In PowerShell, you can pass parameters to a `.ps1` file by defining the parameters in the script and using command-line arguments when invoking the script, like so:
# MyScript.ps1
param(
[string]$Name
)
Write-Host "Hello, $Name!"
To execute the script with a parameter, you would run:
.\MyScript.ps1 -Name "World"
What are Parameters in PowerShell?
Parameters are a fundamental concept in PowerShell that allow you to pass data into scripts, making them more dynamic and flexible. By leveraging parameters in a `.ps1` script, you can tailor the behavior of your code based on input values.
Types of Parameters
Understanding the types of parameters is crucial for effectively utilizing them in your scripts. There are generally two primary types:
- Mandatory Parameters are required for the script to run successfully. Failure to provide these will result in an error.
- Optional Parameters may be omitted; if not provided, they can have default values set in the script.
Additionally, parameters can be classified into named and positional categories. Named parameters are specified by their name, while positional parameters rely on their order in relation to other parameters.
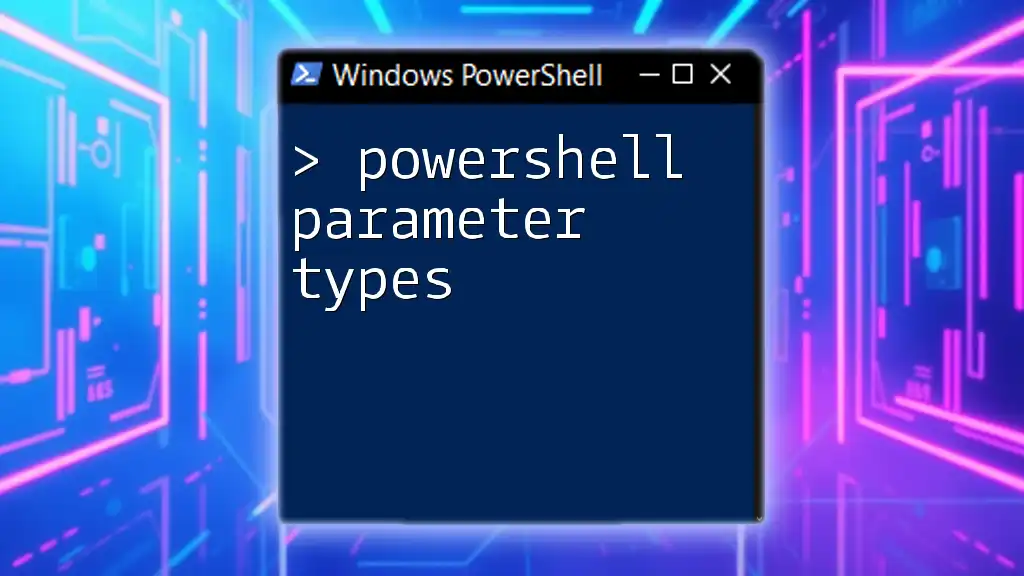
How to Define Parameters in a PS1 File
To define parameters within your PowerShell script, you use the `param` block. This is where you specify the parameters that your script will accept, including their types.
Using the Param Block
The typical syntax for defining parameters is:
param(
[string]$name,
[int]$age
)
In this example, `$name` is a string parameter, and `$age` is an integer. This structure creates clear expectations about the input your script will handle.
Advanced Parameter Attributes
PowerShell provides advanced attributes through the [Parameter()] attribute that customize the behavior of your parameters. You might use attributes like `Mandatory`, `Position`, and `ValueFromPipeline` to enhance the parameters' functionality.
For example:
param(
[Parameter(Mandatory=$true)]
[string]$name,
[Parameter(Position=1)]
[int]$age
)
In this case, the `Mandatory` attribute ensures that the user must provide a value for `$name`, while the `Position` attribute allows you to specify the expected order of parameters.
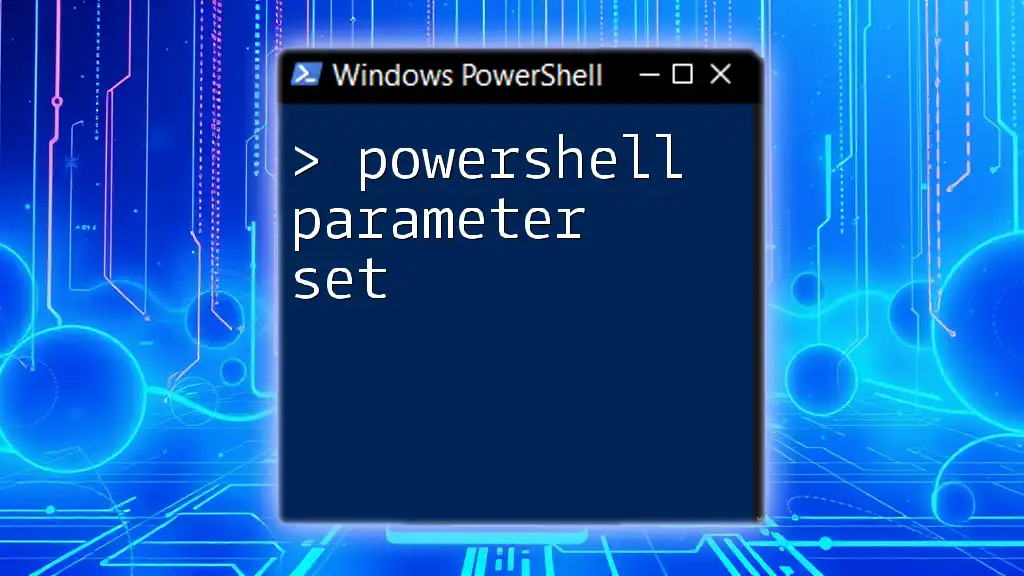
How to Call a PS1 File with Parameters
Once your parameters are defined, calling your script and passing parameters is straightforward.
Basic Invocation
To run your `.ps1` script with parameters, the syntax is as follows:
.\script.ps1 -name "John" -age 30
In this line, you directly pass named parameters, ensuring clarity in what values are being assigned.
Using Positional Parameters
You can also use positional parameters, which allow you to skip explicitly naming them as long as you provide them in the correct order. The command would look like this:
.\script.ps1 "John" 30
In this case, `"John"` is assigned to `$name`, and `30` is assigned to `$age` based on their position in the command.
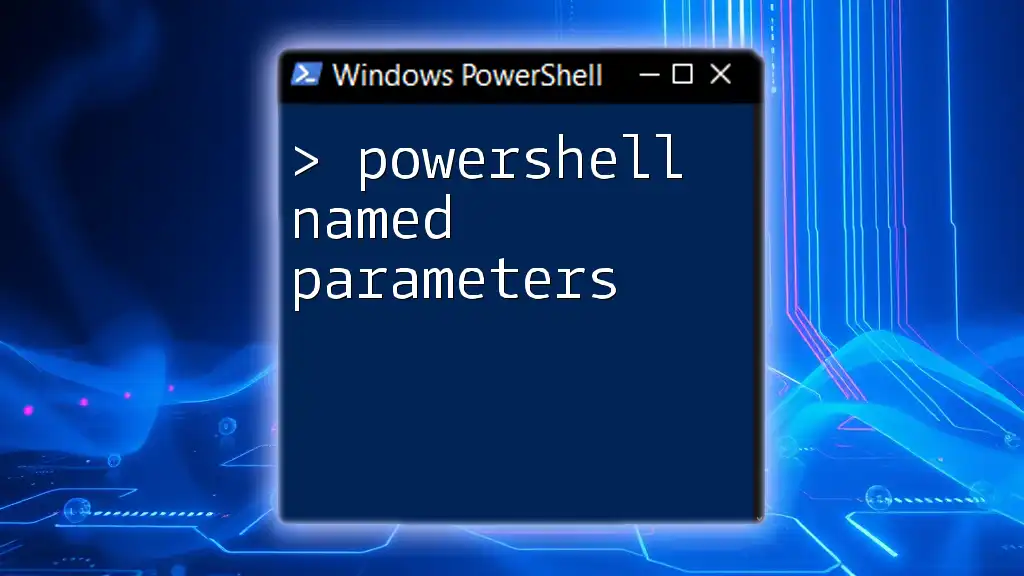
Handling Parameters in the Script
Once parameters are passed to the script, you can utilize their values throughout your code.
Accessing Parameter Values
You simply reference parameter values directly within your script:
Write-Host "Hello, $name! You are $age years old."
This command uses the values of `$name` and `$age` to output a friendly message.
Default Values for Optional Parameters
For optional parameters, you can set default values that will be assigned when users don’t provide input:
param(
[string]$name = "Guest"
)
In this example, if the user does not specify a value for `$name`, it defaults to `"Guest"`.
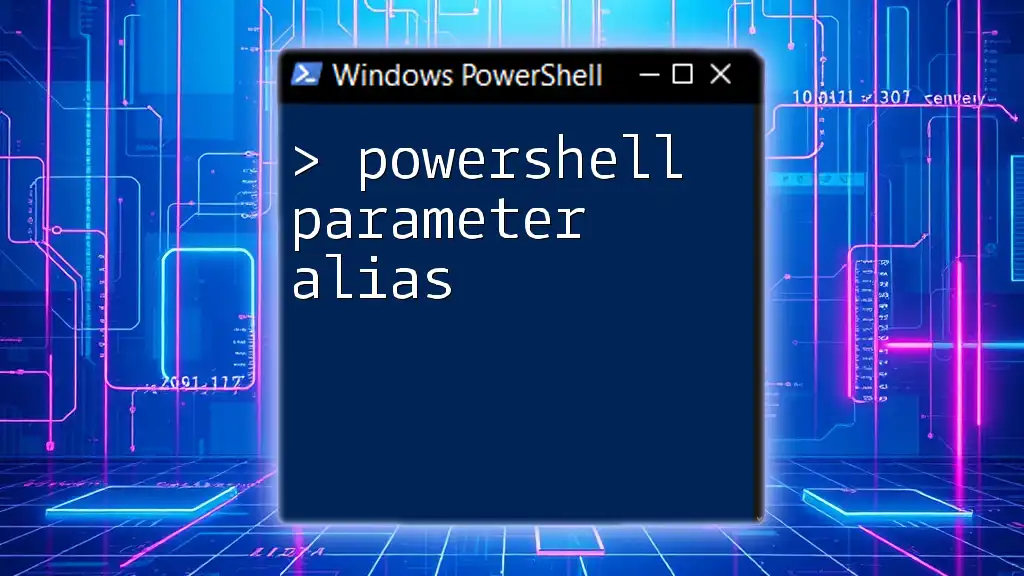
Error Handling with Parameters
Effective error handling is crucial for creating robust scripts.
Validation of Inputs
You can validate parameters to ensure users provide appropriate input. Using attributes like [ValidateRange()] helps in restricting the range of allowable values:
param(
[Parameter(Mandatory=$true)]
[ValidateRange(1, 120)]
[int]$age
)
This example enforces that `$age` must be between 1 and 120, automatically generating an error if the condition is not met.
Using Try-Catch to Handle Runtime Errors
To further fortify your scripts, you can implement `try-catch` blocks for error handling:
try {
# Some operation
Write-Host "Processing age: $age"
} catch {
Write-Host "An error occurred: $_"
}
This structure allows your script to continue running smoothly while offering informative feedback on any encountered issues.
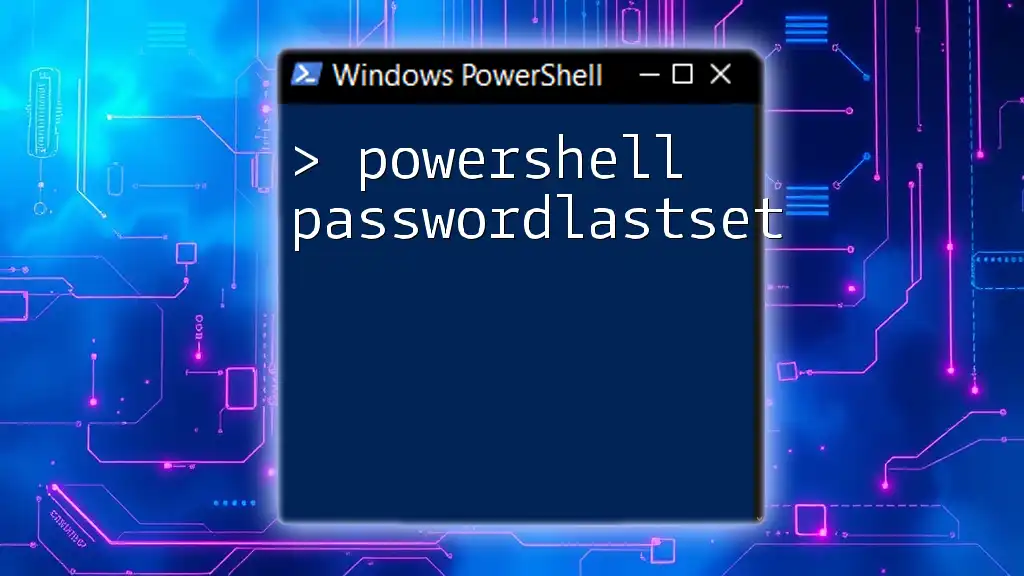
Best Practices for Using Parameters
To ensure that your scripts are clear and maintainable, adhere to these best practices:
- Clear Naming Conventions: Choose meaningful names for your parameters that clearly define their purpose. For example, using `$userName` instead of a generic `$name` will provide clarity.
- Documentation within the Script: Use comments to describe each parameter at the beginning of your script. This helps other users (or yourself in the future) understand the intended use.
Examples of Good Parameter Practices
When creating scripts, consider the following template as a guiding structure:
param(
[Parameter(Mandatory=$true)]
[string]$userName,
[Parameter()]
[int]$userAge = 18
)
This template clearly defines necessary and optional parameters while contributing to fewer errors during script execution.
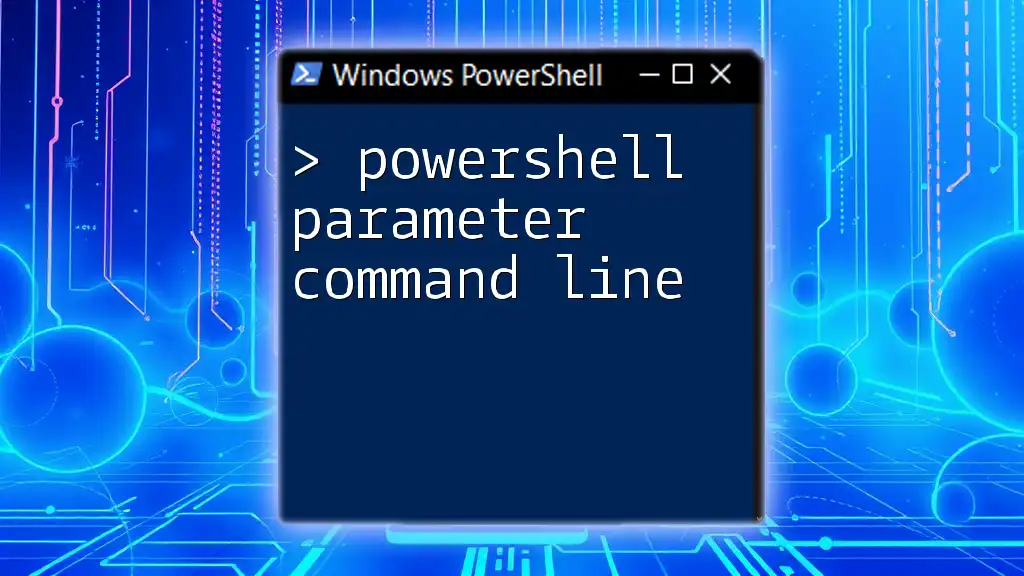
Real-World Use Cases
Example 1: A Script to Process User Data
Consider a hypothetical script designed to process user data where parameters allow for filtering by age and name.
Example 2: Automating Tasks with Parameters
A practical example in automation could involve a script that backs up files. By using parameters to specify source and destination paths, users gain customized backup options without modifying the script.
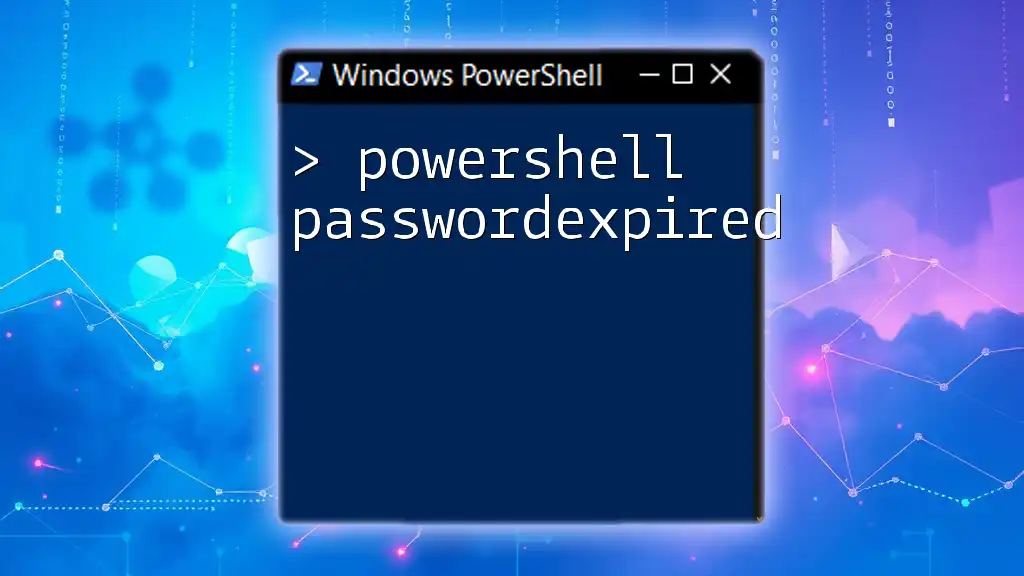
Conclusion
Passing parameters to `.ps1` files in PowerShell enriches your scripts with dynamic capability, fosters better practices, and enhances user experience. Implementing these techniques encourages modular scripts, allowing them to adapt easily within various contexts. By mastering this essential aspect of PowerShell, you empower your automation processes to be more effective and user-friendly. Don’t hesitate to practice these concepts and explore the endless possibilities they offer!
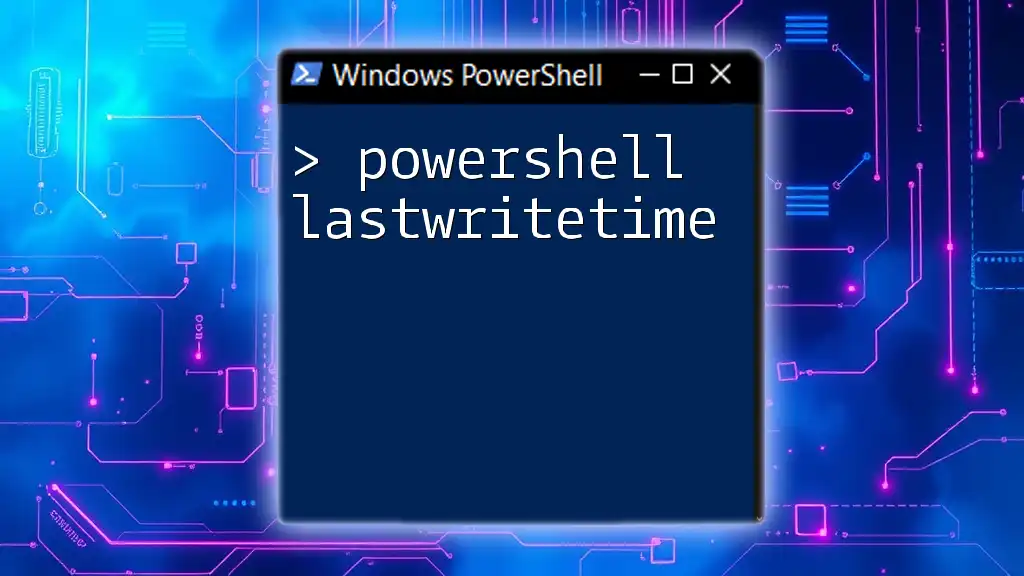
Additional Resources
For further learning, check out the official Microsoft PowerShell documentation and recommended books on the subject that elaborate on the nuances of PowerShell scripting and parameter management.