PowerShell's `sed` equivalent, often achieved via the `-replace` operator, allows you to perform text substitutions in strings efficiently.
$originalText = "Hello, World!"
$modifiedText = $originalText -replace "World", "PowerShell"
Write-Host $modifiedText
Understanding `sed` and its Purpose
What is `sed`?
`sed`, short for Stream Editor, is a powerful tool primarily used for parsing and transforming text in Unix and Linux environments. It operates on a stream of text and can be employed for various tasks such as:
- Searching for patterns
- Text substitution
- Inserting and deleting lines
- Manipulating large volumes of data efficiently
By leveraging `sed`, users can automate repetitive text processing tasks and manage large datasets without the need for manual intervention.
Why Use `sed` in PowerShell?
Although PowerShell has its own rich set of string manipulation capabilities, understanding `sed` can provide unique advantages. PowerShell sed can simplify certain operations, particularly when integrating with other Unix-like tools or scripts, especially in mixed environments.
There are scenarios where `sed` commands can be more concise and targeted. Moreover, the learning of these expressions can enhance your overall text manipulation skill set, particularly if you're transitioning between Unix and Windows systems.
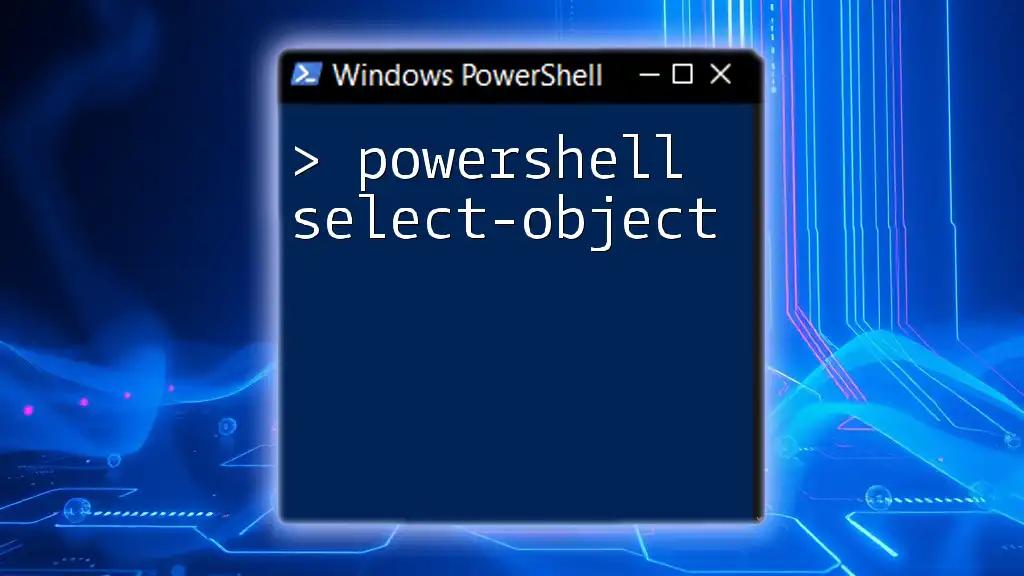
PowerShell Equivalent of `sed` Commands
Basic Scripting Syntax
In PowerShell, commands are set in a structured format that uses cmdlets, as opposed to the more linear approach found in `sed`. It’s important to grasp these differences, as they directly influence how you write and execute your commands.
Commonly Used `sed` Commands and Their PowerShell Equivalents
Substitute Command (`s/pattern/replacement`)
The substitute command in `sed` allows you to replace occurrences of a specified pattern with a new string.
Example `sed` command:
echo "Hello World" | sed 's/World/PowerShell/'
PowerShell equivalent:
"Hello World" -replace "World", "PowerShell"
In this example, `-replace` takes advantage of PowerShell's native regex capabilities to swap "World" with "PowerShell" seamlessly.
Delete Command (`d`)
The delete command is used to remove lines that match a certain pattern from the output or files.
Example `sed` command:
sed '/pattern/d' file.txt
PowerShell equivalent:
Get-Content file.txt | Where-Object {$_ -notmatch 'pattern'}
This PowerShell command reads from `file.txt`, filters out unwanted lines using `Where-Object`, and outputs the clean results to the console.
Print Command (`p`)
The print command in `sed` displays specific lines or patterns from a text stream.
Example `sed` command:
sed -n '/pattern/p' file.txt
PowerShell equivalent:
Get-Content file.txt | Where-Object {$_ -match 'pattern'}
This allows PowerShell users to print all lines containing the specified `pattern` from a file, closely mimicking the functionality of `sed`.
Append Command (`a`)
To append new lines after a specific pattern, you can use the append command in `sed`.
Example `sed` command:
sed '/pattern/a\new line' file.txt
PowerShell equivalent:
(Get-Content file.txt) + "new line" | Set-Content file.txt
In PowerShell, this approach reads the content of `file.txt`, appends "new line," and writes it back to the file.
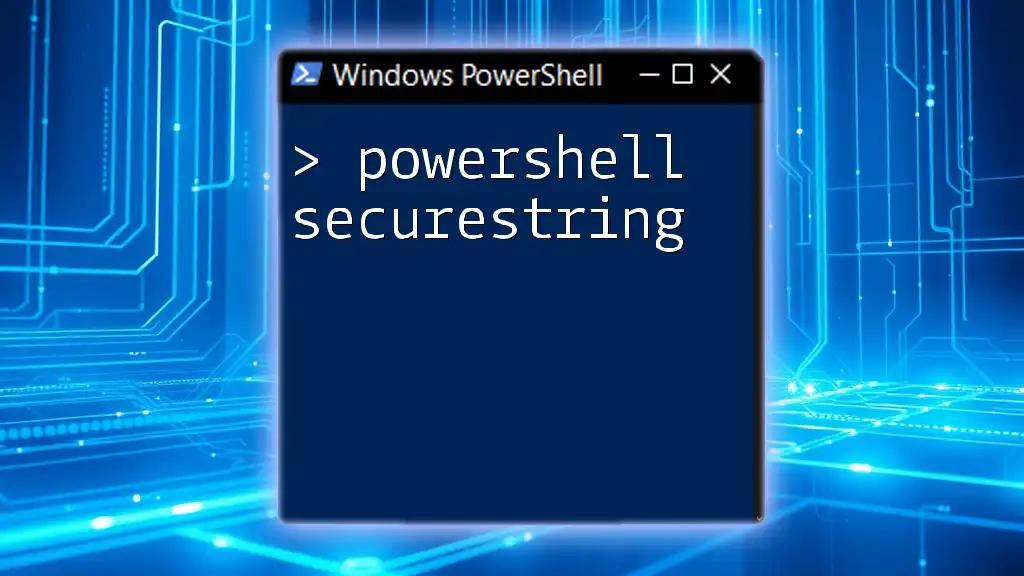
Advanced `sed` Techniques in PowerShell
Regular Expressions
Regular expressions (regex) are crucial for powerful string matching in both `sed` and PowerShell. In PowerShell, regex is fully supported, making it easy to run complex text manipulations.
For example, to replace all digits in a string with "456":
"Data 123" -replace '\d+', '456'
This command replaces any digits (`\d+`) in the string with "456." Mastering regex in PowerShell greatly enhances your text processing capabilities.
Editing Multiple Files
When you're dealing with multiple files, PowerShell shines with its simple syntax and powerful cmdlets.
Here is an example using a loop to replace text across multiple files:
Get-ChildItem "*.txt" | ForEach-Object { (Get-Content $_) -replace 'old', 'new' | Set-Content $_ }
This command fetches all `.txt` files in the directory, replaces "old" with "new" in each file, and saves the changes. PowerShell's pipelines make such multi-file operations straightforward and efficient.
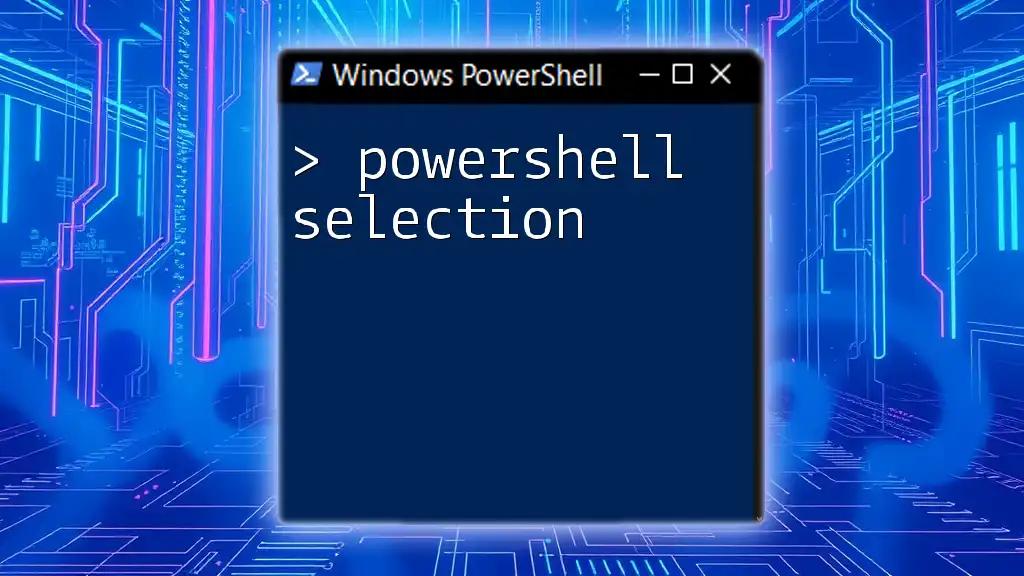
Tips and Best Practices
Debugging Your Scripts
When you're coding in PowerShell, it’s common to run into errors or unexpected behaviors. Some common pitfalls when using PowerShell's equivalents of `sed` include:
- Regex syntax mismatches
- File access issues (permissions)
- Misunderstanding the output format
To troubleshoot effectively, use Write-Host to print interim values or check command outputs at various stages.
Combining Commands to Enhance Functionality
PowerShell allows you to combine commands seamlessly using pipelines. Combining commands can streamline complex tasks into single lines for efficiency and clarity.
For example:
Get-Content file.txt | Where-Object {$_ -match 'pattern'} | ForEach-Object {$_ -replace 'old', 'new'} | Set-Content updated_file.txt
This example efficiently processes a file, searches for a pattern, replaces specified text, and writes the results to a new file.
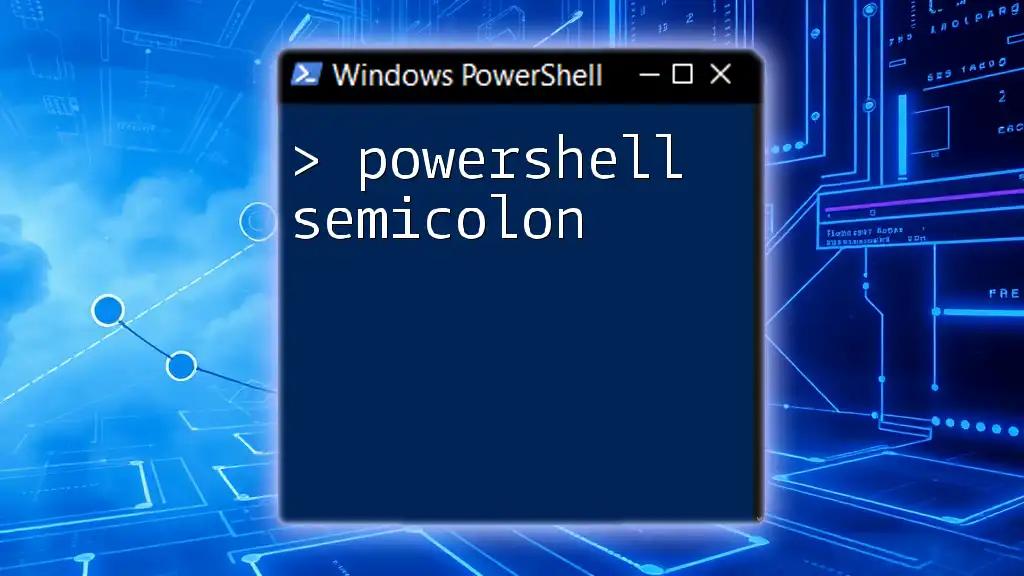
Conclusion
Understanding how to translate `sed` commands into PowerShell provides you with a powerful toolkit for text manipulation. By mastering PowerShell sed, you can enhance your efficiency in processing text and empower your automation scripts.
As you practice with the examples provided, don't hesitate to experiment and expand your toolkit further. The world of PowerShell scripting is vast, offering endless opportunities for automation and efficiency.
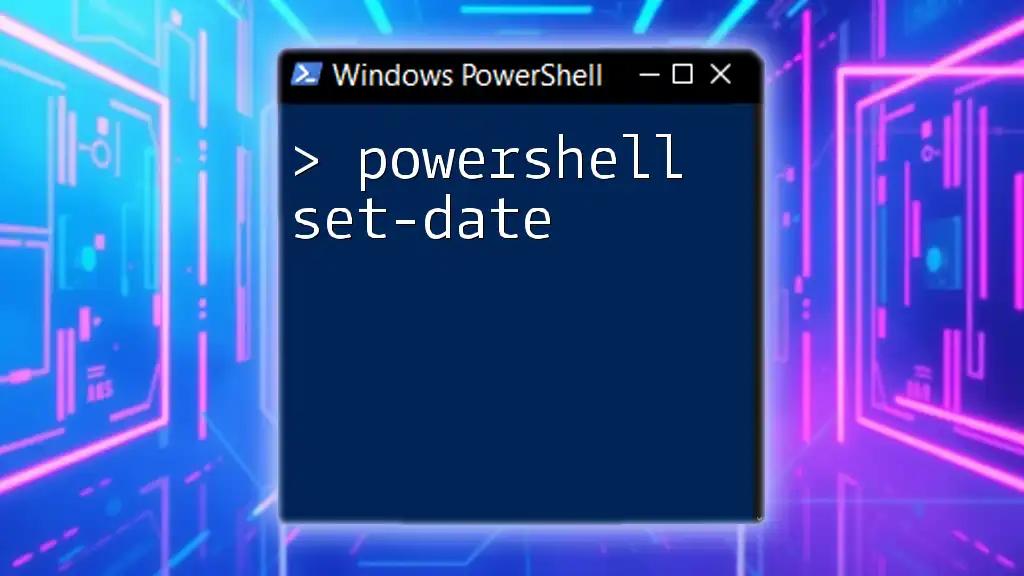
Additional Resources
For ongoing learning, explore the official PowerShell documentation, participate in community forums, and consider various books and online courses to deepen your understanding of PowerShell scripting and text manipulation.

FAQs
Can I use `sed` directly in PowerShell?
While `sed` is not natively included in PowerShell, you can run it through Windows Subsystem for Linux (WSL) or by using Cygwin.
What are the limitations of using PowerShell for text processing?
PowerShell’s cmdlets can sometimes be slower than `sed`, especially when handling very large datasets, due to underlying architecture differences.
How does PowerShell handle large files compared to `sed`?
`sed` is optimized for inline processing of large files without loading the entire content into memory, whereas PowerShell might handle large files less efficiently due to its object-oriented approach. However, PowerShell is improving in this area with cmdlets like `StreamReader`.