In PowerShell, you can retrieve the exit code of the last command executed by accessing the automatic variable `$LASTEXITCODE`, which stores the exit code of the last Windows-based program that was run.
Here’s how you can check it:
# Run a command
ping google.com -n 1
# Get the exit code of the last command
$exitCode = $LASTEXITCODE
Write-Host "The exit code is: $exitCode"
Understanding Exit Codes
What is an Exit Code?
In the realm of command-line interfaces, an exit code (or return code) is a numeric value returned by a command after it has finished executing. In PowerShell, understanding exit codes is crucial for effective scripting and automation. An exit code typically indicates whether a command executed successfully or encountered an error. By adhering to these conventions, scripts can be made more robust, providing developers with insights on what went wrong when issues arise.
Common Exit Codes
While exit codes can vary between different commands and applications, there are some standard meanings:
- 0: Success. The command executed without any errors.
- 1: General error. The command failed but did not provide a specific error code.
- Other codes: Many commands return specific exit codes to indicate different types of errors. For example, a file not found error may return a different code than a permission denied error.
Being aware of these codes can greatly improve your script's ability to handle errors gracefully.

Getting the Exit Code in PowerShell
The `$?` Automatic Variable
In PowerShell, the automatic variable `$?` provides a Boolean value that indicates whether the last command was successful. If the last command was successful, `$?` returns `True`; otherwise, it returns `False`.
Example of using `$?`:
Get-Process
if ($?) {
"The last command was successful."
} else {
"The last command failed."
}
In this example, if `Get-Process` executes successfully, the output will confirm that. If it fails for any reason, the script will indicate a failure.
Accessing the Exit Code Directly
To retrieve the exit code directly, you can utilize the automatic variable `$LASTEXITCODE`. This variable holds the exit code of the last Windows-based command executed. It's essential to note that `$LASTEXITCODE` will contain the exit code only for external CMD commands and not for PowerShell cmdlets.
Example of retrieving the last exit code:
ping nonexistentdomain.com
$LASTEXITCODE
In this scenario, if the ping command fails because the domain does not exist, `$LASTEXITCODE` will typically store a non-zero value (the specific code representing the failure).
Understanding the Implications of Exit Codes
Exit codes play a vital role in powerfully automating scripts. By checking the exit code, you can determine whether to proceed with further actions or handle errors appropriately. For instance, if a critical installation step fails, checking its exit code can prevent subsequent commands from running and potentially causing further errors or complications.
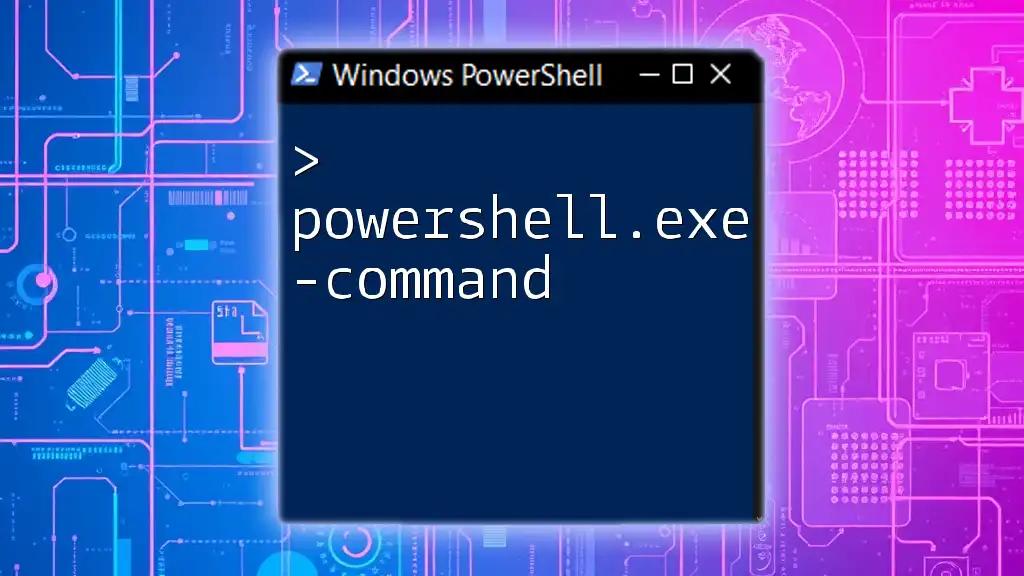
Practical Examples
Example 1: A Simple Command Check
Here's how you can get the exit code from a simple command to determine its success:
Get-Service -Name "wuauserv"
$LASTEXITCODE
If the command successfully retrieves information on the service, `$LASTEXITCODE` should return 0, signifying the command completed without issues. If the service doesn't exist, it may return a non-zero exit code.
Example 2: Handling Errors Gracefully
When executing commands that can fail, checking the exit code immediately afterward can help manage errors gracefully.
Consider the following:
Remove-Item "C:\NonExistentFile.txt"
if ($LASTEXITCODE -ne 0) {
"Failed to delete the file. Exit Code: $LASTEXITCODE"
}
In this example, if the attempted deletion fails (e.g., the file does not exist), you will receive a message that includes the exit code, providing a clear indication of what happened.
Example 3: Using in Scripts
Integrating the exit code into a script enhances its robustness. For instance, launching Notepad and waiting for it to exit:
$process = Start-Process -FilePath "notepad.exe" -PassThru
$process.WaitForExit()
if ($LASTEXITCODE -eq 0) {
"Notepad exited successfully!"
} else {
"Notepad exited with an error. Code: $LASTEXITCODE"
}
By waiting for Notepad to close and checking the exit code, this script can confirm whether Notepad closed without any issues, ensuring that any following commands can proceed with confidence.
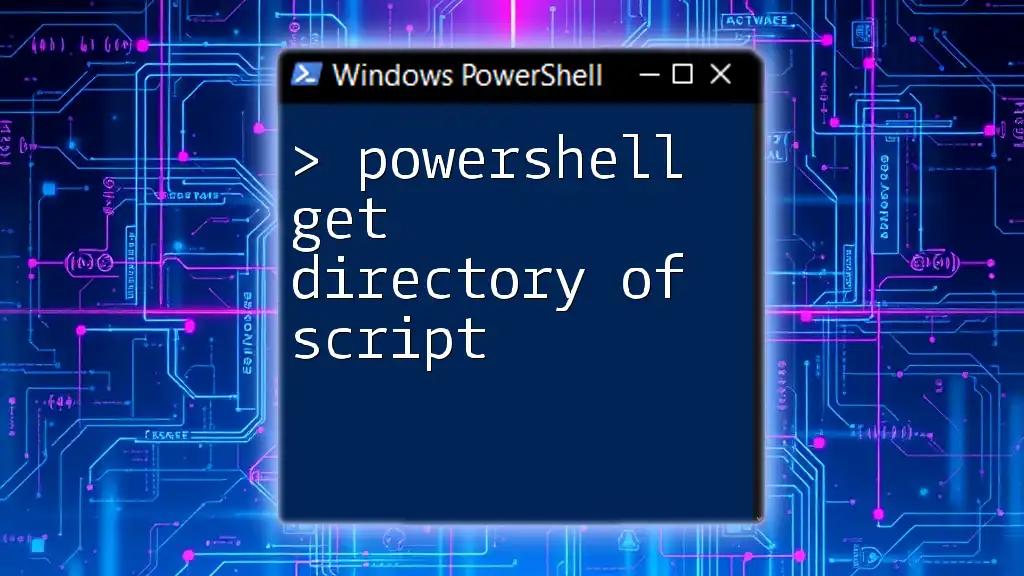
Advanced Techniques
Creating Functions to Wrap Command Execution
To consolidate command execution and exit code retrieval, you can create a PowerShell function. This approach not only enhances readability but also standardizes error handling across your scripts.
PowerShell Function Example:
function Invoke-CommandWithExitCode {
param (
[ScriptBlock]$Command
)
& $Command
return $LASTEXITCODE
}
This function allows you to run any command encapsulated within a script block, capturing its exit code for further processing.
Logging Exit Codes for Analysis
For more complex scripts, especially in production environments, logging exit codes can be invaluable. By saving exit codes to a log file, you can analyze error patterns and address recurring issues, increasing overall script reliability.

Best Practices
When to Check Exit Codes
Checking exit codes should be part of your routine coding practices. For critical commands, especially where the outcome affects downstream actions, always verify exit codes. This practice creates an additional layer of robustness, ensuring your script can react intelligently to errors.
Common Pitfalls to Avoid
When working with exit codes, some common mistakes include:
- Assuming all commands return exit codes: PowerShell cmdlets do not utilize `$LASTEXITCODE`; they indicate success using `$?`.
- Neglecting to check exit codes before proceeding to the next step, which could lead to cascading failures.
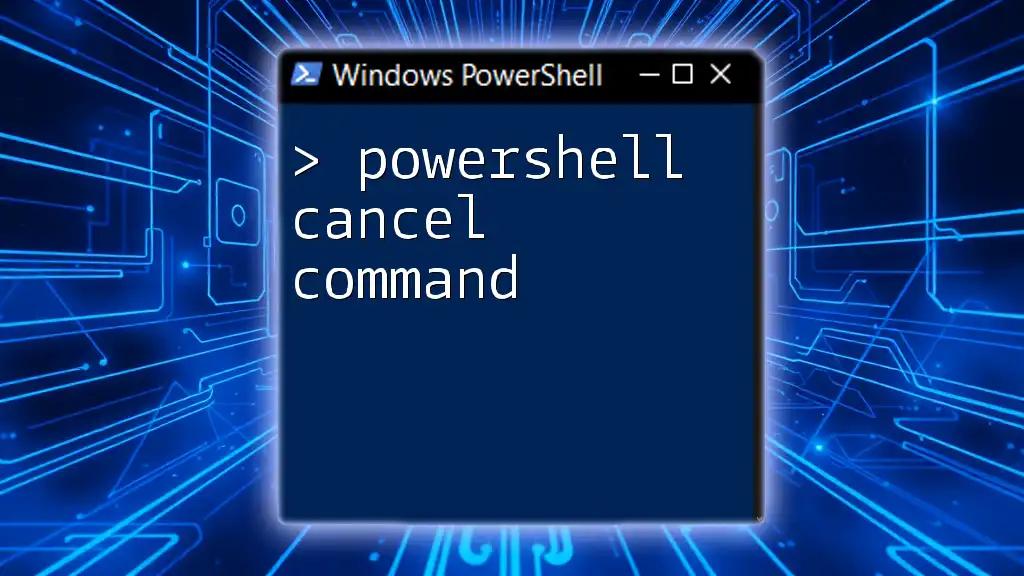
Conclusion
Understanding how to powerfully obtain the exit code of the last command in PowerShell is essential for creating reliable scripts. By integrating exit code checks, you enhance your scripts with error-handling capabilities. This knowledge not only aids in debugging but also empowers users to write more robust and maintainable PowerShell code.
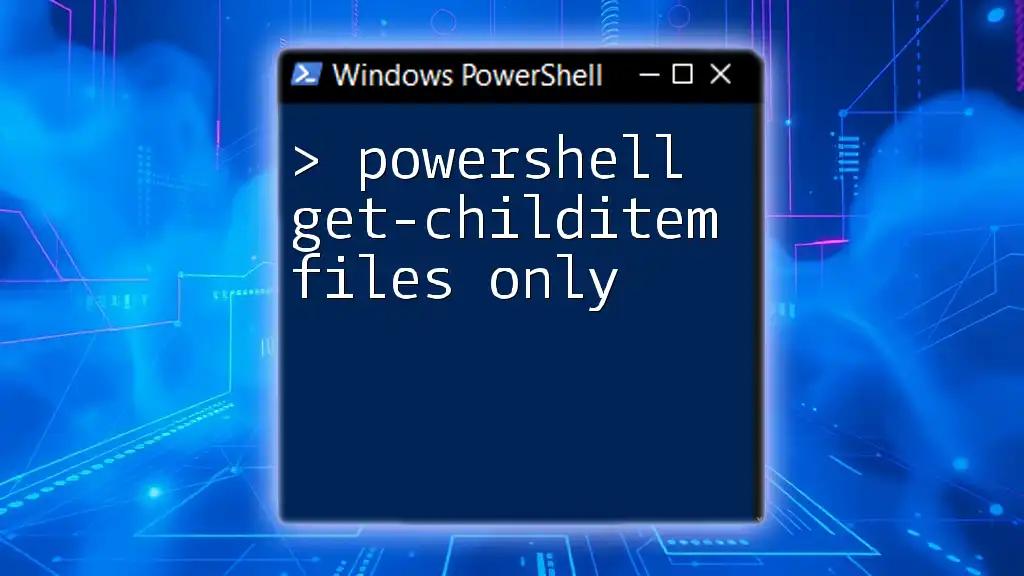
Resources for Further Learning
To deepen your understanding of PowerShell and exit codes, consider reviewing the official PowerShell documentation. Additional tutorials and books can provide further insights into effective scripting practices and advanced command usage.

Call to Action
We encourage you to share your experiences or questions regarding the use of exit codes in PowerShell. Join the community and subscribe for more valuable tips and tricks to enhance your PowerShell skills!