To measure the execution time of a command in PowerShell, you can use the `Measure-Command` cmdlet, which runs a script block and returns the time it took to complete.
Here’s a code snippet to demonstrate this:
$executionTime = Measure-Command { Get-Process }
Write-Host "Execution Time: $($executionTime.TotalSeconds) seconds"
Understanding Command Timing in PowerShell
What Does Timing a Command Mean?
Timing a command in PowerShell refers to measuring the duration it takes for a specific command or script block to execute. This is crucial for assessing performance and identifying bottlenecks in scripts or commands. By quantifying execution times, you can make informed decisions to optimize and streamline your workflows.
Why Timing Matters
Understanding how long a command takes to run helps in several ways:
- Performance Optimization: Identifying slow commands allows you to find faster alternatives or improve the existing script.
- Resource Management: Knowing the execution time assists in managing system resources, especially on shared environments or during scheduled tasks.
- Comparison of Command Efficiencies: Timing various approaches to achieve the same result can reveal the most efficient method for your specific context.
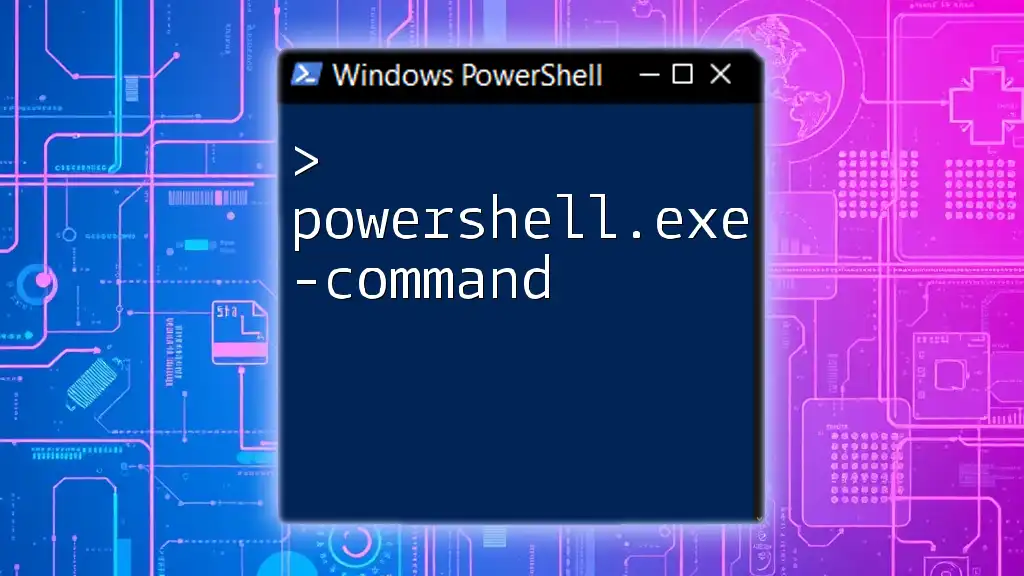
Using the Measure-Command Cmdlet
Overview of Measure-Command
The `Measure-Command` cmdlet is a built-in tool in PowerShell specifically designed for timing commands. It measures the time it takes to run a specified script block and returns an object containing the elapsed time.
Syntax and Parameters
The basic syntax of `Measure-Command` is straightforward:
Measure-Command -Expression { <your_command_here> }
Common parameters:
- `-Expression`: The script block whose execution time you want to measure.
- `-ErrorAction`: Controls how errors are handled.
- `-ErrorVariable`: Defines a variable where error information can be stored.
Example of Measure-Command
Here’s a simple example of using `Measure-Command` to time the `Get-Process` command:
$executionTime = Measure-Command { Get-Process }
Write-Host "Execution Time: $executionTime"
In this example, we wrap the `Get-Process` command inside the `Measure-Command` cmdlet. The `$executionTime` variable stores the resulting time, which can then be displayed to the user.
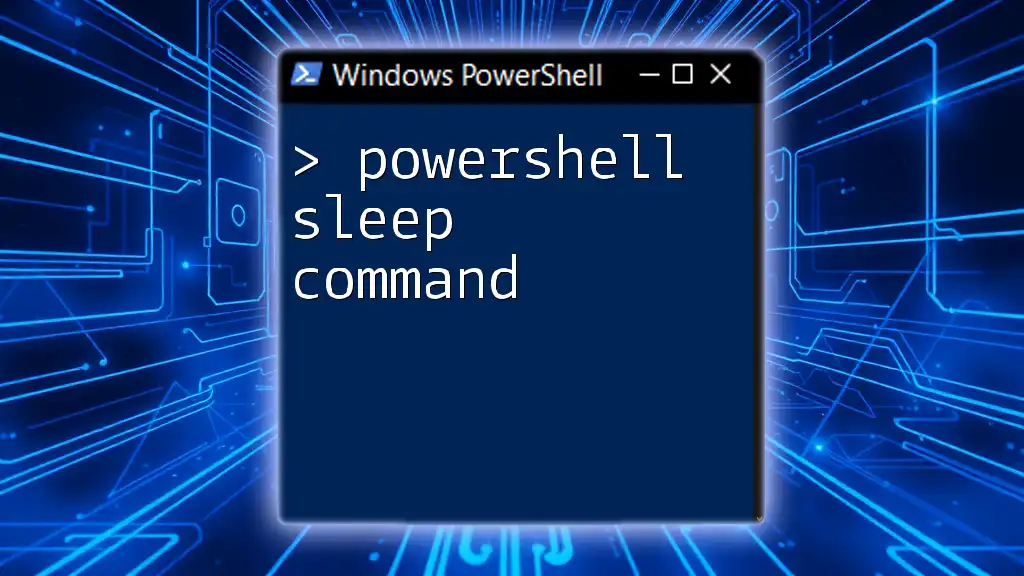
Comparing Different Methods of Timing
Measure-Command vs. Get-Date
While `Measure-Command` is effective, you can also use `Get-Date` for timing commands. Here’s how you’d implement it:
$startTime = Get-Date
Get-Process
$endTime = Get-Date
$duration = $endTime - $startTime
Write-Host "Duration: $duration"
This method calculates the duration by capturing the start and end times, and subtracting them to get the execution duration.
Pros and Cons:
- Measure-Command: Ideal for precise measurements, encapsulating the command execution.
- Get-Date: Provides more flexibility but may not always return as accurate results if the command execution completes quickly.
PowerShell v5 and Above Features
PowerShell version 5 and later include enhancements that streamline the process of timing commands. Reviewing changes in documentation can offer insights into new features that help further optimize command execution times.
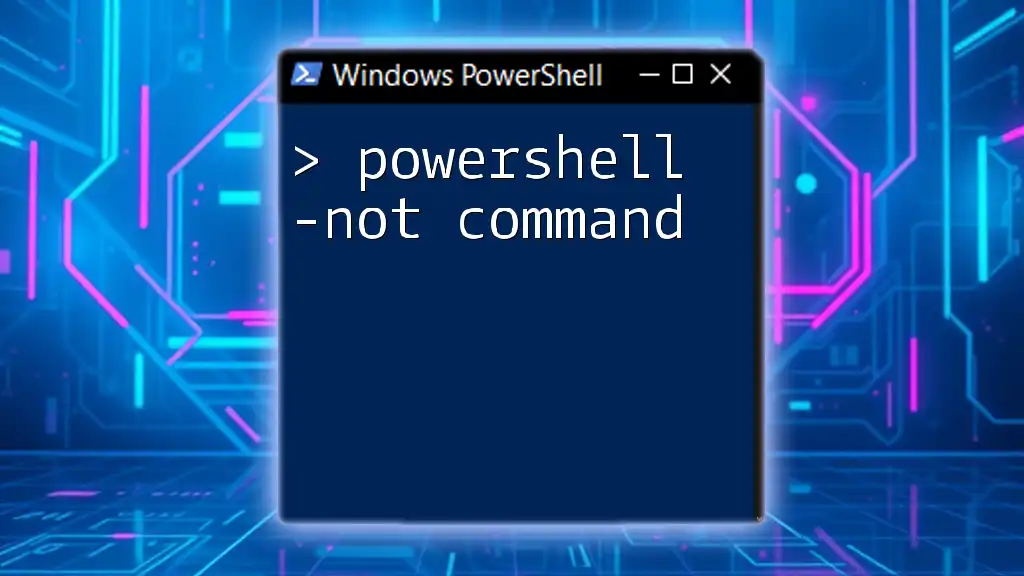
Analyzing Command Performance
Interpreting Results
When you measure performance, interpreting the output is crucial. The results typically include elapsed time formatted as a TimeSpan object, revealing total seconds, milliseconds, and so forth.
Common performance issues might include:
- Commands taking unexpectedly long due to system load or environmental factors.
- Inefficient scripts that could be improved through better coding practices.
Logging Execution Times
For anyone wanting to track performance over time, logging execution times is a formidable approach. The following provides a mechanism to log the execution time of commands:
$log = "execution_times.log"
"Command,ExecutionTime" | Out-File -FilePath $log
$executionTime = Measure-Command { Get-Process }
"$($_),$($executionTime.TotalMilliseconds)" | Out-File -FilePath $log -Append
This example writes the command and its execution time in milliseconds to a log file. Continuous logging enables trend analysis over time, offering invaluable data for performance tuning.
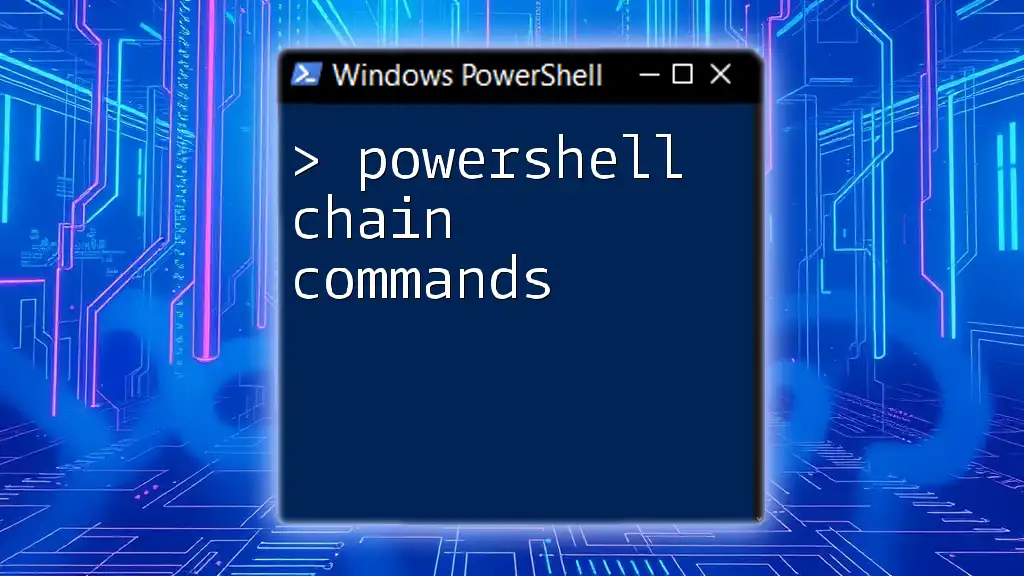
Advanced Timing Techniques
Timing Background Jobs and Scripts
When running commands in background jobs or scripts, timing can become slightly more nuanced. The following illustrates timing with a background job:
Start-Job -ScriptBlock { Measure-Command { Get-Process } }
Understanding how background jobs impact performance timing can help you accurately assess the efficiency of your scripts and tasks.
Using Profiling Tools
Utilizing external profiling tools can complement the built-in capabilities of PowerShell. Tools such as Windows Performance Analyzer or built-in .NET Profilers can provide vast insights into script performance and resource usage.
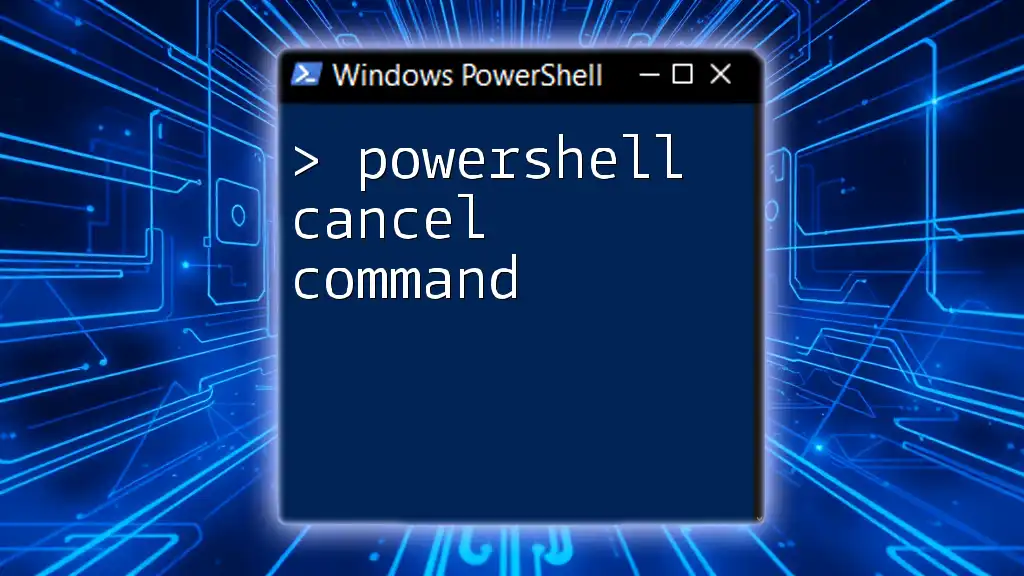
Best Practices for Timing PowerShell Commands
Timing in Scripting
Integrating timing measures into scripts can help automate the analysis and ensure consistent performance evaluations. For example, embedding `Measure-Command` within scripts can reveal areas for adjustment without the need for manual timing.
Tips for Accurate Measurements
For obtaining the most accurate measurements, consider the following recommendations:
- Run multiple times: To account for variability in system load, it’s best to run commands several times and average the results.
- Minimize background tasks: Execute your tests in an environment with minimal other resource demands to isolate performance.
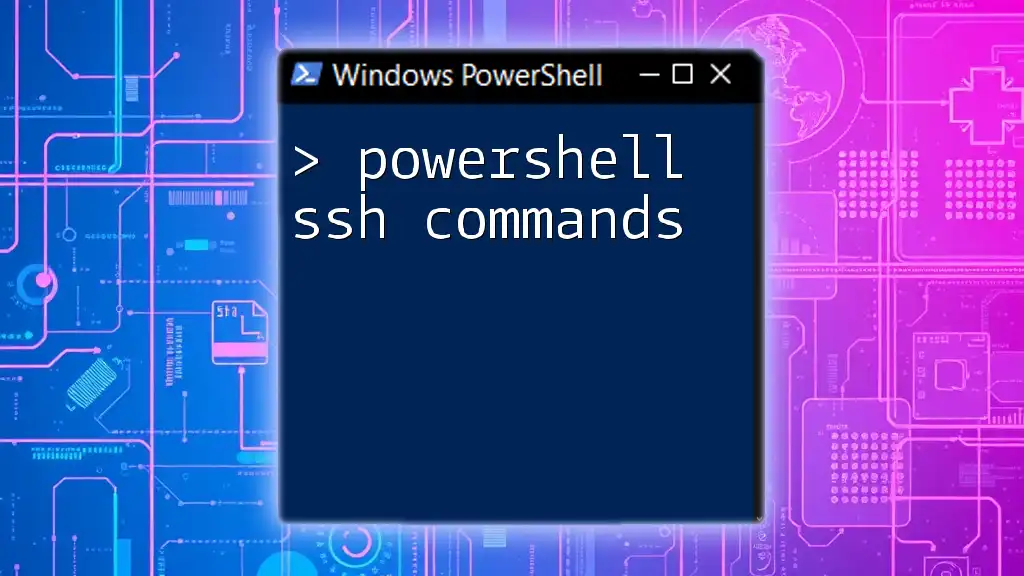
Conclusion
Timing commands in PowerShell is essential for optimizing script efficiency and performance. By leveraging cmdlets like `Measure-Command`, exploring alternative methods, and logging execution times, you can significantly enhance your command-line proficiency.
It's important to practice these techniques and incorporate them into your everyday workflows. As you become familiar with measuring execution time, you'll gain valuable insights that will lead you toward more effective PowerShell scripting.
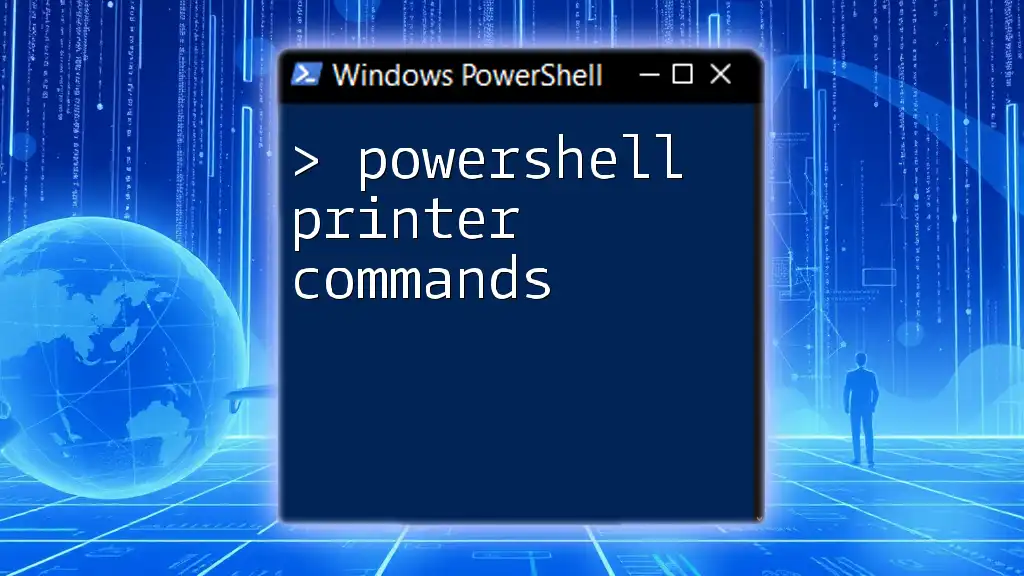
Additional Resources
To expand your knowledge further, consult the official PowerShell documentation, join PowerShell communities and forums, and explore literature on command optimization and effective scripting practices.
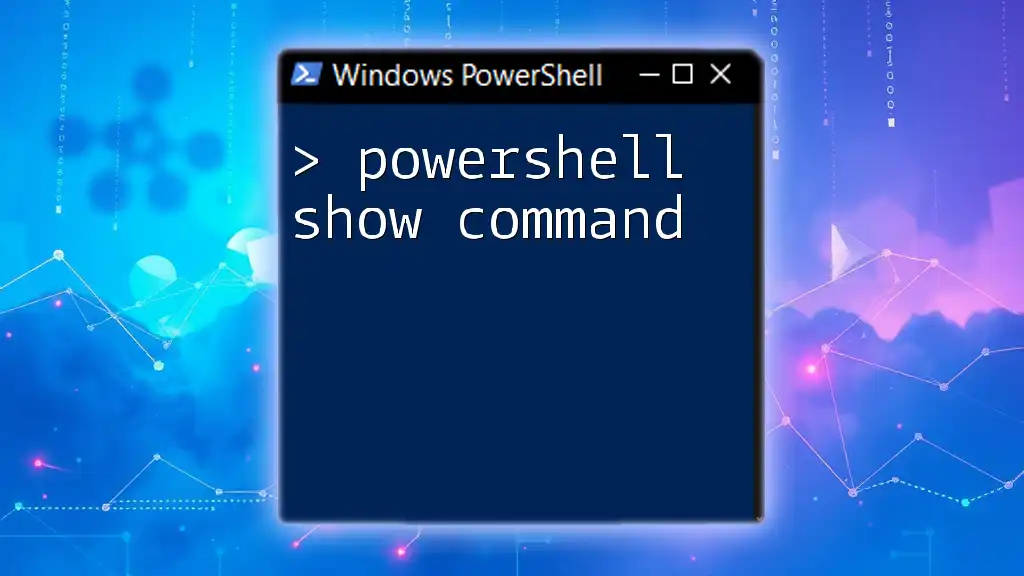
FAQs
Here, you can address common queries regarding timing commands in PowerShell and offer expert insights to deepen understanding. These FAQs can help bridge gaps in knowledge and offer realistic applications of the concepts discussed.