In PowerShell, you can pass an array to a function by declaring the parameter with the array type and then using the array when calling the function, as shown in the following code snippet:
function Show-ArrayElements {
param ([array]$elements)
foreach ($element in $elements) {
Write-Host $element
}
}
# Example usage
Show-ArrayElements -elements @(1, 2, 3, 4, 5)
Understanding Arrays in PowerShell
Definition of Arrays
In PowerShell, an array is a data structure that can hold multiple values. Unlike a single variable that can store only one value, an array can store a collection of values, making data handling more efficient. Understanding how arrays function is essential for developers looking to simplify their scripts and automate repetitive tasks.
Creating Arrays in PowerShell
To create a one-dimensional array in PowerShell, you can use the `@` symbol:
$myArray = @('value1', 'value2', 'value3')
In this case, `$myArray` will now contain three string values. Arrays can also hold different data types, such as numbers and objects, providing flexibility in programming.
Multidimensional Arrays
PowerShell also supports multidimensional arrays, which allow the storage of data in a grid-like format. This is useful for cases where data needs to be organized in rows and columns. You can create a 2D array as follows:
$my2DArray = @(@('row1col1', 'row1col2'), @('row2col1', 'row2col2'))
In this example, `$my2DArray` is a two-dimensional array that holds values organized in rows.
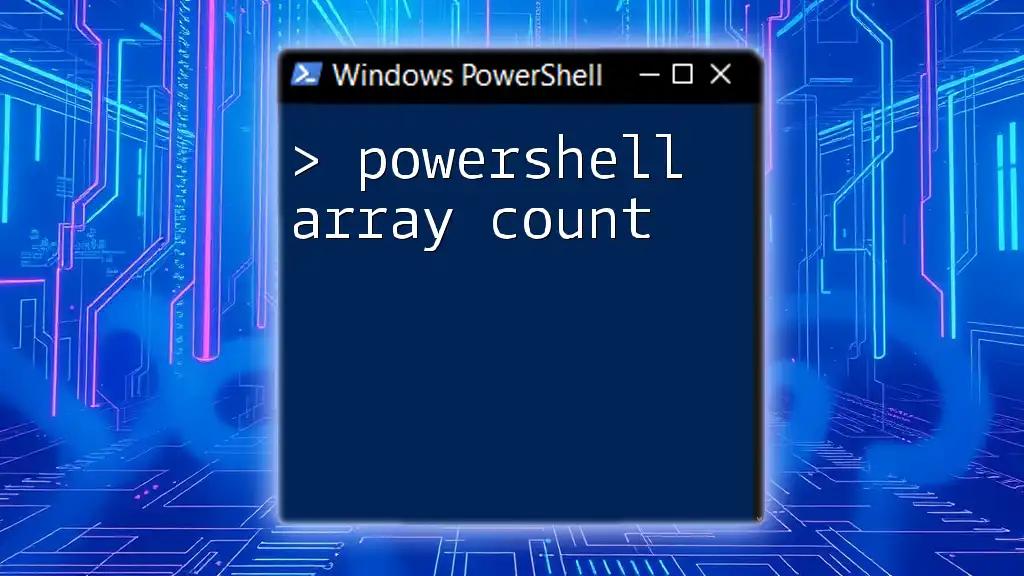
Introduction to Functions in PowerShell
What is a Function?
A function in PowerShell is a reusable block of code designed to perform a specific task. Functions help improve code organization and reusability, making scripts easier to manage.
Creating a Simple Function
Creating a function involves using the `function` keyword followed by the function name and a set of curly braces. Here’s a basic example:
function MyFunction {
param($input)
Write-Output $input
}
This simple function accepts an input parameter and outputs it. As you develop more complex scripts, functions become invaluable.
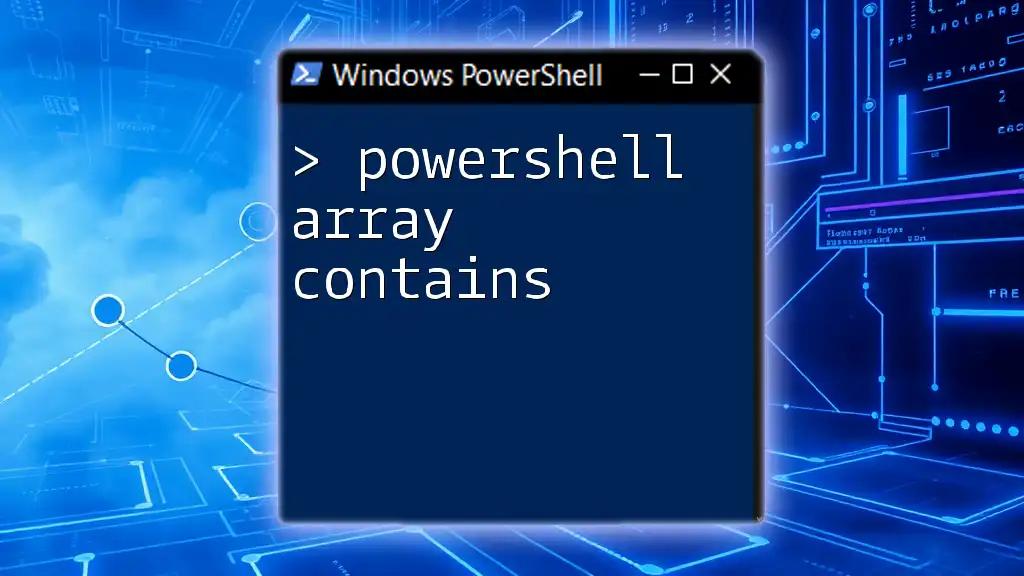
Passing Arrays to Functions
Why Pass Arrays to Functions?
Passing arrays to functions is beneficial for several reasons. First, it allows you to handle multiple values in a single variable, reducing the number of parameters you need to define. Second, using arrays simplifies the management of datasets, particularly in automation tasks.
Syntax for Passing Arrays
To create a function that accepts an array, you need to specify the type of parameter as an array within the `param()` block. For example:
function ProcessArray {
param([string[]]$arrayInput)
foreach ($item in $arrayInput) {
Write-Output $item
}
}
In this function, the parameter `$arrayInput` is defined as an array of strings. The function iterates over each item in the array and outputs it.
Calling the Function with an Array
To call the function, simply pass your array as an argument:
$myArray = @('First', 'Second', 'Third')
ProcessArray -arrayInput $myArray
This will result in the three elements of `$myArray` being outputted by the `ProcessArray` function.
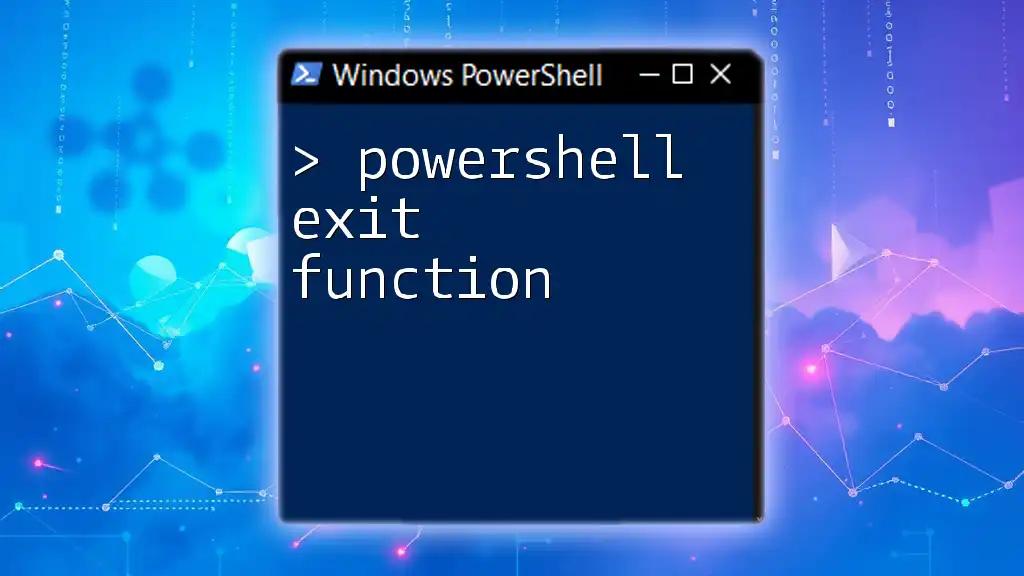
Tips for Working with Arrays in Functions
Validate Input Arrays
Validation is crucial to avoid runtime errors. You can check whether the input is an array using the `-is` operator. Here's an example of how to implement this in a function:
function ValidateAndProcessArray {
param([array]$inputArray)
if ($inputArray -isnot [array]) {
throw "Input is not an array."
}
foreach ($item in $inputArray) {
Write-Output $item
}
}
In this function, if the input is not an array, an error message will be thrown.
Handling Empty or Null Arrays
It's essential to manage cases where the input array might be empty or null. A good practice is to provide default values:
function HandleEmptyArray {
param([string[]]$arrayInput)
if (-not $arrayInput) {
$arrayInput = @('default1', 'default2')
}
foreach ($item in $arrayInput) {
Write-Host $item
}
}
In this example, if `$arrayInput` is not provided, it defaults to an array containing two preset values.
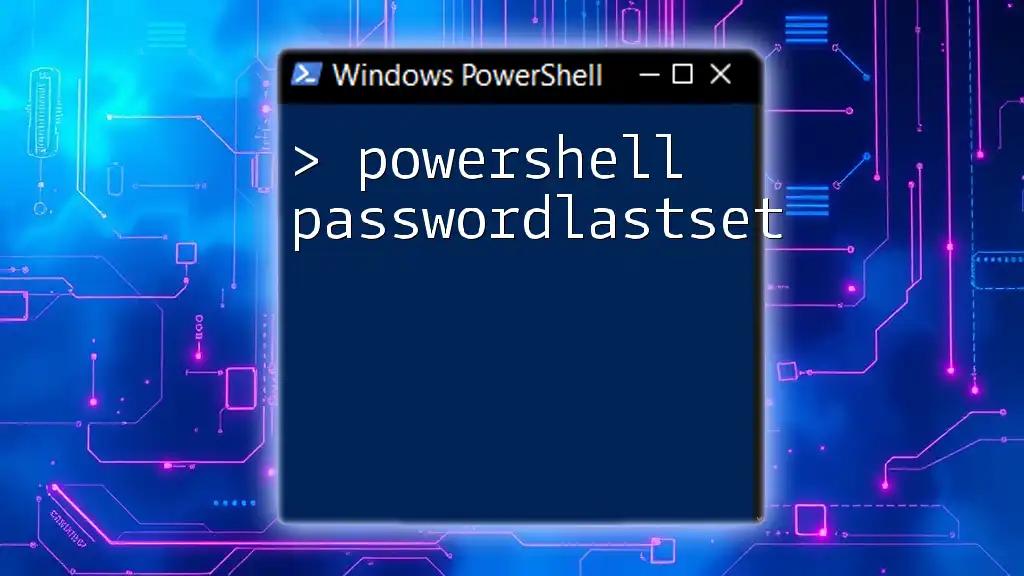
Examples and Use Cases
Example 1: Basic String Array Processing
Consider a scenario where you want to print all elements in a string array. You can create a function for this purpose:
function PrintStringArray {
param([string[]]$stringArray)
foreach ($string in $stringArray) {
Write-Host "Item: $string"
}
}
$fruits = @('Apple', 'Banana', 'Cherry')
PrintStringArray -stringArray $fruits
When invoked, this will output:
Item: Apple
Item: Banana
Item: Cherry
Example 2: Numeric Array Calculation
You might also want to perform calculations on an array, such as summing all numeric values. Here’s how you can do that:
function SumArray {
param([int[]]$numberArray)
$sum = 0
foreach ($number in $numberArray) {
$sum += $number
}
return $sum
}
$numbers = @(1, 2, 3, 4, 5)
$total = SumArray -numberArray $numbers
Write-Host "Total Sum: $total"
This code will output:
Total Sum: 15
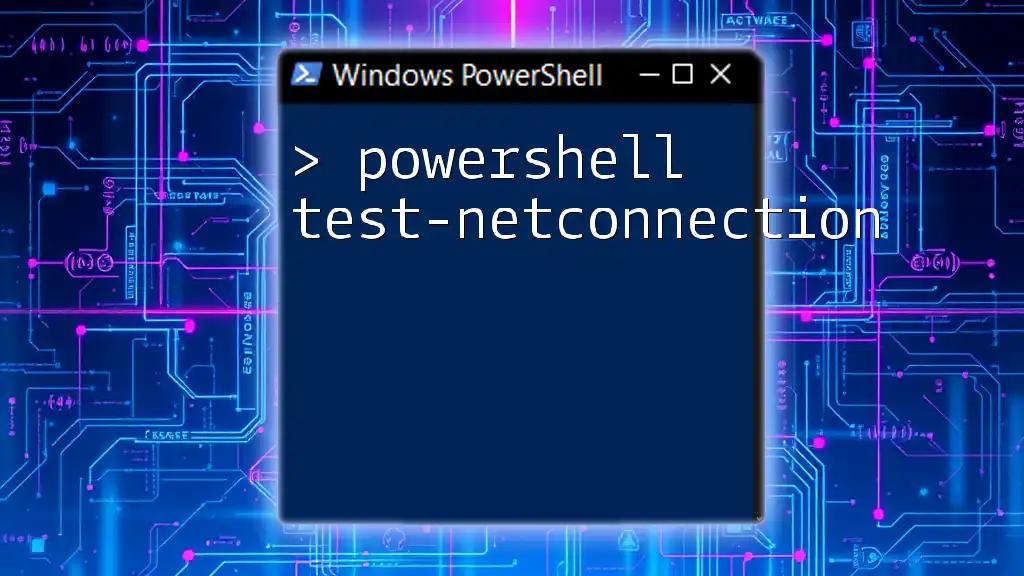
Conclusion
In summary, understanding how to pass arrays to functions in PowerShell unlocks a significant level of efficiency and capability in your scripting. By mastering both arrays and functions, you can automate tasks, improve your code's organization, and enhance its reusability. The examples and techniques discussed in this article provide a strong foundation for any PowerShell user looking to elevate their scripting skills.

Call to Action
We encourage you to explore more advanced resources on PowerShell and share your experiences or questions in the comments below. Your engagement helps build a community of learning and support for all PowerShell enthusiasts!