Sure! Here's a one-sentence explanation along with a code snippet:
If you're a beginner looking to dive into PowerShell, these simple projects will help you build your skills while automating everyday tasks efficiently.
Write-Host 'Hello, World!'
Getting Started with PowerShell
Installing PowerShell
To begin your journey into using PowerShell, it’s essential to first install it properly. PowerShell comes pre-installed on Windows systems, but it's also available on Linux and macOS. Refer to the official Microsoft documentation for detailed installation steps customized for your operating system.
Understanding the Basics of PowerShell
As a command line shell and scripting language, PowerShell is designed specifically for system administration tasks. At its core, PowerShell uses cmdlets, which are small, built-in functions that perform particular operations. Understanding the syntax of these cmdlets and navigating the command-line interface is crucial for executing commands effectively. The PowerShell Integrated Scripting Environment (ISE) and Visual Studio Code are excellent tools for writing, testing, and debugging your scripts.
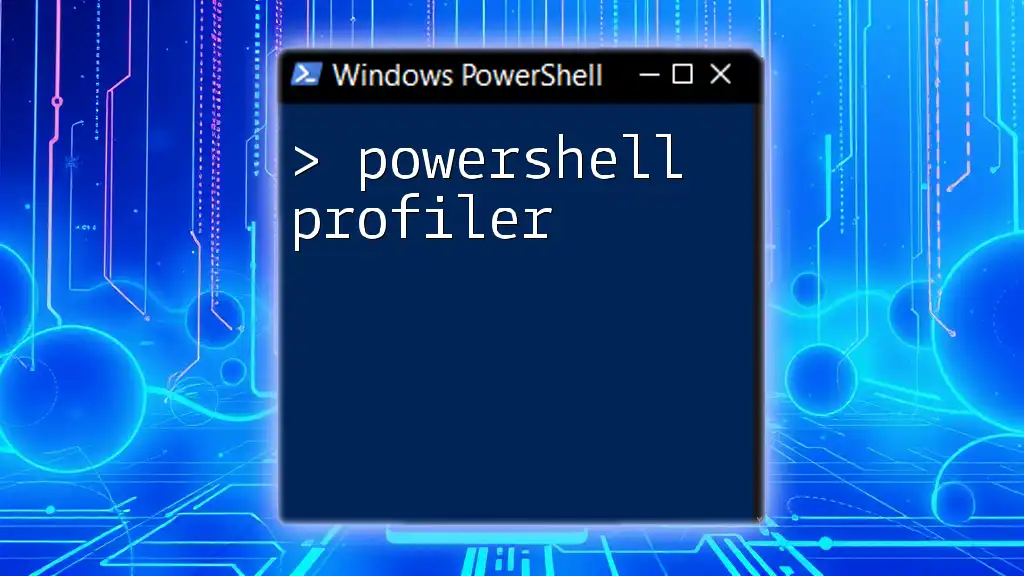
Project 1: Creating a Simple Directory Structure
Overview
Creating a structured directory is a fundamental skill that highlights PowerShell's automation capabilities. This project not only simplifies file management but also serves as an essential exercise in using PowerShell to automate routine tasks.
Steps to Complete the Project
To create a base directory and several subdirectories, you can use the following script:
$baseDir = "C:\Projects\MyProject"
$subDirs = @("Docs", "Scripts", "Logs")
New-Item -Path $baseDir -ItemType Directory
foreach ($dir in $subDirs) {
New-Item -Path "$baseDir\$dir" -ItemType Directory
}
Explanation of the Code
In this code snippet:
- New-Item creates a new item (in this case, directories) at the specified path.
- The script initializes a base directory and iterates through a list of subdirectory names to create them within the base directory.
- This project reinforces understanding directory structures and foundational cmdlet usage.
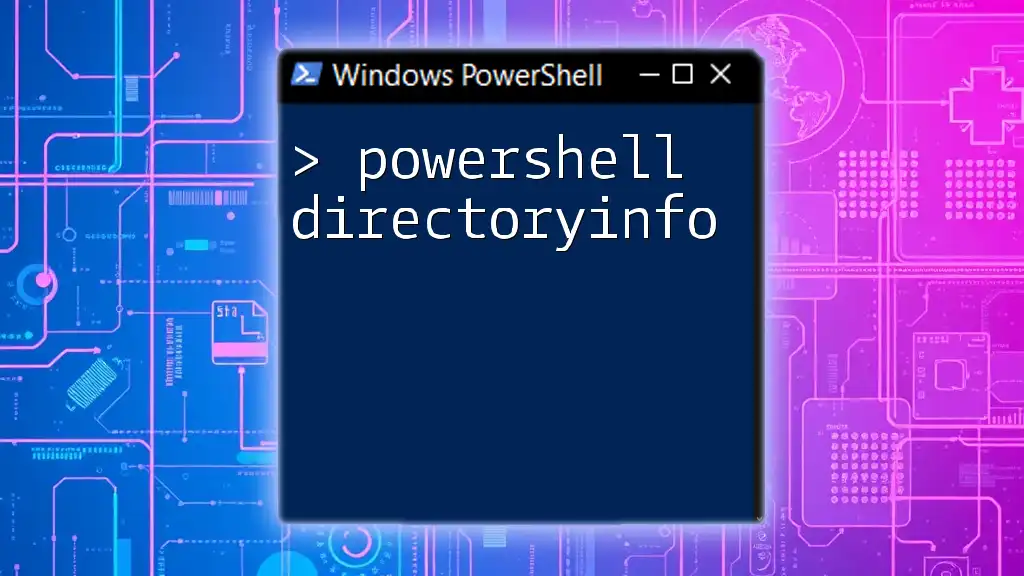
Project 2: Retrieving System Information
Overview
Retrieving system information is crucial for any administrator or IT professional. This project helps you gather important system metrics, aiding in diagnostics and performance monitoring.
Steps to Complete the Project
You can easily retrieve essential system information using the following command:
Get-ComputerInfo | Select-Object CsName, OsArchitecture, WindowsVersion, WindowsEditionId
Explanation of the Code
This command utilizes:
- Get-ComputerInfo, which returns a wealth of system information.
- Select-Object allows you to filter and display specific properties, making it easier to locate the most relevant details.
This project introduces you to the vast amount of data PowerShell can access, improving your administrative capabilities.
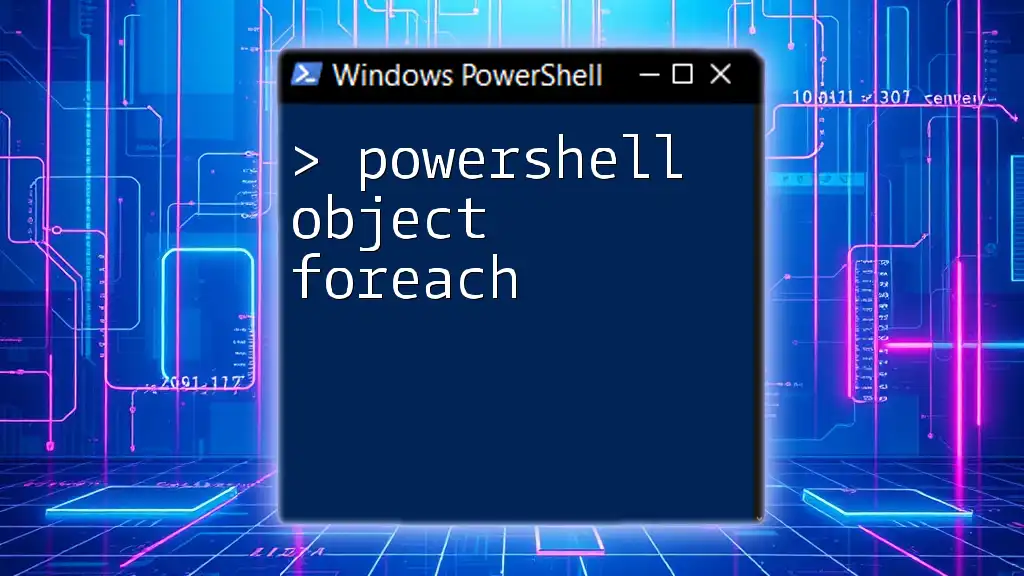
Project 3: Automating File Backups
Overview
Data loss can occur unexpectedly, making automated backups critical. This project teaches you to create scripts that regularly back up important files, an essential automation task in IT environments.
Steps to Complete the Project
Use this script to create a simple file backup operation:
$source = "C:\MyFiles"
$destination = "D:\Backup\MyFilesBackup"
Copy-Item -Path $source -Destination $destination -Recurse -Force
Explanation of the Code
In this snippet:
- Copy-Item is a powerful cmdlet that allows you to copy files or directories from one location to another.
- The `-Recurse` flag ensures that all subdirectories and files in the designated source are included in the backup, while `-Force` overwrites any existing files in the destination.
Through this project, you gain practical skills in data management and the significance of scripting for routine tasks.
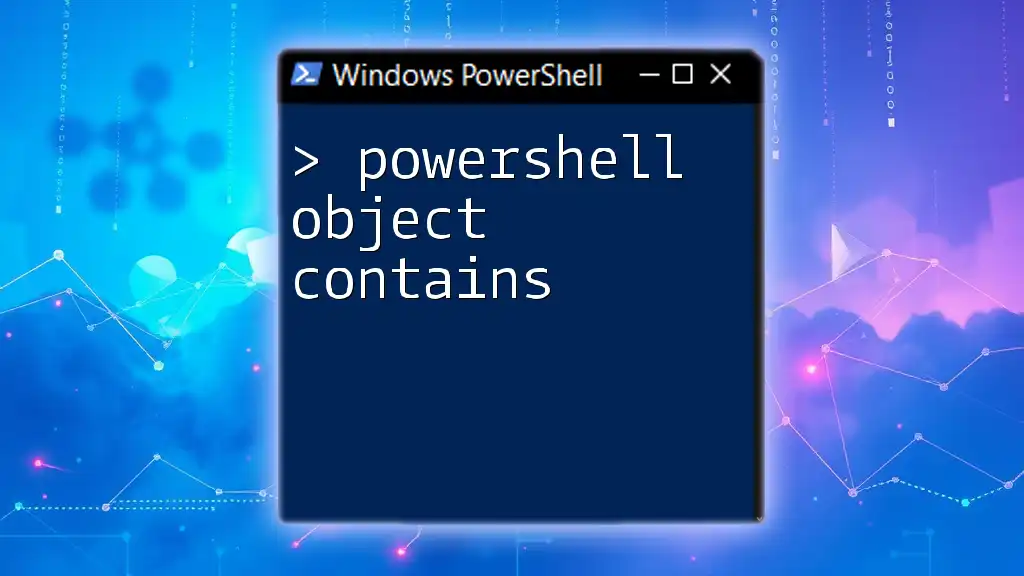
Project 4: Monitoring System Performance
Overview
Monitoring system performance is vital for maintaining optimal productivity and resource allocation. This project introduces the concept of using performance counters to keep tabs on system health.
Steps to Complete the Project
To monitor CPU usage systematically, use this PowerShell command:
Get-Counter '\Processor(_Total)\% Processor Time' -SamplingInterval 5 -MaxSamples 12
Explanation of the Code
This command works as follows:
- Get-Counter retrieves performance data; in this case, it captures CPU utilization.
- The parameters `-SamplingInterval` and `-MaxSamples` dictate how often the data is collected and the total number of samples to retrieve.
This project provides hands-on experience in system monitoring, an essential skill in IT operations.
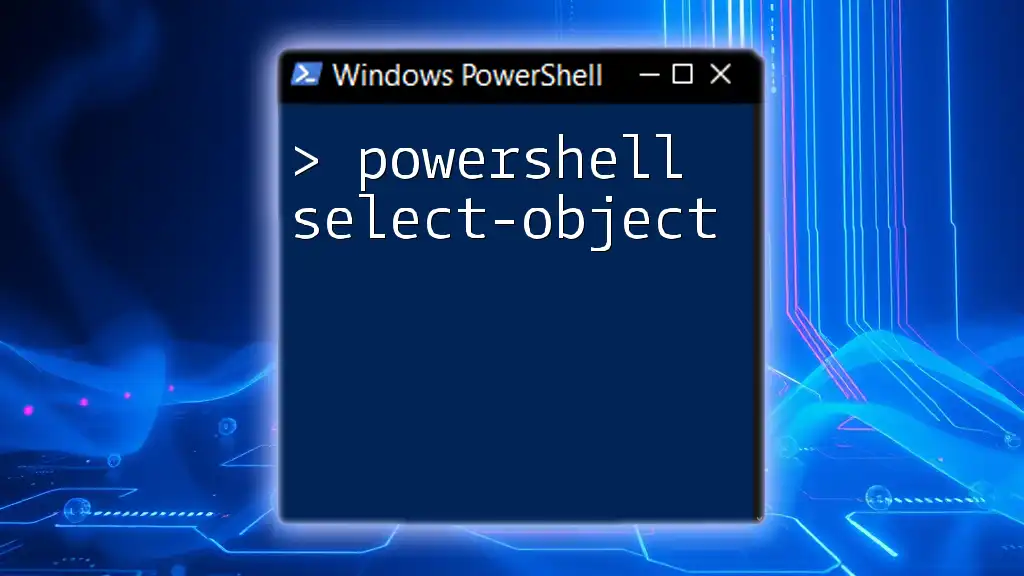
Project 5: Sending Automated Email Alerts
Overview
Automated email alerts are a key feature for proactive monitoring of systems. Learning how to send notifications via PowerShell can help you respond quickly to potential issues.
Steps to Complete the Project
Here's how to configure an automated email alert:
$smtpServer = "smtp.yourserver.com"
$from = "alert@yourdomain.com"
$to = "user@domain.com"
$subject = "Alert: High CPU Usage"
$body = "The CPU usage has exceeded the threshold."
Send-MailMessage -SmtpServer $smtpServer -From $from -To $to -Subject $subject -Body $body
Explanation of the Code
In this script:
- Send-MailMessage is used to send an email alert, with parameters specifying the SMTP server, sender address, recipient address, subject line, and message body.
- Understanding this functionality enables you to create actionable and timely responses to system events.
This project reinforces the concept of automation by teaching how to effectively communicate system status changes.
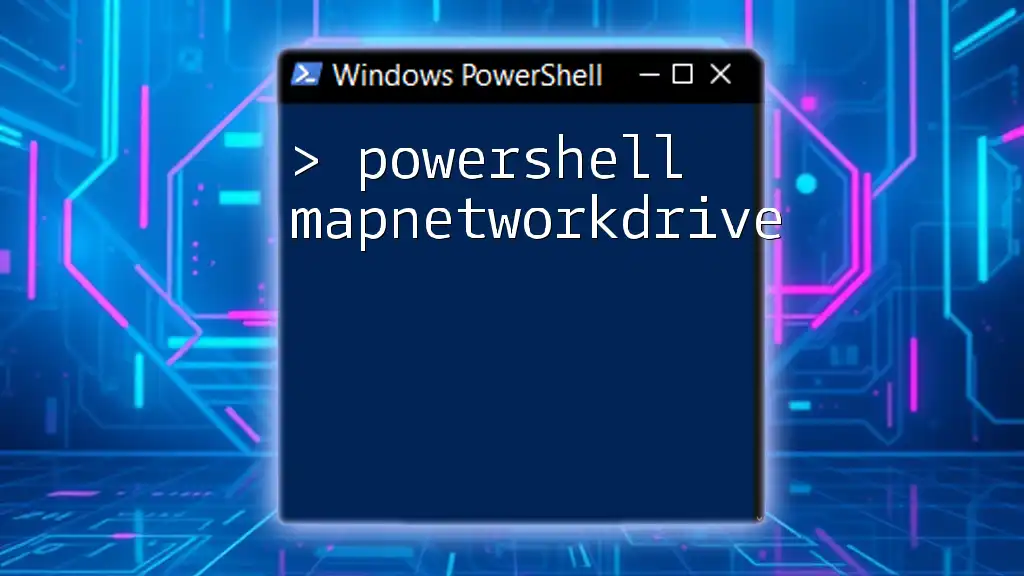
Conclusion
Throughout this article, we explored a variety of PowerShell projects for beginners. From creating directory structures to monitoring system performance, you've learned practical skills that can significantly enhance your workflow.
To further develop your expertise, consider engaging with community resources, participating in forums, and consistently looking for new ways to apply PowerShell in your daily tasks. PowerShell is a powerful tool—start your journey today and discover how it can automate your routine and complex tasks alike.