The `-in` operator in PowerShell checks if a specified value exists within a collection of values and returns a Boolean result.
# Example of using the -in operator
$fruits = 'apple', 'banana', 'orange'
$checkFruit = 'banana'
if ($checkFruit -in $fruits) {
Write-Host "$checkFruit is in the list of fruits."
} else {
Write-Host "$checkFruit is not in the list of fruits."
}
Understanding the -in Operator
What is the -in Operator?
The `-in` operator in PowerShell is a membership operator that checks if a specified value exists within a collection, like an array or list. It evaluates to True or False, allowing for elegant conditional checks in scripts. This operator significantly simplifies checking membership compared to using other methods.
Comparison with Other Operators
-eq vs. -in
While `-eq` checks for equality between a single value and another, `-in` is specifically designed for verifying if an element belongs to a collection. For example, if you want to find out if a fruit exists in a list, `-in` serves this purpose directly:
$fruit = "banana"
$fruits = @("apple", "banana", "cherry")
$fruit -eq "banana" # Evaluates to True
$fruit -in $fruits # Also evaluates to True
Choosing `-in` can make your code cleaner and easier to read, particularly when dealing with arrays.
Performance Considerations
Using the `-in` operator is generally more efficient than looping through each item in a collection to determine if a value exists. For larger datasets, particularly when conducting multiple membership checks, this operator can save time and improve performance.

Syntax of the -in Operator
Basic Syntax
The basic syntax for the `-in` operator is:
$value -in $collection
- $value represents the value you want to examine for membership.
- $collection is the array, list, or any enumerable entity where you are searching for the presence of the value.

Practical Examples of the -in Operator
Example 1: Checking Membership in an Array
To illustrate the `-in` operator in action, consider this snippet that checks if a certain fruit exists in a predefined list:
$fruits = @("apple", "banana", "cherry")
"banana" -in $fruits
In this example, the expression evaluates to True because "banana" is indeed part of the `$fruits` array. If you check for a fruit not in the list, such as "grape", the result will be False.
Example 2: Using -in with Conditional Statements
The strength of the `-in` operator truly shines when incorporated into conditional statements, allowing for streamlined logic control. Here’s an example:
$allowedUsers = @("Alice", "Bob", "Charlie")
$currentUser = "Bob"
if ($currentUser -in $allowedUsers) {
"Access Granted"
} else {
"Access Denied"
}
In this snippet, if `$currentUser` is found in `$allowedUsers`, the output will display "Access Granted". If it were someone not in the list, such as "David", it would output "Access Denied".
Example 3: Combining -in with Other Logic Operators
It is common to use the `-in` operator alongside other operators for more complex logic. Here is an illustrative example:
$statusCodes = @("Active", "Pending", "Closed")
$currentStatus = "Active"
$anotherCondition = $true # Some other condition for illustration
if ($currentStatus -in $statusCodes -and $anotherCondition) {
"Status is valid and other conditions met."
}
In this case, the code checks if `$currentStatus` is among the listed `$statusCodes` and whether another condition is `True`. If both conditions are satisfied, the output confirms the validity of the status.
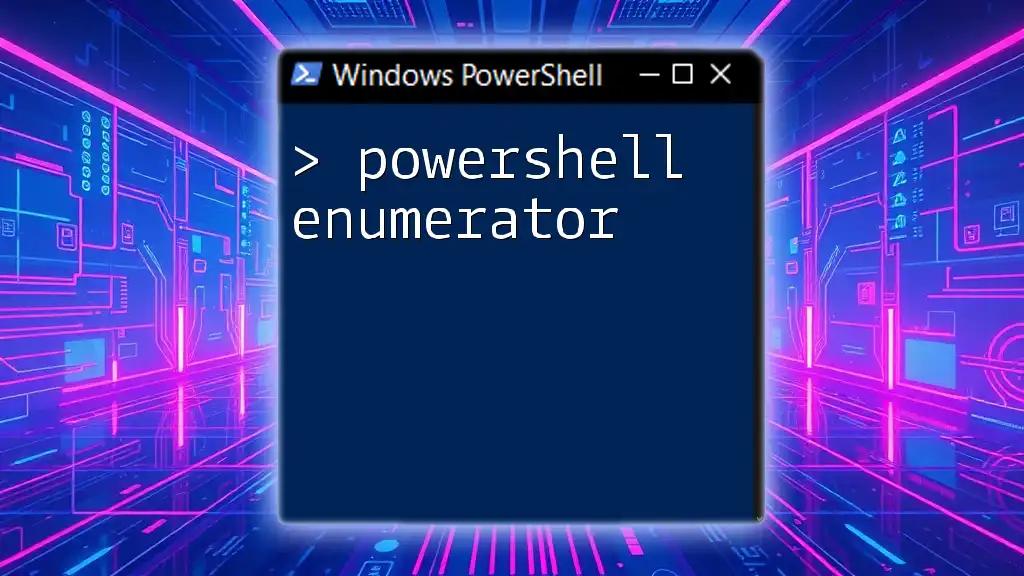
Common Use Cases
Data Filtering
A practical implementation of the `-in` operator is in data filtering scenarios. Using `-in` can simplify the process of eliminating unwanted entries from a dataset. Here’s how it works:
$users = Get-Content "users.txt" # Suppose this retrieves a list of usernames.
$blacklist = @("user1", "user2")
$nonBlacklistedUsers = $users | Where-Object { $_ -notin $blacklist }
In this example, we retrieve a list of users and then filter out those in the `$blacklist`. The resulting `$nonBlacklistedUsers` collection will exclude "user1" and "user2".
Enhancing Readability in Scripts
Using the `-in` operator can enhance the readability and maintainability of your scripts compared to traditional looping mechanisms. Consider this example with a `foreach` loop:
$fruits = @("apple", "banana", "cherry")
$searchFruit = "banana"
$found = $false
foreach ($fruit in $fruits) {
if ($fruit -eq $searchFruit) {
$found = $true
break
}
}
if ($found) {
"Fruit found!"
}
Using the `-in` operator allows you to streamline this process significantly:
$fruits = @("apple", "banana", "cherry")
$searchFruit = "banana"
if ($searchFruit -in $fruits) {
"Fruit found!"
}
This not only reduces code complexity but also makes the intent of the code clearer at a glance.

Common Pitfalls and Troubleshooting Tips
FAQs about the -in Operator
Common Misunderstandings
One common misunderstanding is that the `-in` operator does not perform case-sensitive checks by default. Users should verify their dataset's casing when using `-in`, as "APPLE" and "apple" would be treated as different values.
Troubleshooting Steps
When the `-in` operator yields unexpected results, double-check the contents of your collection. Outputting the collection to examine its contents can clarify whether the expected values are present:
$fruits = @("apple", "banana", "cherry")
$fruits
Outputting the collection can help identify any issues with data entries.
Performance Issues
When dealing with large collections, ensure you're aware of the performance limits of your application. Using `-in` requires iterating through the collection, which can result in performance drawbacks for very extensive datasets. In such cases, consider alternative data structures or filtering methods to optimize performance.

Conclusion
In this article, we’ve explored the PowerShell -in operator, which provides a concise way to check for membership in collections. The `-in` operator not only enhances readability but also allows for efficient scripting while avoiding intricate loops.
As you continue to experiment with PowerShell, employing the `-in` operator will likely enhance your scripting capabilities while making your code cleaner and more efficient. Whether you are filtering data, checking user permissions, or performing complex validations, mastering the `-in` operator can significantly elevate your PowerShell expertise.
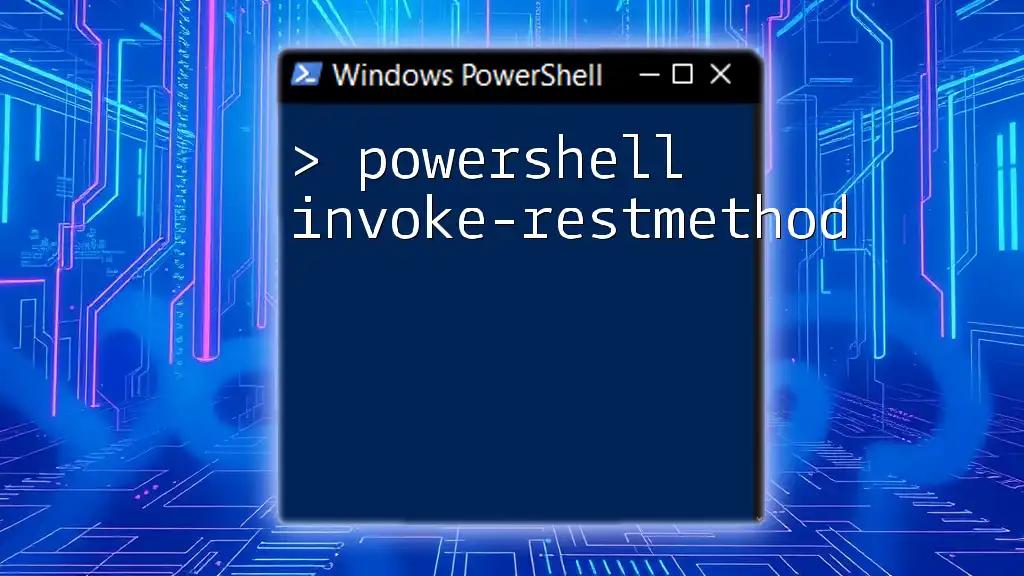
Additional Resources
For further learning, refer to the official Microsoft documentation on the `-in` operator to explore advanced features and use cases. Additionally, consider delving into more extensive PowerShell courses to broaden your command-line skills and automation techniques.
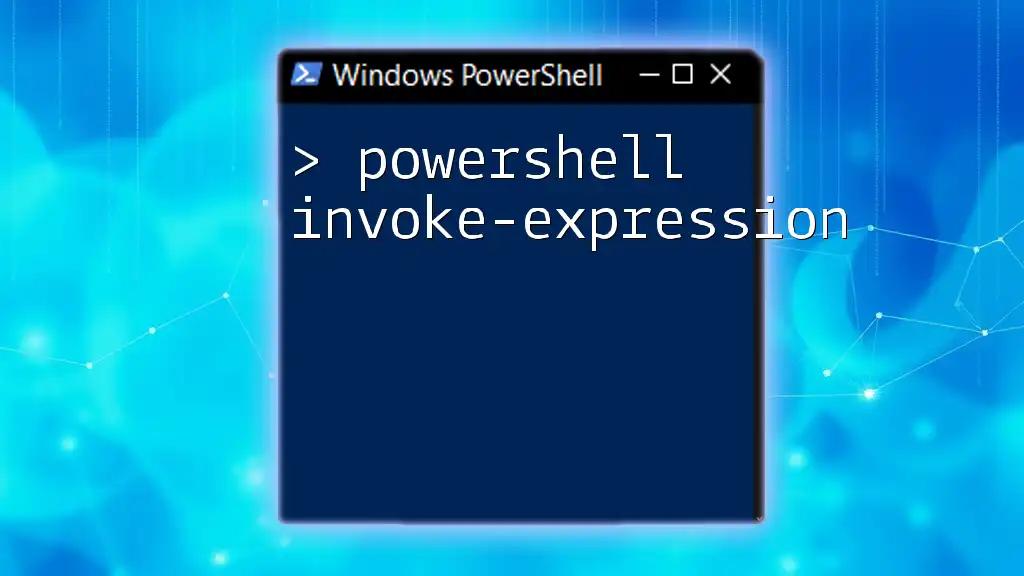
About Us
At our company, we are dedicated to empowering users through concise and effective PowerShell training, enabling you to harness the full potential of this powerful scripting language. Join us in exploring the world of PowerShell and enhance your automation capabilities today!