To execute a PowerShell script on a remote computer, you can use the `Invoke-Command` cmdlet along with the script block you want to run.
Here's a code snippet demonstrating this:
Invoke-Command -ComputerName "RemotePC" -ScriptBlock { Write-Host 'Hello from remote!' }
Understanding Remote Execution in PowerShell
What Does It Mean to Run PowerShell Scripts Remotely?
Running PowerShell scripts remotely means executing commands or scripts on a computer that is not physically present at your location. This powerful feature is essential for system administrators managing multiple machines, enabling them to automate tasks efficiently across a network.
Benefits of Remote Script Execution
Executing scripts remotely offers significant advantages, such as:
- Efficiency: You can manage multiple computers from a single location.
- Automation: Automate everyday tasks without needing physical access to the machine.
- Cost-Effective Management: Less time spent on routine tasks allows for a more focused use of IT resources.
Key Concepts in Remote PowerShell
PowerShell Remoting
PowerShell Remoting is a technology that allows commands to be run on one or multiple remote computers. This capability is built on the WS-Management protocol and utilizes the Common Information Model (CIM). Understanding these concepts is crucial in mastering how to use PowerShell scripts on remote computers.
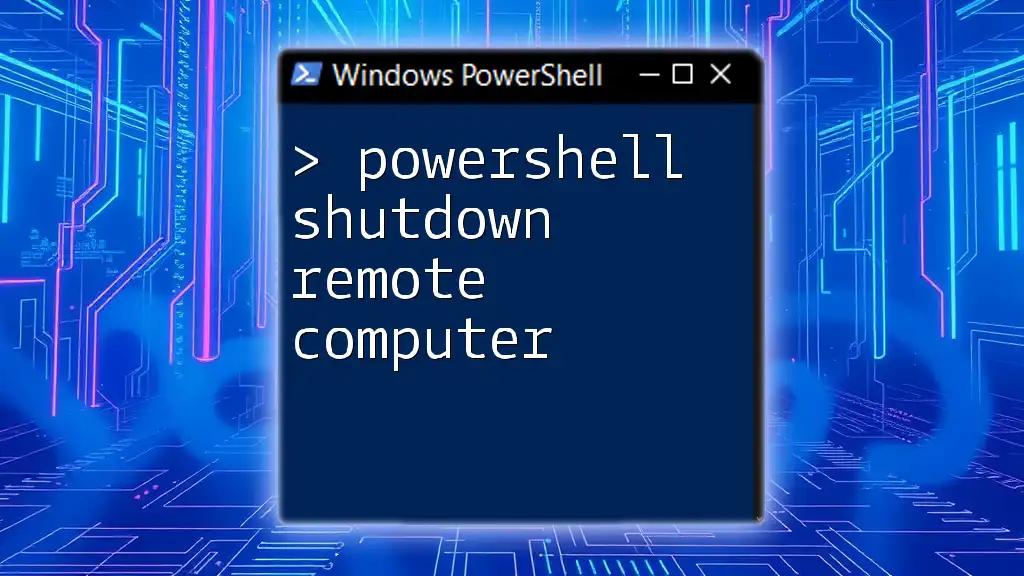
Preparing the Remote Computer
Configuring the Remote Computer for PowerShell Remoting
Before you can run a PowerShell script on a remote computer, you must ensure that PowerShell remoting is enabled on that machine.
Enable PowerShell Remoting Using `Enable-PSRemoting`
You can enable PowerShell remoting with a simple command. Use the following snippet:
Enable-PSRemoting -Force
This command configures the necessary settings and firewall rules to allow remote management, thus making it the first step in your process.
Understanding Firewall Rules and Permissions
The remoting feature may rely on specific firewall settings. Ensure that the Windows Firewall allows incoming traffic for PowerShell remoting, which typically happens when `Enable-PSRemoting` is executed correctly.
Set Up TrustedHosts if Needed
If the remote computer is not part of a domain, set it as a TrustedHost. This allows you to specify which computers are authorized for PowerShell remoting:
Set-Item WSMan:\localhost\Client\TrustedHosts -Value "RemoteComputerName"
This step is crucial to avoid warnings or errors when you attempt to connect to the remote machine.
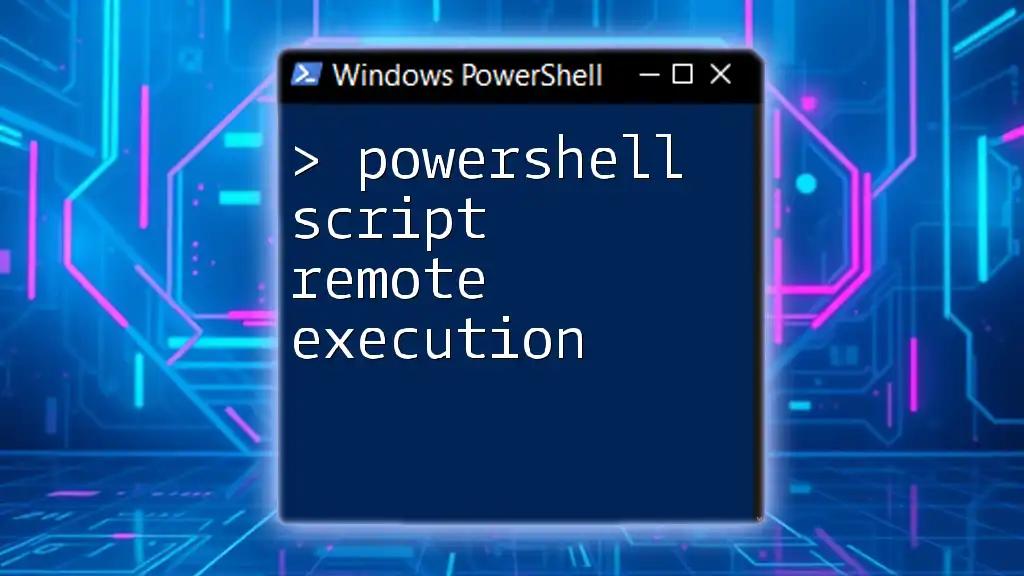
How to Run a PowerShell Script on a Remote Computer
Using Invoke-Command
The most common way to run a PowerShell script on a remote computer is by using the `Invoke-Command` cmdlet.
What is `Invoke-Command`?
`Invoke-Command` allows you to execute commands or scripts on one or more remote machines seamlessly. It is a preferred method due to its simplicity and flexibility.
How to Use `Invoke-Command` to Run Scripts Remotely
To run a script on a specified remote computer, you can use:
Invoke-Command -ComputerName "RemoteComputerName" -FilePath "C:\path\to\your\script.ps1"
This command tells PowerShell to execute the script located at `C:\path\to\your\script.ps1` on the designated remote computer.
Passing Parameters to Scripts
You can also pass parameters to your script by including the `-ArgumentList` parameter. Here’s an example:
Invoke-Command -ComputerName "RemoteComputerName" -FilePath "C:\path\to\your\script.ps1" -ArgumentList "param1", "param2"
This method allows your scripts to accept inputs, making them more versatile and powerful.
Using PSEXEC as an Alternative
While PowerShell Remoting is often sufficient, PSEXEC is another tool that can achieve similar results, especially in environments with restrictions.
Overview of PSEXEC
PSEXEC is a lightweight telnet-replacement that allows executing processes on remote systems. This tool provides a command-line alternative when PowerShell remoting isn't feasible or permitted.
Basic Example to Run a Command Remotely
Here’s a basic command using PSEXEC:
psexec \\RemoteComputerName -u UserName -p Password powershell.exe -ExecutionPolicy Bypass -File "C:\path\to\your\script.ps1"
This command runs the PowerShell executable and executes your script without adhering to the execution policy restrictions, making for a flexible option when needed.

Security Considerations
Understanding Security Context
When executing scripts remotely, it's vital to understand the security context. Verify that the user account has the appropriate permissions on both the local and remote machines.
Encrypting Your PowerShell Sessions
To secure your communications during remote connections, consider encrypting your PowerShell sessions. Use SSL with WinRM, which will secure data in transit. Follow these steps:
- Configure WinRM to use HTTPS: Ensure that your WinRM configuration is set to use SSL.
- Utilize self-signed certificates or certificates from a trusted authority to secure your sessions.
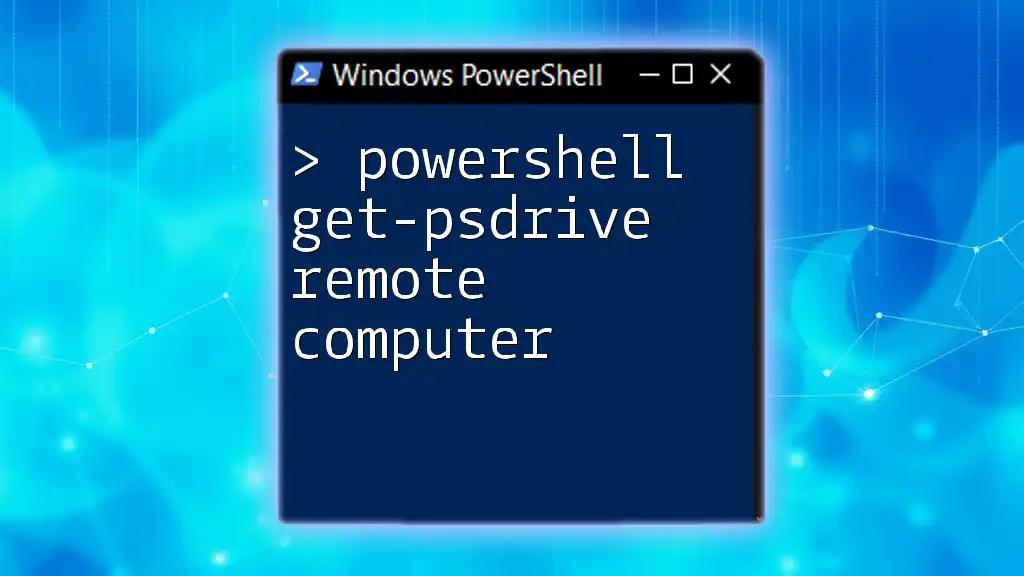
Troubleshooting Common Issues
Error Messages and Solutions
When running PowerShell scripts on remote computers, you may encounter various errors. Here are common issues:
- Access Denied: Ensure the executing user has adequate permissions to run the script remotely.
- Network-related Issues: Verify that the remote computer is reachable over the network.
Best Practices for Successful Execution
Adhere to these practices to ensure successful execution of your scripts:
- Validate script execution policies across machines.
- Perform connectivity checks to ensure all machines can communicate effectively.
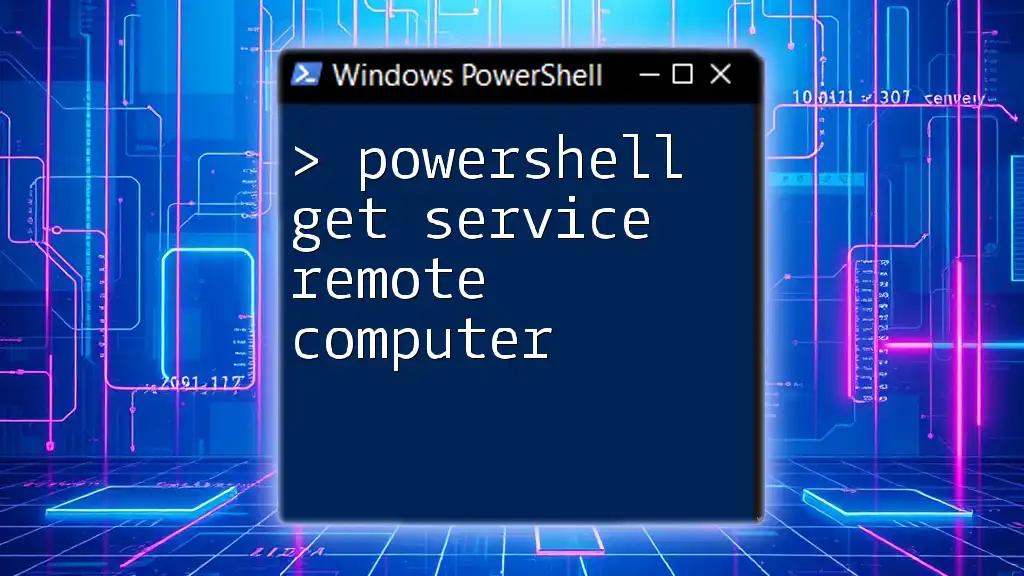
Conclusion
Recap of Key Points
Understanding how to PowerShell run script on remote computer significantly enhances your ability to manage multiple systems efficiently. By following the outlined steps—from enabling remoting to executing scripts—you can streamline various tasks.
Next Steps
Start setting up your own remoting environment today and explore more complex scripts to maximize your productivity. Resources, such as official Microsoft documentation and community forums, can provide additional insights as you continue your learning journey.
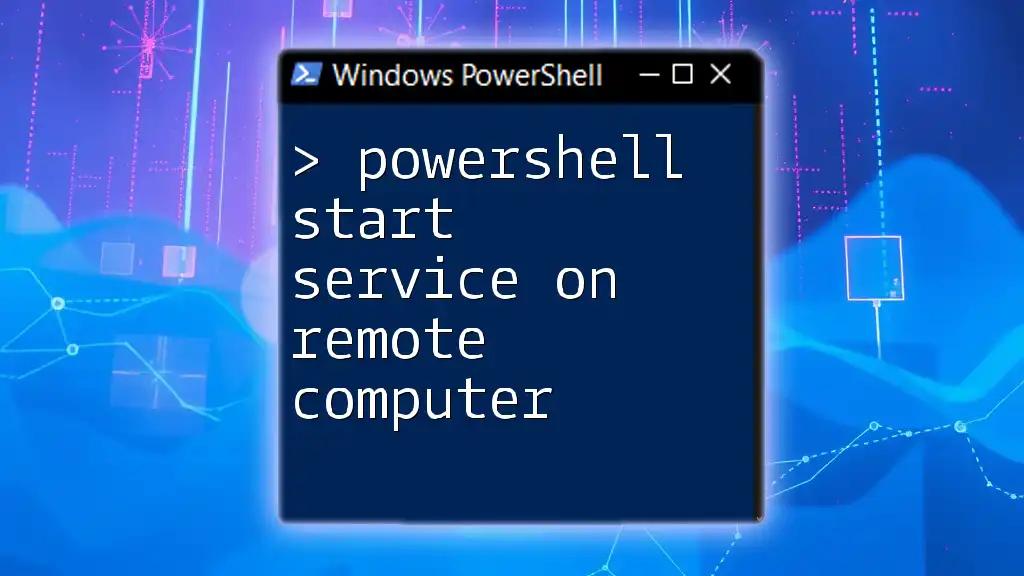
Frequently Asked Questions (FAQs)
Can I run PowerShell scripts on multiple remote computers at once?
Yes, by using `-ComputerName` with an array of computer names, you can target multiple remote systems simultaneously.
What are the limitations of PowerShell remoting?
Certain environmental factors, such as firewalls or misconfigured permissions, may limit remote execution capabilities.
How can I check if remoting is enabled on a remote PC?
Use the following command on your local machine:
Test-WSMan -ComputerName "RemoteComputerName"
This command checks if the remote machine supports PowerShell remoting.
Is it possible to execute commands without waiting for them to finish?
Yes, by using the `-AsJob` parameter with `Invoke-Command`, you can run scripts in the background, allowing you to continue working while the script executes.
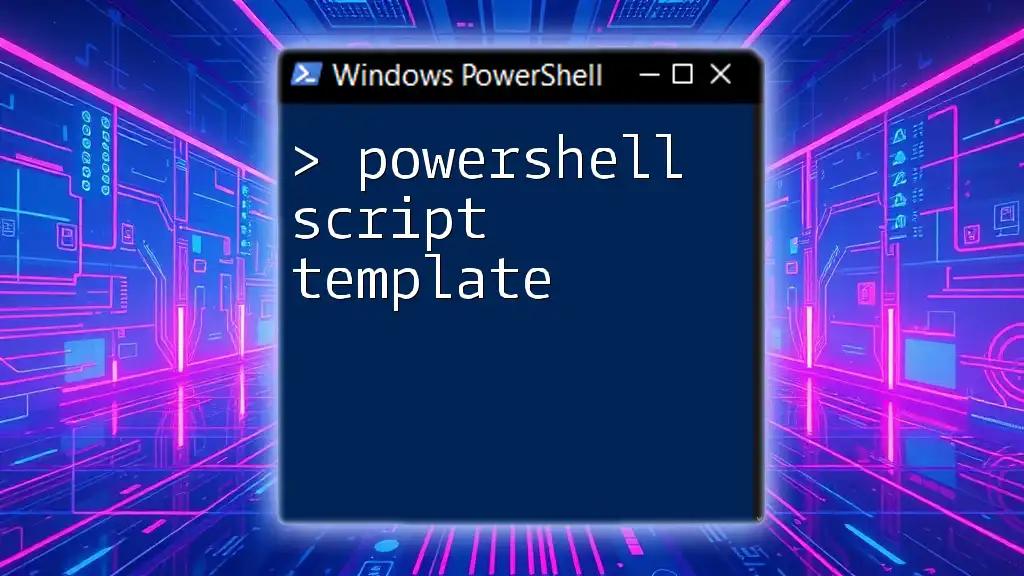
Code Examples and Resources
For deeper learning, explore these resources:
- Official [Microsoft documentation](https://docs.microsoft.com/en-us/powershell/scripting/learn/deep-dives/remote-management?view=powershell-7.1)
- PowerShell community forums for networking and support.
"PowerShell run script on remote computer" is no longer a complex task with these tools and best practices at your disposal!