In PowerShell, the `-like` operator can be used with wildcards (e.g., `*` for any sequence of characters and `?` for a single character) to match strings within conditional statements, enhancing your ability to filter data efficiently.
Here's a code snippet demonstrating the use of wildcards with the `-like` operator:
$files = Get-ChildItem | Where-Object { $_.Name -like '*.txt' }
Write-Host "Text files in the directory: $($files.Count)"
What are Wildcards?
Definition of Wildcards
Wildcards are special characters used in computing to replace or represent one or more other characters in a string. They play a vital role in search operations, allowing for flexible matching rather than exact text when querying data. In PowerShell, the two most common wildcard characters are:
-
`*` (asterisk): This wildcard matches zero or more characters. For instance, searching for `*.txt` will match any file that has a `.txt` extension, regardless of the file name.
-
`?` (question mark): This wildcard matches exactly one character. For example, a search for `file?.txt` looks for files like `file1.txt` or `fileA.txt`, but not `file10.txt`.
Importance of Wildcards in PowerShell
Wildcards enable users to perform powerful searching and filtering operations efficiently. By using wildcards, you no longer need to specify exact strings, which can save time and reduce the chance of errors during scripting. They are particularly useful when dealing with data that is often unpredictable, such as file names or user input.
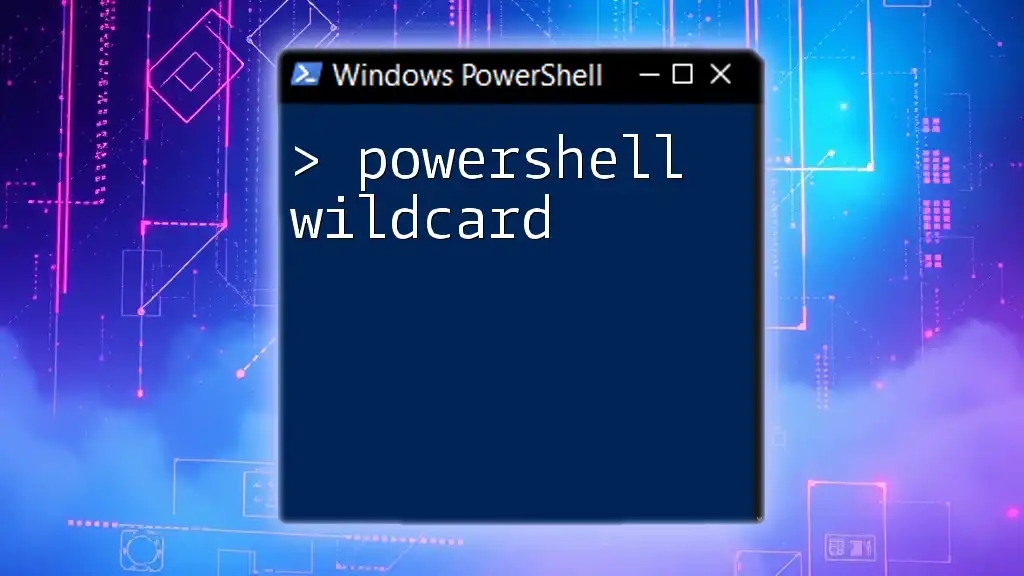
Using Wildcards with PowerShell Commands
The `-like` Operator
The `-like` operator is an essential comparison tool in PowerShell that allows for pattern matching using wildcards. It is particularly useful for filtering collections of objects and strings.
Syntax of the `-like` Operator
The basic syntax for the `-like` operator is:
Expression -like 'Pattern'
One crucial point to remember is that wildcard patterns need to be enclosed in single quotes. This ensures that PowerShell interprets the pattern correctly without any unintended substitutions.
Examples of the `-like` Operator
Basic Examples
- Example 1: Searching for files with a specific extension
To list all text files in a folder, you can use:
Get-ChildItem -Path "C:\MyFolder" -Filter "*.txt" -like "*.txt"
This command retrieves all files with a `.txt` extension in the specified directory, showcasing the straightforward filter capability of the `-like` operator.
- Example 2: Filtering strings that match a pattern
Suppose we have a list of names and want to filter those that start with "C":
$names = "Alice", "Bob", "Carol", "David"
$matchingNames = $names | Where-Object { $_ -like "C*" }
In this example, the command returns `"Carol"` as the only match, demonstrating how to leverage the `-like` operator for string filtering.
Advanced Examples
- Example 3: Complex filtering with multiple wildcards
Let's say you want to find all processes related to Google Chrome:
Get-Process | Where-Object { $_.Name -like "*Chrome*" }
This command will return any process with "Chrome" in its name, allowing you to manage related processes effectively.
- Example 4: Combining `-like` with other conditions
You can further refine your queries by combining the `-like` operator with other conditions. For instance, if you want to find text files that are larger than 1,000 bytes:
Get-ChildItem | Where-Object { ($_.Extension -like "*.txt") -and ($_.Length -gt 1000) }
This command showcases the power of logical operators, filtering files based on extension and size together.
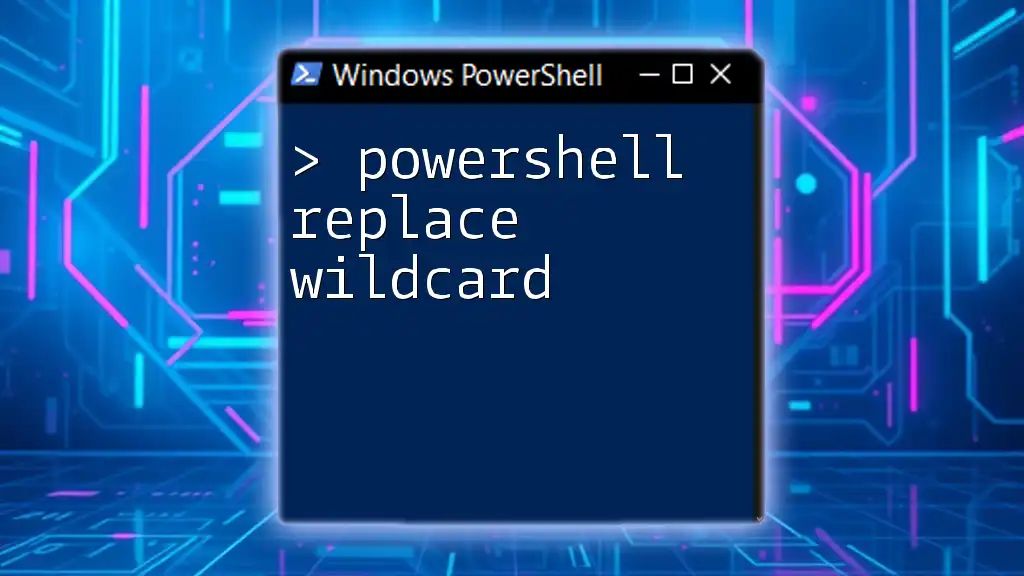
Understanding Escaping Wildcards
The Need for Escaping
In certain cases, you may want to search for a string that includes wildcard characters as part of the actual content rather than their functional significance. In such scenarios, you need to "escape" the wildcard character.
Escaping Wildcards in PowerShell
To escape wildcards in PowerShell, you can use the backtick character (`\``). This tells PowerShell to interpret the following character literally.
Example: If you’re searching for files that include the literal string "report*.txt":
$filename = "report`*.txt"
This command searches for an exact filename that includes the string "report*" rather than treating `*` as a wildcard.
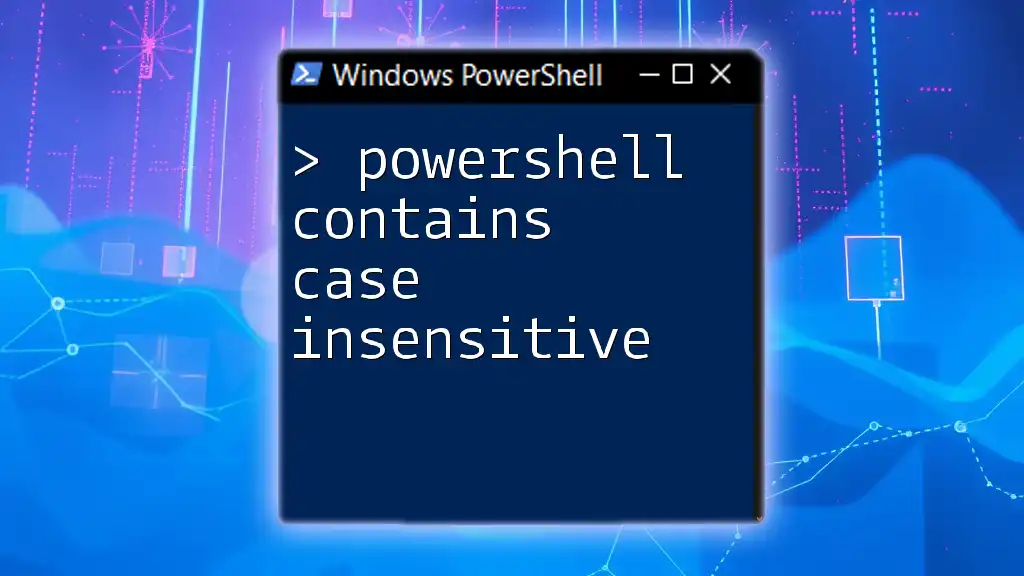
Common Pitfalls When Using Wildcards
Case Sensitivity
The `-like` operator is case-insensitive, but it's essential to be aware of this when working with file systems or data sources that may enforce case sensitivity. It's a good practice to test your filters with varying cases to ensure accurate results.
Incorrect Wildcard Usage
Misusing wildcards can lead to unexpected results or errors. A common mistake occurs when someone incorrectly combines wildcard expressions. For example:
Get-ChildItem -Path "C:\MyFolder" -Filter "*.txt" -like "*.doc"
This command will not work properly because the filter expects filenames. The proper usage would typically involve one or the other without mixing conditions in the Filter and in `-like`.
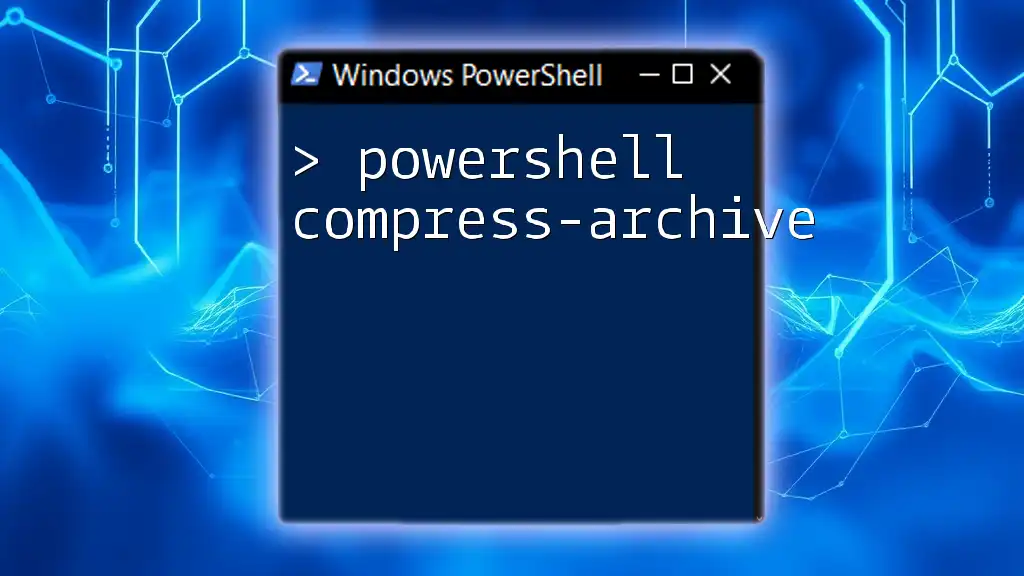
Conclusion
Understanding how to use wildcards, specifically with the `-like` operator, can dramatically improve your productivity and efficiency with PowerShell. By leveraging these tools, you can navigate and manipulate data much more effectively.
Call to Action
Feel free to start experimenting with wildcards in your PowerShell scripts! If you have questions or tips about using wildcards, share them in the comments. Don’t forget to spread the knowledge by sharing this article on social media!
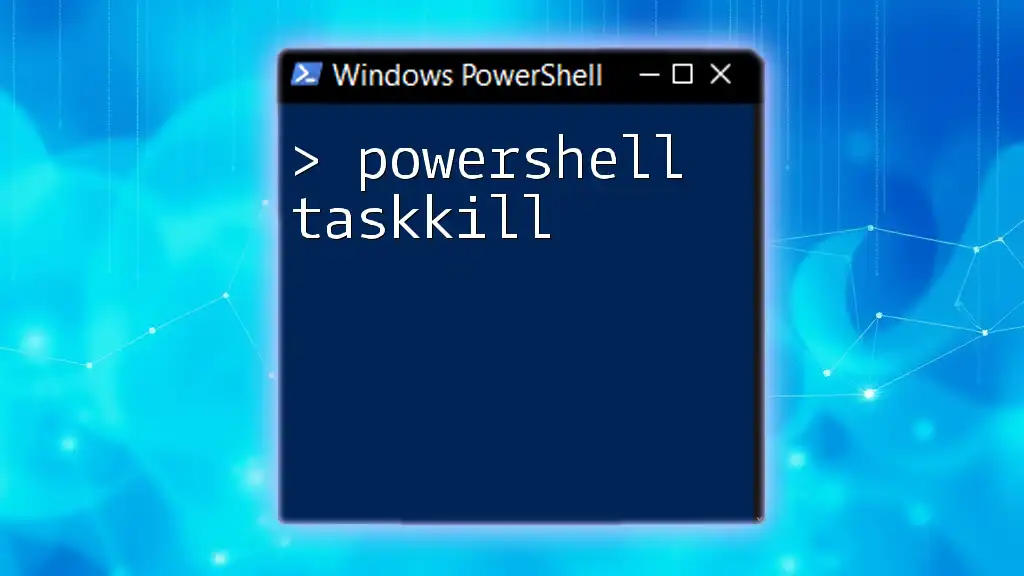
Additional Resources
For further learning, consider checking out official PowerShell documentation or specialized PowerShell courses that focus on scripting best practices and advanced filtering techniques.