The `Get-Input` command in PowerShell allows you to prompt the user for input and can be implemented as follows:
$input = Read-Host "Please enter your input"
Write-Host "You entered: $input"
Understanding PowerShell Input Mechanisms
User input in PowerShell refers to the data entered by users that can shape the execution of scripts. This capability allows for dynamic and interactive scripts that adapt to specific user needs. When developing scripts, understanding how to ask for and handle user input is crucial for creating versatile and user-friendly automation.
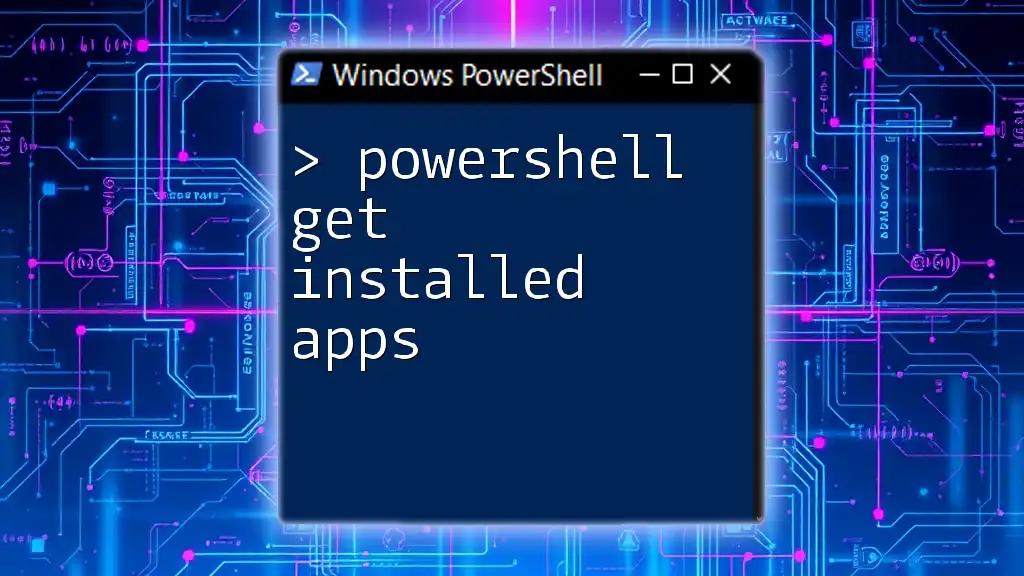
PowerShell Get Input: The Basics
What is "Get Input"?
In PowerShell, "Get Input" pertains to requesting and retrieving data from users through prompts. This allows scripts to incorporate user-specific data, enhancing their functionality.
Benefits of Using User Input in PowerShell Scripts
- Personalization: Scripts can be tailored to user preferences and needs, resulting in a more engaging experience.
- Dynamic execution: By incorporating user responses, scripts can execute different paths based on input, making them versatile.
- Enhanced user experience: Engaging the user throughout the script execution encourages interaction and ensures that the output is relevant to them.

PowerShell Prompt User for Input: Key Commands
Using the Read-Host Cmdlet
One of the simplest ways to prompt users for input in PowerShell is employing the `Read-Host` cmdlet. This built-in cmdlet allows you to display a prompt and capture user input effectively.
Basic Syntax:
$input = Read-Host "Please enter your input"
Example Code Snippet
$name = Read-Host "Enter your name"
Write-Host "Hello, $name!"
Explanation: In this example, the script asks for the user’s name and then greets them. The `Read-Host` cmdlet captures whatever the user types, and `Write-Host` displays a personalized greeting.
Using the Parameter Attribute for Input
Another efficient method for getting user input is to define parameters within your script. This approach is especially useful for scripts that need to accept multiple inputs.
Example:
param (
[string]$name
)
Write-Host "Hello, $name!"
Discussion: By using the `param` keyword, you define `$name` as a parameter that users can provide when running the script. This method promotes reusability and clarity in your scripts.
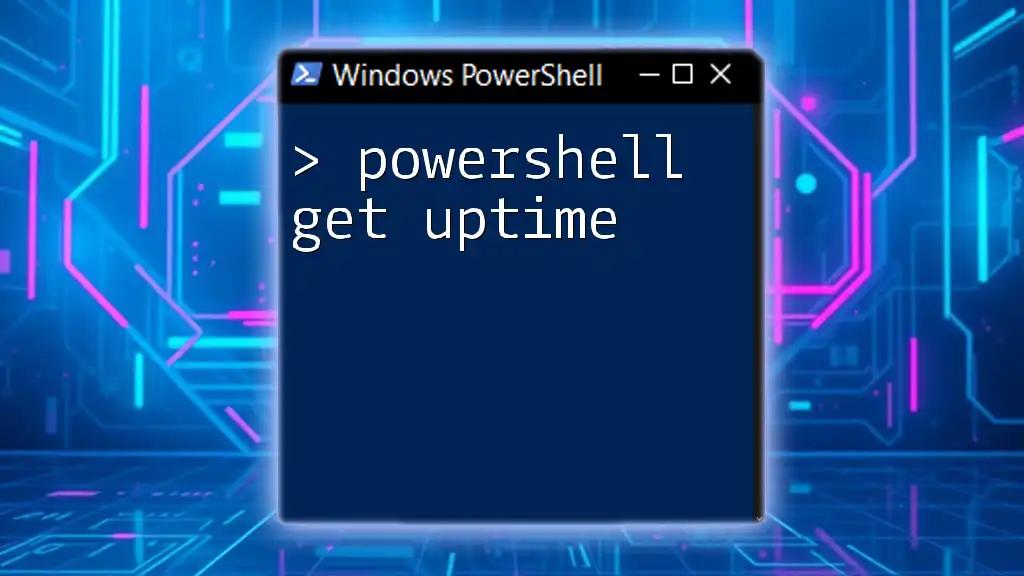
Advanced Techniques for Asking Input in PowerShell
Validating User Input
Input validation is crucial; it ensures that the data users supply meets the expected format, enhancing script reliability. You can implement basic validation through conditional checks.
Example Code Snippet:
$age = Read-Host "Enter your age (must be a number)"
if ($age -match '^\d+$') {
Write-Host "You are $age years old."
} else {
Write-Host "Invalid input."
}
In this example, the script checks if the provided input is a valid number. If not, it provides a clear message indicating the input is invalid, thus improving the user experience.
Creating Custom Input Prompts
Using Functions for Reusable Input
Creating functions to handle user input can make your code cleaner and more organized. This technique allows developers to create reusable prompts throughout their scripts.
Example Implementation:
function Get-UserInput {
param (
[string]$prompt
)
return Read-Host $prompt
}
$username = Get-UserInput "What is your username?"
Write-Host "Welcome, $username!"
In this example, the `Get-UserInput` function simplifies the process of gathering input, making the script more modular.
Using GUI Input Forms
For those looking to create a more visually engaging input method, PowerShell can utilize Windows Forms for graphical input.
Example Code Snippet:
Add-Type -AssemblyName System.Windows.Forms
$form = New-Object System.Windows.Forms.Form
$textBox = New-Object System.Windows.Forms.TextBox
$button = New-Object System.Windows.Forms.Button
$button.Text = "Submit"
$button.Add_Click({ $form.Close() })
$form.Controls.Add($textBox)
$form.Controls.Add($button)
$form.ShowDialog()
$input = $textBox.Text
Write-Host "You entered: $input"
In this example, a simple Windows Form is created with a text box and a button. Users can enter information in the text box, providing a more interactive form of input.
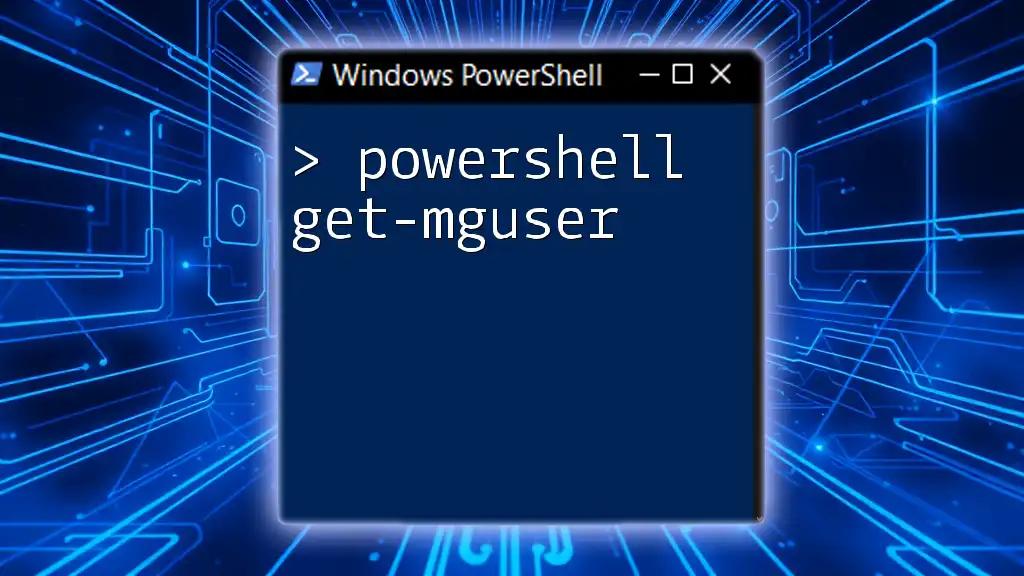
PowerShell Get User Input: Practical Use Cases
Use Case 1: Collecting Script Parameters for Automation
For automation scripts, gathering parameters is essential. You might build a script that manages system users and requires input like usernames and permissions. For example:
param (
[string]$username,
[string]$role
)
Write-Host "Assigning user $username the role of $role."
This script assigns a new role to a user, showcasing how user input can directly drive automation tasks.
Use Case 2: Interactive Script for System Administration
In a scenario where you need to restart a service based on user input, your script could look like this:
$service = Read-Host "Which service would you like to restart?"
Restart-Service -Name $service
Write-Host "Service $service has been restarted."
Here, user input directly influences administrative actions, enhancing flexibility in managing services.
Use Case 3: Data Processing with User Input
User input can also shape data-driven applications. For example, collecting search parameters from a user can refine a search operation:
$searchTerm = Read-Host "Enter the term to search for"
Get-ChildItem -Path C:\ -Recurse | Where-Object { $_.Name -match $searchTerm }
This command searches a specified directory and its subdirectories for files matching the user's input, directing the flow of data processing based on that input.

Troubleshooting Common Issues with PowerShell Input
Handling Incorrect Input Types
To ensure your scripts are robust, it's essential to handle incorrect input types efficiently. Here’s a suggestion for ensuring scripts continue to run smoothly:
do {
$age = Read-Host "Enter your age (numeric value only)"
} until ($age -match '^\d+$')
Write-Host "Thank you, your age is $age."
Using a loop, this example repeatedly prompts the user until they provide valid input.
Debugging User Input Errors
When debugging user input errors, encourage clear messaging. Providing feedback such as “Please try again,” or outlining specific input requirements can be beneficial.
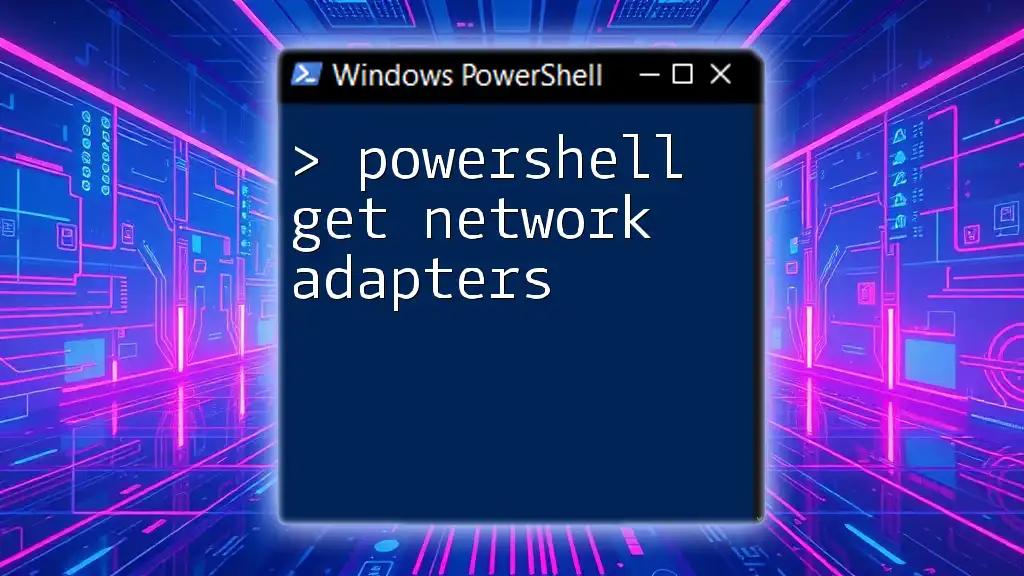
Best Practices for PowerShell User Input
To create effective scripts that utilize user input, consider these best practices:
- Clear Prompts: Ensure your input prompts are specific and clear to avoid confusion.
- User-Friendly Options: Provide guidance and examples on the expected format for input.
- Consistent Handling: Apply uniform validation and error checking techniques throughout the script to maintain consistency and reliability.
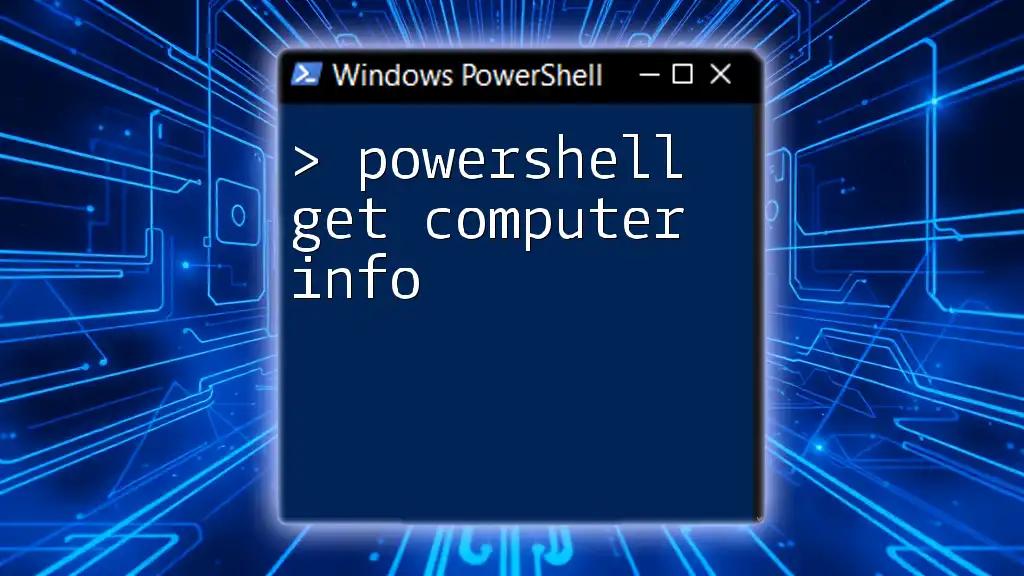
Conclusion
Incorporating user input into PowerShell scripts not only improves engagement but also adds significant versatility to your automation tasks. By mastering methods like `Read-Host`, parameter attributes, and even GUI forms, you can transform static scripts into dynamic, interactive tools.
Practicing these techniques will enhance your proficiency, and as you explore the wider capabilities of PowerShell scripting, you’ll find ever more ways to refine the user experience and deliver precise automation solutions.

Call-to-Action
Feel free to leave questions or feedback in the comments section! Join our upcoming webinars or courses on PowerShell mastery to deepen your knowledge and skills.
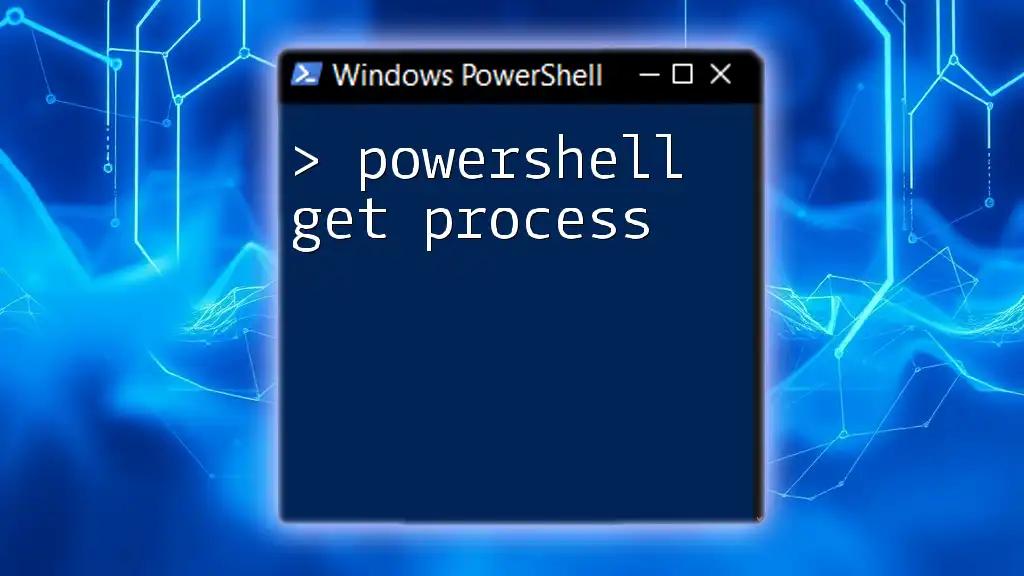
Additional Resources
For more information on PowerShell, consider visiting the official documentation and exploring recommended books or tutorials to further enhance your learning journey.