The "PowerShell move mouse" command allows you to programmatically control the mouse cursor's position on the screen using the .NET framework.
Here's a simple code snippet to move the mouse cursor to the specified coordinates (e.g., X=100, Y=200):
Add-Type -AssemblyName System.Windows.Forms
[System.Windows.Forms.Cursor]::Position = New-Object System.Drawing.Point(100, 200)
Understanding Mouse Movement in PowerShell
What is Mouse Automation?
Mouse automation refers to the ability to programmatically control the mouse cursor’s position, movements, and actions (like clicks and scrolls). In various scenarios such as testing applications, performing repetitive tasks, or even automating UI interactions, mouse automation can save significant time and mitigate human error. Using PowerShell commands to automate mouse movements allows you to script and execute these tasks efficiently.
How Does Mouse Movement Work?
Mouse movement involves manipulating the mouse cursor's coordinates on the screen. The X and Y positions correspond to where the cursor appears, starting from the top-left corner of the screen. Understanding how these coordinates function is vital for accurate mouse movements. PowerShell can interact with the Windows API, particularly the `System.Windows.Forms` namespace, to achieve this manipulation.

Setting Up Your PowerShell Environment
Required Tools and Libraries
For optimal mouse automation using PowerShell, you may need specific libraries that provide extended functionalities, like AutoIt or the .NET framework, particularly `System.Windows.Forms`. These libraries help access mouse movement functionalities seamlessly with PowerShell scripts.
To set up your environment, ensure you have the necessary libraries installed:
- AutoIt: A tool for automating the Windows GUI that can be accessed from PowerShell.
- .NET's System.Windows.Forms: Usually pre-installed with Windows but requires usage in your scripts by adding the assembly in PowerShell.
Verifying Your PowerShell Version
Before proceeding, verify that you're using a PowerShell version compatible with the features you want to implement. You can check your PowerShell version with the following command:
$PSVersionTable.PSVersion
Ensure you are using at least PowerShell 5.0 for the most robust capabilities.

PowerShell Mouse Move: Code Examples
Moving the Mouse to a Specific Coordinate
To initiate a simple mouse movement, you can set the mouse cursor to a designated screen coordinate using basic PowerShell commands. An example of this is shown below:
Add-Type -AssemblyName System.Windows.Forms
[System.Windows.Forms.Cursor]::Position = New-Object System.Drawing.Point(200, 200)
This code snippet first loads the necessary assembly and then sets the cursor to the coordinates (200, 200). Upon running this command, you will observe the cursor jump to that position on your screen.
Smooth Mouse Movement
Sometimes, rather than instantaneous jumps, you may want the cursor to move smoothly from one position to another. This can create a more natural feel, especially in simulations or automated tasks that interface with users directly. Here’s how to implement smooth mouse movement:
function Move-MouseSmoothly {
param (
[int]$TargetX,
[int]$TargetY,
[int]$Steps = 10
)
$CurrentPosition = [System.Windows.Forms.Cursor]::Position
for ($i = 1; $i -le $Steps; $i++) {
$X = $CurrentPosition.X + (($TargetX - $CurrentPosition.X) * $i / $Steps)
$Y = $CurrentPosition.Y + (($TargetY - $CurrentPosition.Y) * $i / $Steps)
[System.Windows.Forms.Cursor]::Position = New-Object System.Drawing.Point([math]::Round($X), [math]::Round($Y))
Start-Sleep -Milliseconds 10
}
}
Move-MouseSmoothly -TargetX 600 -TargetY 400
In this code, we define a function `Move-MouseSmoothly` which takes the target X and Y coordinates and the number of steps for the movement. The function computes the intermediate coordinates, creating a gradual and smooth transition of the cursor across the screen.
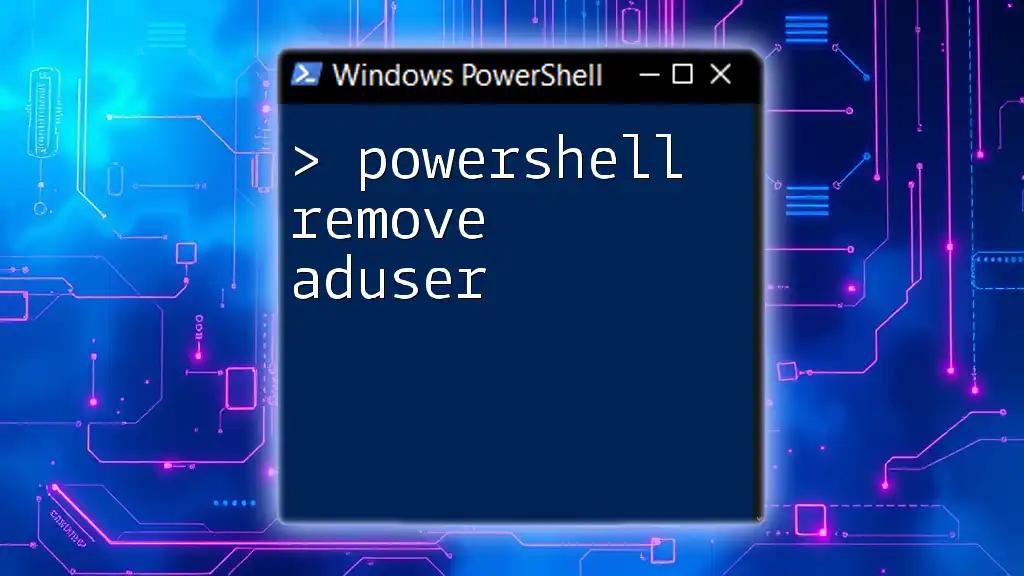
Advanced Mouse Movement Techniques
Combining Mouse Movement with Click Actions
Once you've positioned the mouse, you may need to perform clicks. Combining mouse movements with click actions allows you to interact with UI elements programmatically. Here’s how you can achieve that:
Add-Type -AssemblyName System.Windows.Forms
function Move-AndClick {
param (
[int]$X,
[int]$Y
)
[System.Windows.Forms.Cursor]::Position = New-Object System.Drawing.Point($X, $Y)
[System.Windows.Forms.SendKeys]::SendWait("{LEFTCLICK}")
}
Move-AndClick -X 500 -Y 300
This function `Move-AndClick` both moves the mouse to the specified coordinates and simulates a left-click action. It is especially useful for automating tasks that require clicking buttons or menus in GUIs.
Automating with Loops
For continuous or repetitive tasks, looping through your mouse movements and clicks can enhance automation. However, this must be handled cautiously to avoid unintended industry-wide repercussions. Here’s an example of a continuous mouse action:
while ($true) {
Move-AndClick -X 700 -Y 500
Start-Sleep -Seconds 2
}
In this script, the function `Move-AndClick` will execute indefinitely, clicking every 2 seconds at the specified coordinates. This illustrates the power of loops in automation; however, it is essential to define a safe exit strategy or condition to prevent accidental overload.

Troubleshooting Common Issues
Potential Errors and Solutions
When using PowerShell to move the mouse, various issues may arise, primarily concerning permissions or environmental settings. Common errors might include access denied, cursor position not updating, or .NET Framework conflicts. Make sure your PowerShell session has adequate permissions (run as administrator, if necessary), and consider checking that the required libraries and assemblies are correctly loaded.
Best Practices for Smooth Mouse Automation
- Use Short Movements: This minimizes the risk of unexpected interactions.
- Avoid Conflicts with User Actions: Ensure running scripts do not interfere with the user’s input.
- Optimize Performance: Experiment with sleep intervals and movement steps to strike a balance between responsiveness and resource consumption.
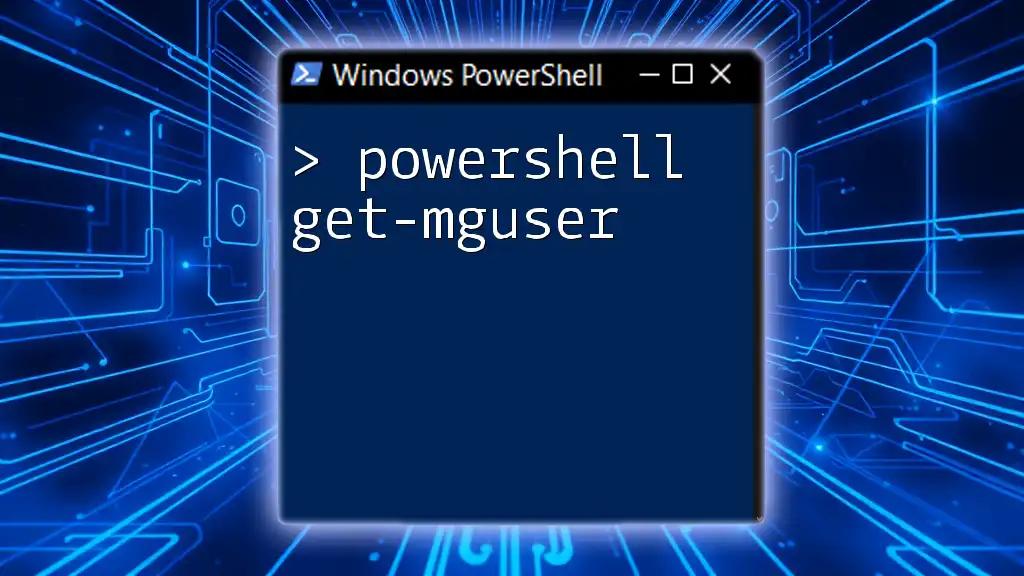
Conclusion
PowerShell’s capability to move the mouse programmatically opens up a multitude of possibilities for automation in Windows environments. By leveraging your PowerShell skills to automate mouse movements and clicks, you can simplify repetitive tasks, streamline testing, and enhance productivity. Dive into scripting with PowerShell and explore creative ways to apply these techniques in your daily workflows!