In this post, you'll learn how to use PowerShell to interact with a REST API by sending a GET request to retrieve data from a web service.
# Example of a PowerShell script to send a GET request to a REST API
$response = Invoke-RestMethod -Uri 'https://api.example.com/data' -Method Get
Write-Host $response
Understanding REST APIs
What is a REST API?
A REST API (Representational State Transfer Application Programming Interface) is a set of rules and conventions for building and interacting with web services. It allows different software applications to communicate over the internet using standard HTTP methods. Key characteristics of REST APIs include:
- Statelessness: Each API call contains all the information the server needs to fulfill that request, making it independent of earlier requests.
- Resources: In REST, data is represented as resources, often using URLs to access them.
- Representations: Resources can be represented in multiple formats, usually JSON or XML, which can be easily parsed by applications.
Common HTTP Methods Used in REST APIs
Understanding the different HTTP methods used in REST APIs is vital as they dictate the type of action being performed:
- GET: Retrieves data from a server.
- POST: Sends data to create a new resource.
- PUT: Updates an existing resource with new data.
- DELETE: Removes a resource from the server.
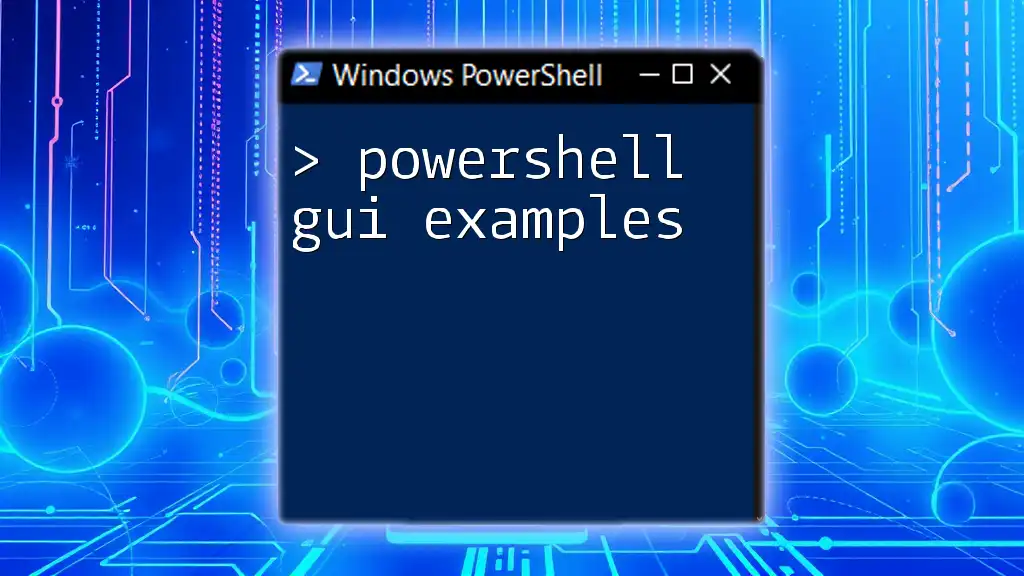
Setting Up Your Environment
Prerequisites for Using PowerShell with REST APIs
Before working with PowerShell REST API examples, ensure you have either Windows PowerShell or PowerShell Core installed on your system. Familiarity with basic PowerShell commands will make the learning process smoother.
Installing Required Modules
PowerShell comes with built-in cmdlets like Invoke-RestMethod and Invoke-WebRequest. While these are often pre-installed, you can check their availability by typing:
Get-Command Invoke-RestMethod
Get-Command Invoke-WebRequest
If you need additional functionality, consider installing specific modules from the PowerShell Gallery.
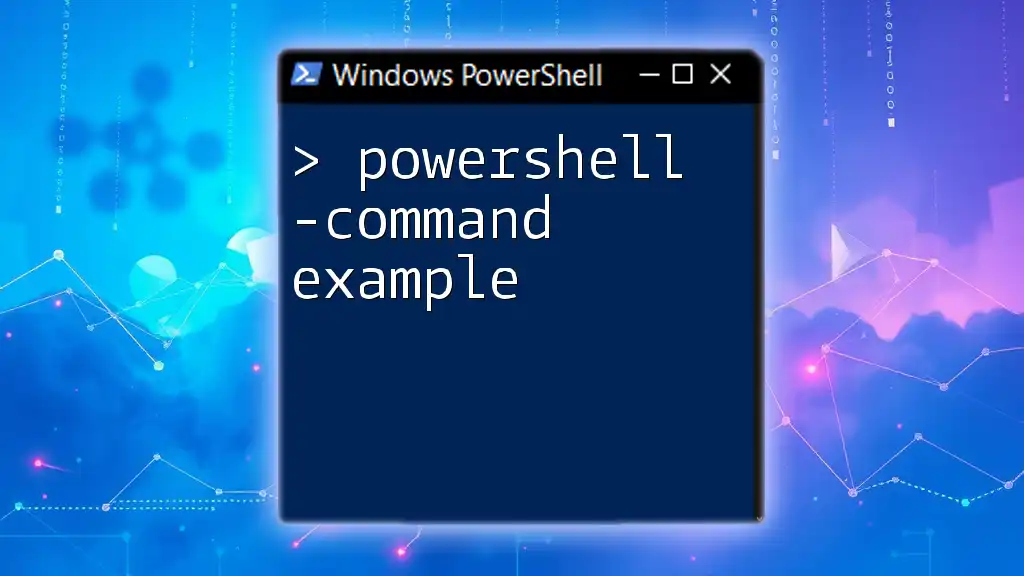
Making Basic REST API Calls with PowerShell
Using Invoke-RestMethod
Making a GET Request
The most straightforward way to begin is with a GET request using the `Invoke-RestMethod` cmdlet. Here’s an example of how to retrieve data:
$url = "https://api.example.com/data"
$response = Invoke-RestMethod -Uri $url -Method Get
$response
In this code snippet, we define the URL of the API endpoint. The response will contain the data fetched from the server, often formatted in JSON.
Making a POST Request
To create resources, you can use a POST request. Here is how to send data:
$url = "https://api.example.com/data"
$body = @{ name = "John Doe"; email = "john@example.com" } | ConvertTo-Json
$response = Invoke-RestMethod -Uri $url -Method Post -Body $body -ContentType "application/json"
$response
In this example, we're constructing a PowerShell hashtable, converting it to JSON, and sending it as the body of our POST request. The server's response will typically provide confirmation or the created resource's details.
Handling Different Content Types
Working with JSON Data
Working with JSON data is common in web APIs. When you receive data from a REST API response, it usually comes in JSON format. You can convert PowerShell objects into JSON format using:
$jsonData = $response | ConvertTo-Json
Working with XML Data
Some APIs may use XML instead of JSON. Here’s how you can send and receive XML data:
$url = "https://api.example.com/xml"
$xmlBody = "<user><name>John Doe</name><email>john@example.com</email></user>"
$response = Invoke-RestMethod -Uri $url -Method Post -Body $xmlBody -ContentType "application/xml"
$response
In this case, the body is an XML representation of the data we wish to send.
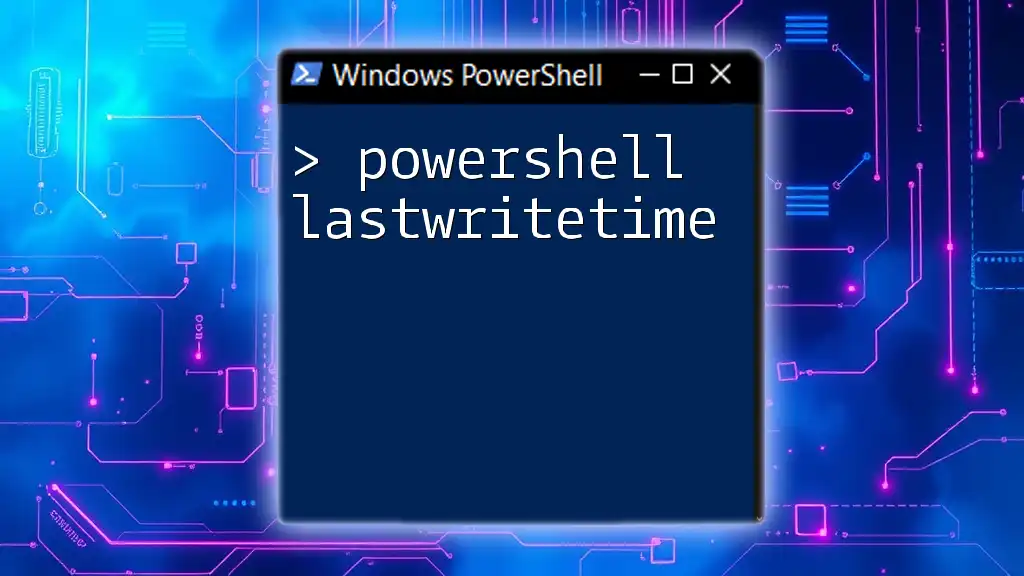
Authentication with REST APIs
Types of Authentication
API Key Authentication
Many APIs require an API key for access. Here’s a simple example of how to include an API key in a GET request:
$url = "https://api.example.com/data?apiKey=your_api_key"
$response = Invoke-RestMethod -Uri $url -Method Get
Make sure to replace `your_api_key` with the actual key provided by the API service.
OAuth 2.0 Authentication
Some APIs utilize OAuth 2.0 for secure access, which typically involves multiple steps:
- Obtain an access token by sending your credentials to a token endpoint.
- Use the access token as a Bearer token in the authorization header for subsequent API calls.
While the implementation can vary by service, understanding these principles is essential for working with secure APIs.
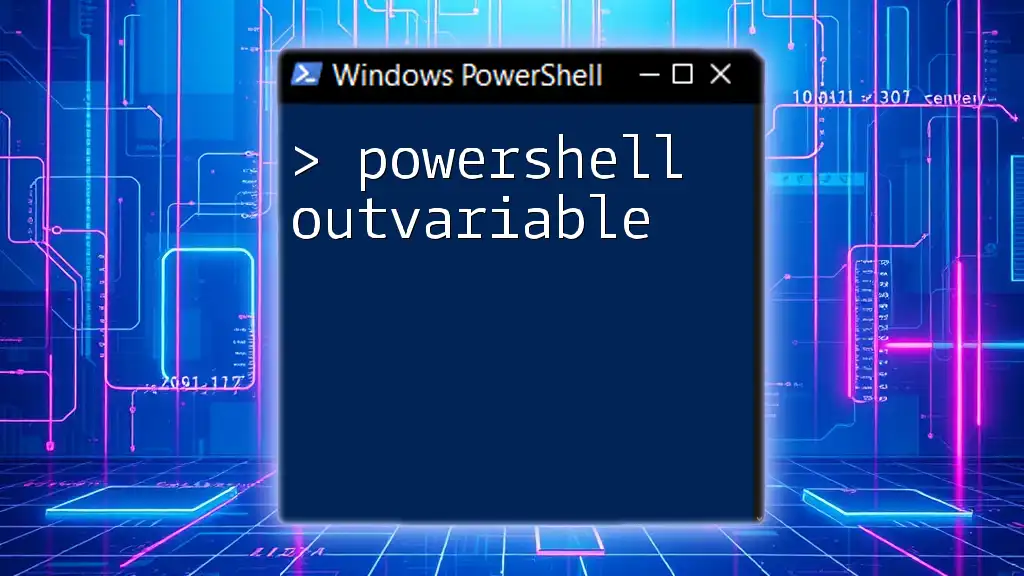
Error Handling in PowerShell API Calls
Understanding Common HTTP Status Codes
Effective error handling is crucial when interacting with REST APIs. Here's a quick overview of significant HTTP status codes you might encounter:
- 200 OK: The request has succeeded.
- 400 Bad Request: The server could not understand the request due to invalid syntax.
- 401 Unauthorized: Authentication is required and has failed or has not yet been provided.
- 404 Not Found: The server could not find the requested resource.
- 500 Internal Server Error: The server has encountered an error.
Implementing Try/Catch for Error Handling
In PowerShell, you can manage errors using try/catch blocks:
try {
$response = Invoke-RestMethod -Uri $url -Method Get
Write-Output $response
} catch {
Write-Error "Error occurred: $_"
}
This will help you catch and handle errors gracefully without crashing your script.
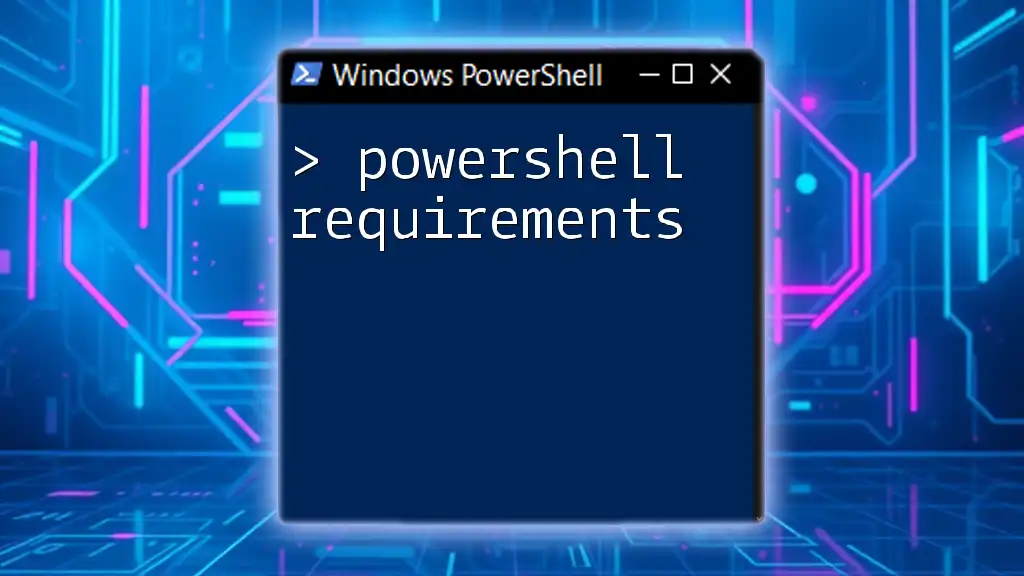
Advanced Usage of PowerShell with REST APIs
Scripting REST API Interactions
For automating tasks, you can script API interactions. For instance, you could loop through a list of resource IDs and perform API calls for each one:
$ids = @("1", "2", "3")
foreach ($id in $ids) {
$response = Invoke-RestMethod -Uri "https://api.example.com/data/$id" -Method Get
Write-Output $response
}
This approach streamlines interactions with multiple resources.
Creating PowerShell Modules for REST API
To encapsulate API functionality, consider creating a PowerShell module. This allows you to bundle related functions and maintain cleaner, more reusable code.
Example Module Structure
- Create a folder for your module.
- Create a `.psm1` file and define your functions.
- Export functions that should be accessible to others.
Using modules promotes modular design and makes managing complex scripts simpler.
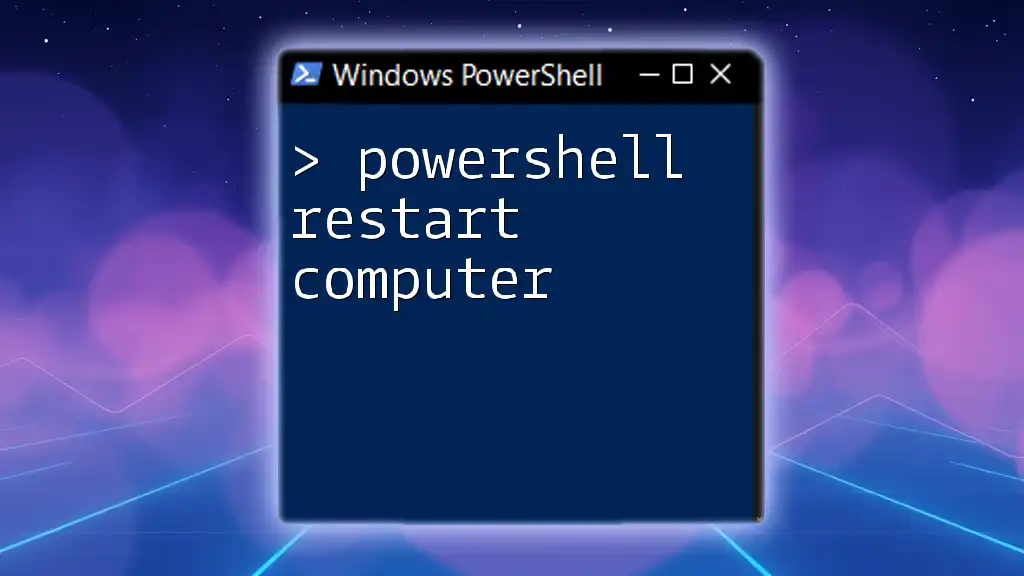
Conclusion
Throughout this article, we've explored various aspects of working with PowerShell REST API examples. From setting up your environment to making GET and POST requests, handling authentication, managing errors, and advanced scripting techniques, PowerShell equips users with powerful tools to interact with RESTful services.
Engaging with REST APIs can enhance your ability to automate tasks and integrate services efficiently. Practice these examples to deepen your understanding, and don't hesitate to seek out resources or community forums for further learning.
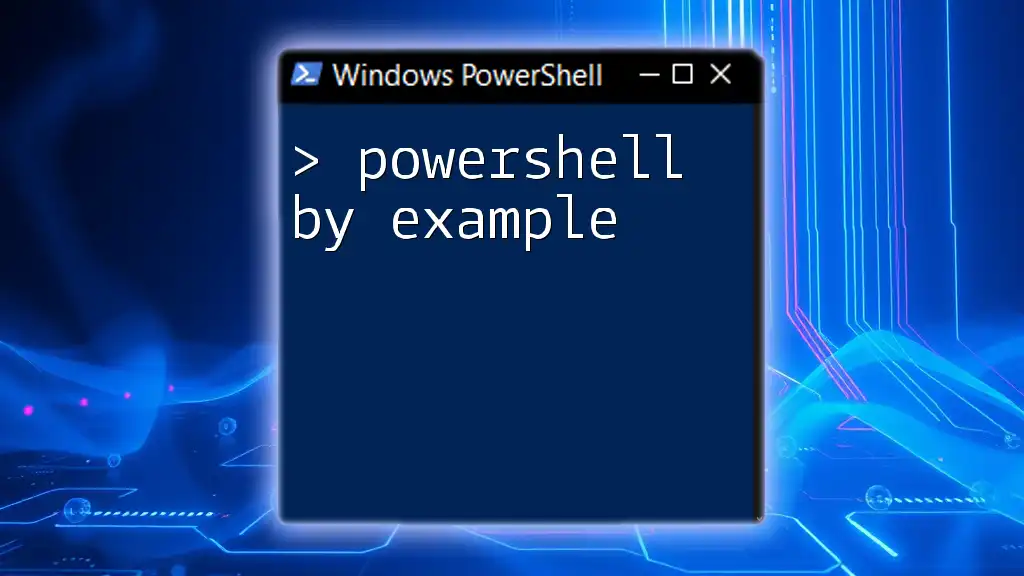
Call to Action
Share your experiences or questions in the comments below. If you're interested in expanding your skills, consider enrolling in our specialized courses or workshops designed to give hands-on experience with PowerShell and REST APIs.