PowerShell GUI examples demonstrate how to create simple graphical user interfaces for scripts, enabling users to interact with tools in a more visual manner.
# Create a simple GUI with a button that shows a message box when clicked
Add-Type -AssemblyName System.Windows.Forms
$form = New-Object System.Windows.Forms.Form
$form.Text = 'Hello PowerShell GUI'
$form.Size = New-Object System.Drawing.Size(300,200)
$button = New-Object System.Windows.Forms.Button
$button.Text = 'Click Me!'
$button.Location = New-Object System.Drawing.Point(100,70)
$button.Add_Click({ [System.Windows.Forms.MessageBox]::Show('Hello, World!') })
$form.Controls.Add($button)
$form.ShowDialog()
Understanding PowerShell GUIs
What is a GUI in PowerShell?
A Graphical User Interface (GUI) provides a visual way to interact with programs and their features. In PowerShell, GUIs allow users to create applications that utilize the power of scripts while being user-friendly, enabling those who may not be as familiar with command-line interfaces to engage with automation tools effectively.
Common GUI Frameworks for PowerShell
-
Windows Forms: This is a versatile framework for building Windows-based applications. It offers a straightforward method to creating forms and user controls, making it ideal for simpler applications.
-
Windows Presentation Foundation (WPF): WPF is more advanced and provides greater flexibility in designing rich user interfaces. It supports binding data to UI elements, creating animations, and more sophisticated layouts. Use WPF when you require a more modern look or extensive UI elements.
-
Custom Controls in PowerShell: Custom controls can significantly enhance the user experience. They allow developers to encapsulate functionality and provide reusable components tailored to specific needs.
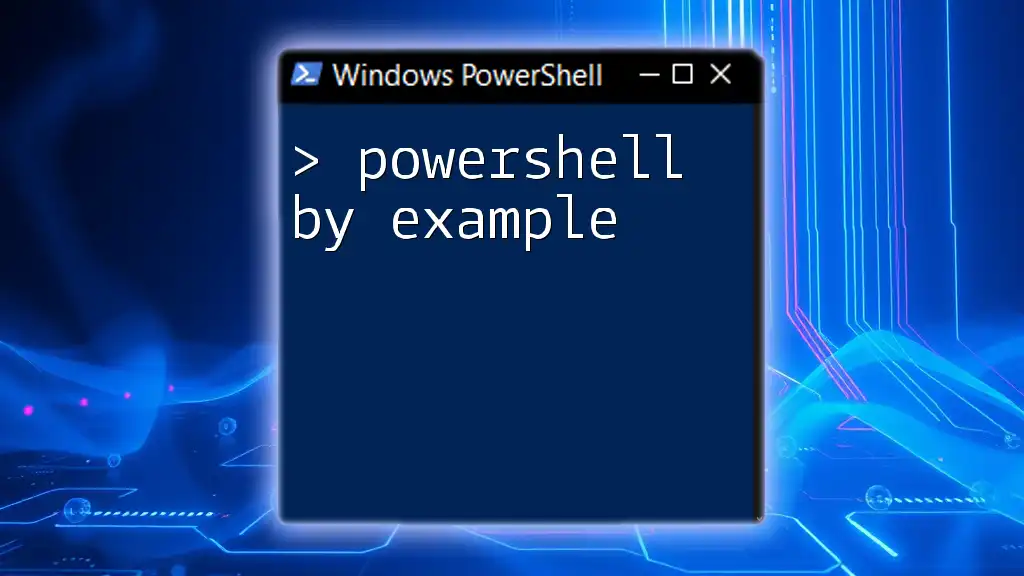
Getting Started with PowerShell GUIs
Setting Up Your Environment
Before diving into GUI development, ensure that you have the latest version of PowerShell installed, preferably PowerShell 5.1 or later, as these versions come with better GUI support. You may also require additional modules like `WindowsForms` if you are working with Windows Forms.
Basic Elements of a PowerShell GUI
Essential components of a GUI include:
- Buttons: Often used to trigger actions or events.
- Text Boxes: For input or display of data.
- Labels: Used to display static text that helps inform the user about input requirements.
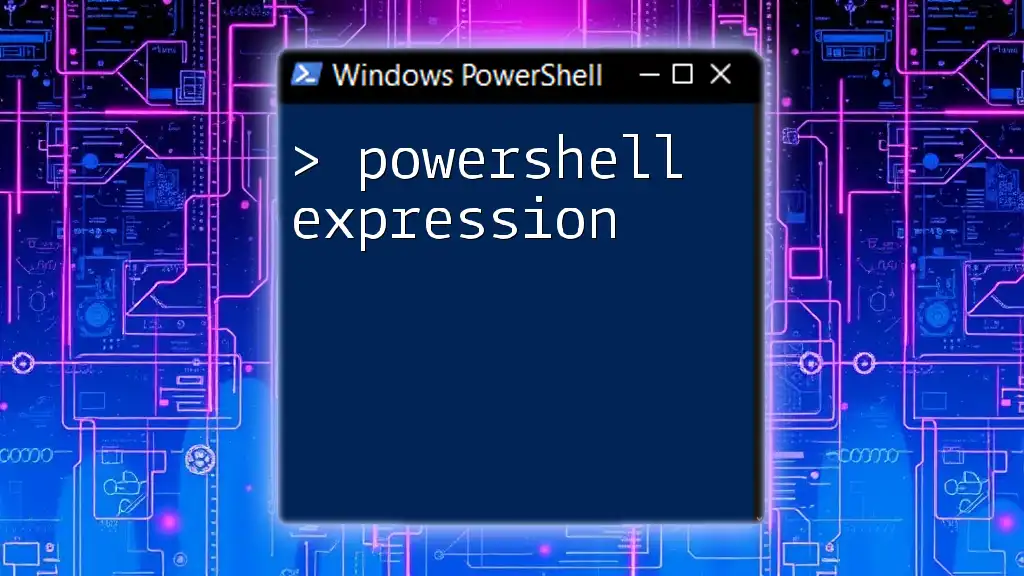
Creating a Simple PowerShell GUI
Step-by-Step Guide to Build a Simple Form
To build your first PowerShell GUI, you will use Windows Forms. Start by initializing the form:
Add-Type -AssemblyName System.Windows.Forms
$form = New-Object System.Windows.Forms.Form
$form.Text = 'My First PowerShell GUI'
$form.Size = New-Object System.Drawing.Size(300, 200)
$form.StartPosition = 'CenterScreen'
$form.Add_Shown({$form.Activate()})
$form.ShowDialog()
Adding Controls
Next, enhance your form with components. For example, adding a button and a text box can be done as follows:
$textBox = New-Object System.Windows.Forms.TextBox
$textBox.Location = New-Object System.Drawing.Size(50, 50)
$form.Controls.Add($textBox)
$button = New-Object System.Windows.Forms.Button
$button.Text = "Show Message"
$button.Location = New-Object System.Drawing.Size(50, 80)
$form.Controls.Add($button)
Event Handling in PowerShell GUIs
Event handling is crucial for creating interactive applications. For instance, let’s add functionality to respond when the button is clicked:
$button.Add_Click({
[System.Windows.Forms.MessageBox]::Show("You entered: " + $textBox.Text)
})
With this setup, whenever the user clicks the button, a message box will display the text they've entered.
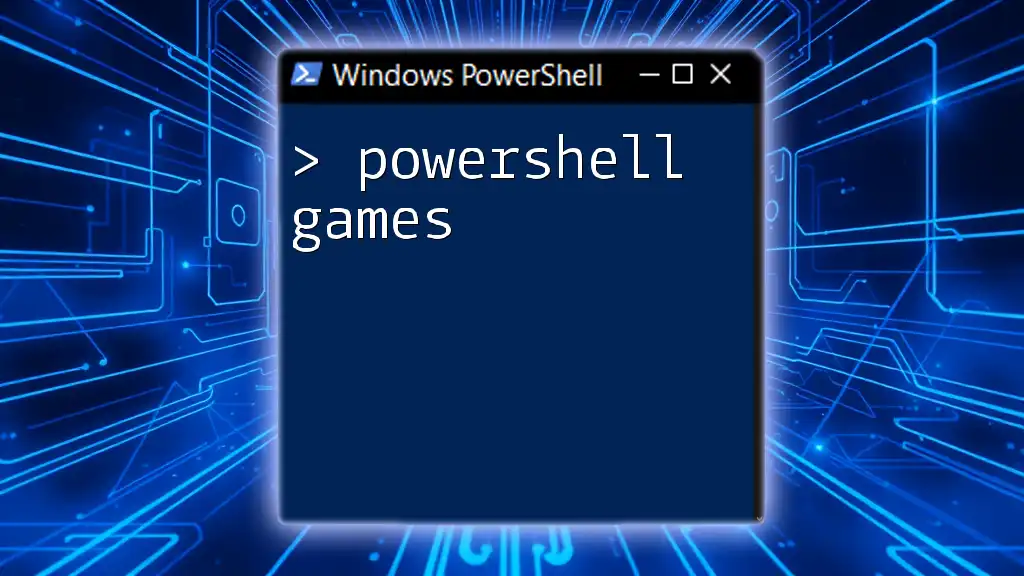
Creating an Advanced PowerShell GUI
Designing a Multi-Functional GUI
To develop a more complex GUI, consider an application that utilizes various controls like checkboxes, dropdown menus, and grids. This allows users to interact with multiple features seamlessly.
Sample Project: A Simple To-Do List Application
To illustrate a functional application, let’s create a To-Do List.
Setting Up the Application Structure
Your GUI will need a list box for displaying tasks, a text box for adding new tasks, and buttons for add/delete functionality.
Code Snippets for Each Part
Here’s how to put everything together:
$listBox = New-Object System.Windows.Forms.ListBox
$listBox.Location = New-Object System.Drawing.Size(50, 50)
$listBox.Size = New-Object System.Drawing.Size(200, 100)
$form.Controls.Add($listBox)
$addButton = New-Object System.Windows.Forms.Button
$addButton.Text = "Add Task"
$addButton.Location = New-Object System.Drawing.Size(50, 160)
$form.Controls.Add($addButton)
$removeButton = New-Object System.Windows.Forms.Button
$removeButton.Text = "Remove Task"
$removeButton.Location = New-Object System.Drawing.Size(150, 160)
$form.Controls.Add($removeButton)
Adding Functionality
You’ll need to implement the logic for adding and removing tasks:
$addButton.Add_Click({
if ($textBox.Text -ne "") {
$listBox.Items.Add($textBox.Text)
$textBox.Clear()
}
})
$removeButton.Add_Click({
if ($listBox.SelectedItem -ne $null) {
$listBox.Items.Remove($listBox.SelectedItem)
}
})
This code allows users to input a task and add it to the list or select and remove existing tasks.
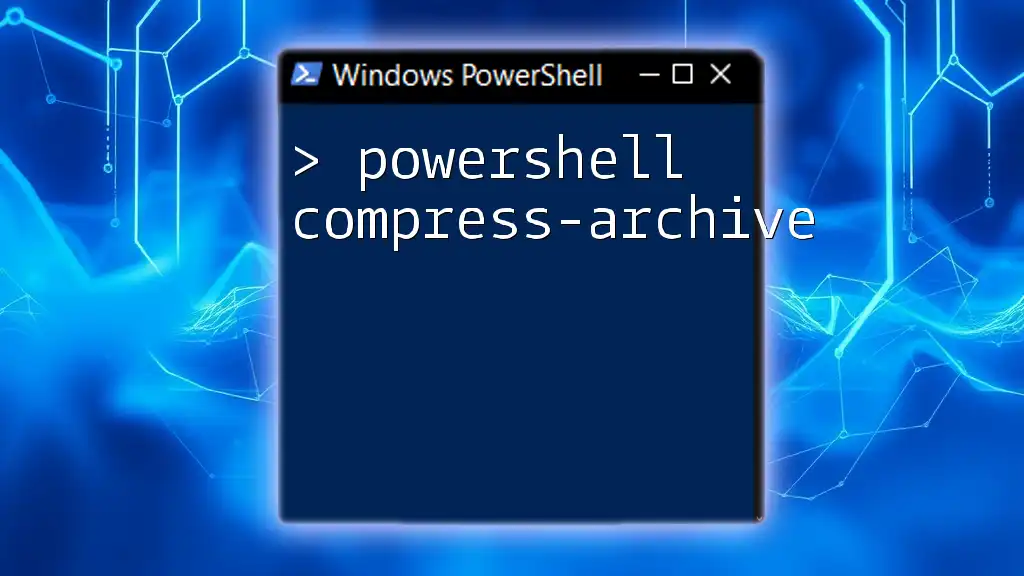
Customizing Your PowerShell GUI
Styling Your GUI
Enhancing your GUI's aesthetics can improve user engagement. Adjust properties such as background color, font size, and control alignment to create a polished look.
Adding Icons and Images
Incorporating icons can help users navigate your application. Use the `Image` property on buttons and other components to enhance visual appeal. For example:
$button.Image = [System.Drawing.Image]::FromFile("path-to-icon.png")
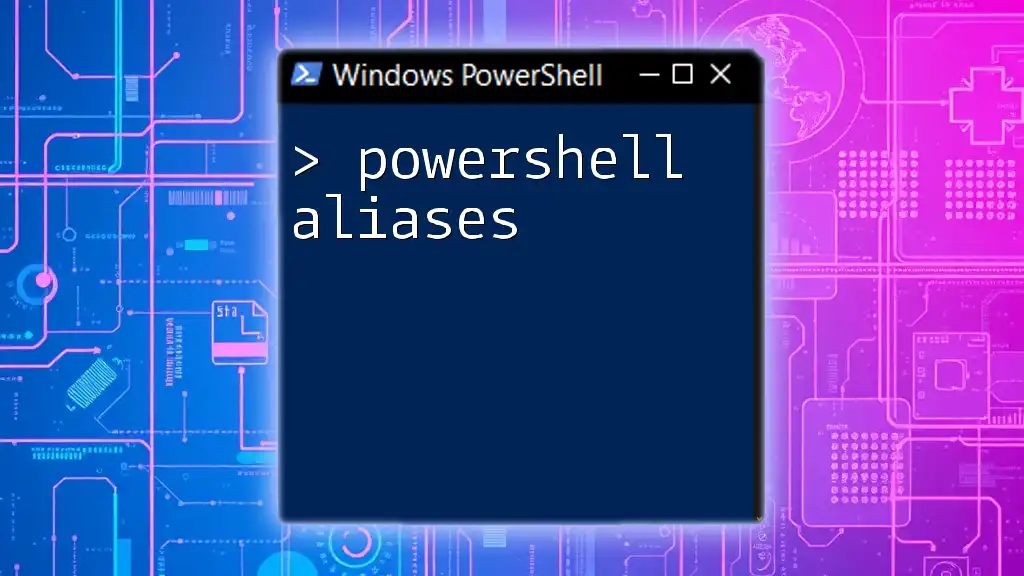
Debugging and Error Handling in PowerShell GUIs
Common Issues and Solutions
When developing GUIs, you may encounter problems like unresponsive forms or errors due to invalid inputs. It’s essential to validate user inputs before processing them.
Using Try/Catch for Error Handling
Implementing error handling can provide a smoother user experience. Here’s how you can handle exceptions:
try {
# Code that may fail
# Assume this is where interaction logic goes
} catch {
[System.Windows.Forms.MessageBox]::Show("An error occurred: $_")
}
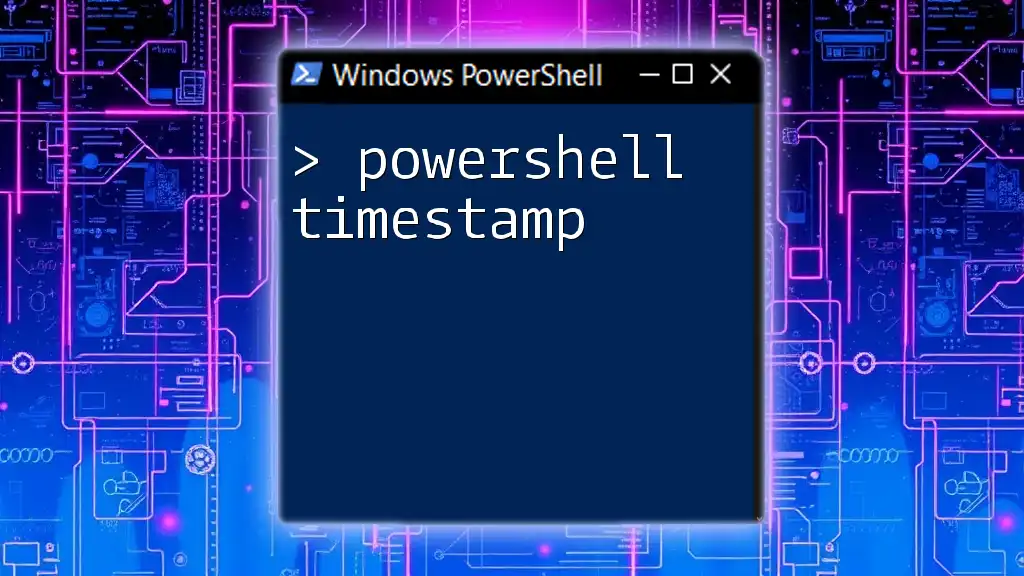
Conclusion
In this exploration of PowerShell GUI examples, we've examined everything from the foundational concepts of GUIs to practical implementations. By leveraging graphical interfaces, you can unlock the potential of PowerShell, making it accessible and user-friendly for a wider audience. We encourage you to further experiment with these components and build upon the examples presented, leading to innovative automation solutions.
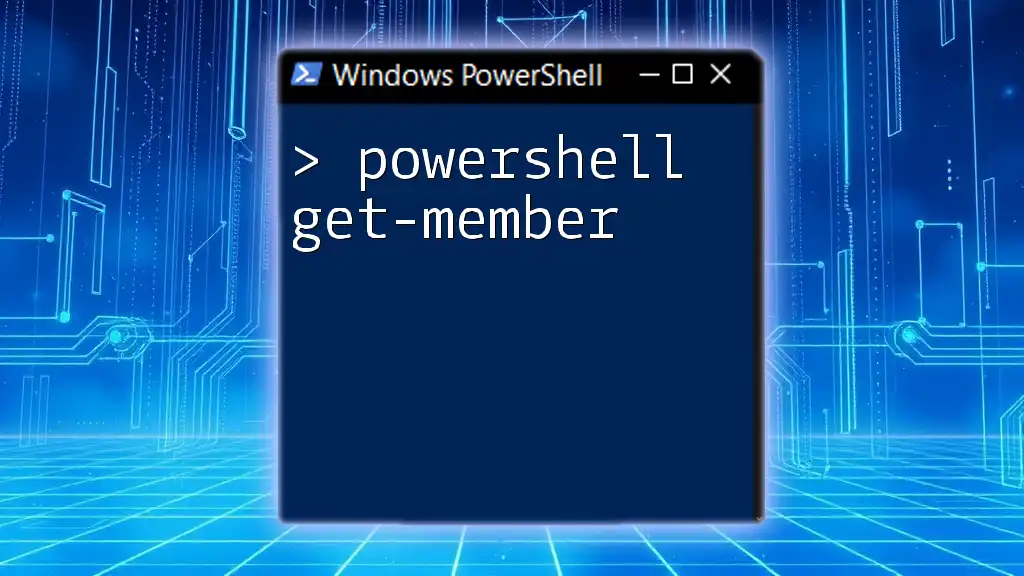
Additional Resources
For further learning, consult official PowerShell documentation and community forums that provide tutorials, support, and networking opportunities to delve deeper into PowerShell GUI development. Whether you are a beginner or an experienced developer, the PowerShell community is rich with resources to help you succeed.