"PowerShell by example empowers users to grasp key commands quickly through practical demonstrations and real-world scenarios."
Here’s a simple code snippet to illustrate this concept:
Write-Host 'Hello, World!'
Understanding PowerShell Basics
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed for system administration and automation. It allows administrator-level tasks to be performed efficiently, streamlining the management of Windows and other systems. By utilizing cmdlets, which are specialized .NET classes designed to perform specific functions, users can complete complex tasks with simple commands, making system management easier for both professionals and beginners alike.
Basic PowerShell Syntax
To effectively use PowerShell, familiarity with its command structure is essential. PowerShell commands, known as cmdlets, generally follow a Verb-Noun format, providing clarity about their intended action. For instance, the command below retrieves information about running processes:
Get-Command -Name "Get-Process"
Here, “Get” is the verb indicating the action of retrieving, and “Process” is the noun representing the target.
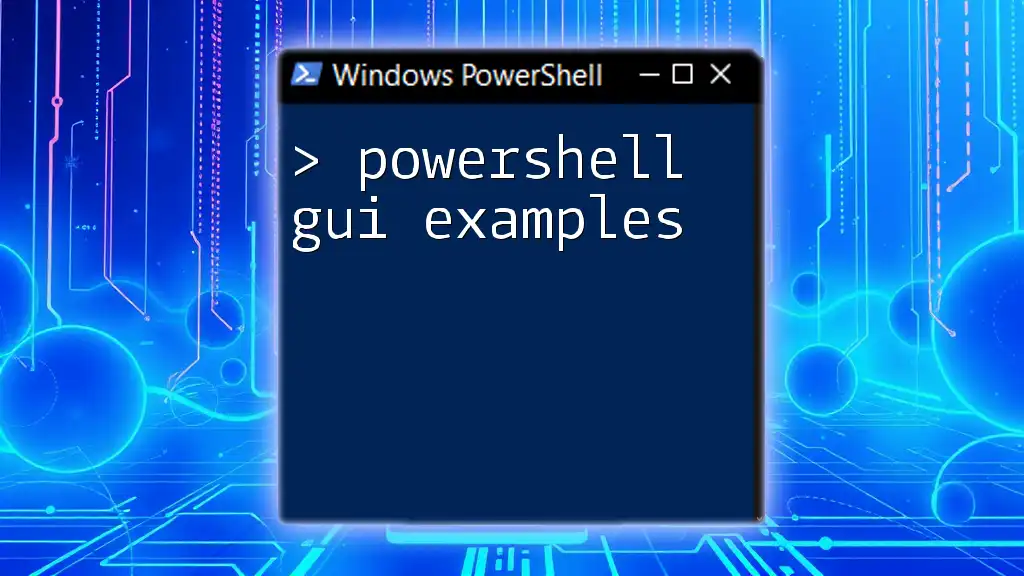
PowerShell by Example: Real-World Applications
Example of PowerShell Script for System Information
Gathering system information can be crucial for troubleshooting and monitoring purposes. A simple script such as:
Get-ComputerInfo | Select-Object CsName, WindowsVersion, WindowsBuildLabEx
This example provides a brief overview, displaying the system name, Windows version, and build number. By breaking down the script, we see that `Get-ComputerInfo` retrieves comprehensive system data, while `Select-Object` filters this information to show only the specified properties. This kind of tailored data retrieval can significantly enhance productivity during audits or diagnostics.
PowerShell Example Script for File Management
Managing files is another common task that PowerShell excels at. Suppose you want to find all `.txt` files in a specific folder and its subfolders. The following command achieves that:
Get-ChildItem -Path "C:\MyFolder" -Recurse | Where-Object { $_.Extension -eq ".txt" }
In this script, `Get-ChildItem` lists all files and directories in “C:\MyFolder,” while the `-Recurse` flag allows the command to dig through subdirectories. The `Where-Object` clause filters the results, returning only items with the `.txt` extension. Such scripts are invaluable for file organization and management tasks, saving time that would otherwise be spent manually sifting through files.
Example of PowerShell Script for System Administration
PowerShell is especially useful for system administrators needing to manage user accounts. Consider a script that creates a new local user account:
New-LocalUser -Name "newuser" -Password (ConvertTo-SecureString "P@ssw0rd!" -AsPlainText -Force) -FullName "New User" -Description "A new user account"
Here, `New-LocalUser` is the cmdlet responsible for creating the user. The `ConvertTo-SecureString` cmdlet generates a secure password, which is necessary for maintaining system security. This example showcases how one simple command can automate user creation, illustrating the efficiency of using PowerShell for system tasks.
Automating Software Installation Using PowerShell
PowerShell's automation capabilities extend to software installation as well. The following script can initiate an installation process:
Start-Process "msiexec.exe" -ArgumentList "/i C:\path\to\installer.msi /quiet"
In this case, `Start-Process` executes `msiexec.exe`, a Windows installer program, while the `-ArgumentList` parameter passes in the required arguments for silent installation. Automating software installations can save valuable time in organizational environments where multiple systems need to be configured, making it a must-know skill for IT professionals.
Collecting System Logs with PowerShell
Retrieving system logs using PowerShell can greatly assist in troubleshooting technical issues. The following command fetches the most recent application logs:
Get-EventLog -LogName Application -Newest 10
This script utilizes `Get-EventLog`, specifying the “Application” log and returning only the ten most recent entries. By understanding how to extract logs effectively, administrators can resolve problems quicker, enhancing overall system health and reducing downtime.
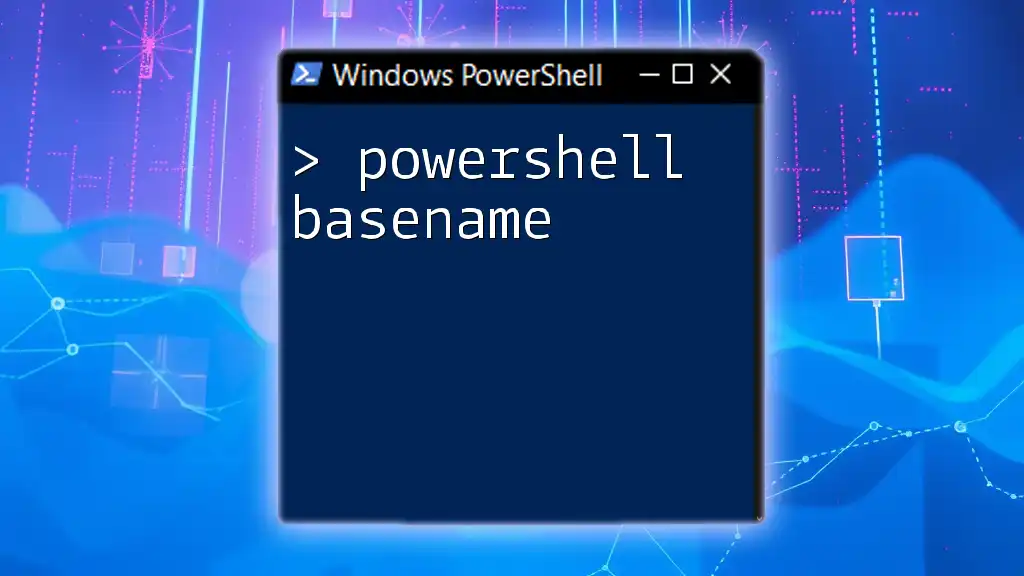
Writing Efficient PowerShell Scripts
Tips for Writing Effective PowerShell Scripts
To maximize the efficiency of your scripts, adhere to the following best practices:
- Keep it Simple: Write clear and concise scripts. Avoid unnecessary complexity.
- Comment Your Code: Regularly use comments to explain the purpose of commands and functions. This practice benefits you and other users in the future.
- Use Functions: When a task is repetitive, encapsulate it in a function. This not only improves readability but also allows for easier maintenance.
Common Errors in PowerShell Scripts to Avoid
While learning PowerShell, beginners often encounter pitfalls that can lead to frustration. Watch out for these common errors:
- Misspelled Cmdlets: Cmdlets are sensitive to spelling errors. Always double-check the syntax.
- Incorrect Path Names: Fit your scripts to the exact paths. A mismatch here can lead to errors in execution.
- Unclear Logic: Avoid convoluted scripts. If you’re struggling to follow your code, others will too.
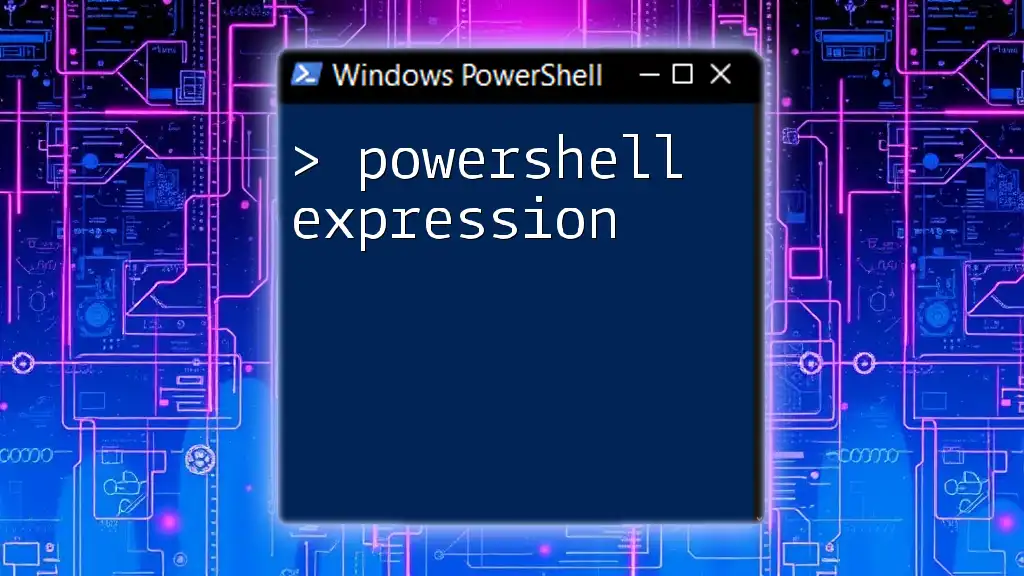
Advanced Examples in PowerShell
PowerShell with JSON Data
PowerShell can interact with JSON data, which is increasingly used in APIs and data interchange formats. The following script illustrates how to work with JSON:
$jsonData = '{"name":"John", "age":30}'
$person = $jsonData | ConvertFrom-Json
Here, `ConvertFrom-Json` transforms the JSON string into a PowerShell object, making it easier to manipulate the data. Understanding how to leverage JSON has become increasingly important in today's data-driven landscape.
Using PowerShell for Network Management
Effective network management is another area where PowerShell shines. Administrators can quickly retrieve IP address configurations with:
Get-NetIPAddress | Where-Object {$_.AddressFamily -eq "IPv4"}
This command retrieves all network IP addresses and filters them to show only IPv4 addresses. Mastering network management through PowerShell scripts can streamline processes like troubleshooting connectivity issues or documenting network configurations.
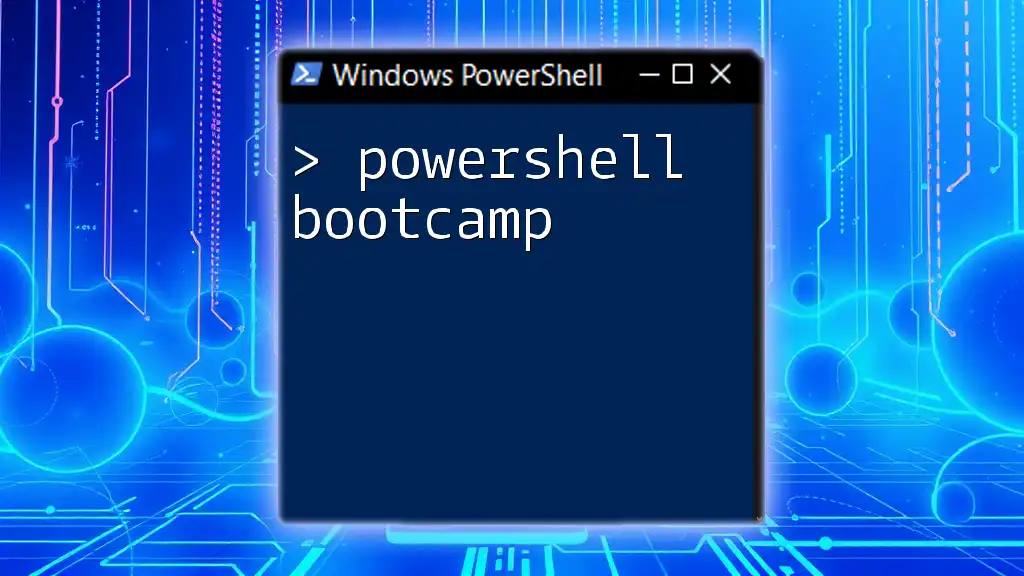
Conclusion
Learning PowerShell by example provides a solid foundation for automating tasks and managing systems efficiently. By understanding the practical applications of PowerShell scripts, from gathering system information to automating installations, you empower yourself to enhance productivity and troubleshoot issues effectively. Continued practice and exploration of advanced scripts will only deepen your expertise.
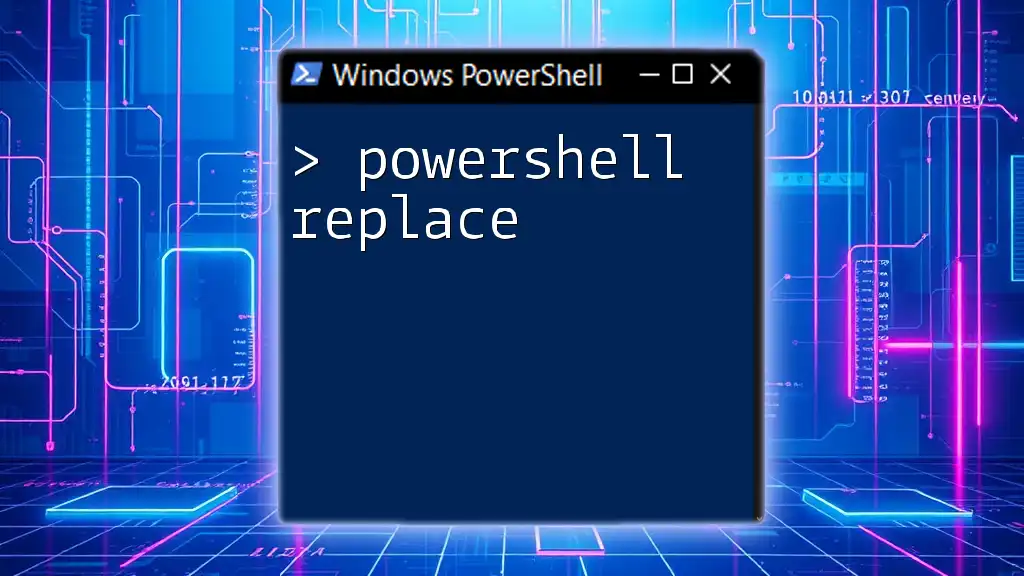
Additional Resources
To deepen your understanding, explore official PowerShell documentation for in-depth information. Consider joining community forums for tailored advice and learning opportunities, and check out the latest books and online courses designed to enhance your PowerShell skills.
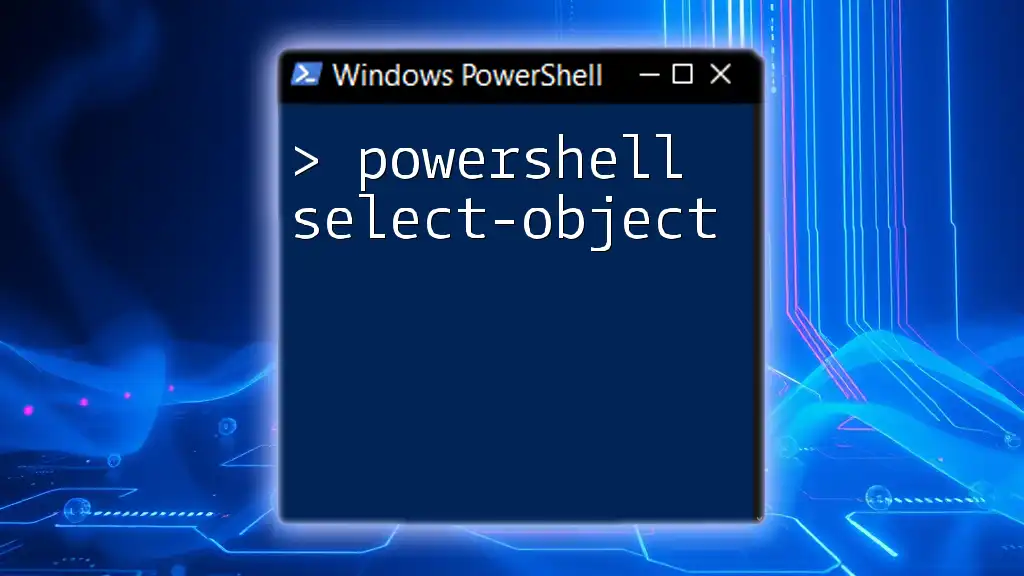
FAQs
- What are the prerequisites for learning PowerShell? Familiarity with basic command-line operations is helpful, but PowerShell is designed for users of all skill levels, making it accessible to beginners.
- How can I troubleshoot PowerShell scripts? Begin by executing smaller portions of your scripts and checking for errors. Use community resources and forums for additional support when needed.
By embracing PowerShell through practical examples, you will unlock the potential for automation and efficiencies in your task management, becoming a more capable and confident user.