You can prompt users for input in PowerShell using the `Read-Host` cmdlet, which captures the input from the console.
$userInput = Read-Host 'Please enter your input'
Write-Host "You entered: $userInput"
Understanding User Input in PowerShell
User input refers to any data entered by a user during the execution of a program or script. PowerShell scripts can benefit significantly from user input as it provides dynamic interactions, allowing scripts to adapt based on data entered during runtime.
Utilizing user input transforms static scripts into dynamic ones, capable of tailoring their behavior according to the needs of different users or specific scenarios.
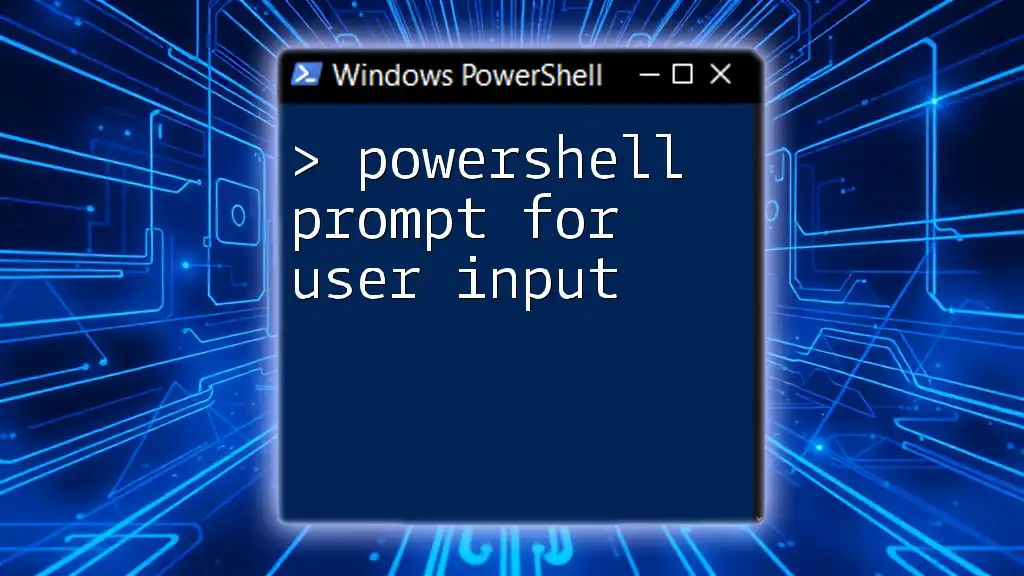
Collecting User Input in PowerShell
Using Read-Host
The `Read-Host` cmdlet serves as the primary method for obtaining user input in PowerShell. This command prompts the user with a specified message and captures the input.
Basic Syntax and Usage
$userInput = Read-Host -Prompt "Please enter your name"
In this example, the user is asked to provide their name, which is then stored in the variable `$userInput`.
Example Scenario: Greeting Users Based on Input
$userInput = Read-Host -Prompt "Please enter your name"
Write-Output "Hello, $userInput!"
This script will greet the user with a personalized message, demonstrating the interactive capacity of the script.
Combining Read-Host with Other Cmdlets
Using `Read-Host` with other PowerShell cmdlets enhances the functionality of your scripts.
Using Read-Host with Write-Output
$inputName = Read-Host -Prompt "Enter your name"
Write-Output "Hello, $inputName!"
This code captures the user’s name and displays a greeting based on the provided input.
Validating User Input It's crucial to validate the data entered to ensure it meets the expected criteria. For example, check if the input is non-empty:
$userInput = Read-Host -Prompt "Enter a non-empty value"
if (-not [string]::IsNullOrWhiteSpace($userInput)) {
Write-Output "User input is valid!"
} else {
Write-Output "Input cannot be empty!"
}
This code snippet checks whether the user has provided a valid entry and displays an appropriate message.
Multi-Choice User Input
Using Switch Statements
In scenarios where multiple options are available, a switch statement can facilitate decision-making based on user input.
Example Scenario:
$option = Read-Host -Prompt "Choose an option: A, B, or C"
switch ($option) {
"A" { Write-Output "You chose A!" }
"B" { Write-Output "You chose B!" }
"C" { Write-Output "You chose C!" }
default { Write-Output "Invalid choice!" }
}
This code allows users to select an option by entering a letter, and the script responds accordingly. The use of a switch statement optimizes handling multiple input cases.
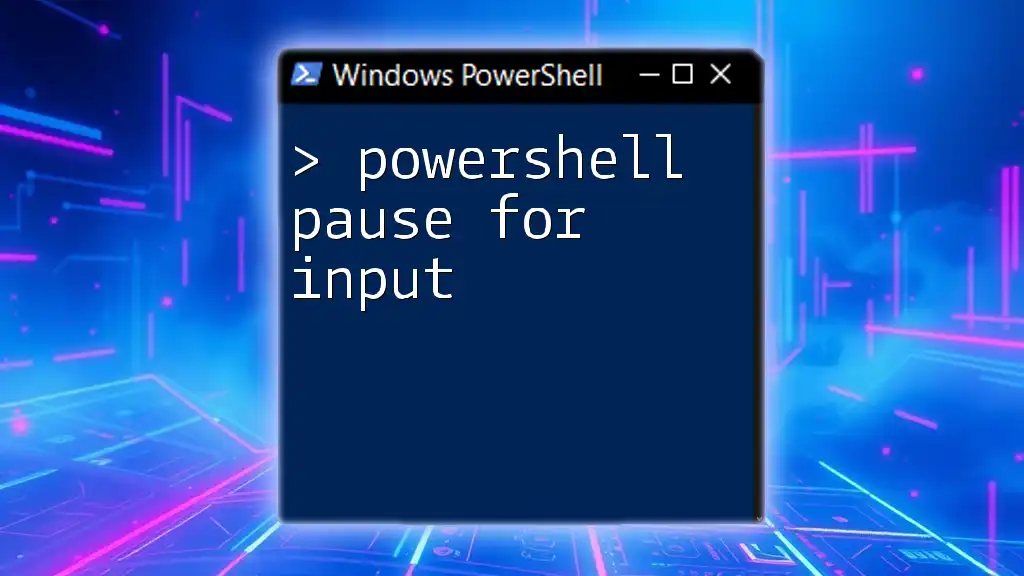
Advanced User Input Techniques
Creating a Functional User Input Form
Beyond command-line interaction, PowerShell allows you to create GUIs for gathering user data, which can be especially useful for non-technical users.
Overview of GUI Options Using Windows Forms, you can design a simple interface that collects user data through text boxes and buttons.
Example Code Snippet Using Windows Forms
Add-Type -AssemblyName System.Windows.Forms
$form = New-Object System.Windows.Forms.Form
$form.Text = "User Input Form"
$form.Size = New-Object System.Drawing.Size(300,200)
$textbox = New-Object System.Windows.Forms.TextBox
$textbox.Location = New-Object System.Drawing.Point(50,50)
$form.Controls.Add($textbox)
$button = New-Object System.Windows.Forms.Button
$button.Text = "Submit"
$button.Location = New-Object System.Drawing.Point(50,100)
$button.Add_Click({ $name = $textbox.Text; [System.Windows.Forms.MessageBox]::Show("Hello, $name!") })
$form.Controls.Add($button)
$form.ShowDialog()
In this code, a basic form is created with a text box for input and a button to submit the input. When pressed, the button captures the user's name and displays a greeting message.
Using InputBox for Quick User Input
The InputBox function is useful for quick prompts, especially suitable for obtaining simple strings without the need for complex forms.
What is InputBox? This approach displays a dialog box, allowing users to input data quickly.
Example Usage
$userInput = [System.Windows.Forms.MessageBox]::Show("Enter your input:", "Input Required", [System.Windows.Forms.MessageBoxButtons]::OKCancel)
This code provides a simple dialog box and can be extended to capture actual input rather than just a confirmation.
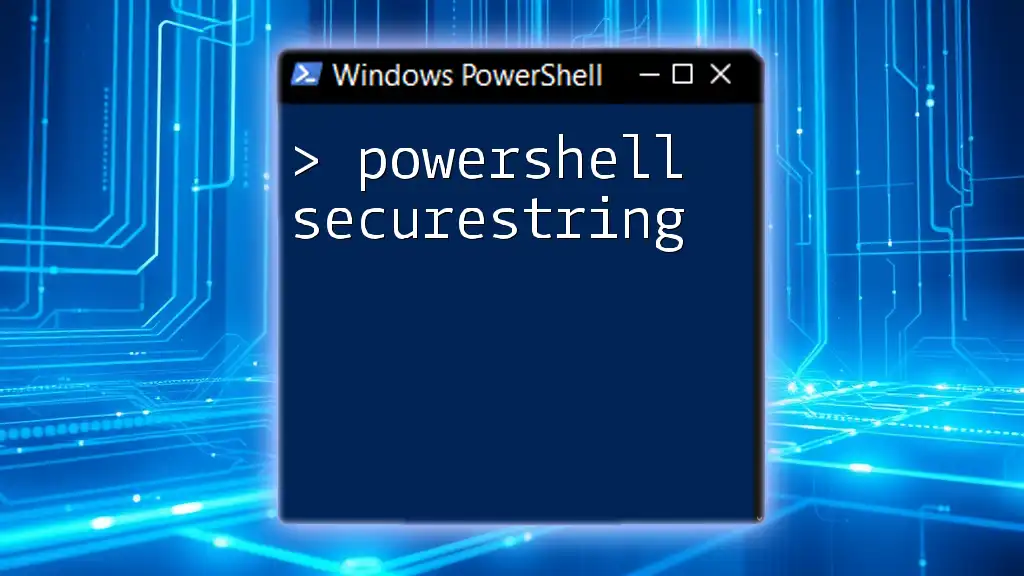
Examples of User Input in Real-World Scenarios
Dynamic Parameterization of Scripts
One common use of user input in PowerShell is dynamically configuring scripts based on user actions. This approach contributes significantly to automated tasks.
Example: Backup Script with User Input for Source/Destination
$source = Read-Host -Prompt "Enter backup source path"
$destination = Read-Host -Prompt "Enter backup destination path"
Copy-Item -Path $source -Destination $destination -Recurse
This script allows users to specify where to copy files from and where to place them, eliminating the need for hardcoded paths.
User Input for Configuration Files
User input can also be used for generating or modifying configuration files, simplifying setup processes for various applications.
Example Script
$settingValue = Read-Host -Prompt "Please enter the configuration value"
Set-Content -Path "C:\Config\settings.config" -Value $settingValue
This script captures a configuration setting from the user and saves it to a specified file, demonstrating how user input can streamline the configuration process.
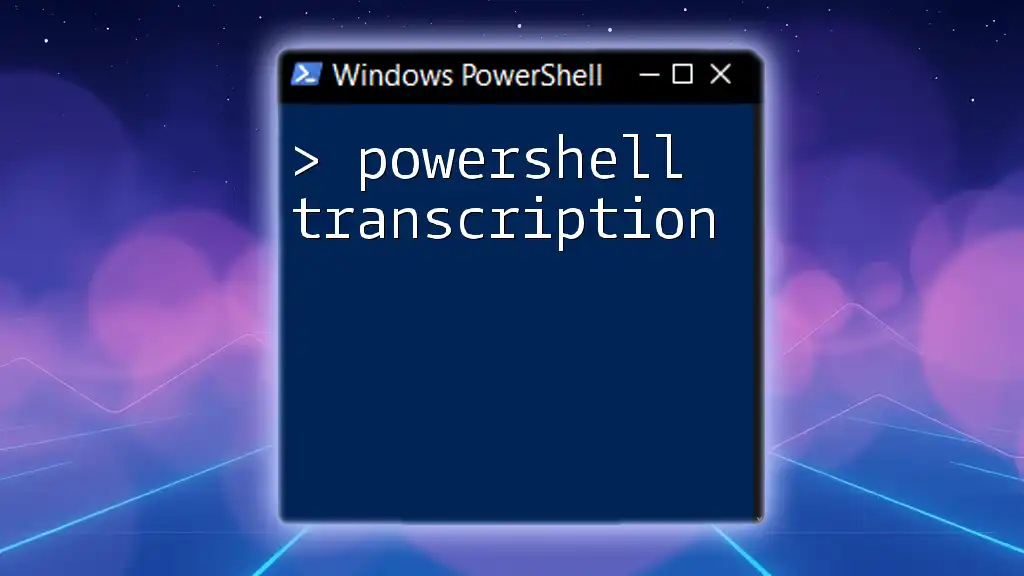
Conclusion
Incorporating user input into your PowerShell scripts not only enhances their interactivity but also allows for personalization and flexibility. From simple prompts with `Read-Host` to complex GUI applications, PowerShell provides various ways to collect and utilize user input effectively. Explore these techniques further to empower your scripting capabilities and create more dynamic and user-friendly scripts.