To delete the contents of a folder in PowerShell, you can use the `Remove-Item` cmdlet combined with the `-Recurse` flag, as shown below:
Remove-Item 'C:\Path\To\Your\Folder\*' -Recurse -Force
Understanding PowerShell and File Management
PowerShell is a powerful scripting language and shell designed specifically for system administration. With its ability to automate tasks and manage various Windows components seamlessly, it's a favorite among IT professionals and developers alike.
When it comes to file management, PowerShell allows users to efficiently manipulate files and folders through a vast array of cmdlets. Understanding how to utilize these cmdlets effectively can save a lot of time, especially when deleting the contents of a folder.
Why Use PowerShell for Deleting Folder Contents?
Automation is one of the most significant advantages of using PowerShell for file management. Whether you're managing large directories or performing repetitive tasks, PowerShell simplifies the process, allowing you to handle content with minimal effort.
For example, instead of manually navigating through directories to delete files, a simple command can accomplish this in seconds. Furthermore, PowerShell offers the ability to script these actions, enabling users to create reusable code that can be modified for various purposes.
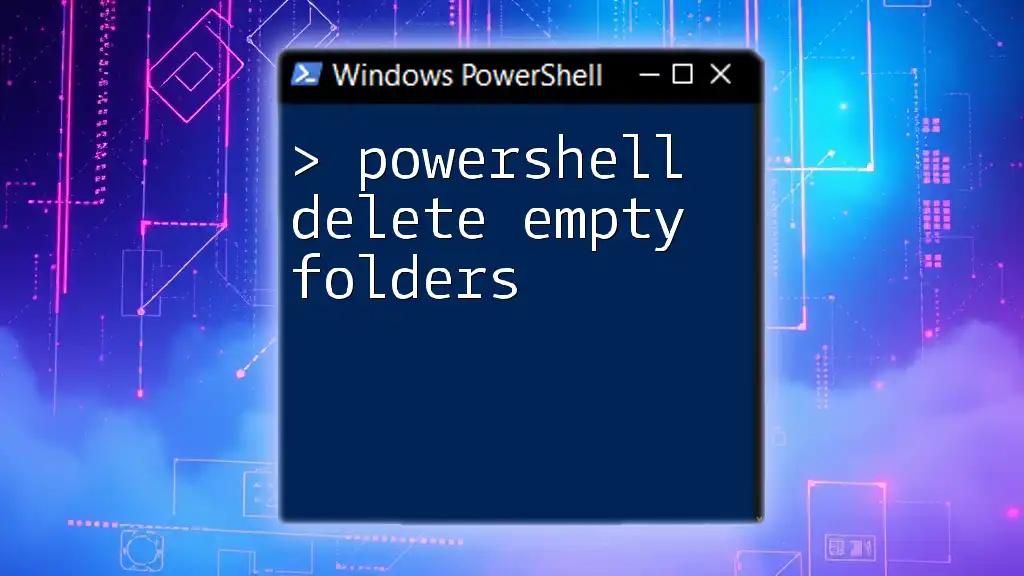
Basics of File Manipulation in PowerShell
Key Cmdlets for File Management
Two essential cmdlets play a pivotal role in managing file contents in PowerShell:
-
Get-ChildItem: This cmdlet retrieves the items (files and folders) in a specified location. For example, if you wish to see the contents of a folder, you would use:
Get-ChildItem -Path C:\Folder\
-
Remove-Item: This cmdlet is responsible for deleting files and folders. Here's a basic example that demonstrates how to delete all contents within a folder:
Remove-Item -Path C:\Folder\*
Syntax Overview
Understanding the syntax of PowerShell cmdlets is crucial. Generally, cmdlets follow this pattern:
<Verb>-<Noun> -Parameter Value
The cmdlet's verb indicates the action to be performed (e.g., `Remove`), while the noun specifies the object being affected (e.g., `Item`). Parameters are additional arguments that modify the cmdlet's behavior, such as `-Recurse` when wanting to delete contents in subdirectories.
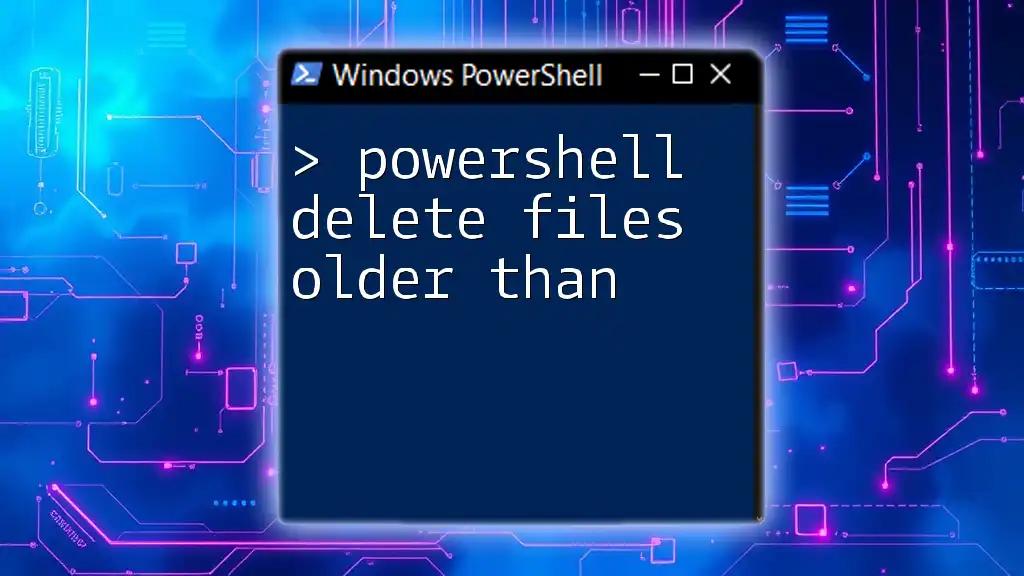
Step-by-Step Guide to Deleting Folder Contents
Preparing to Delete Files
Before executing any deletion commands, it's essential to backup important files. Accidental deletions can lead to significant data loss. You can use the following command to create a backup of files:
Copy-Item -Path C:\Folder\* -Destination C:\Backup\ -Recurse
This copies all files from `C:\Folder` to `C:\Backup`, preserving the hierarchy.
Deleting All Files and Subfolders
Once you've ensured that you have a backup, deleting all contents can be done easily with the `Remove-Item` cmdlet. The command below deletes everything within the specified directory, including subfolders:
Remove-Item -Path C:\Folder\* -Recurse
Adding the `-Recurse` parameter ensures that all items in the folder, including any nested folders and their contents, are deleted simultaneously. However, exercise caution with this command, as it will permanently erase all specified contents.
Deleting Specific File Types
PowerShell also allows you to target specific file types for deletion, which can be an efficient way to clear space or manage files. For instance, to delete all `.txt` files from a folder, use the following command:
Remove-Item -Path C:\Folder\*.txt
Using file extensions and patterns can significantly reduce the risk of accidentally deleting non-targeted files.
Excluding Certain Files from Deletion
By employing the `Where-Object` cmdlet, you can filter files and exclude certain ones from the deletion process:
Get-ChildItem -Path C:\Folder\* | Where-Object { $_.Name -ne 'important-file.txt' } | Remove-Item
In this case, all contents in the specified folder will be deleted, except for `important-file.txt`. This method is particularly useful in scenarios where you need to maintain specific files while clearing out the rest.
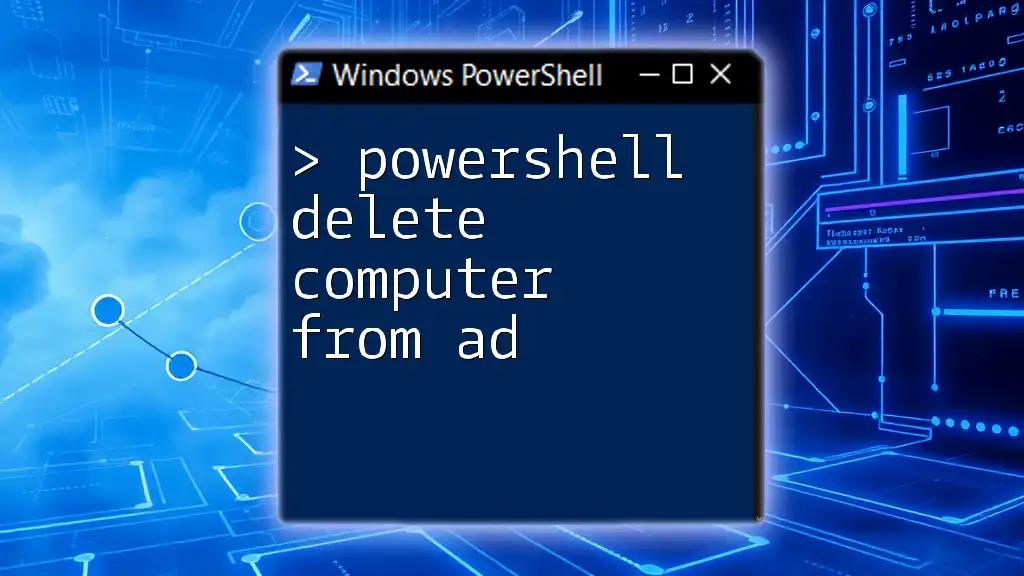
Advanced Techniques
Deleting Files Based on Age
Sometimes, you might want to clean up files based on how old they are. For instance, to delete files older than 30 days, you could use the following script:
Get-ChildItem -Path C:\Folder\* | Where-Object { $_.LastWriteTime -lt (Get-Date).AddDays(-30) } | Remove-Item
In this example, the `LastWriteTime` property is compared with the current date minus 30 days, effectively targeting older files for deletion.
Confirming Deletion with Prompts
Before executing a deletion command, adding a confirmation prompt can help prevent accidental loss of data. Use the `-Confirm` parameter in your command:
Remove-Item -Path C:\Folder\* -Recurse -Confirm
This command will prompt you for confirmation before proceeding with the deletion, adding an extra layer of security.
Implementing Try/Catch for Error Handling
PowerShell allows for robust error handling with the `try/catch` blocks. If an error occurs during the deletion process, you can catch the error and handle it gracefully, like this:
try {
Remove-Item -Path C:\Folder\* -Recurse -ErrorAction Stop
} catch {
Write-Host "An error occurred: $_"
}
This method provides user-friendly error messages and helps in troubleshooting issues that may arise during execution.

Safety Measures to Consider
Understanding Risks of Deletion
It's crucial to fully understand the risks associated with the Remove-Item cmdlet. Once a file or folder is deleted, it is generally not recoverable unless backups have been made. Always double-check the target path before executing deletion commands to avoid unwanted data loss.
Alternatives to Permanent Deletion
For those concerned about the permanence of deleting files, consider alternatives that move files to the recycle bin instead. While PowerShell does not have a built-in recycle bin cmdlet, you can utilize third-party modules or scripts to achieve this functionality.

Conclusion
In summary, mastering the commands to PowerShell delete contents of a folder can enhance your file management capabilities significantly. By leveraging cmdlets like `Remove-Item`, `Get-ChildItem`, and employing various techniques for filtering and error handling, you can execute deletions more effectively and safely. Always remember to backup important data before performing bulk deletions.
As you continue to practice and explore PowerShell, implementing these principles will foster better file management, saving you both time and frustration in the long run.