To quickly retrieve your public IP address using PowerShell, you can use the following command:
(Invoke-WebRequest -Uri 'http://api.ipify.org').Content
Understanding Public IP Addresses
What is a Public IP Address?
A public IP address is an Internet Protocol address that is assigned to your network device by your Internet Service Provider (ISP). It is used to identify your network on the internet, enabling other devices and services to communicate with you.
The key distinction between public and private IP addresses lies in their accessibility. Public IPs are routable on the internet, while private IPs, used within local networks, are not directly accessible from external sources. Understanding your public IP is essential for multiple scenarios, including:
- Configuring remote access to devices.
- Setting up servers to be reachable from the internet.
- Troubleshooting connectivity issues.
Importance of Knowing Your Public IP
Knowing your public IP is vital in several contexts. For instance, if you're hosting a web server or need to set up VPN access, you'll require your public IP to configure services correctly. Additionally, with growing concerns around online privacy, understanding your public IP can help in managing which information is shared with third parties online.
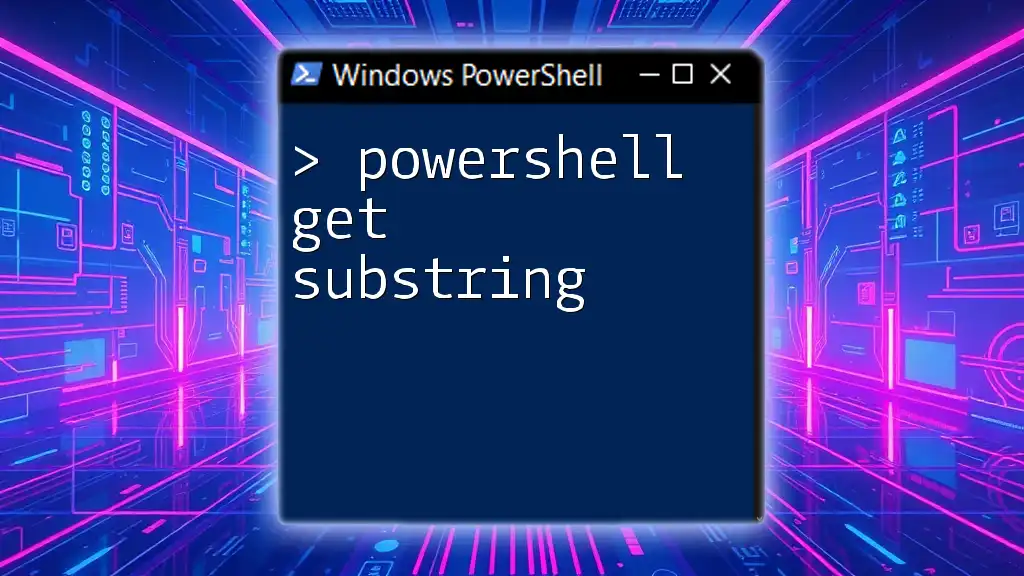
Pre-Requisites
To effectively follow along with this guide on how to powershell get public ip, ensure you have:
- A basic understanding of PowerShell commands and navigation.
- A compatible operating system installed with PowerShell (Windows, or PowerShell Core for macOS and Linux).
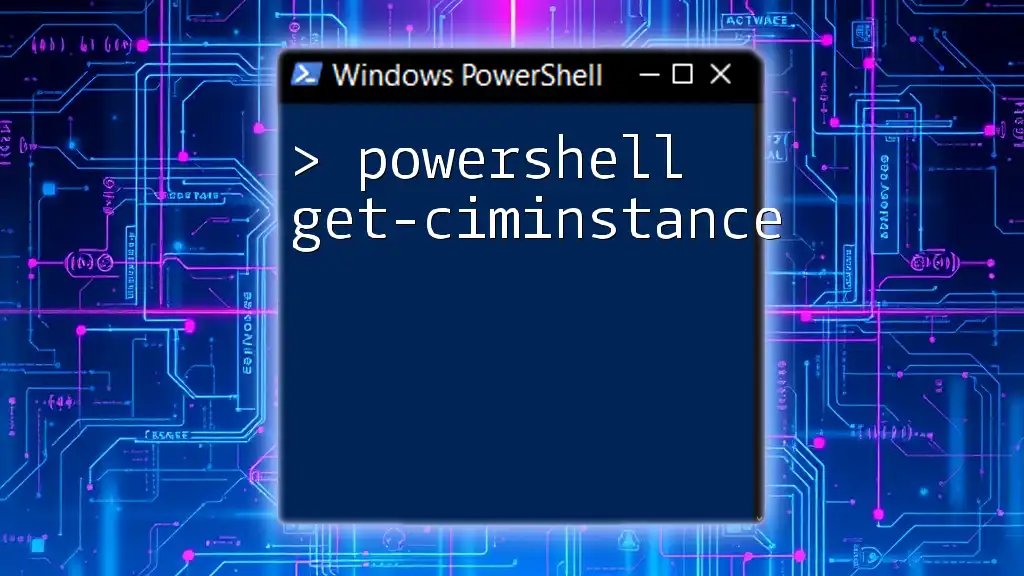
Getting Your Public IP Address Using PowerShell
Using `Invoke-RestMethod`
One of the simplest ways to fetch your public IP address in PowerShell is by using the `Invoke-RestMethod` cmdlet. This cmdlet allows you to send HTTP requests to a URL, making it a perfect fit for API calls.
Simple Command to Get Public IP
You can easily get your public IP address with the following command:
$publicIP = Invoke-RestMethod -Uri "http://api.ipify.org"
Write-Output "Your Public IP Address is: $publicIP"
In this code snippet:
- `Invoke-RestMethod` queries the API from `api.ipify.org`, which returns your public IP.
- The result is stored in the variable `$publicIP`, and the IP is printed to the console using `Write-Output`.
Using `curl` Command
`curl` is another powerful command available in PowerShell that can be used similarly to `Invoke-RestMethod`.
Fetching Public IP with `curl`
Here's how you can retrieve your public IP using `curl`:
curl http://api.ipify.org
While both `Invoke-RestMethod` and `curl` serve the same purpose, you might prefer `curl` for its flexibility and lightweight nature in certain scenarios, especially in scripts where simplicity is essential.
Using External APIs for IP Address Lookup
Multiple APIs can be utilized to obtain your public IP address, providing different features and information beyond just the IP.
Sample API Command
A popular alternative API is `ipinfo.io`. To use it, execute:
$publicIP = Invoke-RestMethod -Uri "https://ipinfo.io/ip"
Write-Output "Your Public IP Address is: $publicIP"
This command works similarly to the previous examples, but it leverages a different API, offering you flexibility in choosing the service that best fits your needs.
Error Handling and Troubleshooting
When working with external services, errors can occur due to network issues or API downtime. It's essential to have proper error handling in automation scripts.
Common Issues When Fetching Public IP
Some of the typical errors might include:
- The inability to connect to the API endpoint.
- Getting an empty response if the API is down.
How to Implement Error Handling
In PowerShell, you can use a try-catch block to handle errors gracefully:
try {
$publicIP = Invoke-RestMethod -Uri "http://api.ipify.org"
Write-Output "Your Public IP Address is: $publicIP"
} catch {
Write-Output "Error: Unable to fetch your public IP address."
}
This code ensures that if an error occurs while trying to fetch the public IP, an informative message will be displayed instead of the script failing unexpectedly.
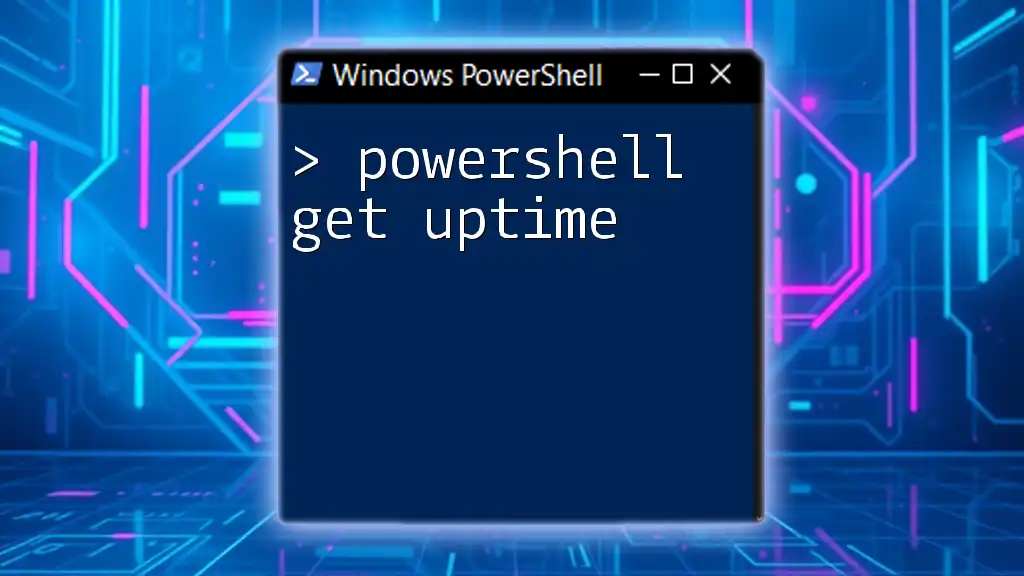
Advanced Techniques
Saving the Public IP Address to a File
In certain situations, you might want to keep a record of your public IP address. This could be useful for monitoring or documentation purposes.
Why Save IP Address?
Saving your public IP can assist you in keeping track of any changes over time, which might be essential for security or when configuring remote access.
Code Example to Save Public IP
Here's how you can save your obtained public IP to a text file:
$publicIP = Invoke-RestMethod -Uri "http://api.ipify.org"
Out-File -FilePath "public_ip.txt" -InputObject $publicIP
Write-Output "Public IP saved to public_ip.txt"
In this snippet, the command `Out-File` writes the public IP stored in `$publicIP` to a file named `public_ip.txt`, providing you with a log of your external IP.
Automating Public IP Retrieval
If you need to check your public IP periodically, automating the process through scheduled tasks can save time and ensure you always have the latest information.
Scheduled Tasks
To automate retrieval, you can set up Windows Task Scheduler to run your PowerShell script at specified intervals.
Sample Script for Automation
You can use this PowerShell script to gather the public IP and save it:
$publicIP = Invoke-RestMethod -Uri "http://api.ipify.org"
Out-File -FilePath "public_ip.txt" -InputObject $publicIP
Create a scheduled task that runs this script every day or week, depending on your needs.
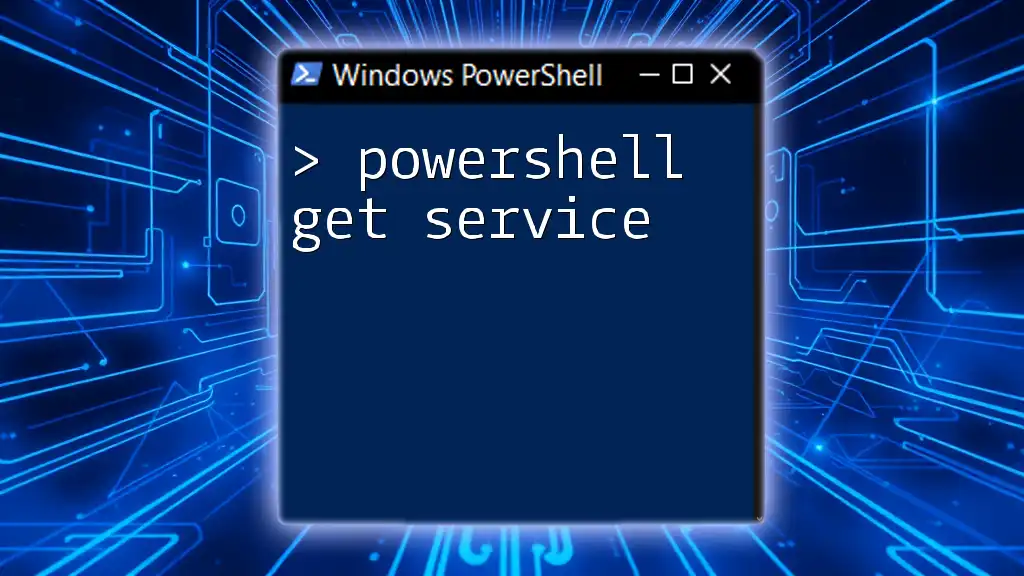
Conclusion
In summary, we've explored how to retrieve your public IP address using PowerShell effectively. From utilizing `Invoke-RestMethod` and `curl` to interfacing with various APIs, you now have the tools to manage your public IP with confidence.
Remember, experimenting with these commands will deepen your understanding and enhance your networking skills. Don't hesitate to explore further and apply what you've learned to your personal projects or in professional settings.
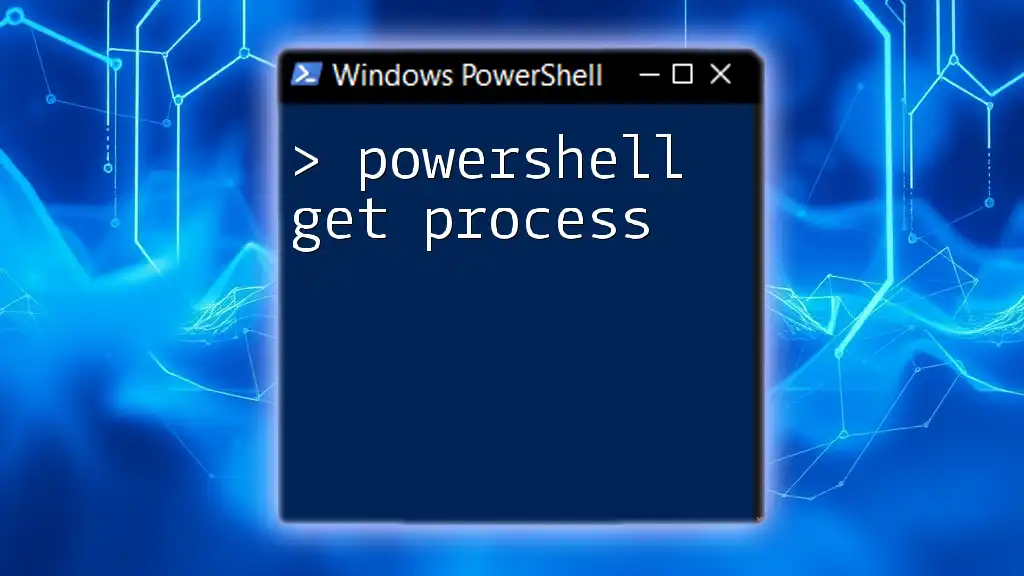
Additional Resources
For those looking to expand their PowerShell knowledge further, consider diving into the official PowerShell documentation or tutorials covering topics related to networking diagnostics and automation.
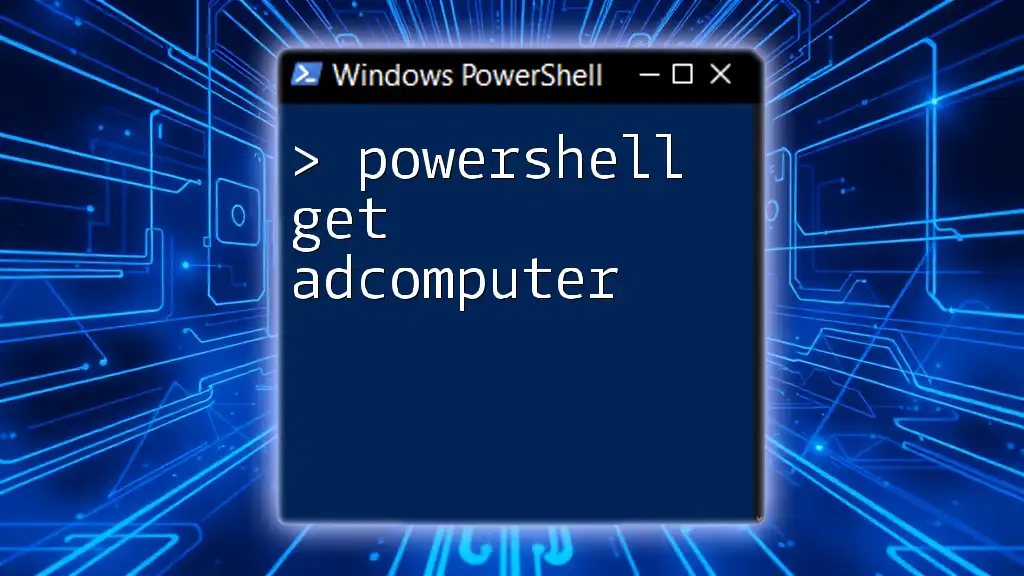
Frequently Asked Questions (FAQ)
What if I can't connect to the API?
If you cannot connect, first check your internet connection. Ensure that the API service you're trying to use is up and running. You may also want to try using an alternative API.
Are there limitations to using public IP APIs?
Yes, many free APIs come with usage limits, restricting the number of requests you can make within a given timeframe. For higher usage, consider paid services.
Can I get the local IP address using PowerShell?
Yes, you can retrieve local IP addresses by using the `Get-NetIPAddress` cmdlet, which provides various networking information for your system.