In PowerShell, you can prompt the user for input using the `Read-Host` cmdlet, which allows you to gather data directly from the console.
$userInput = Read-Host 'Please enter your input'
Write-Host "You entered: $userInput"
Understanding User Input in PowerShell
What is User Input?
User input refers to the information provided by an end-user during the execution of a script or program. In PowerShell, receiving user input is essential for creating dynamic scripts that respond to the user's preferences, needs, or specific data. Capturing user input allows scripts to be more versatile and interactive, transforming them from static operations into adaptive utilities.
Why Use Prompts for User Input?
Prompts for user input are a fundamental part of many PowerShell scripts. They allow for:
- Customization: Users can provide values to personalize the execution of a script tailored to their specific needs.
- Flexibility: Scripts can adapt to different scenarios based on the inputs provided, making them reusable and efficient.
- User Engagement: Engaging the user creates a more interactive experience, making the tool more user-friendly.
Real-world scenarios that highlight the need for user input might include gathering authentication details for server access, prompting for file paths, or requesting configuration parameters.

PowerShell Methods for Reading User Input
Using `Read-Host`
The simplest way to prompt for user input in PowerShell is by using the `Read-Host` cmdlet. This cmdlet displays a prompt message and captures what the user types.
Syntax:
$variable = Read-Host -Prompt "Enter your input"
Example:
$name = Read-Host -Prompt "Please enter your name"
Write-Host "Hello, $name!"
In this example, the script prompts the user for their name and then responds with a personalized greeting.
Using `Get-Credential`
For scenarios requiring secure input—such as password entry—`Get-Credential` is the go-to cmdlet. This cmdlet prompts the user for a username and a password and returns a credential object.
Syntax:
$credential = Get-Credential
Example:
$creds = Get-Credential -Message "Enter your credentials"
Write-Host "Username: $($creds.UserName)"
Here, the user is prompted to enter their credentials, which can then be utilized for authentication purposes within a script.
Combining `Read-Host` with Data Validation
Basic Input Validation
Given that user input can sometimes be unpredictable, validating data is crucial for maintaining the integrity of the script's operations. For instance, you can check if the input meets specific criteria before proceeding.
Example:
$age = Read-Host -Prompt "Enter your age"
if ($age -lt 0) {
Write-Host "Please enter a valid age."
}
In this snippet, the script verifies that the age is a non-negative value, providing feedback if the input is invalid.
Advanced Input Validation with Regex
Using regular expressions (regex) for validation allows for sophisticated input controls. This can be particularly useful for validating formats, such as email addresses.
Example:
$email = Read-Host -Prompt "Enter your email address"
if ($email -notmatch '^[^@\s]+@[^@\s]+\.[^@\s]+$') {
Write-Host "Please enter a valid email."
}
In this case, the script checks whether the entered email matches the regular expression defined for a standard email format. If not, it prompts the user for a correction.
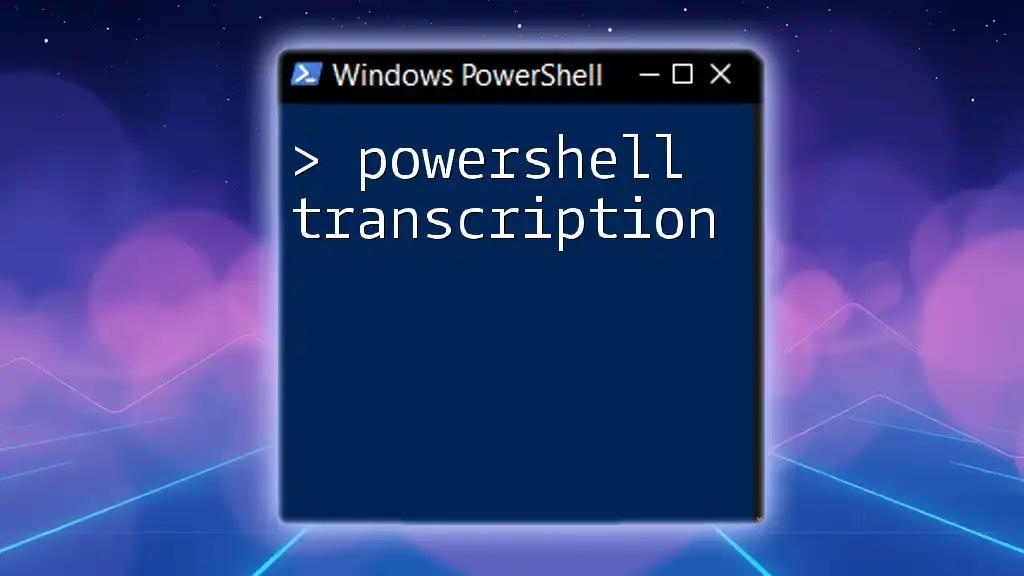
Storing and Using User Input
Storing Input in Variables
Once you've received user input, it’s essential to store it in variables for later use in your scripts. This allows for easy reference throughout the script’s execution.
Code Snippet:
$inputData = Read-Host -Prompt "Enter some data"
Here, whatever the user inputs is stored in the variable `$inputData`, which can be used later in the script.
Utilizing User Input in Scripts
Example Showing Practical Use Cases
To illustrate how user input can be utilized in practical scenarios, consider a script that asks the user for a message and the number of times to display it.
Example Script:
$message = Read-Host -Prompt "Enter a message"
$repeat = Read-Host -Prompt "How many times do you want to display it?"
for ($i=0; $i -lt $repeat; $i++) {
Write-Host $message
}
In this example, the user provides a message and a repetition count. The script displays the message as many times as specified, showcasing how user input drives script behavior.
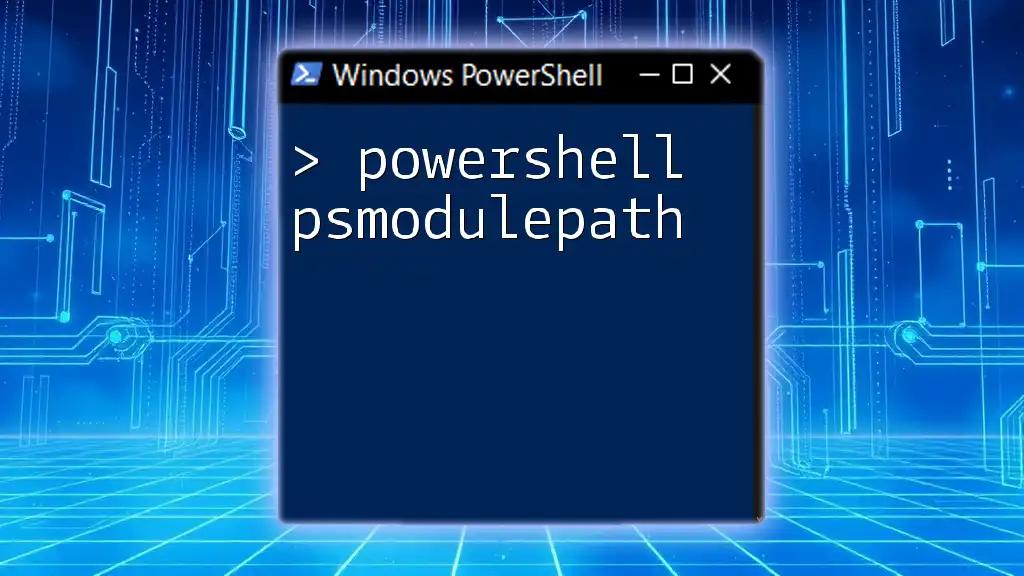
Best Practices for Prompting User Input
Keeping User Prompts Clear
Clarity is key when prompting for input. Ensure that your prompts are straightforward and devoid of jargon. This simplicity helps users understand precisely what is being requested, leading to more accurate data entry.
Handling User Errors Gracefully
It’s crucial to manage errors without causing frustration. Scripts should guide users to correct mistakes while maintaining a user-friendly experience.
Code Snippet Example:
do {
$number = Read-Host -Prompt "Enter a positive number"
} while ($number -lt 0)
This loop continues to prompt the user until a valid positive number is entered, effectively guiding them toward correct input while minimizing disruption.

Conclusion
Prompting for user input in PowerShell scripts adds interactivity and flexibility, creating a more engaging experience. By leveraging tools like `Read-Host` and `Get-Credential`, and applying validation techniques, you can significantly enhance the functionality and usability of your scripts. Practicing these methods and integrating them into your PowerShell toolkit can transform how you develop scripts, leading to more adaptive and user-friendly solutions.
Be bold in experimenting with user input prompts, and discover how they can elevate your automation tasks to new heights.