To convert a UTC time to local time in PowerShell, you can use the following command:
$utcDateTime = Get-Date "2023-10-03T15:00:00Z"; $localDateTime = [TimeZone]::CurrentTimeZone.ToLocalTime($utcDateTime); $localDateTime
Understanding Time Zones
What is UTC?
Coordinated Universal Time (UTC) is the primary time standard by which the world regulates clocks and time. It does not change with the seasons and serves as the same reference point across the globe. In computing, UTC is crucial as it allows systems to coordinate time-related events regardless of geographical location. This consistency is especially important in logging data, scheduling events, and synchronizing systems.
What is Local Time?
Local time refers to the time observed in a specific geographical location, which can differ significantly from UTC, especially when accounting for local time zones and variations like Standard Time and Daylight Saving Time (DST). Local time reflects factors such as:
- Differences in daylight savings policies across regions, which can shift local time by an hour.
- The geographic location, with longitudinal differences affecting time.
Understanding the differentiation between UTC and local time is imperative because the conversion between them is frequently required in scripts and applications that deal with time-sensitive data.
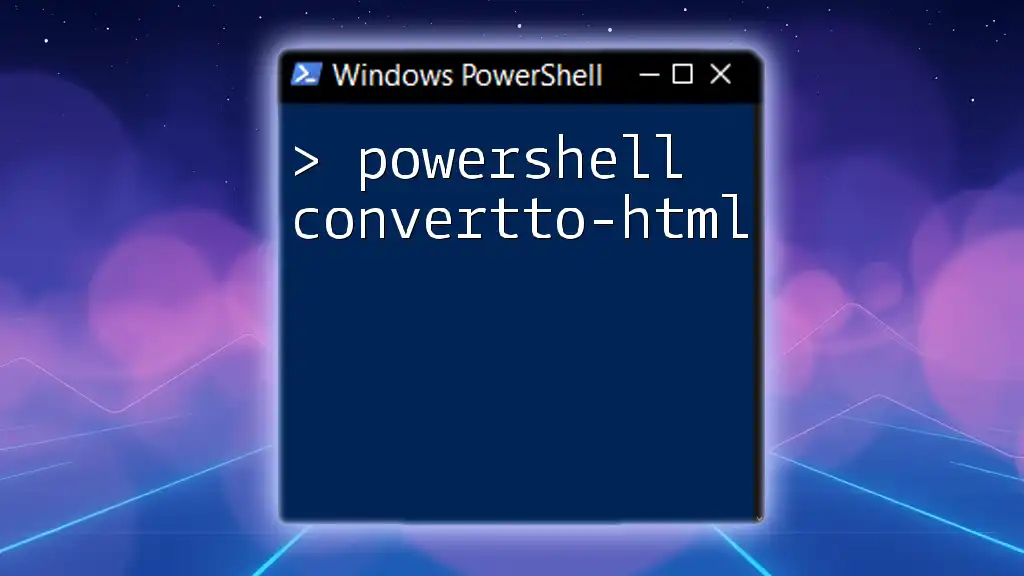
PowerShell Basics for Time Management
Introduction to DateTime Object
In PowerShell, the DateTime object is fundamental for handling date and time operations. This object allows script developers to manipulate and format dates easily.
To create a DateTime object representing the current UTC time, you can use the following command:
$utcDateTime = [DateTime]::UtcNow
This command initializes `$utcDateTime` with the current date and time set to UTC.
Retrieving Local Time
To find the local time in PowerShell, the `Get-Date` cmdlet serves well. By calling `Get-Date`, you retrieve the current local date and time. For instance:
$localDateTime = Get-Date
This command simply fetches and stores the local time in the variable `$localDateTime`.
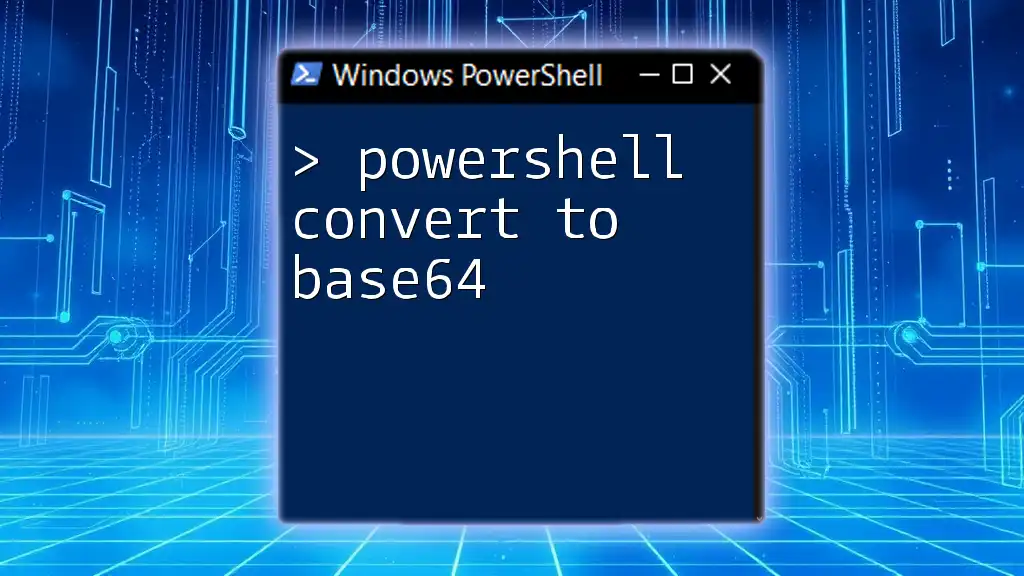
Converting UTC to Local Time in PowerShell
Using the TimeZoneInfo Class
PowerShell provides robust capabilities for converting time through the `TimeZoneInfo` class. This class offers information about the time zones and allows for conversions between UTC and local time.
To convert UTC to local time, you can apply this code:
$utcDateTime = [DateTime]::UtcNow
$localTimeZone = [System.TimeZoneInfo]::Local
$localDateTime = [System.TimeZoneInfo]::ConvertTime($utcDateTime, [System.TimeZoneInfo]::Utc, $localTimeZone)
In this example, `$localDateTime` will now contain the equivalent local time for the currently captured UTC time.
Using Get-Date with -Date Parameter
Another method for converting UTC to local time is by leveraging the `Get-Date` cmdlet with the `-Date` parameter. This approach is straightforward:
$localTime = Get-Date -Date ($utcDateTime.ToString("yyyy-MM-ddTHH:mm:ssZ")) -Format "yyyy-MM-dd HH:mm:ss"
Here, `Get-Date` formats the UTC DateTime into a string, which is then parsed into local time.
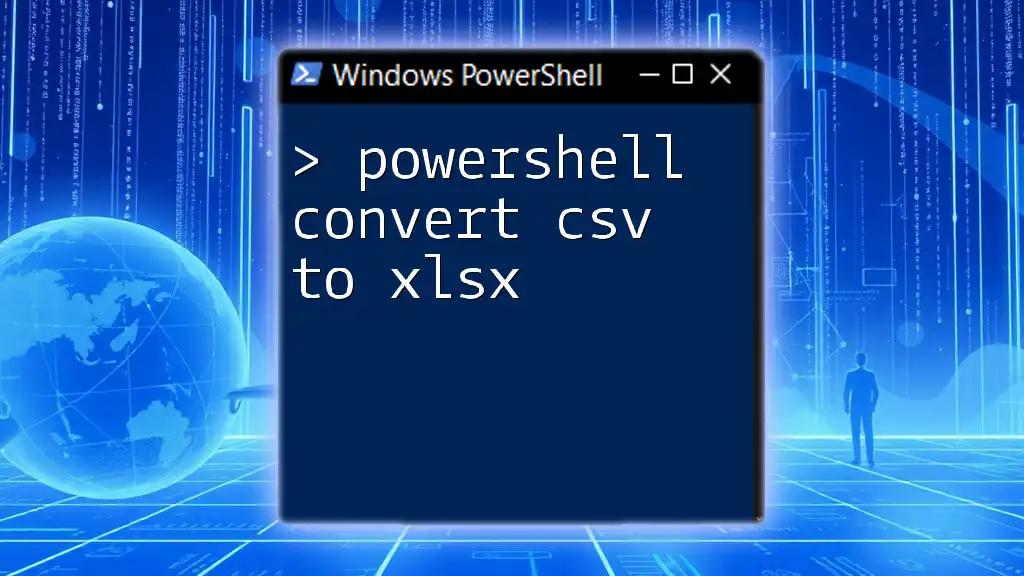
Practical Applications
Scenario 1: Logging Events with Timestamps
When managing logs in a system, timestamps are often recorded in UTC. Understanding how to convert those timestamps into local time for analysis is essential for accurate reporting.
For example, if you have a log entry recorded in UTC:
$logEntryUTC = "2023-10-05T15:30:00Z"
$utcDateTime = [DateTime]::Parse($logEntryUTC)
$localDateTime = [System.TimeZoneInfo]::ConvertTime($utcDateTime, [System.TimeZoneInfo]::Utc, $localTimeZone)
Write-Output "Local Time: $($localDateTime)"
In this scenario, after parsing the UTC timestamp, the script will output the corresponding local time, making it easier to analyze logged events relevant to the local time zone.
Scenario 2: Scheduling Tasks Across Time Zones
When scheduling tasks, ensuring that the timing aligns with local time is essential, particularly if users are in different regions.
If you wish to schedule a task according to UTC time of `2023-10-05T15:00:00Z`, you can convert it using:
$taskScheduledUTC = [DateTime]::Parse("2023-10-05T15:00:00Z")
$localScheduledTime = [System.TimeZoneInfo]::ConvertTime($taskScheduledUTC, [System.TimeZoneInfo]::Utc, $localTimeZone)
This sample script converts the scheduled UTC time to the local equivalent, ensuring tasks execute at the right time for users in various regions.
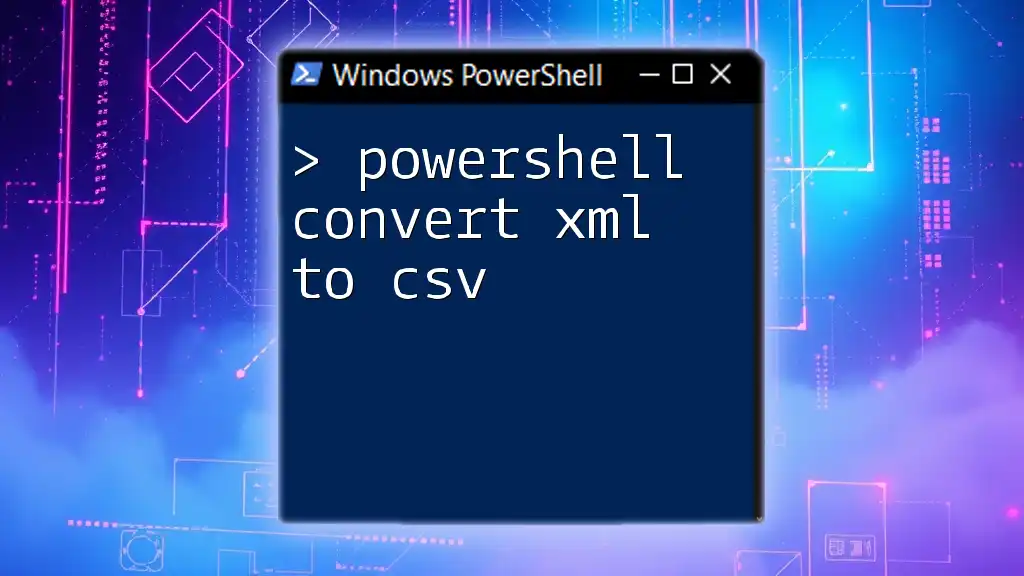
Troubleshooting Common Issues
TimeZone Differences
When converting between UTC and local time zones, it's important to note that not all locations observe Daylight Saving Time uniformly. This inconsistency can lead to discrepancies. To ensure accurate conversions, always check the target time zone's rules regarding DST.
Handling Invalid Date Formats
One common pitfall developers face when working with date and time conversions in PowerShell is invalid date formats. It’s crucial to validate dates before parsing them to prevent runtime errors.
For instance:
try {
$localDateTime = [DateTime]::Parse("InvalidDateString")
} catch {
Write-Output "Error: Invalid date format."
}
In this code, the `try-catch` block captures the error from an invalid date format, allowing you to gracefully handle issues without crashing the script.
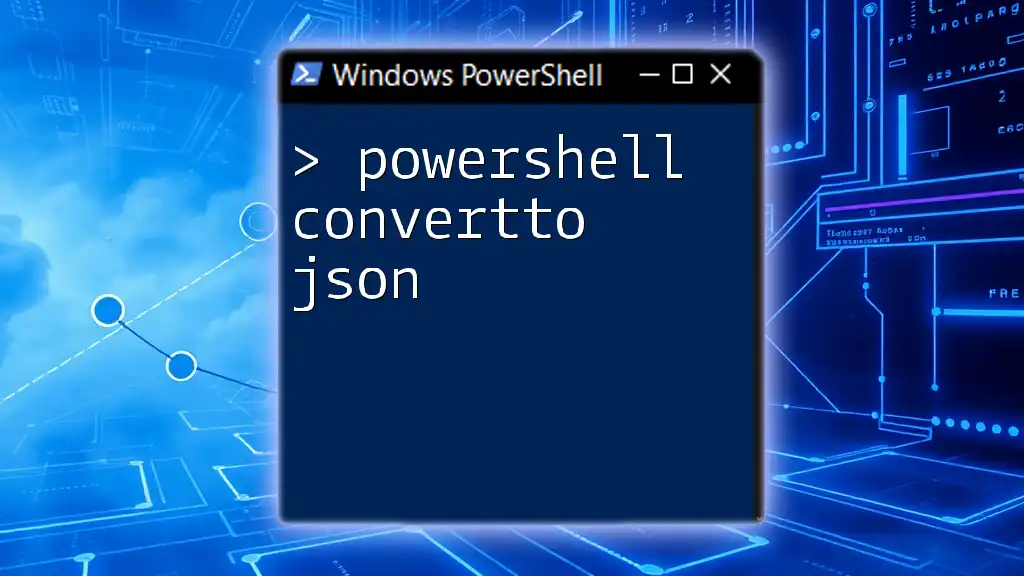
Conclusion
Understanding how to effectively powerfully convert UTC to local time in PowerShell is key to enhancing your scripting capabilities. Whether you are handling time-stamped logs, scheduling tasks, or converting user-defined times, mastering these techniques allows for greater accuracy and efficiency in your scripts. Practice the examples provided to solidify your understanding of this essential topic, and continue exploring PowerShell's extensive functionalities to further enhance your proficiency.