` and outputs its value, making it easy to display or manipulate all entries.
Sorting Hash Tables
Sorting is another common operation you may want to perform on hash tables. For instance, if you want to sort the keys alphabetically, you can do this:
$sortedKeys = $myHashTable.Keys | Sort-Object
This command allows you to retrieve a sorted list of keys, which can be useful when you need to present data in a specific order.
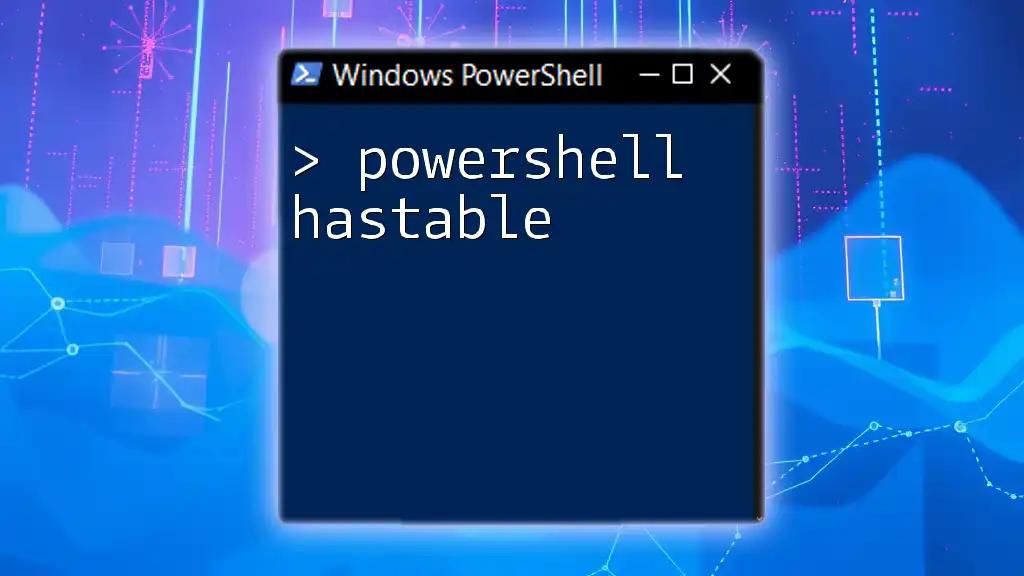
Best Practices for Using Hash Tables
Unique Keys
When working with hash tables, it’s crucial to maintain unique keys. Reusing keys can lead to unintentional overwrites and potential data loss. To manage duplicates, consider using a naming convention that incorporates context, such as timestamps or user identifiers.
Performance Considerations
Hash tables are typically faster than arrays and lists for lookups, insertions, and deletions of data, especially as the dataset grows. However, if you do not need associative arrays or prefer ordered data structures, you might consider using lists or arrays.
When to Use Hash Tables
Use hash tables when:
- You need to store and retrieve data quickly.
- Your data naturally fits a key-value format.
- You require a simple way to manage configurations or settings.

Conclusion
In this guide, we've discussed the concept of hash tables, how to create and manipulate them in PowerShell, and best practices for their use. Hash tables are a powerful feature in PowerShell that can help you manage data efficiently, and understanding their implementation will significantly enhance your scripting capabilities.
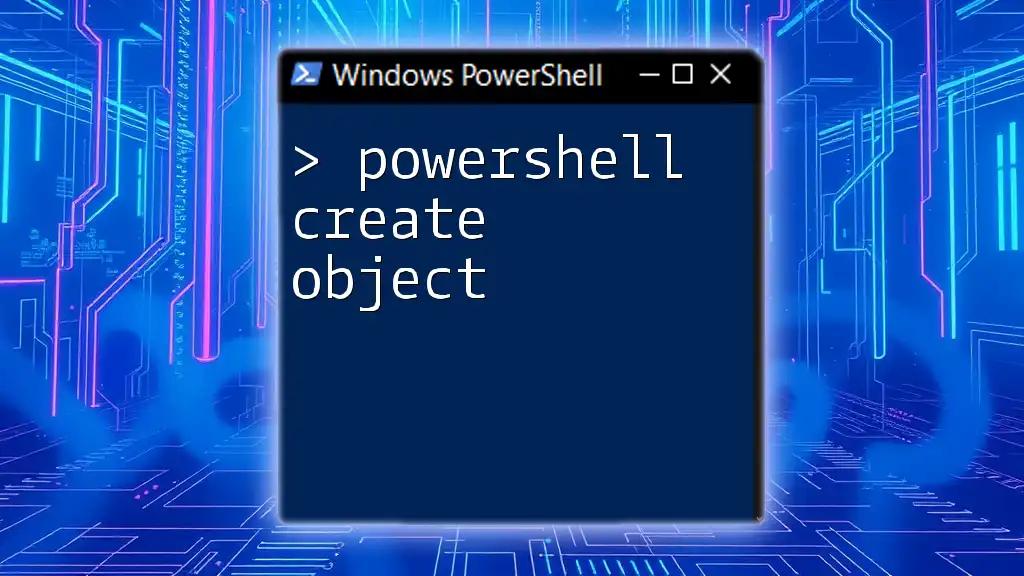
Call to Action
If you’re eager to dive deeper into PowerShell scripting and learn more about hash tables or other advanced features, consider signing up for workshops or online courses tailored to your needs. Enhance your skills and become proficient in PowerShell scripting!
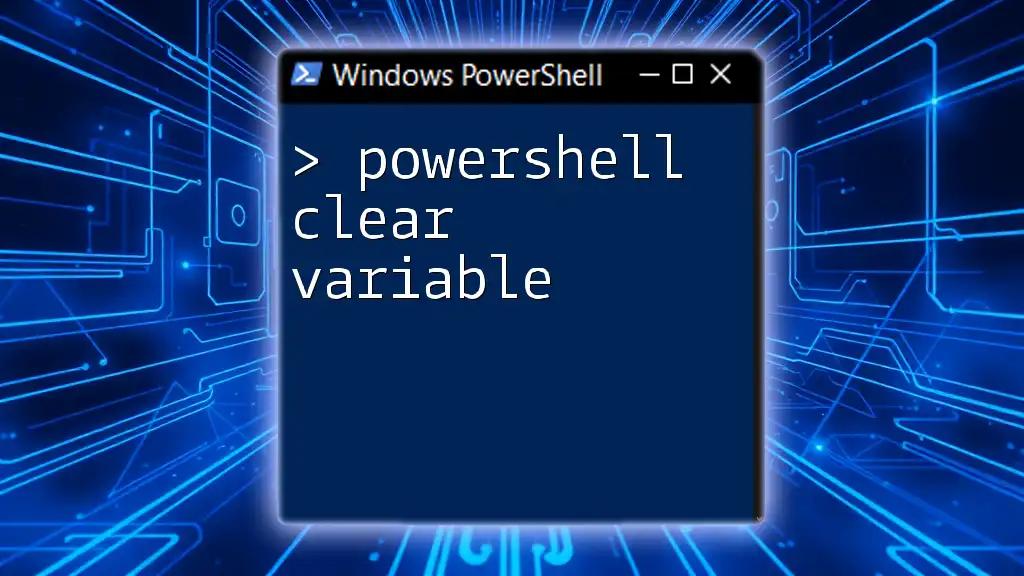
Additional Resources
For further learning, explore the official PowerShell documentation, community blogs, and forums where you can find helpful discussions on hash tables and other scripting topics. Happy scripting!