In PowerShell, "args" refers to the automatic variable `$args`, which stores any additional arguments passed to a script or function when it's invoked.
Here's a code snippet demonstrating how to use `$args` in a simple script:
# Save this as script.ps1
Write-Host "You passed the following arguments:"
foreach ($arg in $args) {
Write-Host $arg
}
To run the script with arguments, use:
.\script.ps1 "Hello" "World" "from" "PowerShell"
Understanding PowerShell `$args`
In PowerShell, `$args` is an automatic variable that stores command-line arguments passed to a script or function. Its versatility makes it essential for writing scripts that can handle flexible input, allowing users to provide various data without hard-coding those values into the script. Understanding how to effectively use `$args` is crucial for making your scripts more dynamic and user-friendly.
How to Use `$args` in PowerShell
Basic Syntax of `$args`
To start, let’s explore the basic syntax. The `$args` array can be accessed directly in your script. For example, when you define parameters within a script, you can utilize `$args` to retrieve those values:
# Example Script
Write-Host "First Argument: $($args[0])"
Write-Host "Second Argument: $($args[1])"
In this script, if you were to run it with two arguments, it would output the first and second arguments you provide.
Passing Arguments to a PowerShell Script
To pass arguments to your PowerShell script, you simply invoke it from the command line and include your desired parameters. Here's how:
.\MyScript.ps1 "Hello" "World"
In this example, `"Hello"` will be stored in `$args[0]`, and `"World"` in `$args[1]`.
Accessing and Using Elements from `$args`
Inside your script, you can easily access each element of the `$args` array using indexing. For instance, check if any arguments were passed, and if so, display the first one:
if ($args.Count -gt 0) {
Write-Host "The first argument is: $($args[0])"
}
This conditional check ensures that you only attempt to access `$args[0]` if there’s at least one argument in the array. This is a good way to prevent runtime errors.
Iterating Over `$args`
Another powerful feature of `$args` is the ability to iterate over all passed arguments. Using a `foreach` loop allows you to process each item in the `$args` array easily:
foreach ($arg in $args) {
Write-Host "Argument: $arg"
}
This loop will print each argument provided to the script, making it handy for processing multiple inputs at once.

Practical Examples of Using `$args`
Example 1: Concatenating Strings
You can leverage `$args` to create a simple string concatenation utility. The code below concatenates all provided arguments into a single string:
$result = ""
foreach ($arg in $args) {
$result += $arg + " "
}
Write-Host "Concatenated String: $result"
This script will take any number of arguments and print a single string containing all of them, separated by spaces.
Example 2: File Processing Script
A common use case for `$args` is processing files. With this script, you can supply filenames as arguments and read their contents:
foreach ($file in $args) {
if (Test-Path $file) {
Get-Content $file
} else {
Write-Host "$file does not exist."
}
}
Here, each file name you provide will be checked for existence, and if found, its content will be displayed. This demonstrates how `$args` enhances your scripts’ capabilities to accept dynamic input.
Example 3: Simple Arithmetic Calculation
Another example is a simple arithmetic calculator that sums numeric inputs. Here’s how it can be done with `$args`:
$sum = 0
foreach ($num in $args) {
$sum += [int]$num
}
Write-Host "Total Sum: $sum"
In this case, the script converts each argument to an integer and accumulates the total, showcasing how `$args` can be used for more complex calculations based on user input.

Best Practices when Using `$args`
When working with `$args`, it's crucial to adopt best practices for clarity and usability:
- Keep it clear and concise: Always ensure your scripts have clear argument names when using `$args` for better maintainability.
- Validate Input: Implement checks to confirm that you have received the expected number of arguments, which can prevent runtime errors and provide a better user experience.
- Use Comments: Clearly document what each argument does. This will help anyone who reads or maintains your script in the future.

Common Errors and Troubleshooting
As with any programming task, you may encounter errors when using `$args`. Some common issues include:
- Accessing Non-Existent Arguments: Always check the count of `$args` before attempting to access it. For example:
if ($args.Count -eq 0) {
Write-Host "No arguments provided. Please provide arguments."
exit
}
This error handling checks if any arguments are present. If not, it gracefully informs the user and exits the script.
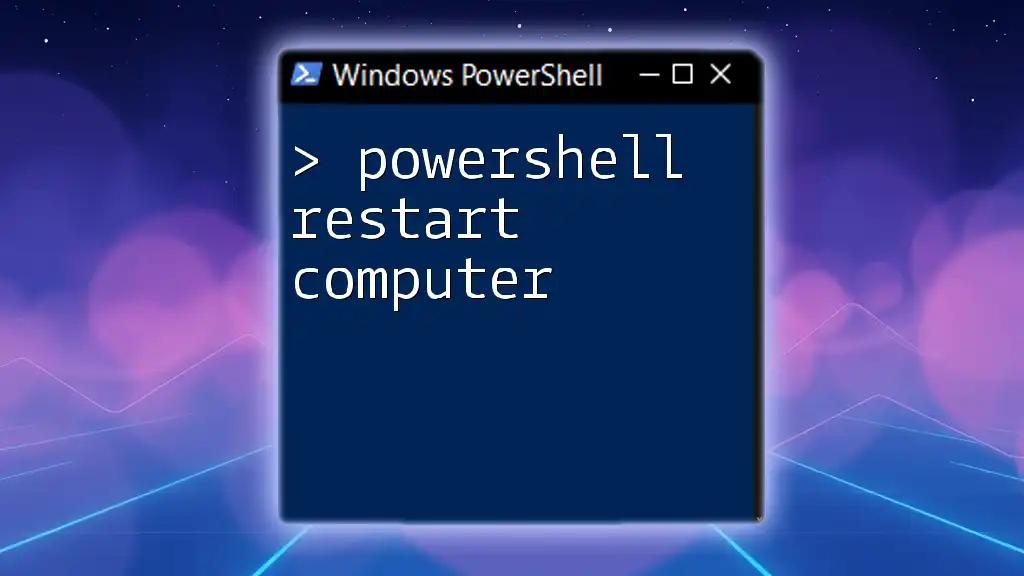
Summary
In conclusion, mastering PowerShell `$args` significantly enhances your scripting capabilities, allowing for flexible and dynamic input. By practicing the techniques discussed here and implementing the best practices, you'll be well on your way to creating more robust and user-friendly PowerShell scripts.
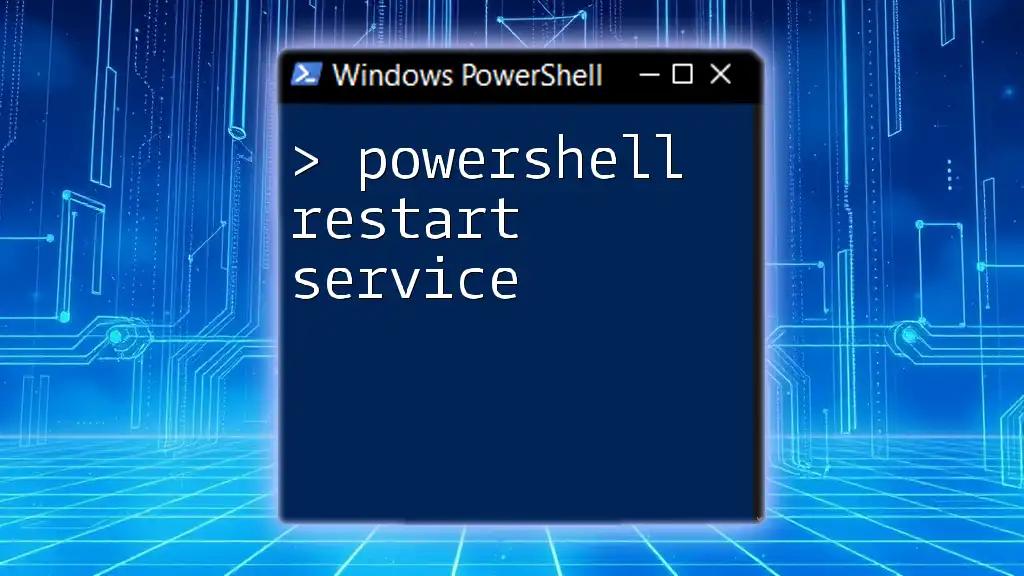
Additional Resources
For further learning, you can refer to the official PowerShell documentation and explore advanced scripting techniques that can broaden your knowledge and skills.
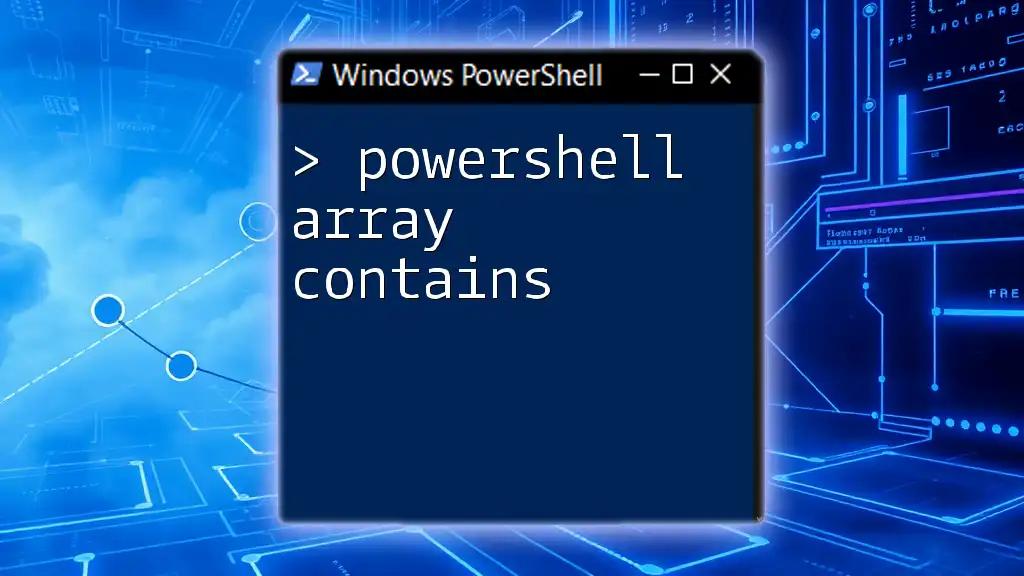
Call to Action
We invite you to participate in the conversation! Feel free to leave your questions or comments below, and don’t forget to sign up for our newsletter for more tips and tutorials on PowerShell.