The `Get-PSDrive` command in PowerShell allows you to retrieve information about the drives available on a remote computer by utilizing the `-ComputerName` parameter.
Here’s a simple code snippet demonstrating this command:
Get-PSDrive -ComputerName 'RemoteComputerName'
Understanding PSDrive
What is PSDrive?
PSDrive is a PowerShell provider that enables users to access various storage locations such as the file system, registry, and certificates right within the PowerShell environment. Think of PSDrives as virtual drives that map to data stores, allowing you to interact with them similarly to how you would with physical drives on your machine.
PSDrive can represent various data types, such as:
- FileSystem: Represents file system drives.
- Env: Represents environment variables.
- Cert: Represents the certificate store.
Understanding PSDrive is crucial for efficient remote administration in PowerShell, particularly when using commands that require drive and directory access.
Why Use PSDrive?
Using PSDrive provides multiple advantages, especially for remote computing. It simplifies the management of files, folders, and system resources without the need for separate tools. Here are some reasons to consider:
- Unified Access: Access disparate data stores under a single interface.
- Ease of Use: Utilize familiar cmdlets and commands for resource management.
- Remote Availability: Manage remote resources as easily as local ones.
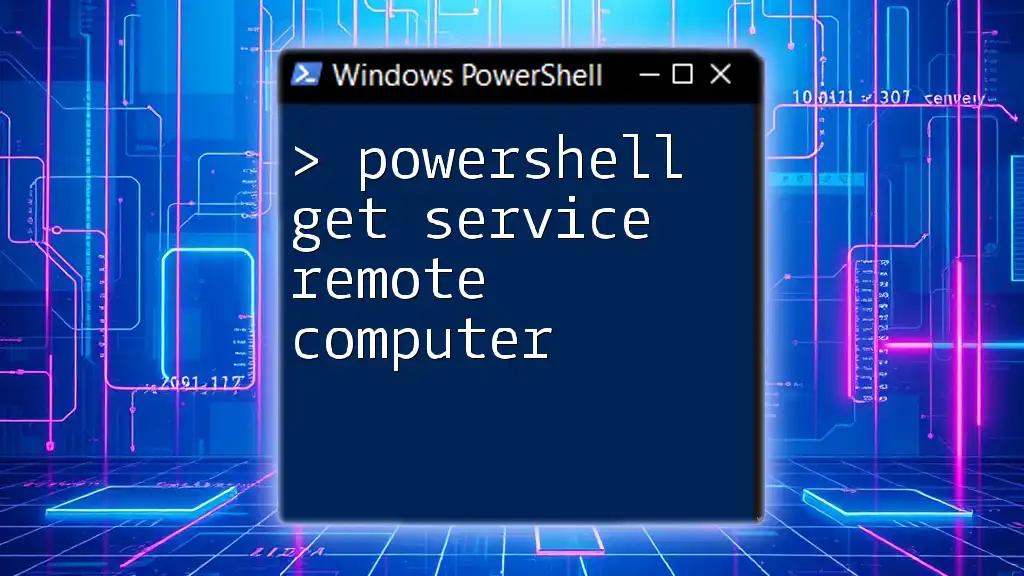
The `Get-PSDrive` Cmdlet
Overview of `Get-PSDrive`
`Get-PSDrive` is a cmdlet that allows users to retrieve information about all the PSDrives present in their PowerShell session. It provides insights on the drive type, used space, available space, and the root of each drive.
The general syntax of the `Get-PSDrive` cmdlet is as follows:
Get-PSDrive [-Name <String>] [-PSProvider <String>] [-Scope <String>] [<CommonParameters>]
Basic Usage of `Get-PSDrive`
To list all available PSDrives in your current session, simply run:
Get-PSDrive
This command will produce an output displaying all drives along with information such as:
- Name: The name of the drive.
- Used: Space currently being utilized.
- Free: Available space.
- Provider: The type of data store (e.g., FileSystem).
The output provides a clear snapshot of your current storage environment, invaluable when managing local or remote resources.
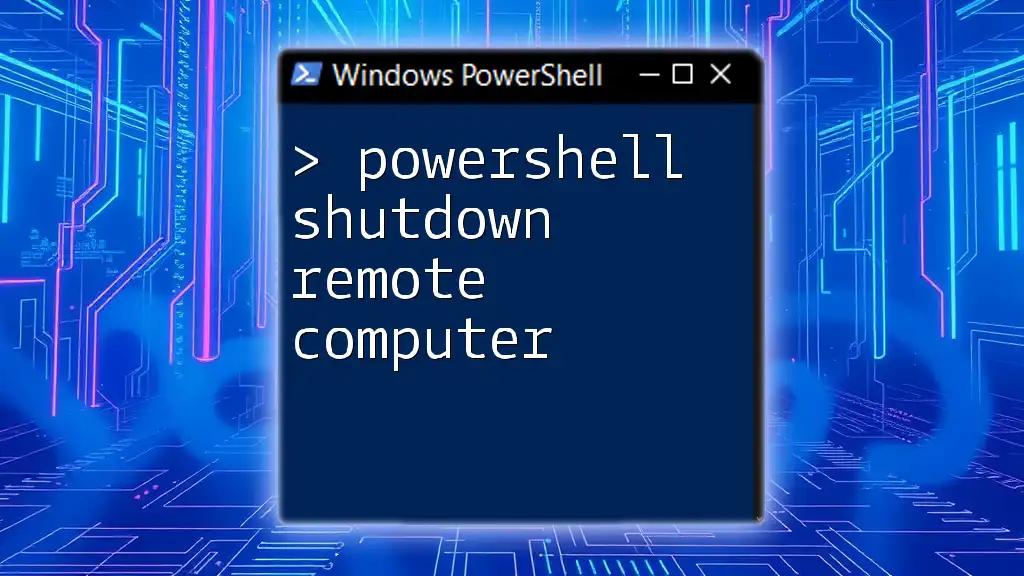
Accessing Remote Drives
Prerequisites for Accessing Remote Drives
Before accessing drives on a remote computer, ensure the following:
- Permissions: You must have administrative permissions on the remote computer.
- Network Availability: The remote computer should be accessible over the network.
- Remote Management: Windows Remote Management (WinRM) must be enabled on the remote system.
Using `Get-PSDrive` on a Remote Computer
Establishing a Remote Session
To remotely access resources, you'll need to create a remote session using the `Enter-PSSession` command. Here’s how:
Enter-PSSession -ComputerName RemoteComputerName
Replace `RemoteComputerName` with the actual name or the IP address of the target system. This command allows you to interact with the remote environment as if it were local.
Executing `Get-PSDrive` Remotely
Once connected, you can use `Get-PSDrive` to access information about PSDrives on the remote computer:
Invoke-Command -ComputerName RemoteComputerName -ScriptBlock { Get-PSDrive }
This command runs `Get-PSDrive` in the context of the specified remote computer, returning details about its drives. Utilizing `Invoke-Command` is crucial here, as it enables remote execution of PowerShell commands.
Interpreting the Results
When executing the command, you'll get an output similar to what you observe locally. Understanding this information will help you manage remote resources effectively and make informed administrative decisions.
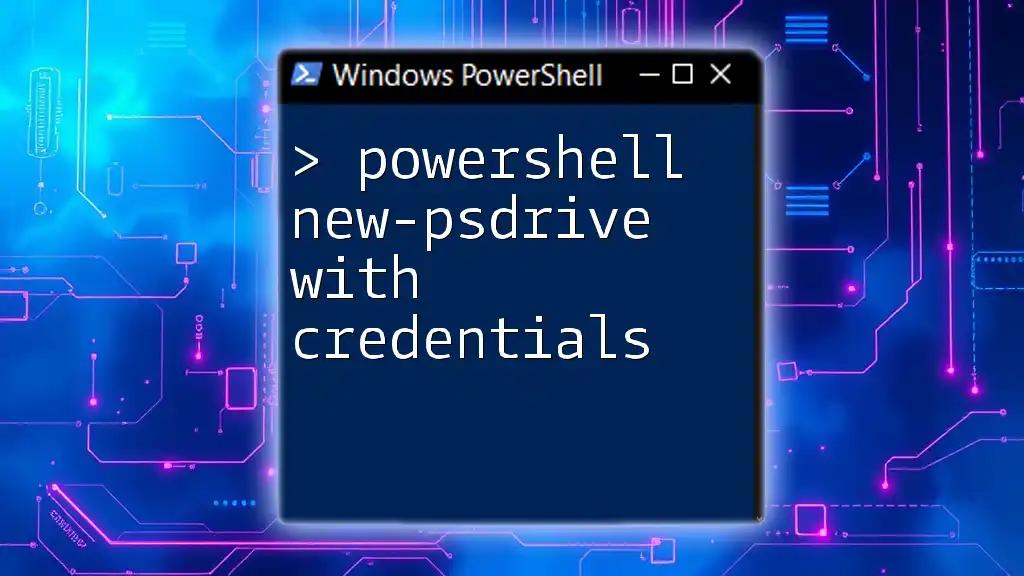
Common Use Cases for `Get-PSDrive` on Remote Computers
Monitoring Drive Space
One common scenario involves monitoring drive space to ensure optimal operation. You can check available space on remote drives by combining `Get-PSDrive` with filtering commands:
Invoke-Command -ComputerName RemoteComputerName -ScriptBlock { Get-PSDrive | Where-Object { $_.Used -gt 100MB } }
This command filters the output to show only drives that have used more than 100MB of space, ensuring you can quickly identify potential storage issues and act on them.
Managing Remote Drives
Using `Get-PSDrive` paves the way for effective management of remote drives. For instance, if you need to map a remote drive, you may use the following code:
New-PSDrive -Name Z -PSProvider FileSystem -Root \\RemoteComputerName\Share -Persist
This creates a new PSDrive that maps to a shared folder on the remote system, making file management seamless and straightforward.
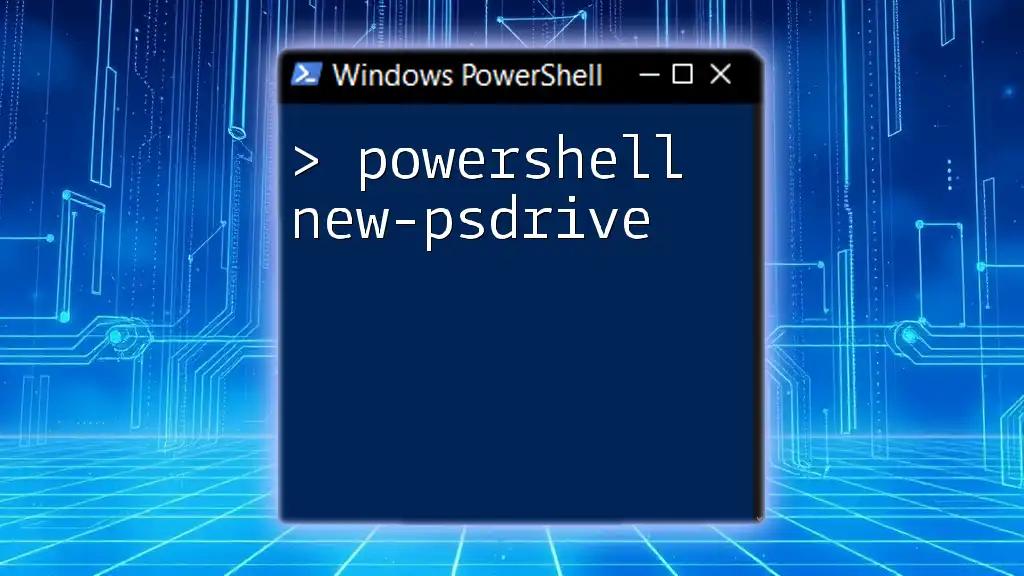
Error Handling
Common Errors with `Get-PSDrive`
When executing `Get-PSDrive` or any related commands remotely, you may encounter common errors such as:
- Access Denied: Notifications indicating lack of permissions.
- Network Path Not Found: Issues arising from incorrect network or computer names.
Understanding these error messages and their sources can help in troubleshooting effectively.
Troubleshooting Connectivity Issues
If you face connectivity problems while trying to access remote drives, consider the following troubleshooting steps:
- Firewall Settings: Ensure that the firewall on the remote computer allows PowerShell remoting.
- Network Configurations: Confirm the remote computer is reachable via ping or similar network tests.
- Service Status: Check if WinRM service is active on the remote machine and that it is properly configured.
With proper checks, most connectivity and permission issues can be quickly diagnosed and resolved.
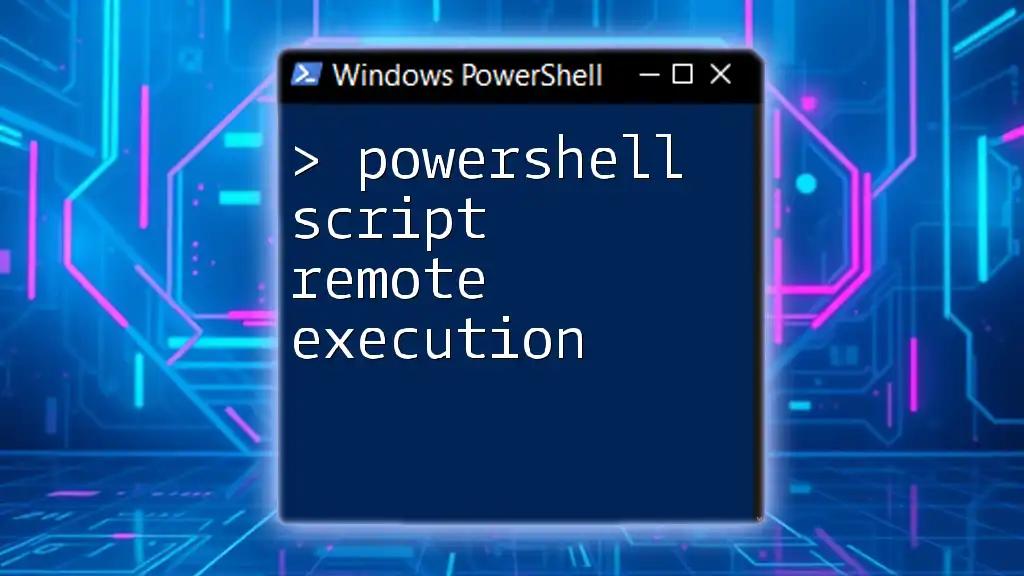
Advanced Techniques
Filtering Results
Efficiently managing results is essential in PowerShell, especially when dealing with multiple remote drives. For example, you can filter results to show only filesystem drives using:
Invoke-Command -ComputerName RemoteComputerName -ScriptBlock { Get-PSDrive -PSProvider FileSystem }
This approach simplifies data management by narrowing down to just the information you need.
Automating Remote Drive Monitoring
To enhance management efficiency, consider writing scripts that automate remote drive monitoring. For example, you can create a scheduled task that runs a PowerShell script to email you drive usage reports.
Building robust scripts ensures you're always aware of your remote environments without requiring constant manual checks.
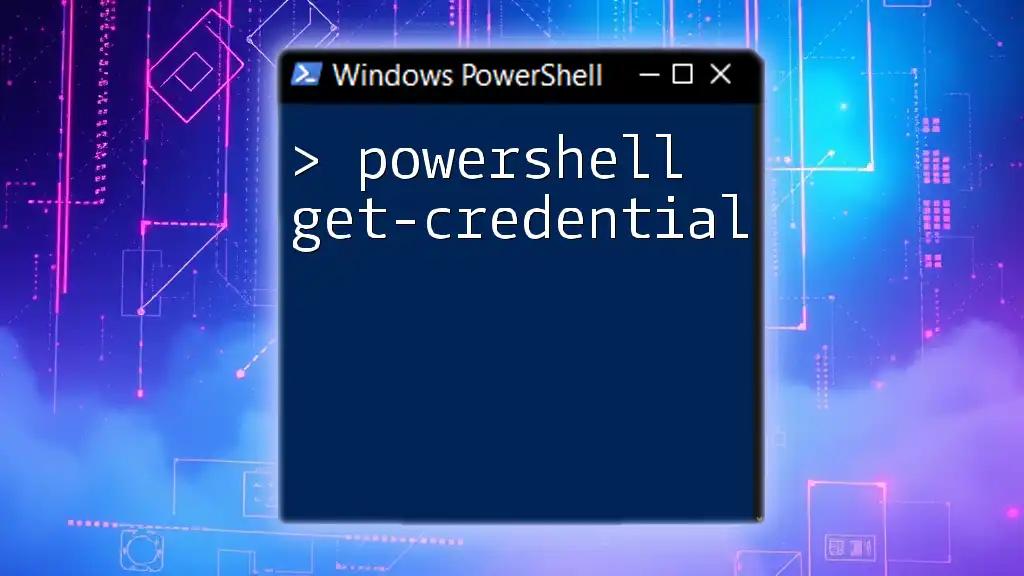
Conclusion
In conclusion, understanding and utilizing the `Get-PSDrive` cmdlet for remote computers greatly enhances your ability to manage storage and resources. Whether monitoring drives or executing administrative tasks, this command is an essential tool in the PowerShell toolkit. For those eager to delve deeper into PowerShell and improve their skills, exploring additional resources and advanced techniques will provide a beneficial path forward.
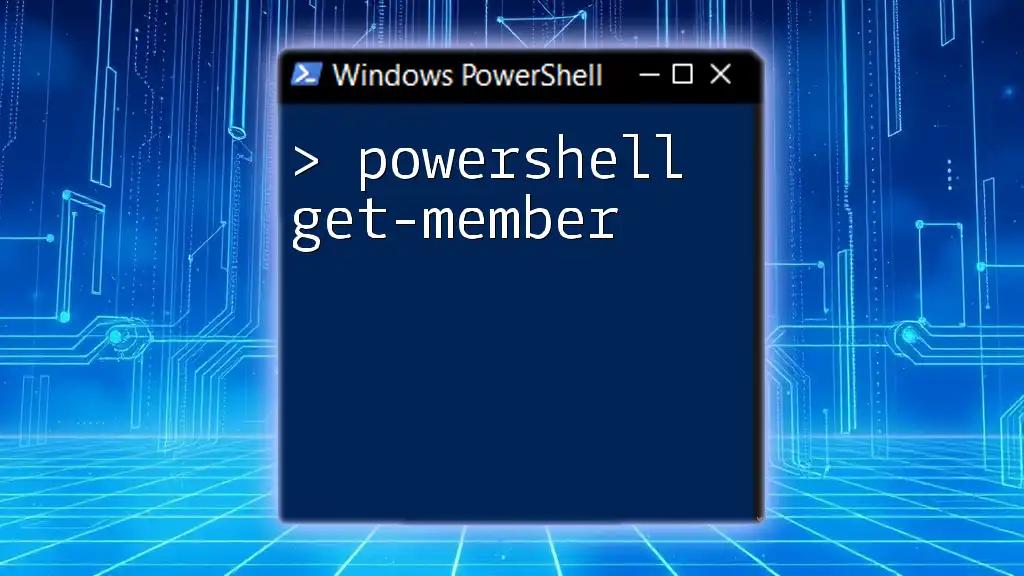
Call to Action
Have you had success with PowerShell in managing remote drives? Share your experiences, ask questions, and connect with others eager to enhance their PowerShell knowledge. Be sure to subscribe for insights on upcoming lessons and courses that dive deeper into the capabilities of PowerShell!