You can efficiently search for an object within an array in PowerShell by using the `Where-Object` cmdlet to filter based on specific properties. Here's a code snippet demonstrating how to search for objects with a specific property value:
$arrayOfObjects = @(
@{ Name = 'Alice'; Age = 30 },
@{ Name = 'Bob'; Age = 25 },
@{ Name = 'Charlie'; Age = 35 }
)
$result = $arrayOfObjects | Where-Object { $_.Name -eq 'Bob' }
$result
Understanding Arrays and Objects in PowerShell
What is an Array?
In PowerShell, an array is a data structure that holds multiple values. It's an essential concept in scripting, as it allows you to manage collections of data easily. You can create a simple array by placing a list of values in `@()`:
# Example of a simple array creation
$colors = @("Red", "Green", "Blue")
This array contains three string values: "Red," "Green," and "Blue."
What is an Object?
An object in PowerShell represents a complex data structure with properties and methods. Objects allow you to encapsulate data and functionality in a single entity, making them ideal for modeling real-world scenarios. You can create an object using the `New-Object` cmdlet or a shortcut syntax for `PSCustomObject`:
# Creating a custom object
$student = [PSCustomObject]@{
Name = "Alice"
Age = 20
Grade = "A"
}
In this example, we have created a student object with three properties: `Name`, `Age`, and `Grade`.
Combining Arrays and Objects
When you combine arrays and objects, you can create a collection of objects. This is particularly useful for managing complex data. Here’s how you can create an array of student objects:
# Creating an array of student objects
$students = @(
[PSCustomObject]@{ Name = "Alice"; Age = 20; Grade = "A" },
[PSCustomObject]@{ Name = "Bob"; Age = 22; Grade = "B" },
[PSCustomObject]@{ Name = "Charlie"; Age = 21; Grade = "C" }
)
With this array of objects, we can now efficiently search for specific student data.

Searching in an Array of Objects
Basics of Searching
Searching through an array of objects allows you to filter data based on specified criteria. You can search using object properties (like `Name` or `Age`) or directly for values.
Using `Where-Object` Cmdlet
Syntax and Usage
To search for objects in an array, `Where-Object` is a powerful cmdlet that allows you to filter based on conditions. The syntax uses a script block to define the criteria for filtering.
Here’s a straightforward example of using `Where-Object` to find students with a specific grade:
# Example: Searching for a specific property value
$searchResult = $students | Where-Object { $_.Grade -eq "A" }
In this example, we piped the `$students` array to `Where-Object`, filtering for objects where the `Grade` property is equal to "A."
Filtering Multiple Properties
You can also filter by multiple properties using logical operators like `-and` and `-or`. For instance, to search for students older than 20 with a grade of "B":
# Example: Searching with multiple criteria
$searchResult = $students | Where-Object { $_.Age -gt 20 -and $_.Grade -eq "B" }
This example showcases how you can combine conditions to narrow down your search results effectively.
Using `Select-Object`
Selecting Specific Properties
After filtering, you often want to display only certain properties of the results. The `Select-Object` cmdlet allows you to specify which properties to display from the filtered objects.
For example, if you want only the names of the students who received an "A":
$searchResult | Select-Object Name
This command will list the names of all students filtered by the previous criteria.
Advanced Searching Techniques
Using Regular Expressions
For more complex searches, you can utilize regular expressions with the `-match` operator. This is particularly useful when searching for patterns in string properties.
For instance, find students whose names start with "A":
# Example: Searching with regular expressions
$searchResult = $students | Where-Object { $_.Name -match "^A" }
This example uses a regex pattern to match names starting with "A."
Sorting and Ordering Results
After searching, you may want to sort your results for better readability. Combining the `Sort-Object` cmdlet can help you order the results.
Here’s how you can sort the previously filtered results by age:
# Example: Sorting the results
$sortedResults = $students | Where-Object { $_.Grade -ne "C" } | Sort-Object Age
In this command, it first filters out students with a grade "C" and then sorts the remaining results by their `Age`.
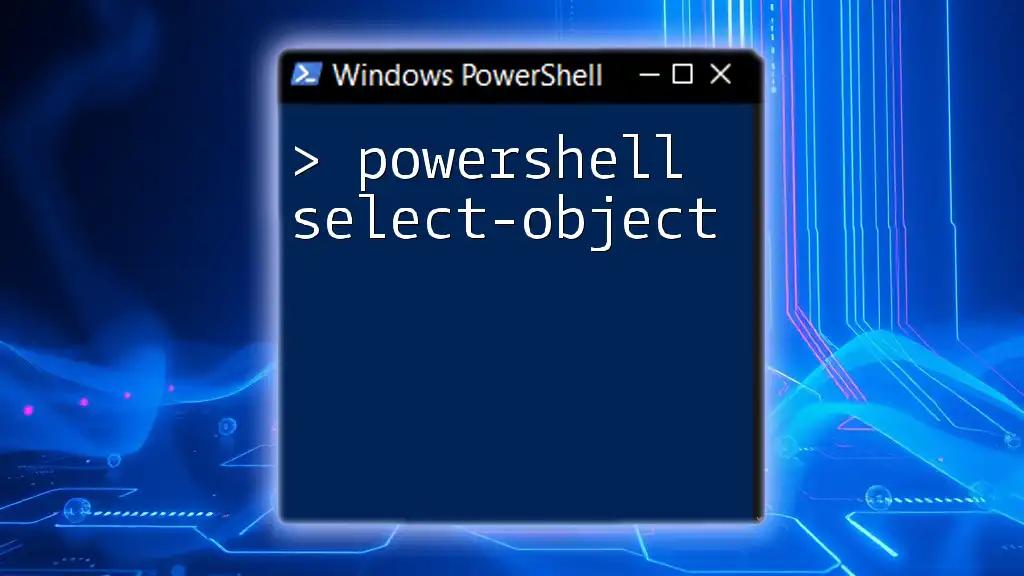
Common Use Cases
Searching for Specific Conditions
Searching for specific conditions in an array of objects is common in scenarios like filtering logs, user records, or data fetched from databases or APIs. By efficiently narrowing down the data, you can extract meaningful insights.
Automation Scripts
In the context of automation scripts, searching within arrays of objects can greatly enhance efficiency. For example, you might have a script that identifies outdated user accounts within an array comprised of multiple user objects
# Example of finding outdated user accounts
$users = @(
[PSCustomObject]@{ Username = "jdoe"; LastLogin = (Get-Date).AddDays(-30) },
[PSCustomObject]@{ Username = "asmith"; LastLogin = (Get-Date).AddDays(-10) }
)
$outdatedUsers = $users | Where-Object { $_.LastLogin -lt (Get-Date).AddDays(-14) }
This snippet finds user accounts that haven’t logged in for over two weeks, showing how searching in object arrays can facilitate decision-making processes.

Performance Considerations
Efficiency of Searching Techniques
When working with large datasets, it's crucial to consider the efficiency of your searches. Using `Where-Object` on large collections can lead to performance issues, mainly because it filters in-memory.
To optimize performance:
- Limit the number of objects processed by narrowing your search as early as possible in your script.
- Consider using `ForEach-Object` with custom logic for more complex operations.
Efficient Handling of Nested Arrays or Complex Objects
When dealing with nested arrays or more complex objects, understanding the structure is vital for efficient searches. Break down the structures and ensure you access properties correctly to enhance performance.

Conclusion
In summary, mastering the technique of PowerShell search in arrays of objects can empower you to handle data more effectively. With tools like `Where-Object` and `Select-Object`, coupled with advanced techniques utilizing regex and sorting, you can conduct thorough searches tailored to your needs. These skills are invaluable for automating tasks and managing data efficiently in various scenarios.
Call to Action
To further enrich your PowerShell abilities, consider subscribing to our channels for more tips and tricks. Additionally, we offer downloadable resources such as cheat sheets to serve as quick references for PowerShell commands related to arrays and objects. Armed with this knowledge, you'll be well-prepared to tackle any data searching tasks in PowerShell.