In PowerShell, you can suppress output by redirecting it to `$null`, which effectively discards the output from the command.
Get-Process > $null
Understanding PowerShell Output
What is PowerShell Output?
PowerShell generates output in the form of objects, text, and even errors as the results of executed commands. Understanding how these outputs work is fundamental to effectively using PowerShell. Output can be classified into two main categories: standard output, which is the regular output of commands, and error output, which captures errors that occur during command execution.
When Output Can Be a Problem
There are several situations where PowerShell output can become an issue:
- Clutter in Scripts: When running scripts, multi-line outputs can clutter your console and make it more difficult to follow along.
- Performance Issues: In certain environments, particularly with high-frequency execution, excessive output can lead to performance degradation.
- Clean Logs: When logging script operations, having unnecessary output can cause confusion and make it difficult to interpret logs.
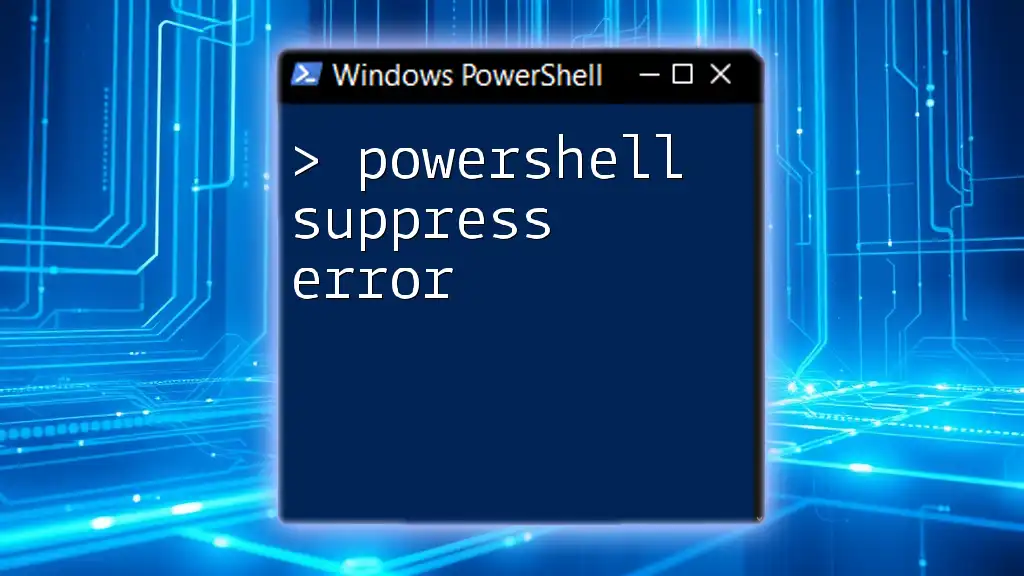
Methods to Suppress Output in PowerShell
Using Out-Null
The `Out-Null` cmdlet is specifically designed to suppress output. This cmdlet simply takes the output of a command and sends it to nowhere.
Example:
Get-Process | Out-Null
In this code snippet, `Get-Process` retrieves all running processes, but with `Out-Null`, none of the output is displayed. This method is especially useful in scenarios where you are interested in the execution of a command without wanting to clog the console with its output.
Pipe to Null (`>` or `2>`)
PowerShell allows for redirection of output using certain operators. Redirecting output to $null can also help eliminate clutter.
Suppressing Standard Output:
Get-Service > $null
This redirects the standard output of `Get-Service`, meaning you won’t see any of the services displayed in your PowerShell console.
Suppressing Error Output:
Get-Service NonExistentService 2> $null
In this example, you are attempting to retrieve a non-existent service. By redirecting error output, you prevent error messages from cluttering your output.
Using $null Variable
Another straightforward way to suppress output in PowerShell is by using the `$null` variable directly.
Example:
$null = Get-Content "file.txt"
In this case, you are reading the content of a file. Instead of displaying the output in the console, assigning the output to `$null` effectively discards it, which is useful when you are executing commands whose results you don’t need.
Using Start-Transcript - Excluding Output
The `Start-Transcript` cmdlet is designed for logging your PowerShell session. However, you might want to execute commands while excluding their output from the transcript.
How to Exclude Output from Transcript:
Start-Transcript -NoClobber
# Commands
Stop-Transcript
With `Start-Transcript`, you initiate logging, but to ensure that unnecessary output doesn’t get logged, you can structure your commands smartly, making use of other suppression techniques where necessary.
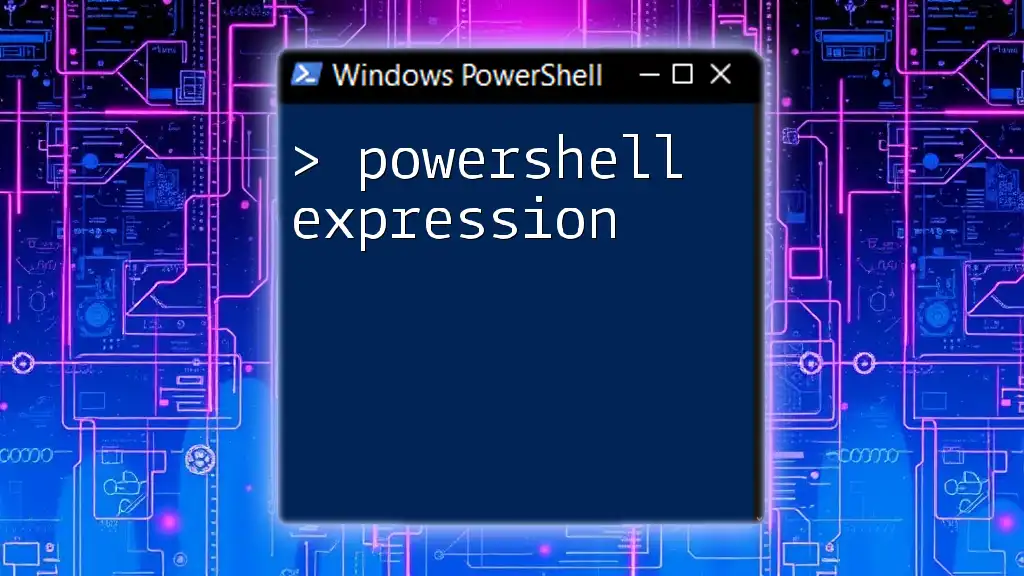
Advanced Techniques
Using Functions to Control Output
Creating custom functions in PowerShell will provide you with greater control over what is output. This allows you to encapsulate logic in one place while managing how much output gets generated.
Example Function:
function Get-Secret {
[CmdletBinding()]
param (
[string]$SecretName
)
# Logic here...
# Suppress direct output
return $null
}
In this example, the function `Get-Secret` retrieves a secret. Using `return $null` at the end prevents any output from cluttering the console, which is particularly useful in scripts designed to be silent agents.
Setting $OutputPreference
PowerShell provides a built-in variable called `$OutputPreference` that controls how output is handled at a global level.
How It Works:
$OutputPreference = "SilentlyContinue"
By setting `$OutputPreference` to "SilentlyContinue," you instruct PowerShell to suppress output from commands unless they return errors. This is especially handy when running batch processes or automated scripts where output is either unnecessary or undesired.
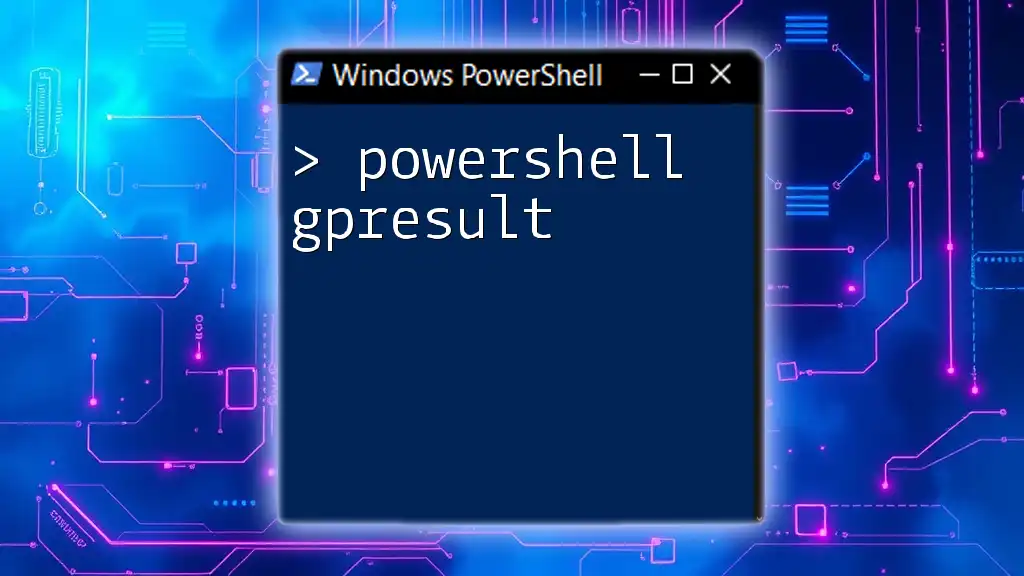
Common Mistakes to Avoid
While using techniques to suppress output, be cognizant of these common pitfalls:
Forgetting to Reset Output Preferences
If you modify `$OutputPreference`, ensure that you reset it back to its default settings after your script runs. Failing to do so could lead to unintended suppression of output in subsequent commands.
Confusing Suppressed Output with Filtering
Remember that suppressing output is different from filtering it. Filtering involves selecting specific types of information to display, while suppressing entirely removes output from view.
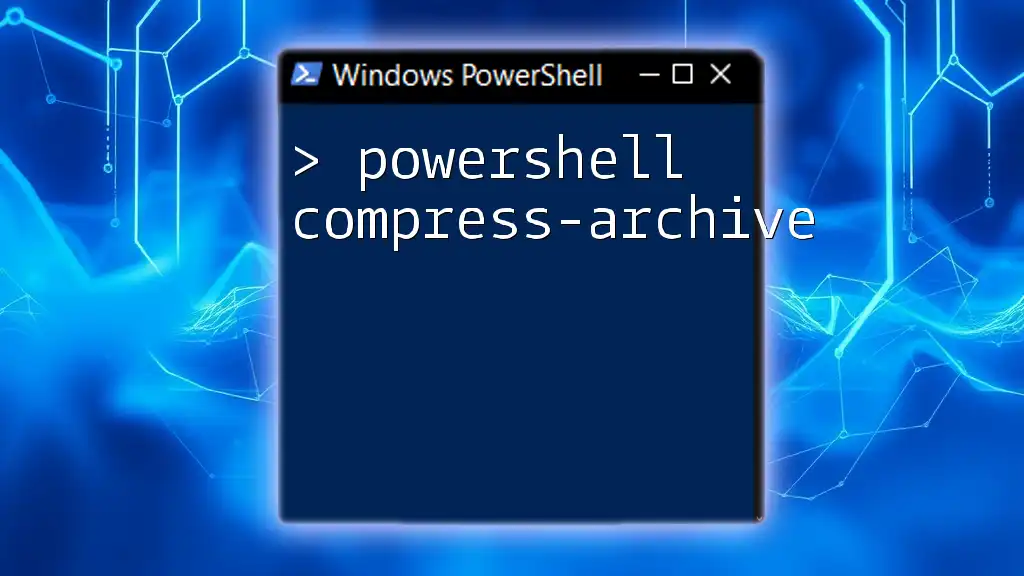
Conclusion
Suppression of output in PowerShell is a powerful tool that can help you create cleaner scripts, improve performance, and ensure your console remains organized. Whether you choose methods like `Out-Null`, redirection to `$null`, or leveraging functions and variables, each technique offers an efficient way to control what's displayed during execution.
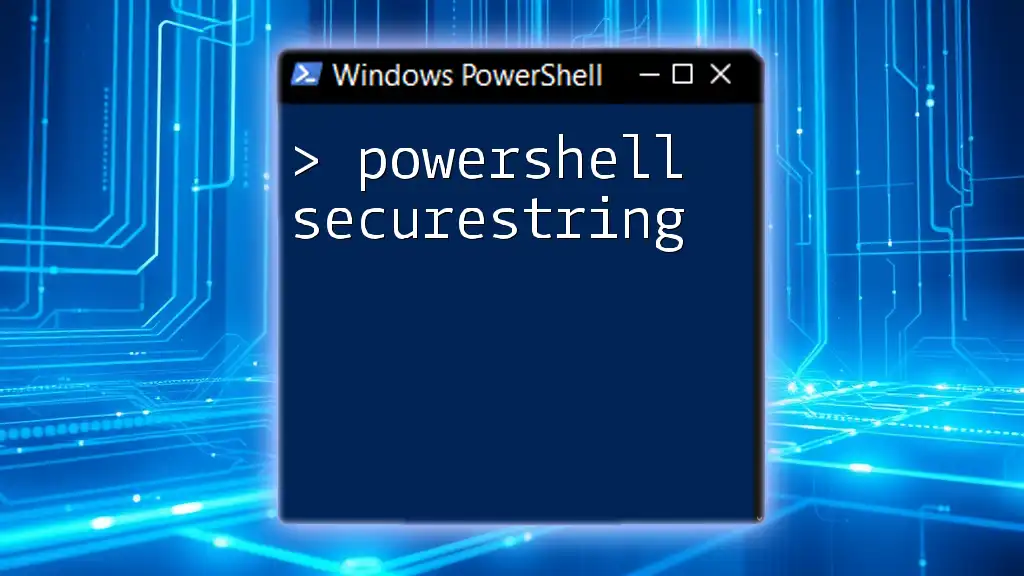
Additional Resources
For those wishing to deepen their understanding of how to manage output in PowerShell, consider exploring the official Microsoft documentation. Many excellent books and courses exist that delve deeper into the intricacies of using PowerShell effectively.
This guide serves as your jumping-off point into the world of PowerShell suppress output techniques. Experiment with these methods to see how they can enhance your scripting experience and streamline your workflows!