Sure! Here's a concise explanation along with a code snippet for your post:
To copy files to remote computers using PowerShell, you can utilize the `Copy-Item` cmdlet with the appropriate credentials and destination path.
Copy-Item -Path "C:\local\file.txt" -Destination "\\remoteComputer\C$\destination\file.txt" -Credential (Get-Credential)
This command securely transfers `file.txt` from your local machine to the specified path on a remote computer.
Understanding PowerShell Remoting
What is PowerShell Remoting?
PowerShell remoting is a powerful feature that allows administrators to run commands and access the file systems of remote computers through a secure connection. This capability is invaluable for managing multiple systems from a central location, thus saving time and effort in IT operations. It enables seamless interactions with remote machines, vastly improving administrative efficiency.
Enabling PowerShell Remoting
To take full advantage of PowerShell remoting, you must enable it on both the local and remote machines. This is a simple process. Use the following command in an elevated PowerShell session on the remote machine:
Enable-PSRemoting -Force
This command activates Windows PowerShell Remoting, ensuring the appropriate services are running. Ensure firewall rules permit PowerShell remoting to avoid connection issues.
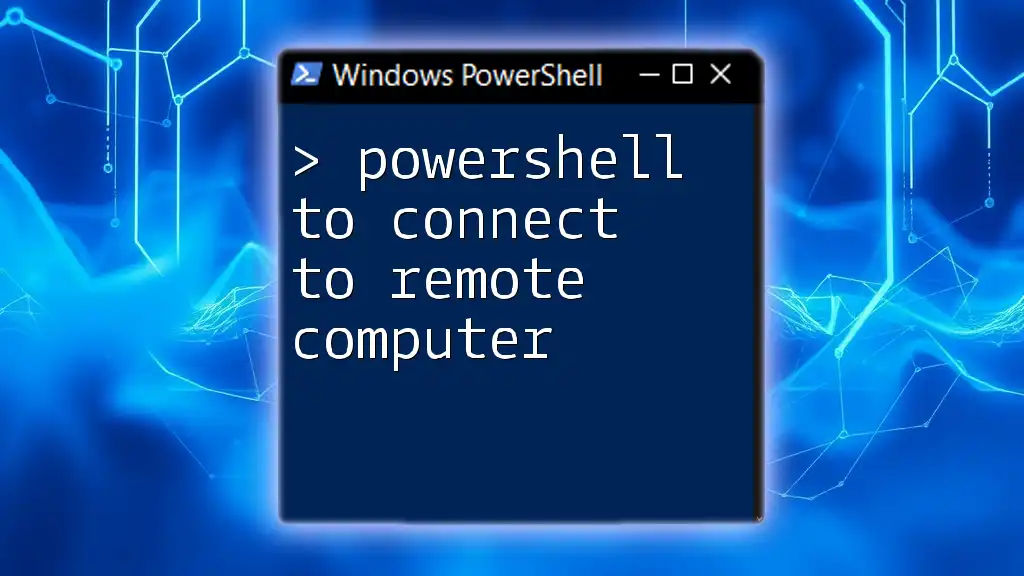
Copying Files to Remote Computers
Using `Copy-Item` for Remote File Transfer
The `Copy-Item` cmdlet is the primary method for transferring files between computers in PowerShell. It allows you to specify source and destination paths, including paths for remote machines. The syntax you will use is straightforward:
Copy-Item -Path "<local file path>" -Destination "<remote path>" -ToSession <session>
Creating a PowerShell Session
Before copying files, you need to establish a session with the remote computer. This involves creating a PowerShell session and authenticating with the necessary credentials. You can do this using the following command:
$session = New-PSSession -ComputerName "RemoteComputerName" -Credential (Get-Credential)
This command prompts for a username and password, which you will use to access the remote machine.
Copying a File to a Remote Machine
Now, with the session established, you can use the `Copy-Item` cmdlet to transfer files to a remote computer. For example, to copy a file named `file.txt` from your local folder to a remote folder, use the following command:
Copy-Item -Path "C:\local\file.txt" -Destination "C:\remote\file.txt" -ToSession $session
This command specifies the local path as the source and the remote path as the destination.
Copying a File to Multiple Remote Machines
If you need to copy a file to several remote computers, it can be performed efficiently using a loop. Here’s how you can automate file transfers to multiple systems:
$computers = "Computer1", "Computer2", "Computer3"
foreach ($computer in $computers) {
$session = New-PSSession -ComputerName $computer -Credential (Get-Credential)
Copy-Item -Path "C:\local\file.txt" -Destination "C$\remote\file.txt" -ToSession $session
Remove-PSSession $session
}
In this snippet, we define an array of computers. The script loops through each computer, establishes a session, and copies the file before removing the session instance, which is a good practice to manage resources effectively.
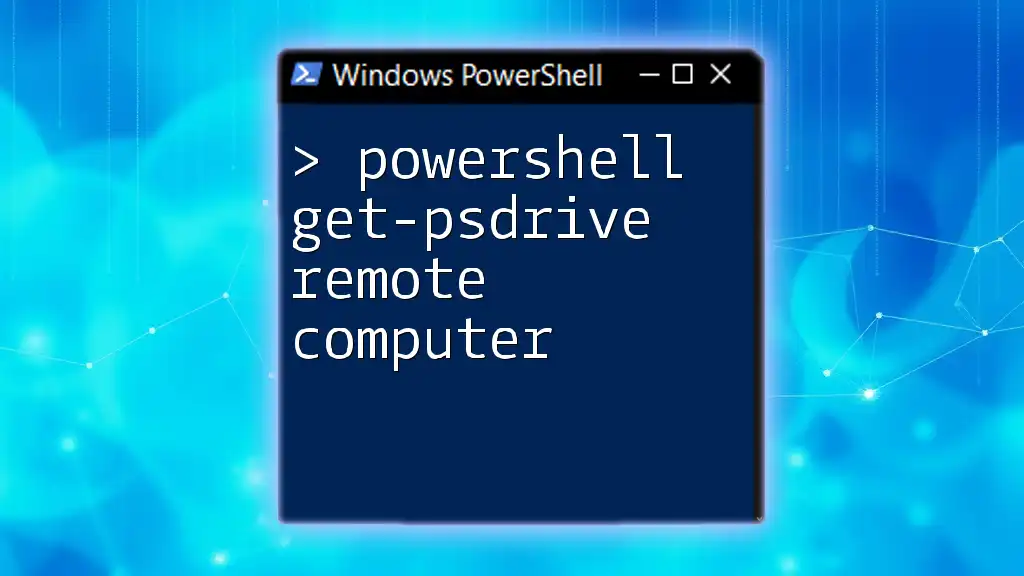
Copying Files from Remote Computers
Using `Copy-Item` to Retrieve Files
Not only can you use PowerShell to copy files to remote machines, but you can also retrieve files from them. This is just as simple and follows a similar syntax as transferring files.
Syntax Overview
To copy files from a remote machine, you will use the command format:
Copy-Item -Path "<remote file path>" -Destination "<local path>" -FromSession <session>
Establishing Connection to the Remote Computer
Again, you will set up a session to the remote computer before executing the copy command. The connection process is identical to when you were transferring files to the remote machine.
Copying a File from a Remote Machine
Once connected, you can easily copy a file from the remote machine to your local system. For instance, if you want to retrieve `file.txt` from a remote folder, you can execute:
Copy-Item -Path "C$\remote\file.txt" -Destination "C:\local\file.txt" -FromSession $session
This command specifies the location of the file on the remote machine and where it should be saved on your local machine.
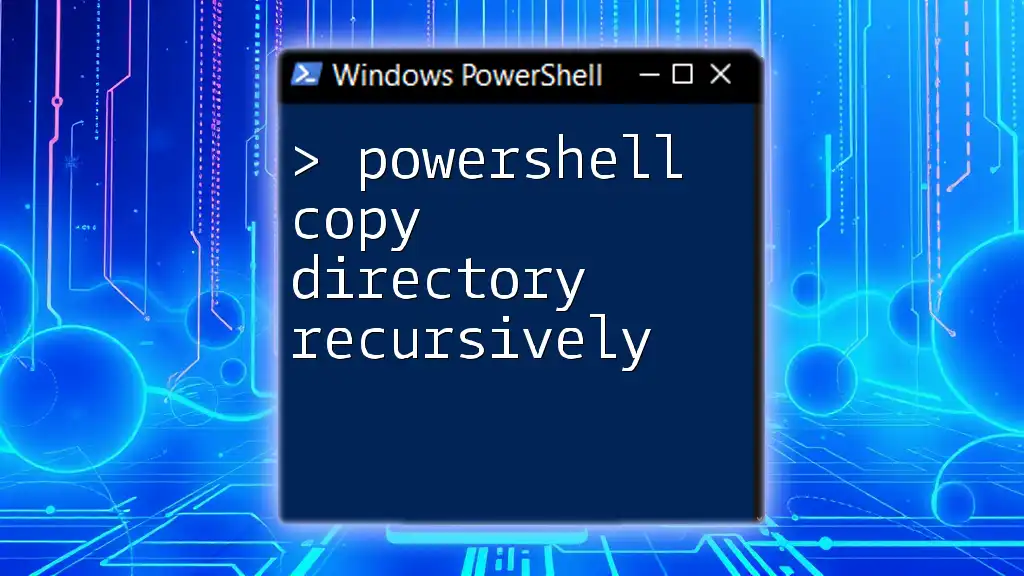
Handling Errors and Troubleshooting
Common Errors When Copying Files
When using PowerShell to copy files, you may encounter various issues. Some common pitfalls include:
- Permission Denied: Ensure you have the necessary permissions to access both the source and destination directories. Using credentials with the appropriate access level is crucial.
- Network Issues: Network connectivity problems can disrupt file transfers. Confirm that both machines are connected and reachable over the network.
Best Practices for File Transfers
To maximize the reliability of your file transfers, consider these best practices:
- Use `Try/Catch` Blocks: Implement these blocks around your copy commands to catch and handle potential errors more gracefully.
try {
Copy-Item -Path "C:\local\file.txt" -Destination "C:\remote\file.txt" -ToSession $session
} catch {
Write-Host "Error: $_"
}
- Validate Transfers: After a transfer completes, you might want to check if the file exists at the destination to validate the success of the operation.
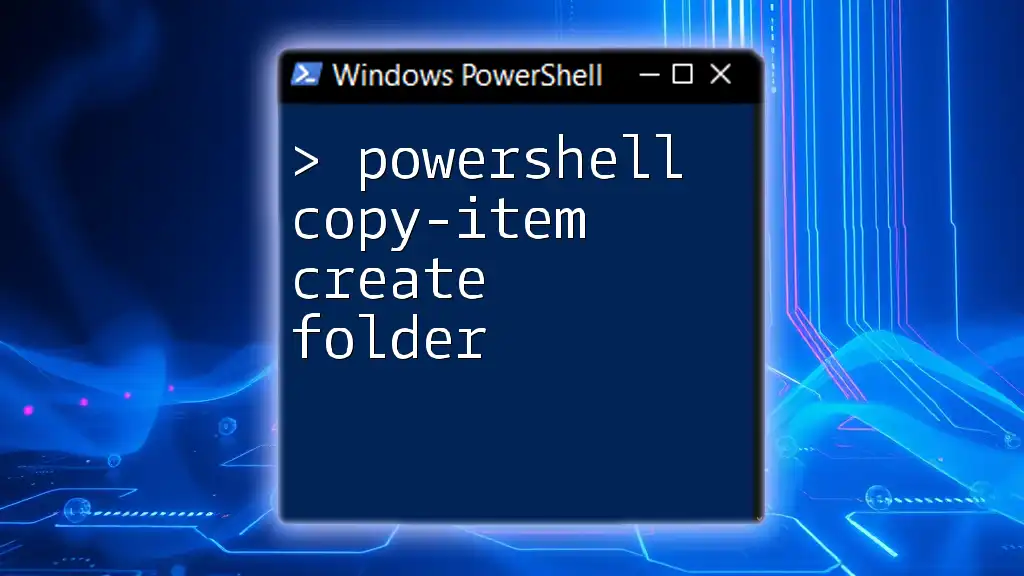
Conclusion
By utilizing PowerShell's powerful remoting features, particularly the `Copy-Item` cmdlet, you can streamline the process of copying files to and from remote computers. This guide has provided you with the essential commands, best practices, and troubleshooting tips so you can effectively manage your file transfers in a swift and secure manner. Remember to practice these commands and explore their potential further to make the most out of PowerShell in your daily IT tasks.
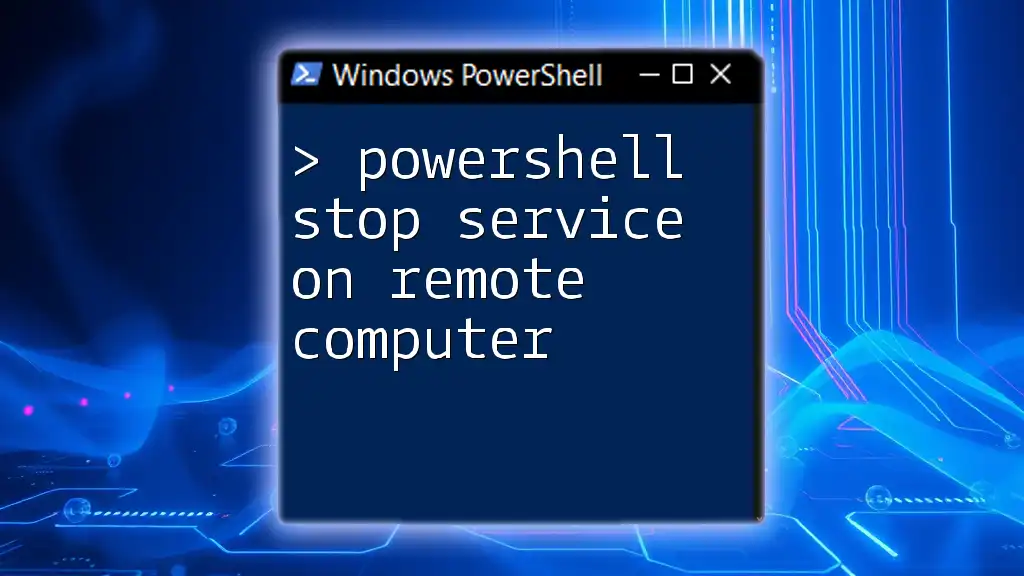
Additional Resources
For deeper learning, consider exploring the official Microsoft PowerShell documentation, which offers extensive resources and community forums for troubleshooting and best practices. Stay current with updates and new features by following PowerShell blogs or participating in online courses. For personalized assistance, feel free to reach out to our company for training and support specific to your needs.