When you encounter "Access Denied" while using the `Get-Content` command in PowerShell, it usually means that you lack the necessary permissions to read the specified file.
Here’s a code snippet to illustrate checking the file permissions:
Get-Acl "C:\path\to\your\file.txt" | Format-List
Understanding the Get-Content Cmdlet
What is Get-Content?
The `Get-Content` cmdlet is an essential PowerShell command that allows users to read the content of a file. It can handle various types of files, including text files, CSVs, and logs, making it a versatile tool for system administrators and power users alike. By using this cmdlet, you can extract lines of text from files directly in your command line interface.
Syntax of Get-Content
The general syntax of the `Get-Content` cmdlet is straightforward:
Get-Content -Path <String> [-Encoding <String>]
- -Path: Specifies the location of the file you want to read.
- -Encoding: Defines the character encoding of the file (options include UTF8, ASCII, etc.).
Example of Usage
To illustrate the use of `Get-Content`, consider this simple command:
Get-Content -Path "C:\example\file.txt"
This command attempts to read the content of "file.txt" located in the "C:\example" directory and outputs each line to the console.
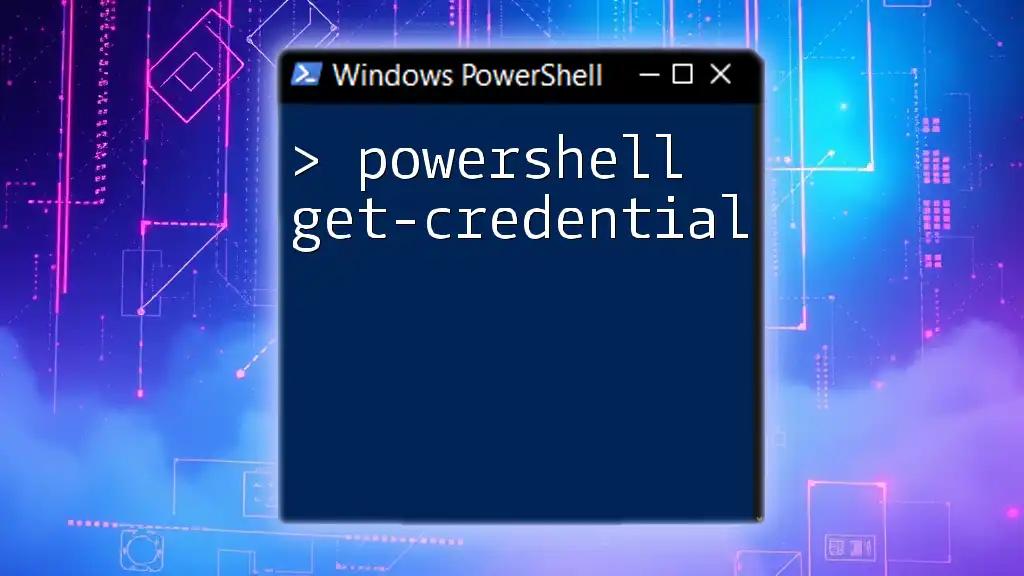
Analyzing the Access Denied Error
What Causes Access Denied Errors?
When you encounter the error message "Access Denied" while trying to use `Get-Content`, it is typically due to permissions issues. The user account executing the command may not have adequate permissions to read the file or access the folder. Other possible causes include:
- File or Folder Ownership: The account does not own the file and lacks permission to read it.
- Active Directory Policies: Group policies may restrict access to certain files or folders.
Common Scenarios Leading to Access Denied
There are specific situations where you are likely to run into access denial:
- Attempting to access system files located in directories like `C:\Windows` or `C:\Program Files`.
- Trying to retrieve content from a network drive without proper access rights.
Example of Access Denied
Attempting to run the following command will deliberately trigger an access denied error:
Get-Content -Path "C:\Windows\System32\config\SAM"
When executing this command, you will likely see an error message stating that access is denied, emphasizing the importance of permissions management.
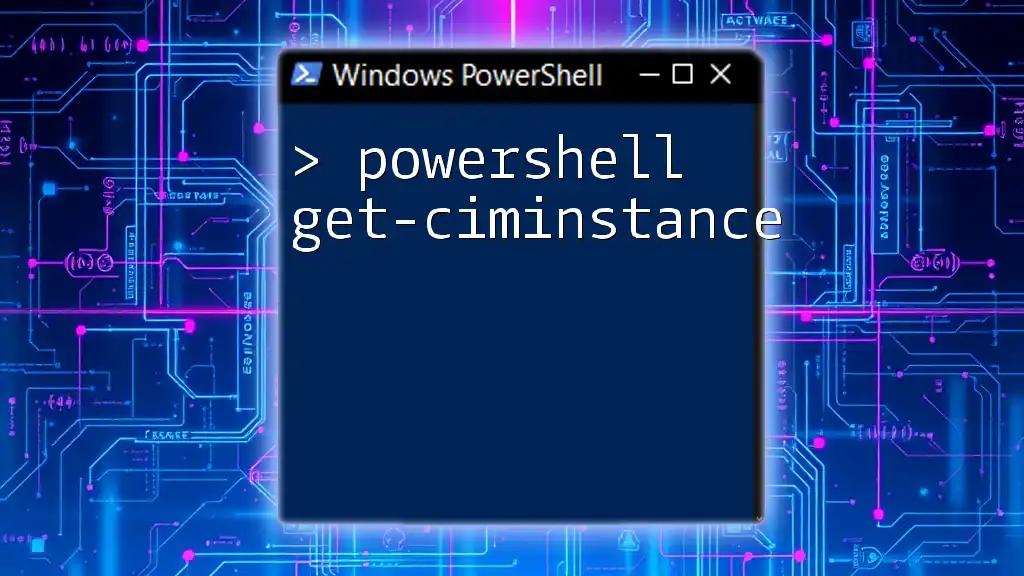
Troubleshooting Access Denied Errors
Checking File Permissions
Before making any changes, it’s essential to review the permissions of the file in question. To do this via PowerShell, use the `Get-Acl` cmdlet:
Get-Acl -Path "C:\example\file.txt"
The output will provide you with insights on the current permissions for the file, including who owns the file and what access rights are granted to various users and groups.
Changing Permissions
If you discover that the permissions need to be altered, you can change them using PowerShell. For example, to grant read access to a specific user, you may use the following commands:
$acl = Get-Acl "C:\example\file.txt"
$permission = "domain\user","Read","Allow"
$a = New-Object System.Security.AccessControl.FileSystemAccessRule($permission)
$acl.SetAccessRule($a)
Set-Acl "C:\example\file.txt" $acl
Caution: Modifying file permissions should be done carefully, as incorrect settings can lead to unintended access issues or security vulnerabilities.
Running PowerShell as Administrator
Often, running PowerShell with elevated privileges will resolve access denied errors. To do so, right-click on the PowerShell icon and select Run as Administrator. This grants the session higher privileges and may allow you to run the `Get-Content` command without encountering access issues.
Using the -ErrorAction Parameter
To manage errors more gracefully, use the `-ErrorAction` parameter, which allows you to specify how PowerShell should respond to errors during execution:
Get-Content -Path "C:\protected.file" -ErrorAction Stop
By setting `-ErrorAction` to `Stop`, execution will terminate immediately upon encountering an error, and you'll receive more explicit error information.

Alternative Methods to Access Content
Using Other Cmdlets
If `Get-Content` continues to cause issues, consider alternative cmdlets that fulfill similar functions. For example, the `Get-Item` and `Get-ItemProperty` cmdlets can sometimes be used to access filemeta-data or check for file existence before attempting to read the content.
Reading Files with Different Approaches
In cases where `Get-Content` may not work due to access restrictions, you can employ .NET classes for reading files. For instance, the following command employs the .NET framework to read all text from a file:
[System.IO.File]::ReadAllText("C:\example\file.txt")
While this approach may bypass certain limitations, it's essential to note that it still requires permission to access the file.
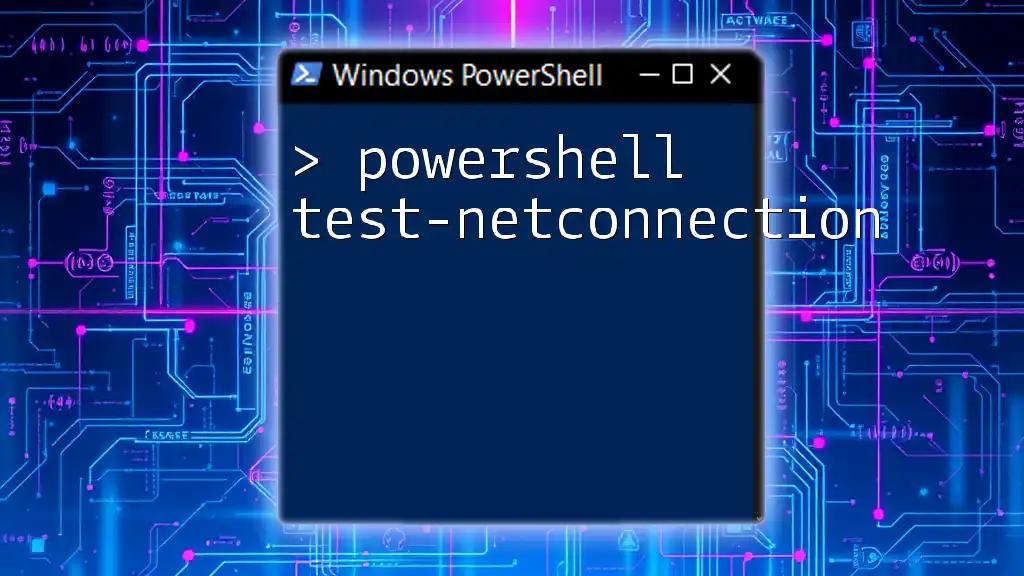
Best Practices
Regularly Audit Permissions
To maintain a secure and efficient working environment, regularly auditing file and folder permissions is paramount. This practice ensures that only authorized personnel have access to sensitive data and system files.
Utilize Proper Exception Handling
Incorporating exception handling into your scripts can save you time and headaches when encountering errors. Here’s an example of how to implement it using a try/catch construct:
try {
Get-Content -Path "C:\example\file.txt"
} catch {
Write-Host "Error occurred: $_"
}
This approach allows you to capture errors dynamically and display more user-friendly error messages.
Educate Yourself on PowerShell Security
Lastly, staying informed about PowerShell security best practices is crucial. Always adhere to your organization's guidelines regarding permissions and access control to prevent unauthorized access and ensure a robust security posture.
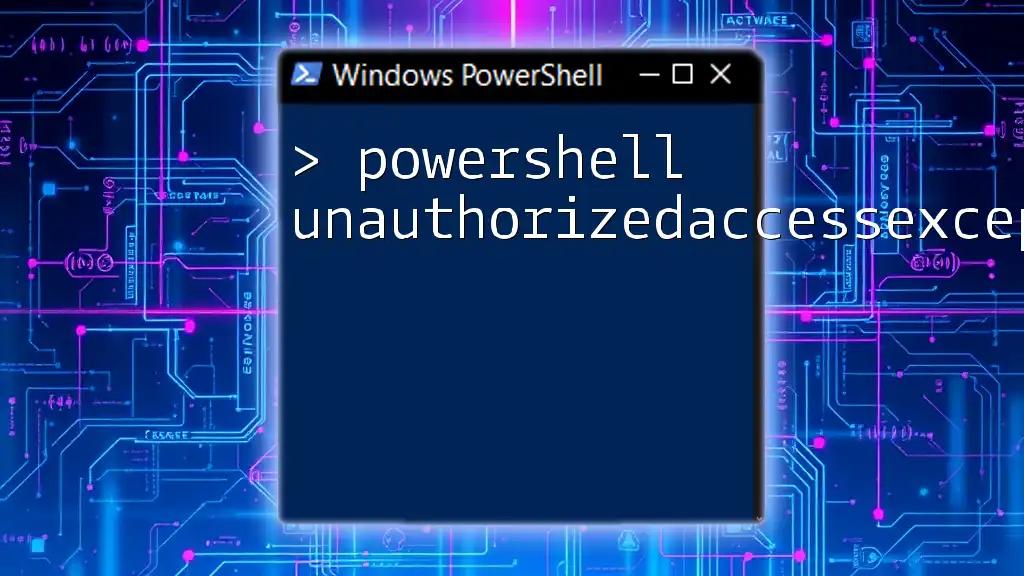
Conclusion
Encountering the powershell get-content access denied error can be a common obstacle for users. However, understanding the underlying causes, employing effective troubleshooting techniques, and adhering to best practices can help you overcome these challenges and utilize `Get-Content` efficiently in your PowerShell scripting endeavors.