PowerShell REST API allows you to interact with web services by sending HTTP requests and handling responses directly within your PowerShell scripts.
Here’s a simple example of a PowerShell command that sends a GET request to a REST API:
$response = Invoke-RestMethod -Uri 'https://api.example.com/data' -Method Get
Write-Host $response
Understanding REST APIs
What is a REST API?
REST, or Representational State Transfer, is an architectural style that defines a set of constraints for building web services. REST APIs are APIs that adhere to these constraints, enabling systems to communicate over HTTP requests. Key principles include:
- Statelessness: Each request from the client to the server must contain all the information needed to understand and process the request. This eliminates the need for maintaining session information on the server.
- Resource-based structure: REST uses resources (identified by URIs) that can be manipulated using standard HTTP methods (GET, POST, PUT, DELETE).
- Client-server architecture: The client and server operate independently, allowing for separation of concerns.
How REST APIs Work
The interaction with REST APIs follows a request-response cycle. When a client sends a request (often in JSON format), the server processes it and sends back a response. This response usually includes a status code, indicating the success or failure of the request.
Common HTTP response statuses include:
- 200 OK: The request succeeded.
- 404 Not Found: The requested resource does not exist.
- 500 Internal Server Error: There was a server error.
JSON is a favored data format because it's lightweight and easy to parse, making it ideal for web applications.

Setting Up PowerShell for REST API Interaction
Requirements
To begin using PowerShell to interact with REST APIs, ensure you have PowerShell version 5.1 or later installed. You might also need to install specific modules, though basic API calls can be performed with built-in cmdlets like `Invoke-RestMethod`. Make sure your system has internet connectivity, as most APIs you will work with are cloud-based.
Basic PowerShell Cmdlets for API Calls
PowerShell offers a couple of powerful cmdlets for executing REST API calls:
- `Invoke-RestMethod`: This cmdlet is ideal for working with REST APIs as it parses JSON responses automatically into PowerShell objects.
- `Invoke-WebRequest`: This cmdlet can perform REST API calls in a more general way and is useful for more complex requirements, such as handling HTML responses or file downloads.
Understanding when to use each cmdlet is essential; `Invoke-RestMethod` simplifies working with APIs due to its built-in functionality for handling common response types.
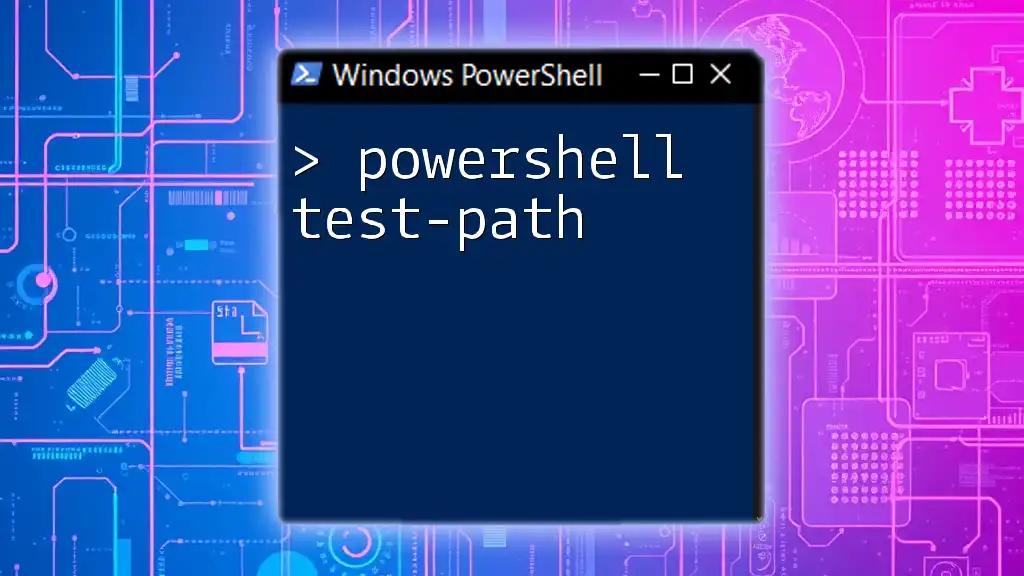
Making Your First API Call
Crafting a GET Request
A GET request is used to retrieve data from a server. The following example showcases how to perform a simple GET request to a public API that returns placeholder posts:
$response = Invoke-RestMethod -Uri "https://jsonplaceholder.typicode.com/posts" -Method Get
$response
In this example, we query a placeholder API, and the response is automatically converted into a PowerShell object, which you can easily work with.
Making a POST Request
Understanding POST Requests
A POST request is typically used to submit data to a server, which then processes that data. It's crucial when you want to create new entries or submit forms.
Example of a POST Request
When using a POST request with JSON data, ensure your body is formatted correctly:
$body = @{ title = "foo"; body = "bar"; userId = 1 } | ConvertTo-Json
$response = Invoke-RestMethod -Uri "https://jsonplaceholder.typicode.com/posts" -Method Post -Body $body -ContentType "application/json"
$response
In this example, we’re sending a JSON object with a title, body, and user ID. The response will include information about the newly created post, and it can be printed out in the console. The `-ContentType` parameter is essential here as it specifies the media type of the resource.
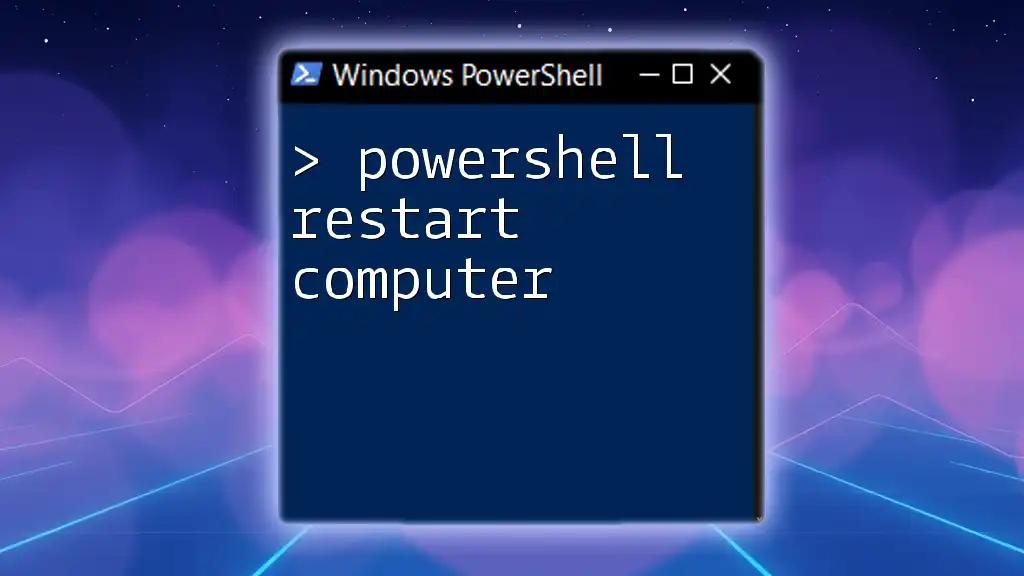
Handling API Responses
Reading JSON Responses
When you receive a JSON response, PowerShell converts it into an object that can be navigated easily. For example, to access the title of the first post in the response:
$post = $response[0]
"Title: $($post.title)"
Using `$(...)` enables you to interpolate the property directly into the string output.
Error Handling
Handling errors effectively is crucial when working with REST APIs. You can use the `Try` and `Catch` construct to capture exceptions. A simple example would look like this:
try {
$response = Invoke-RestMethod -Uri "https://api.example.com/endpoint" -Method Get
} catch {
Write-Host "An error occurred: $_"
}
This code attempts to make an API call, and if it fails, it prints an error message to the console.
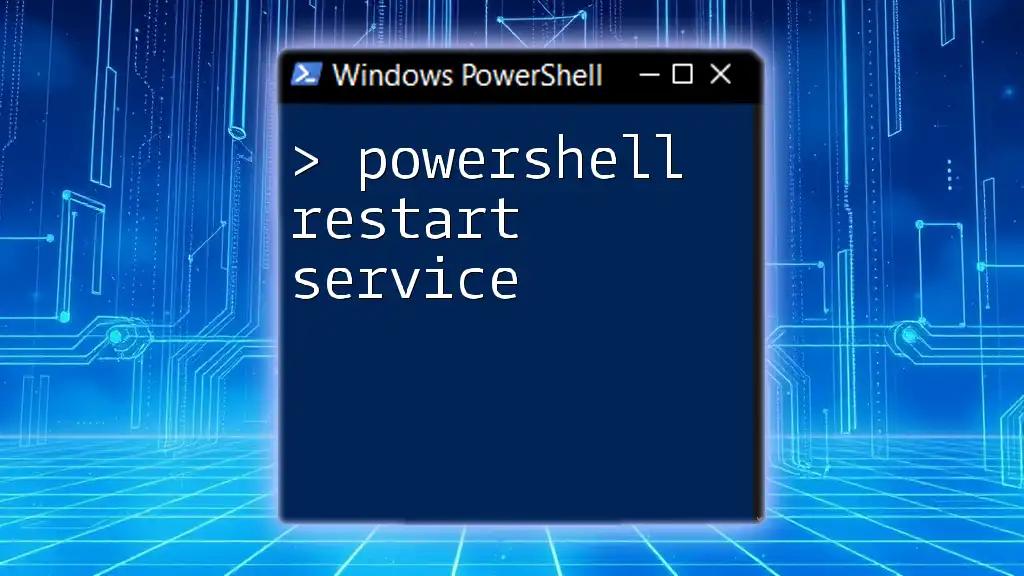
Authentication with REST APIs
Types of Authentication
Basic Authentication
For APIs that require user credentials, basic authentication is a straightforward method. To use it in PowerShell, you first encode your credentials in base64:
$username = "your_username"
$password = "your_password"
$base64AuthInfo = [Convert]::ToBase64String([Text.Encoding]::ASCII.GetBytes(("${username}:${password}")))
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Headers @{Authorization=("Basic {0}" -f $base64AuthInfo)} -Method Get
This approach sends the username and password in the headers, allowing the API to authenticate your request.
Token-Based Authentication
Many APIs now favor token-based authentication methods, such as OAuth. Typically, you first obtain a token and then use it in your API calls like this:
$tokenResponse = Invoke-RestMethod -Uri "https://api.example.com/auth" -Method Post -Body $body -ContentType "application/json"
$token = $tokenResponse.token
Subsequent requests can then incorporate this token into the headers for authentication.
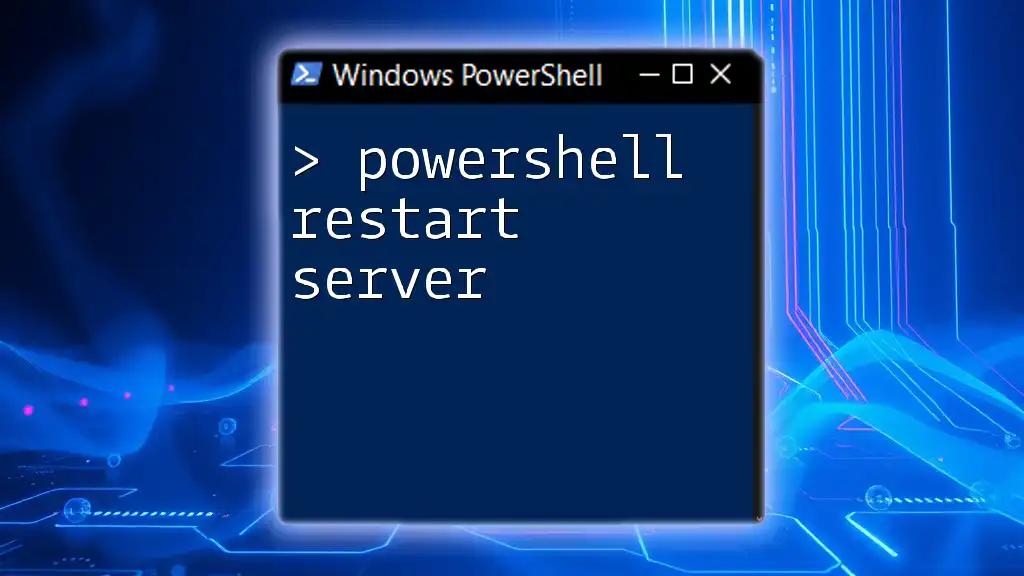
Advanced PowerShell Techniques for REST API
Using Headers and Parameters
Some APIs require custom headers for things like API keys or specific attributes. This can be easily managed in PowerShell:
$response = Invoke-RestMethod -Uri "https://api.example.com/resource" -Headers @{ "Custom-Header" = "value" } -Method Get
Passing headers as a dictionary object allows for more flexibility and customization in your requests.
Working with Different Content Types
APIs vary in supported content types. While JSON is common, you may encounter `application/xml` or others. PowerShell can handle this variety, and you can specify the content type when sending requests as needed.
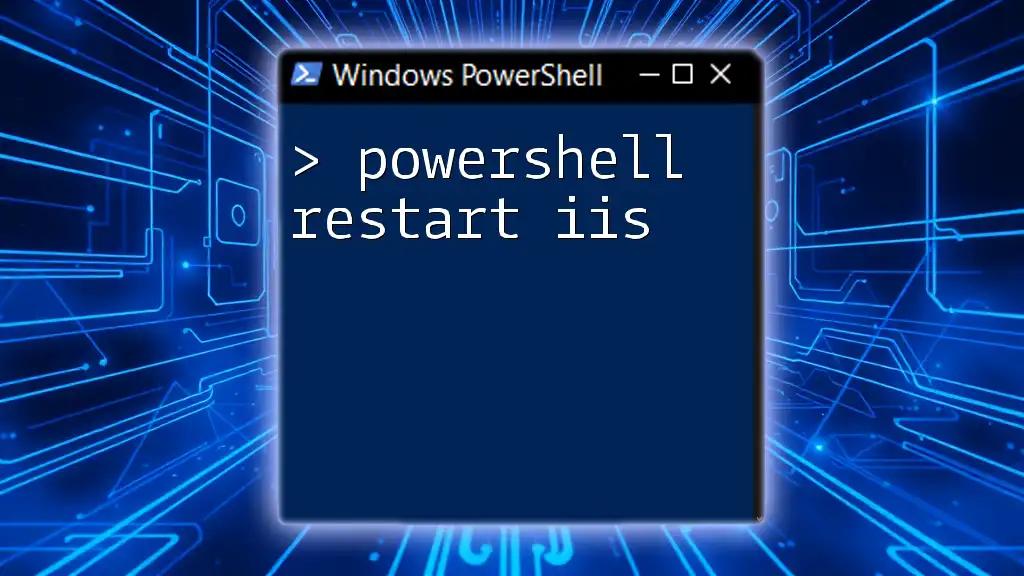
Commonly Used REST APIs with PowerShell
Examples of Popular APIs
Several public APIs can prove useful for practice:
- GitHub API: Interact with repositories, user information, and more.
- Twitter API: Access tweets, user data, and trends.
- Weather APIs (e.g., OpenWeatherMap): Fetch weather data based on location.
Engaging with these APIs through PowerShell will bolster your understanding of making REST API calls.
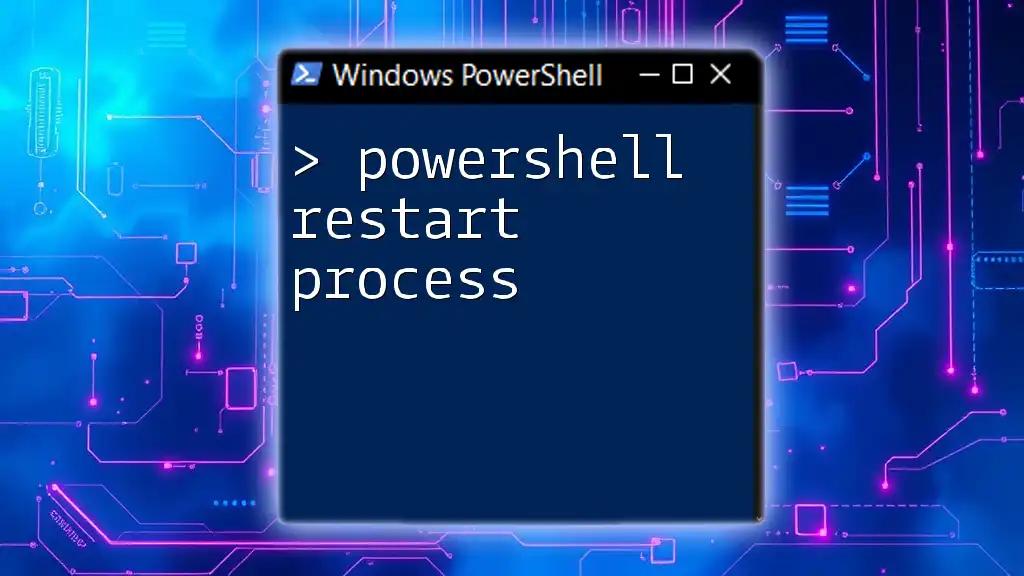
Troubleshooting Common Issues
Debugging API Calls
When troubleshooting requests, ensure that the endpoint and payload are accurate. Review API documentation for precise requirements regarding URL structures and payload formats.
Helpful Tips for Efficient PowerShell Scripting
To improve the maintainability of your PowerShell scripts when working with APIs, consider encapsulating requests in functions. Reusable functions streamline your code and enhance readability. For example:
function Invoke-ApiGet {
param (
[string]$uri,
[hashtable]$headers
)
return Invoke-RestMethod -Uri $uri -Headers $headers -Method Get
}
This `Invoke-ApiGet` function condenses your logic, making it simpler to call the GET method across different endpoints.
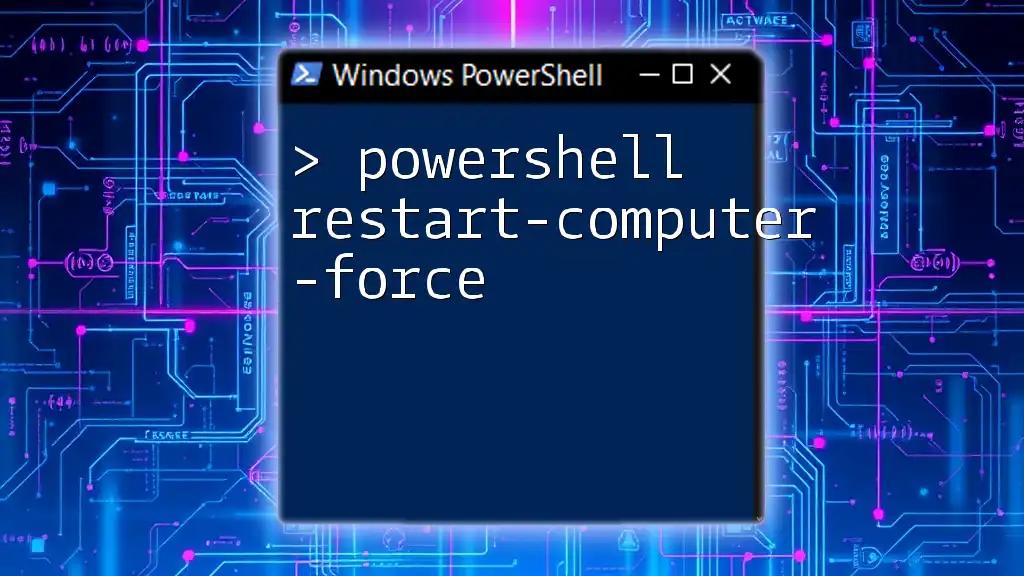
Conclusion
By grasping how to utilize PowerShell REST APIs, you’ll be equipped to interact with numerous web services effectively. Practice is critical; experiment with real-world APIs to consolidate your learning. The journey doesn’t stop here—plenty of resources are available to help you dive deeper into PowerShell and REST APIs, from official documentation to community forums. Continue exploring, and you'll discover the endless possibilities these technologies offer.
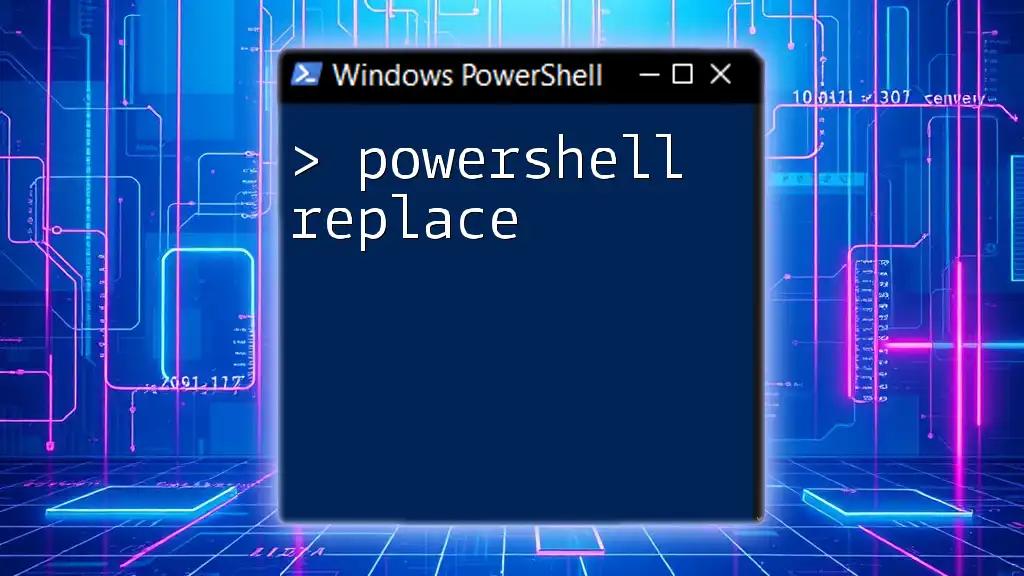
Additional Resources
For further exploration of PowerShell and REST API interactions, consult the official PowerShell documentation and consider engaging with community resources, forums, and online courses specifically focused on web services and PowerShell scripting.