In PowerShell, you can copy an item and overwrite an existing item at the destination using the `Copy-Item` cmdlet with the `-Force` parameter.
Here’s the code snippet:
Copy-Item -Path "C:\Source\file.txt" -Destination "C:\Destination\file.txt" -Force
Understanding `Copy-Item`
What is `Copy-Item`?
The `Copy-Item` cmdlet is a foundational component of PowerShell, designed to aid users in managing files and directories with ease. This cmdlet allows you to duplicate files and folders from one location to another within your file system, providing the flexibility to organize and backup data effectively.
Syntax of `Copy-Item`
The basic syntax of the `Copy-Item` cmdlet includes several parameters, allowing for customization of the operation:
Copy-Item -Path <String> -Destination <String> [-Force] [-Recurse] [-WhatIf] [-Confirm]
Key parameters to note include:
- `-Path`: Specifies the path of the file(s) or folder(s) you want to copy.
- `-Destination`: Specifies the location where you want the item to be copied.
- `-Force`: Allows the cmdlet to overwrite files in the destination if they already exist without prompting for confirmation.
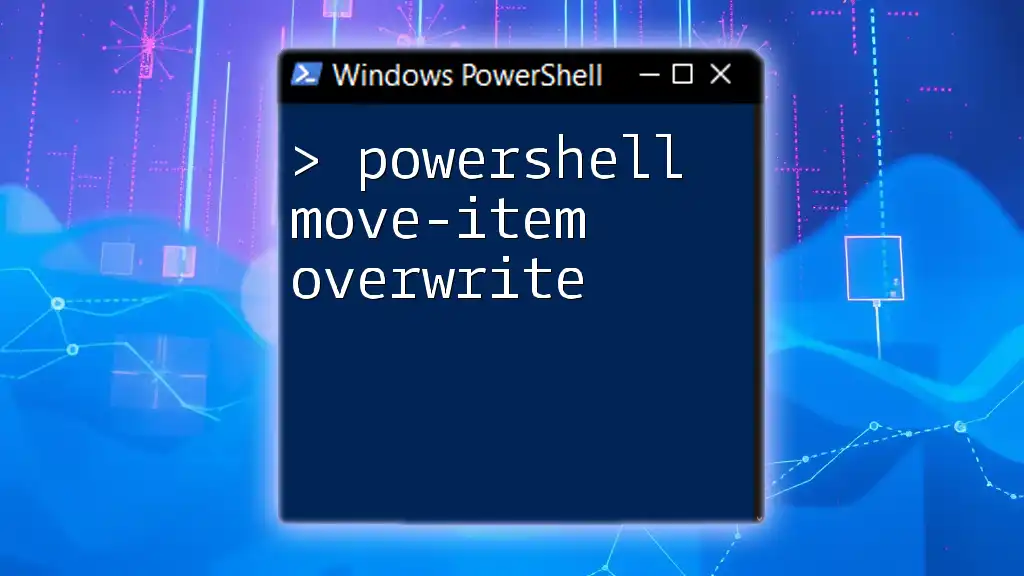
The Concept of Overwriting Files in PowerShell
What Does Overwriting Mean?
Overwriting refers to the process of replacing an existing file or folder with another that has the same name. This is a common necessity in file management, especially when updated versions of files must be stored in the same location.
Importance of Overwrite in Scripts
In scripting scenarios, overwriting files can improve automation workflows. For example, if you regularly export reports, using `Copy-Item` to overwrite older versions means you can ensure the newest data is always readily accessible without leaving a clutter of outdated files. However, caution is crucial—overwriting files without knowing their contents can lead to data loss.
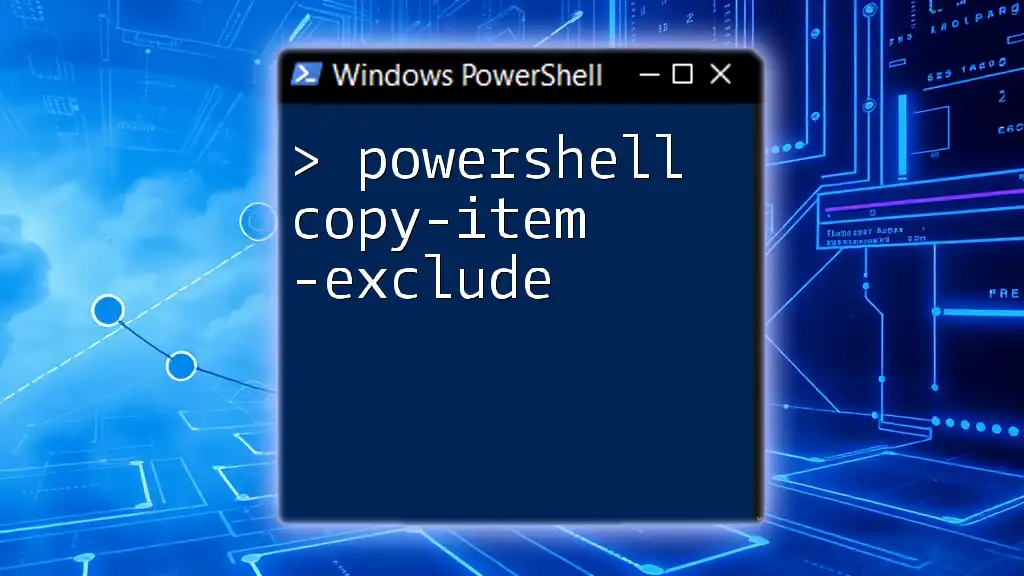
Utilizing `Copy-Item` for Overwriting
Using `-Force` Parameter
The `-Force` parameter is central to the process of overwriting files or folders using `Copy-Item`. When this parameter is included, it tells PowerShell to copy the item even if it means replacing a file that already exists at the destination. This saves time and eliminates the need for manual confirmation or intervention.
Example Usage of `Copy-Item` with `-Force`
Here’s a straightforward example demonstrating how to overwrite a file:
Copy-Item -Path "C:\Source\File.txt" -Destination "C:\Destination\File.txt" -Force
This command will copy `File.txt` from the source directory to the destination, replacing any existing file with the same name without prompting for confirmation.
Copying Files with Overwrite
Simple Copy-Item Example
To illustrate how straightforward the command can be, consider this minimalistic example. You can easily replace a file as shown here:
Copy-Item -Path "C:\Source\Report.docx" -Destination "C:\Backup\Report.docx" -Force
This command will ensure that `Report.docx` in the `Backup` folder is the most recent version, irrespective of whether an older version already exists.
Copying Directories and Overwriting
In scenarios where you need to maintain entire structures, such as copying directories, the `-Recurse` switch comes into play. This allows you to copy the entire contents of a folder while ensuring that existing files are overwritten as necessary. Here’s how it looks:
Copy-Item -Path "C:\Source\Folder" -Destination "C:\Destination\Folder" -Recurse -Force
This command not only duplicates the folder but also ensures that every file within is updated with the new data.
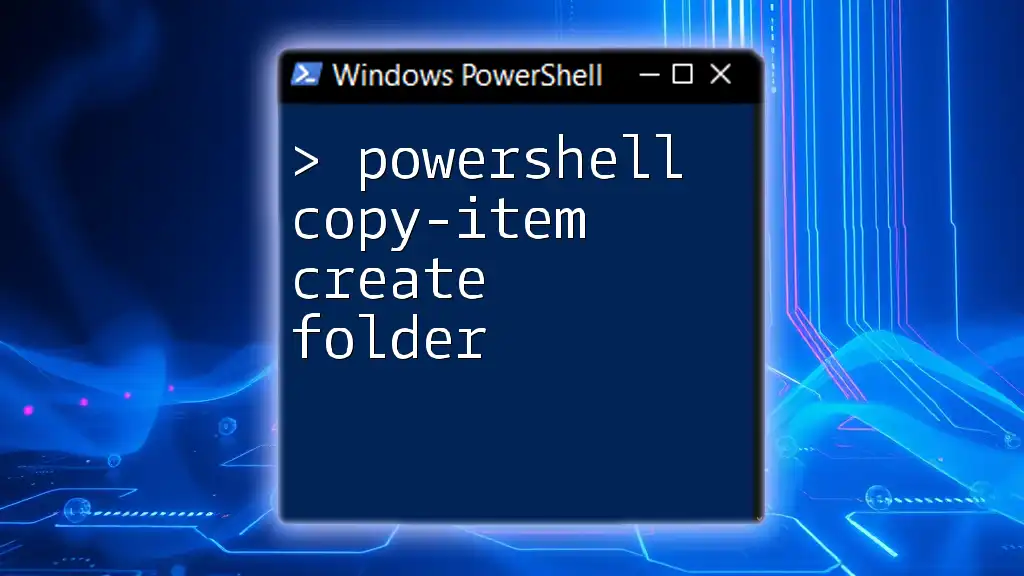
Handling Errors and Warnings
Common Errors Related to File Overwrite
While using `Copy-Item`, users may encounter various errors during the overwrite process. Common issues include:
- File in Use: If the file is currently open or locked by another application, PowerShell cannot overwrite it.
- Permission Issues: Insufficient permissions can prevent overwriting, especially in system directories or for files owned by other users.
It's advisable to check these conditions before performing an overwrite to prevent unexpected disruptions.
Preventing Accidental Overwrites
To add an extra layer of security against accidental loss of data, use the `-Confirm` parameter. This prompts users for confirmation before proceeding with the overwrite. Here’s a basic implementational example:
Copy-Item -Path "C:\Source\File.txt" -Destination "C:\Destination\File.txt" -Confirm
This way, if you issue the command by mistake, you'll have the opportunity to cancel it before any changes are made.
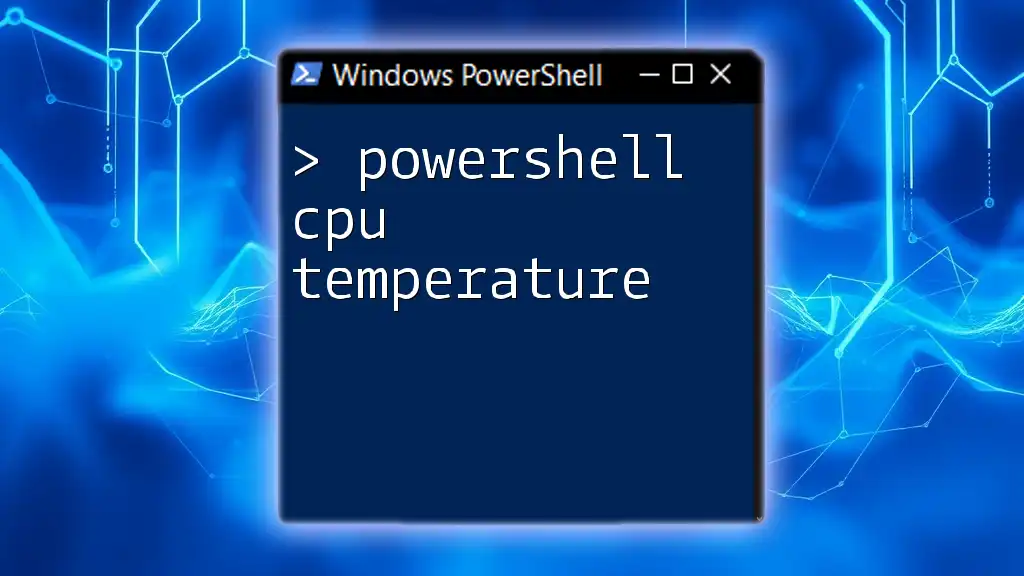
Best Practices for Using `Copy-Item` with Overwrite
Planning Your Overwrite Strategy
Before overwriting files, it's crucial to have a strategy in place to safeguard against unintentional data loss. This includes keeping backups of important files, especially if they are irreplaceable. Always consider if a file has recent modifications or critical information that should not be lost.
Testing with Sample Files
A recommended best practice is to test PowerShell commands on sample files first. This ensures you understand the impact of the `Copy-Item` cmdlet without risking actual, critical data. To simulate a command without executing, you can employ the `-WhatIf` parameter like this:
Copy-Item -Path "C:\Source\File.txt" -Destination "C:\Destination\File.txt" -Force -WhatIf
This displays what the command would do without making any actual changes, letting you verify your intention beforehand.
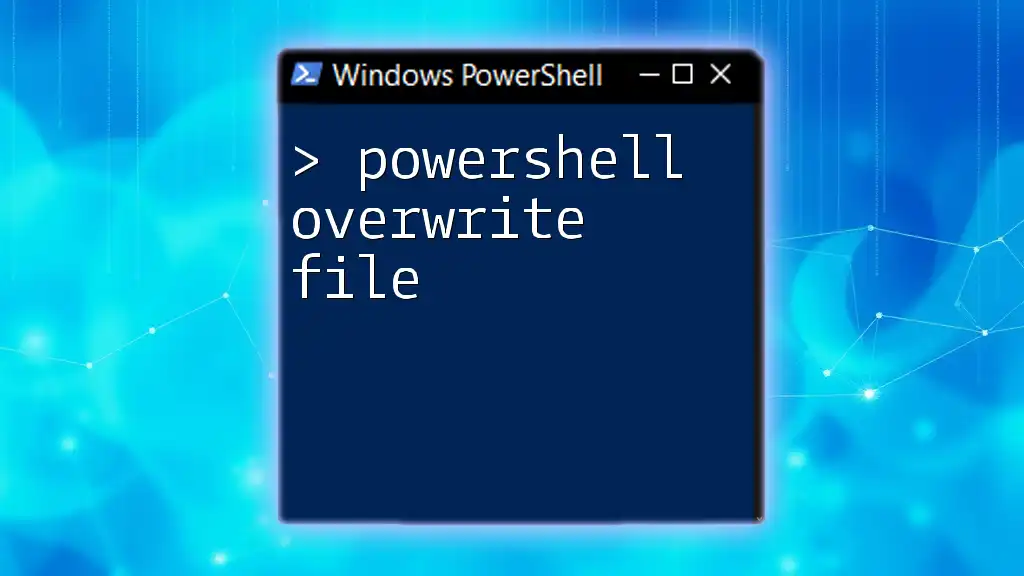
Conclusion
Mastering the `Copy-Item` cmdlet with an emphasis on overwriting is essential for effective file management in PowerShell. By understanding how to use the `-Force` parameter, practicing best strategies, and employing built-in safeguards, users can reduce the risk of data loss while enhancing their workflow efficiencies.
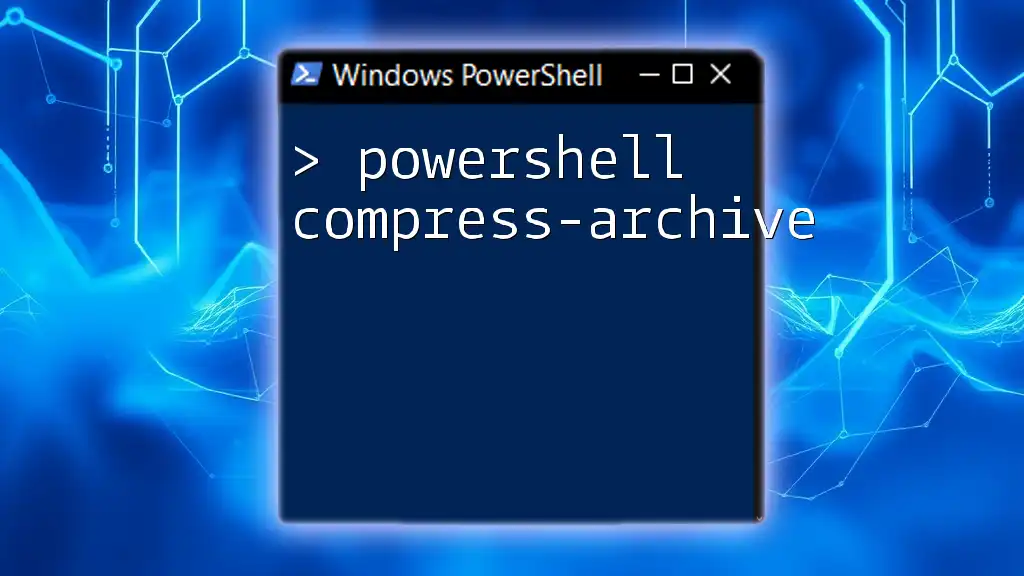
FAQs
What happens if a file doesn't exist when using `Copy-Item`?
If the specified file does not exist at the source when using the `Copy-Item` cmdlet, PowerShell will return an error, indicating that it could not find the specified file.
Can I overwrite files with different file types using `Copy-Item`?
Yes, the `Copy-Item` cmdlet is versatile and can be used to overwrite files of different types as long as the command parameters are correctly specified.
How do I check if a file already exists before overwriting it?
To check if a file exists prior to overwriting, you can use the `Test-Path` cmdlet:
if (Test-Path "C:\Destination\File.txt") {
Write-Host "File exists, careful with overwriting."
} else {
Copy-Item -Path "C:\Source\File.txt" -Destination "C:\Destination\File.txt"
}
This snippet will notify you if the target file exists, allowing you to make informed decisions before proceeding with an overwrite.