To disable a computer account in PowerShell, you can use the `Disable-ADAccount` cmdlet along with the appropriate identity parameter. Here's a code snippet to illustrate:
Disable-ADAccount -Identity "COMPUTER_NAME"
Simply replace `"COMPUTER_NAME"` with the name of the computer account you wish to disable.
What is a Computer Account?
A computer account in Active Directory (AD) serves as a digital identity for the computers within a network. This account allows the computer to connect to the domain, authenticate its identity, and receive security policies. Each computer registered in AD has its account that facilitates communication with other domain services and resources, enabling features such as centralized management, policy enforcement, and secure access.
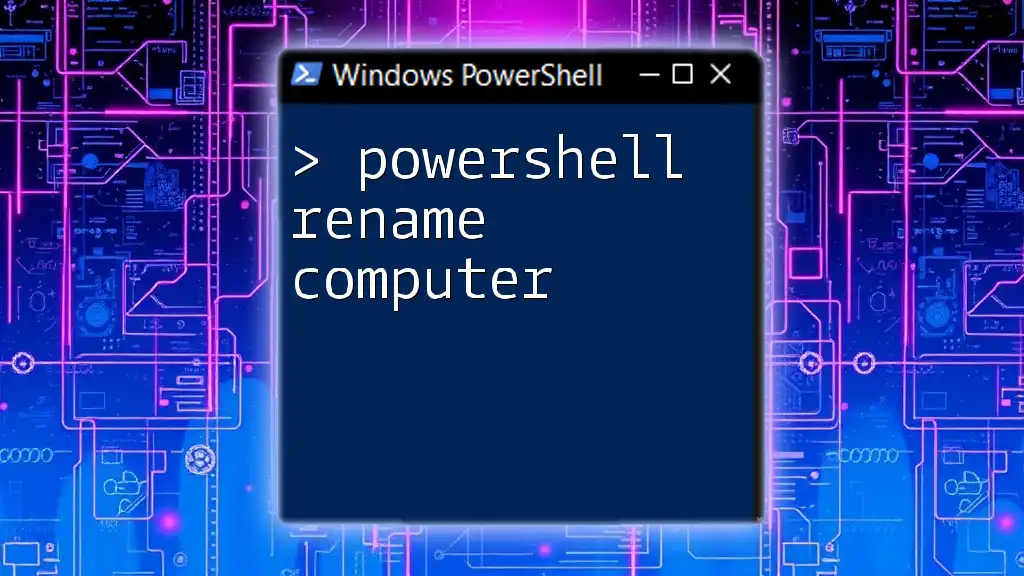
Why Disable a Computer Account?
Disabling a computer account is essential for several reasons:
Security Reasons
- Preventing Unauthorized Access: Disabling accounts for devices that are no longer in use or that may be accessed by former employees helps mitigate security risks.
- Managing Departed Employees and Decommissioned Devices: When a user leaves an organization or a device becomes obsolete, it is crucial to disable their associated accounts to prevent potential misuse.
Operational Reasons
- Maintaining a Tidy Active Directory: Regularly disabling unused accounts helps keep Active Directory organized, improving overall system performance.
- Troubleshooting and Resolving Conflicts: Sometimes a disabled account can resolve conflicts or login issues related to domain resources.
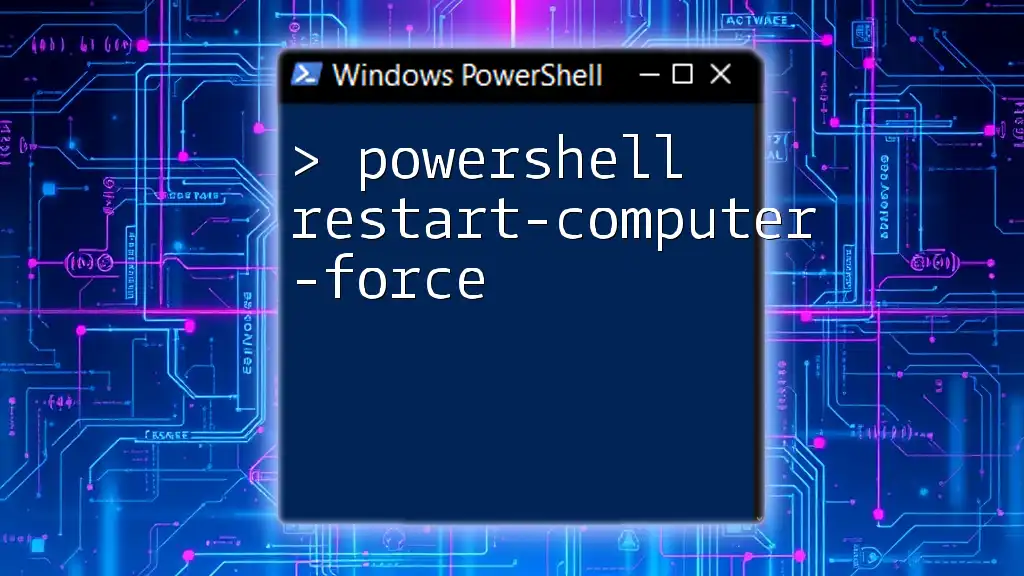
Prerequisites for Disabling a Computer Account
Before you proceed with PowerShell disable computer account tasks, ensure that you have:
-
Administrator Privileges: You must have permissions to manage Active Directory accounts. Typically, you need to be a member of the Domain Admins group or have equivalent rights.
-
PowerShell Environment Setup: Make sure you have the Active Directory module installed. You can load the module by running:
Import-Module ActiveDirectory
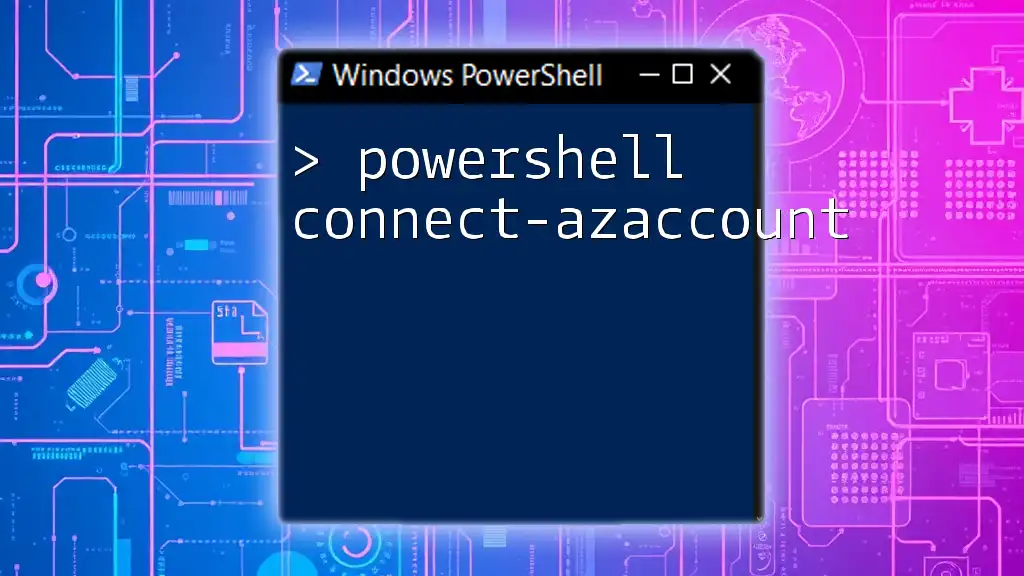
How to Disable a Computer Account Using PowerShell
Basic Command Syntax
The primary cmdlet used for disabling a computer account in Active Directory is `Disable-ADAccount`. The basic syntax is as follows:
Disable-ADAccount -Identity "COMPUTER_NAME"
Replace `"COMPUTER_NAME"` with the actual name of the computer account you wish to disable.
Identifying the Computer Account to Disable
Before you can disable a computer account, you need to ensure you have the correct account identified. You can utilize the `Get-ADComputer` cmdlet for this:
- Finding a Computer Account: To search for a specific computer account, use the command below. This is helpful in case you need to confirm the name or details of the account.
Get-ADComputer -Filter * | Where-Object { $_.Name -eq "COMPUTER_NAME" }
Disabling the Computer Account
Disabling the account can be completed in a few straightforward steps:
-
Open PowerShell as Administrator: Ensure you have the proper administrative rights.
-
Execute the disable command:
Disable-ADAccount -Identity "COMPUTER_NAME"
-
Verify the account has been disabled: It’s vital to confirm that the action was successful and the account is indeed disabled.
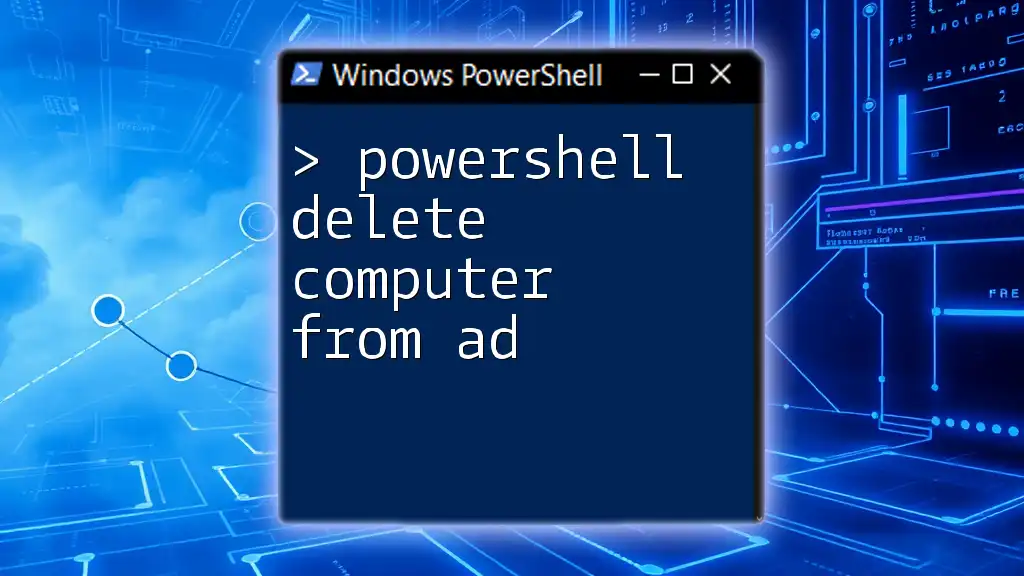
Confirming the Status of the Computer Account
Using PowerShell to Check Account Status
To check if the account has been successfully disabled, you can use the following command:
Get-ADComputer -Identity "COMPUTER_NAME" | Select-Object Name, Enabled
This command will give you a quick view of the account’s status, showing whether it is enabled or disabled. The `Enabled` property should reflect `False` for disabled accounts.
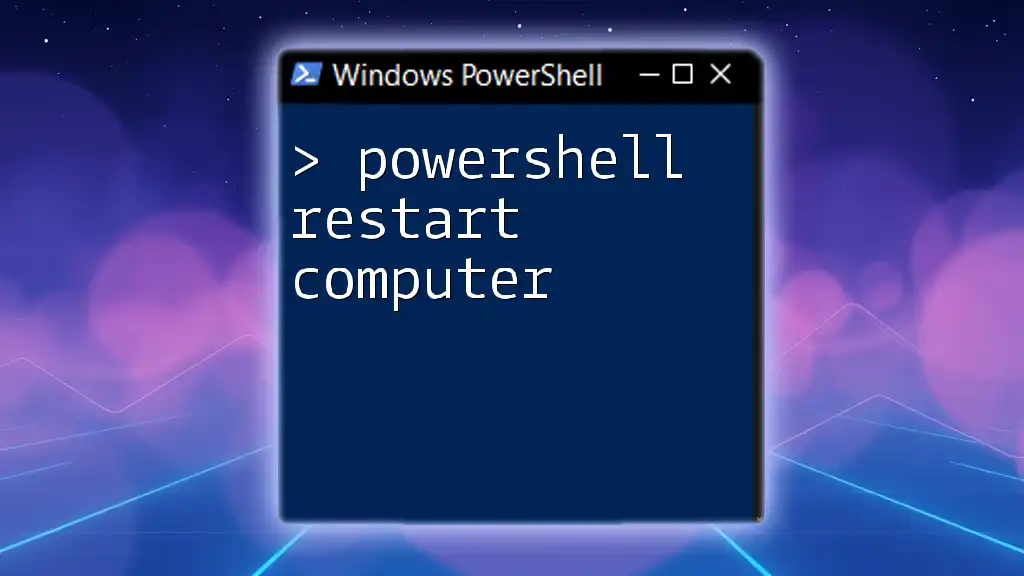
Best Practices for Managing Computer Accounts
Regular Audits
Regularly auditing computer accounts is a proactive strategy that ensures your Active Directory remains organized and secure. You might consider utilizing PowerShell scripts to automate this process, perhaps running periodic checks to identify accounts that haven’t been used in a while.
Documenting Changes
Maintaining records of disabled accounts is important. This can be achieved by logging changes in a simple text file or database, ensuring you have a historical record of all account management actions. For example, you might implement a logging mechanism like this:
$account = "COMPUTER_NAME"
Disable-ADAccount -Identity $account
Add-Content "C:\Logs\DisabledAccounts.log" "$(Get-Date) - $account has been disabled."
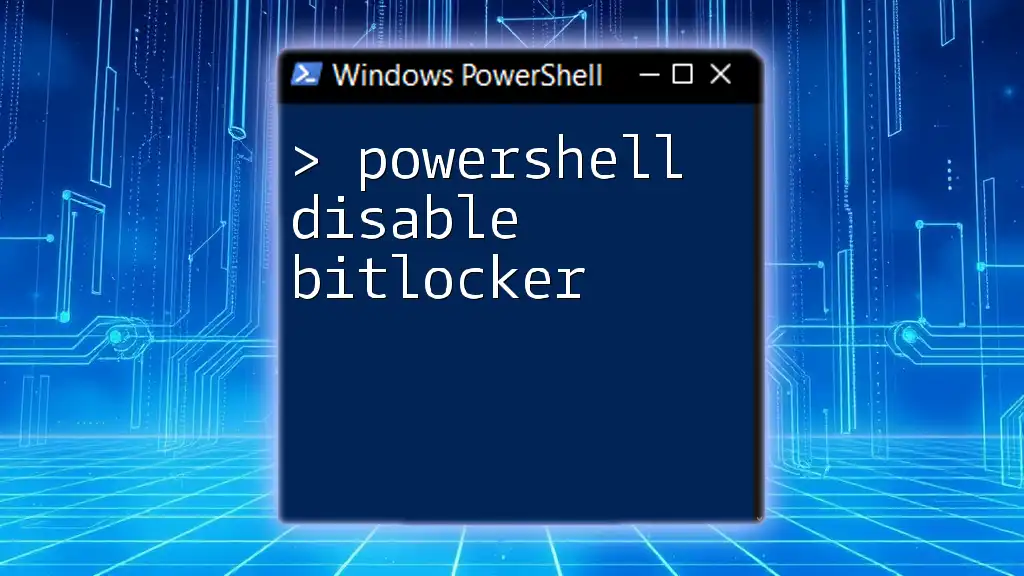
Troubleshooting Common Issues
Account Doesn't Disable
If you encounter issues where the account does not disable, consider the following potential causes:
- Lack of Permissions: Ensure that your user account has the necessary permissions.
- Incorrect Account Name: Double-check that the computer name you are using is accurate.
The Account Still Appears Enabled
If after running the disable command, the account still appears enabled, it could be due to several reasons:
- Refresh the PowerShell Session: Sometimes changes may not reflect immediately due to caching. You might need to restart your PowerShell session or refresh your AD console.
- Utilize GUI Tools: If the command line does not reflect changes, verify using Active Directory Users and Computers (ADUC) for any discrepancies in GUI-based views.
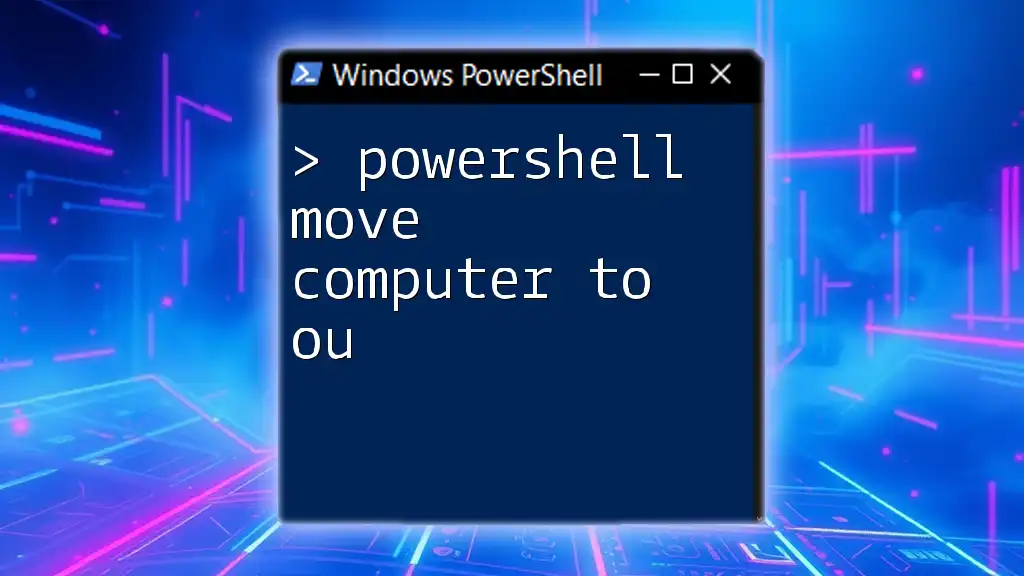
Conclusion
Managing computer accounts actively within Active Directory is a critical aspect of maintaining system security and operational efficiency. The `PowerShell disable computer account` command is a powerful tool in any system administrator's toolkit, enabling them to control access effectively and keep their networks secure. Regular audits, documentation of changes, and troubleshooting methods will ensure successful administration throughout your organization.
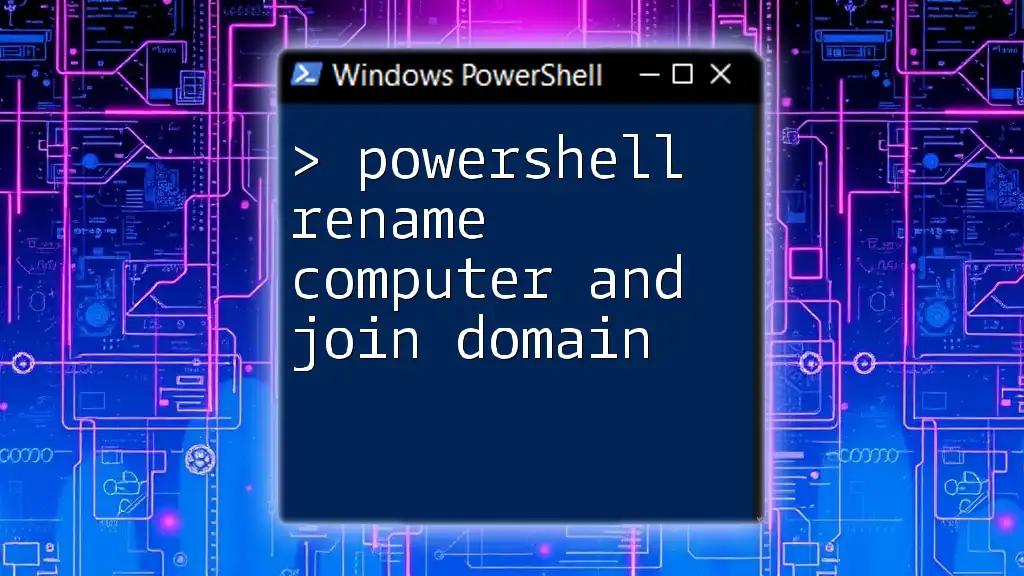
Additional Resources
For further reading, explore the following links:
- Microsoft Official Documentation on PowerShell and Active Directory.
- Additional PowerShell tutorials for beginners and experts alike.
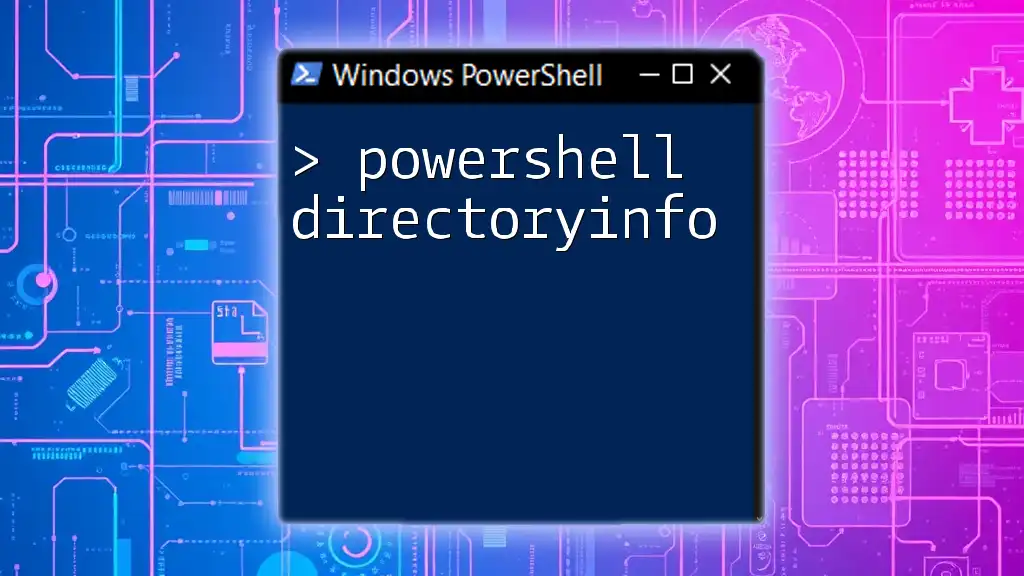
Call to Action
Begin applying what you've learned today by practicing the `PowerShell disable computer account` command. For deeper insights and tailored training, consider signing up for our specialized workshops and share your experiences or queries about PowerShell in the comments below!