To exit a PowerShell script gracefully, you can use the `Exit` command followed by an optional exit code to signify the script's termination status.
Exit 0 # Exits the script with a success code
Understanding the Need to Exit a PowerShell Script
In PowerShell scripting, it’s crucial to know when and how to properly terminate a script. Exiting a script can be necessary for several reasons:
- Error Handling: When a script encounters an error that it cannot recover from, it should exit to prevent further issues.
- User Interruptions: Users may need to stop a running script if they realize it is not producing the desired results.
- Unmet Running Conditions: Sometimes, a script may rely on certain prerequisites. If those conditions are not met, terminating the script is the best course of action.
Failing to exit a script properly could lead to unpredictable behavior and resource wastage, making it essential to implement exit strategies effectively.
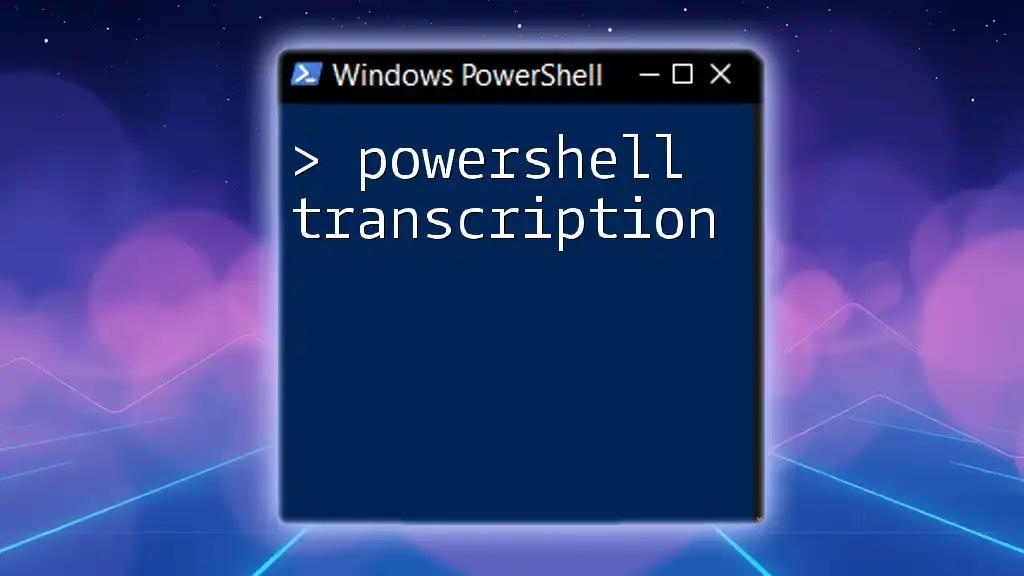
Methods to Exit from PowerShell Scripts
Using the `Exit` Command
The simplest way to exit a PowerShell script is to use the `exit` keyword. This command will terminate the script immediately, making it very effective for conditions where you need to stop execution without further processing.
Example of using `exit`:
if ($errorCondition) {
exit
}
In this example, the script will exit if the condition `$errorCondition` evaluates to true.
Using `return` for Exiting Functions
While `exit` terminates the entire script, `return` is used to stop the execution inside a specific function. It's essential to differentiate between the two, as they serve different purposes in scripting.
Example of a function using `return`:
function Test-Exit {
if ($condition) {
return
}
# additional code here
}
In this case, the function `Test-Exit` will stop executing further code if `$condition` is true, allowing for a more granular control within functions.
Raising Errors to Stop Execution
Another method to exit a PowerShell script is by utilizing the `throw` command, which raises an error. When this command is called, it stops script execution and can also provide a message indicating why the script was terminated.
Example of raising an error:
throw "An error occurred; terminating script."
In this example, an error message is showcased, highlighting the importance of clear communication in scripts.
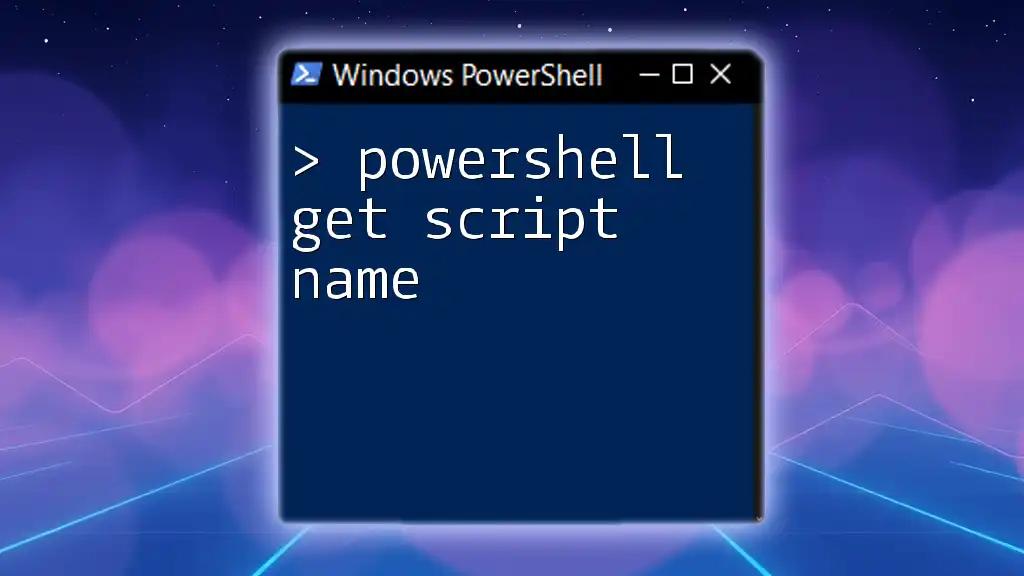
Stopping Script Execution: Advanced Techniques
Using `Try-Catch` Blocks
PowerShell allows for robust error handling through `try-catch` blocks. This approach ensures that any errors can be caught and managed gracefully. When an error occurs, control passes to the catch block, where you can decide to exit the script.
Example of using try-catch to stop script execution:
try {
# some code that might fail
} catch {
Write-Host "Error encountered, exiting script."
exit
}
In this way, the script will notify the user about the issue and then exit accordingly, which enhances maintainability.
Conditional Statements for Controlled Exits
Utilizing conditional statements allows for more controlled exits based on specific criteria. This technique can help maintain the intended flow of the script by halting execution only when it truly makes sense to do so.
Example of conditional exit:
if (-not (Test-Path $path)) {
Write-Host "Path does not exist. Stopping script."
exit
}
In this example, if the specified path does not exist, the script outputs a message and then terminates, preventing unnecessary execution of additional code.
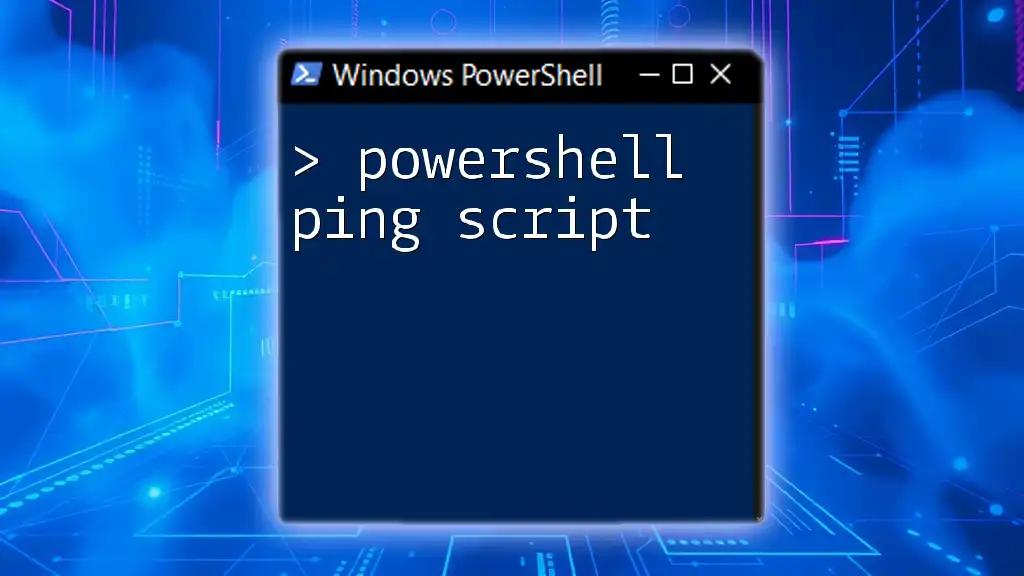
Stopping a Running Script from the Console
Using Keyboard Interrupt
In instances where a script is running in the console, users can exercise control over execution by using a keyboard interrupt. By pressing Ctrl + C, the user can stop a script immediately. This method is particularly useful for long-running scripts where immediate action is required.
Terminating Background Jobs
If you are working with background jobs, PowerShell provides functionality to terminate them using the `Stop-Job` command. It’s beneficial for managing multiple tasks that may be running concurrently.
Example of stopping a job:
$job = Start-Job { Get-Process }
Stop-Job -Job $job
This code initiates a job to get process information and then immediately stops it, showcasing an efficient way to manage script execution in a multi-tasking environment.
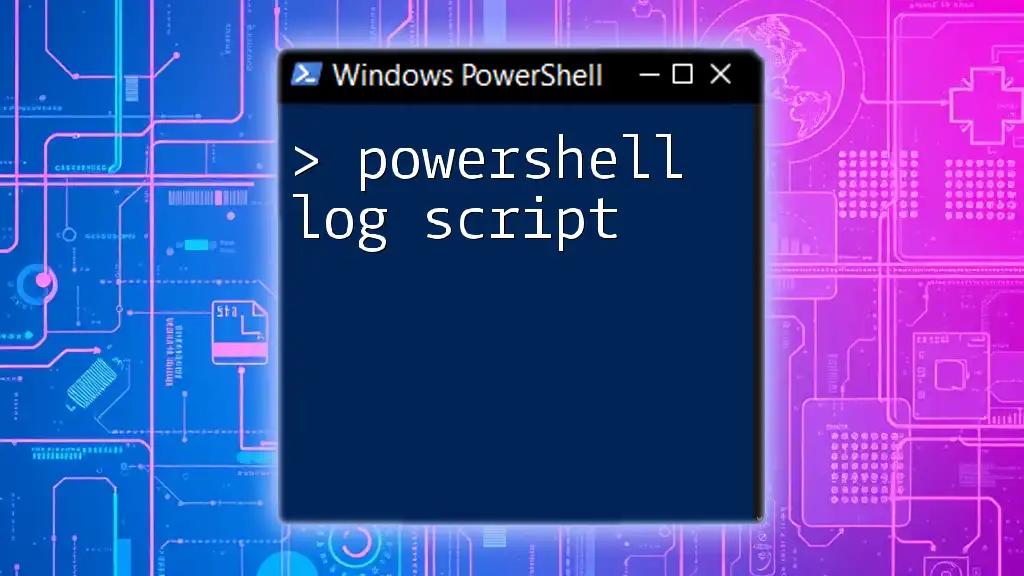
Best Practices for Exiting PowerShell Scripts
To ensure scripts exit properly and avoid issues, consider the following best practices:
- Ensure Clean Exits: Utilize commands that free up resources before exiting to prevent memory leaks or hanging processes.
- Log Reasons for Termination: Documenting why a script was exited can be beneficial for debugging and improving future iterations of the script.
- User Messages: Providing clear messages to users upon termination enhances the user experience and improves understanding.
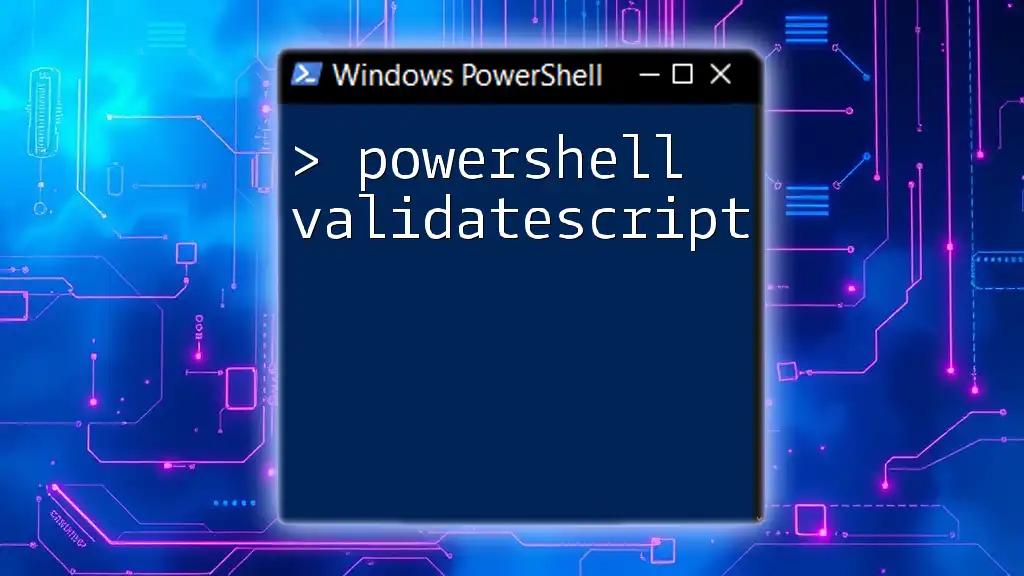
Conclusion
Mastering the nuances of exiting scripts in PowerShell is essential for effective automation. Whether through the `exit` command, using error handling, or employing conditional statements, knowing how to control execution ensures that scripts run smoothly and responsively. By implementing these techniques, you can enhance your PowerShell scripting skills and improve the robustness of your automation activities.
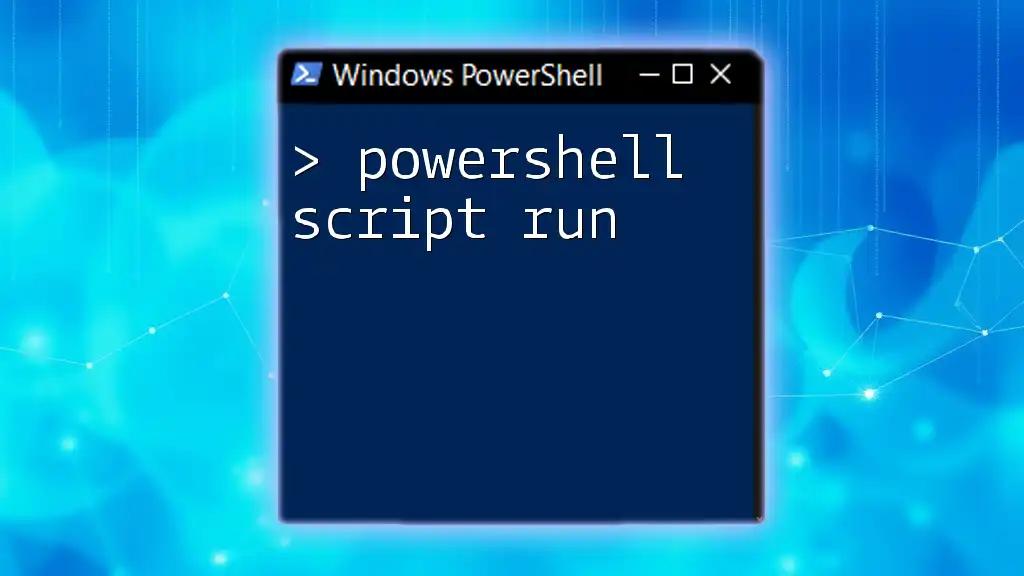
Additional Resources
For further information, it is recommended to explore online tutorials, PowerShell documentation, and community forums where you can engage with other PowerShell users and improve your scripting capabilities.
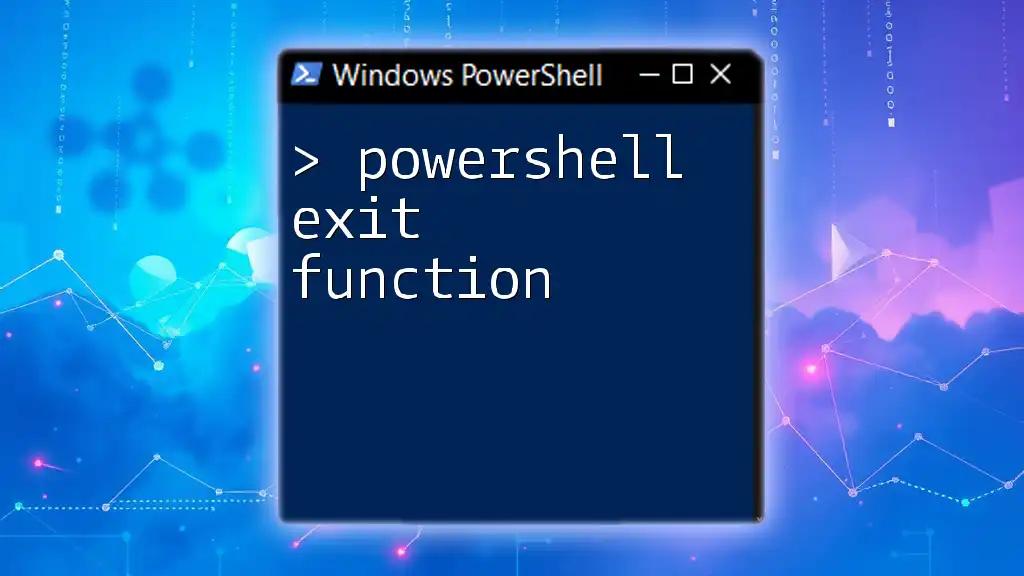
FAQs
What is the difference between exit and return in PowerShell?
While `exit` stops the entire script execution, `return` only stops the execution within a function, making it important to use them appropriately based on the desired outcome.
How do I stop a PowerShell script from running?
This can be achieved through several methods, including using the `exit` command, keyboard interrupts (Ctrl + C), or conditional exits based on specific criteria.
Can I exit PowerShell script based on user input?
Yes! You can implement user prompts to check conditions, using `if` statements to exit if certain user inputs signal a need to stop execution.