PowerShell ADSI (Active Directory Service Interfaces) allows users to interact with and manage Active Directory objects and properties programmatically.
# Example: Retrieve a user from Active Directory
$user = [ADSI]"LDAP://CN=John Doe,OU=Users,DC=example,DC=com"
Write-Host $user.mail
Introduction to PowerShell ADSI
What is ADSI?
Active Directory Services Interface (ADSI) is a powerful Microsoft technology that allows administrators to manage and interact with Active Directory objects from scripts or applications. This interface provides a consistent programming model for accessing directory services, database systems, and object repositories.
Why Use PowerShell with ADSI?
PowerShell integrates seamlessly with ADSI, giving users the ability to execute commands and scripts in a straightforward way. The combination allows for automation of tasks like user management, group membership control, and policy application, significantly reducing manual workloads. This efficiency is essential in real-world IT environments, where time and accuracy are critical.

Setting Up PowerShell for ADSI
Installing PowerShell
PowerShell comes pre-installed on Windows 10 and Windows Server 2016. For earlier versions of Windows or to install newer versions, users may download it from the official Microsoft site.
Configuring Required Permissions
To interact with ADSI, you must have the appropriate permissions. Most Active Directory operations require administrator privileges. To check your current permissions, you can use these commands:
Get-Acl "AD:\"
This command retrieves and displays the current Access Control List (ACL) for Active Directory objects.
Loading the ADSI Provider
To access ADSI with PowerShell, you need to load the provider. This can be done easily with the following command:
$adsi = [ADSI]"LDAP://CN=Users,DC=yourdomain,DC=com"
This command connects to the specified location in the LDAP directory.

Understanding the ADSI Namespace
Breaking Down the ADSI Namespace
The ADSI namespace is structured hierarchically. The most common paths are `LDAP://` and `WinNT://`, which point to different types of objects stored within Active Directory.
- LDAP://: Mainly used for querying Active Directory.
- WinNT://: Suitable for accessing Windows domain objects.
Accessing Various Objects with ADSI
Using ADSI, you can access different directory objects such as users, groups, and computers. For example, to connect to the Active Directory:
$adsi = [ADSI]"LDAP://CN=Users,DC=yourdomain,DC=com"
This allows you to perform operations on the Users container directly.
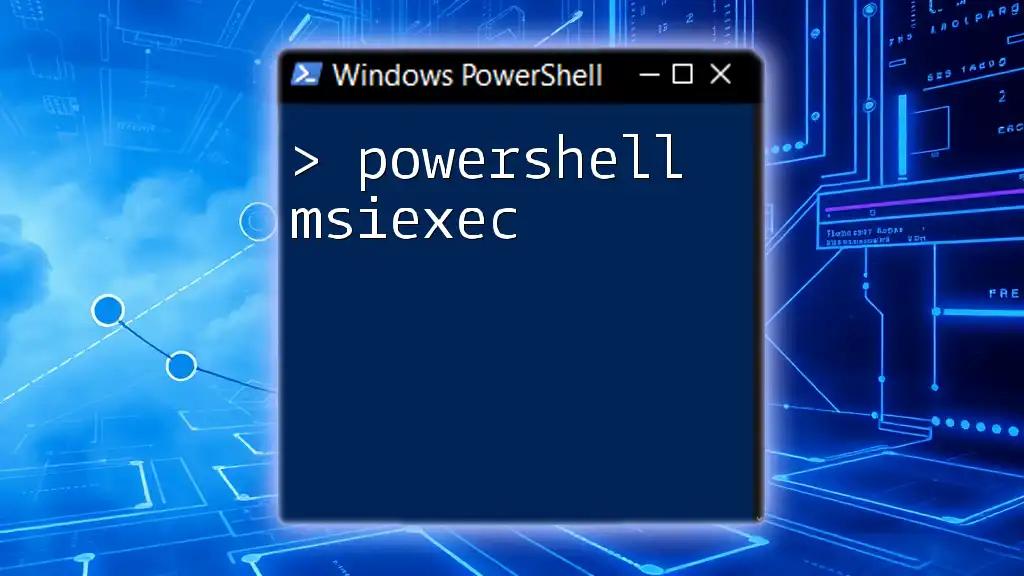
Common PowerShell ADSI Commands
Creating New Active Directory Objects
Creating objects in Active Directory can be accomplished using ADSI's `Create` method. The following example demonstrates how to create a new user:
$user = $adsi.Create("user", "CN=New User")
$user.Put("sAMAccountName", "newuser")
$user.SetInfo()
In this snippet, a new user object named "New User" is created, followed by setting the username.
Retrieving Information from Active Directory
You can read properties of directory objects easily with ADSI. For example, to retrieve an existing user’s details:
$user = [ADSI]"LDAP://CN=Existing User,CN=Users,DC=yourdomain,DC=com"
$user.displayName
This code locates an existing user and displays their `displayName` attribute.
Updating Active Directory Objects
To modify user attributes in ADSI, you can set the desired property and call `SetInfo()` to apply changes. For instance, updating a user’s email address looks like this:
$user.mail = "newemail@domain.com"
$user.SetInfo()
This command updates the user's email address in Active Directory.
Deleting Active Directory Objects
Removing objects, such as users, requires caution. Here’s how to delete a user safely:
$user = [ADSI]"LDAP://CN=User to Delete,CN=Users,DC=yourdomain,DC=com"
$user.Delete("user", "CN=User to Delete")
This code snippet finds the user and deletes them from the directory.
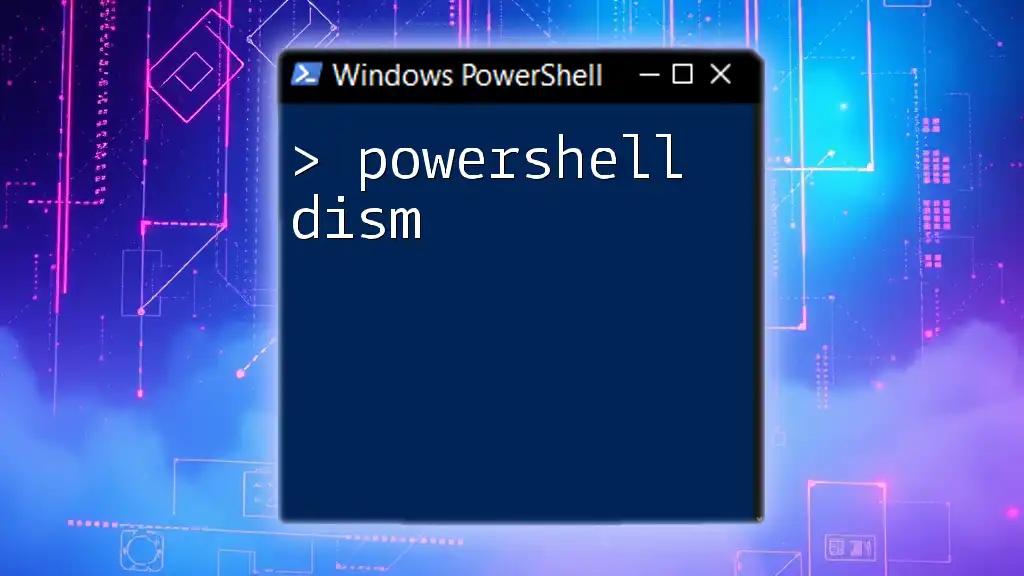
Working with Groups and Membership
Creating and Managing Groups
You can create groups in Active Directory using ADSI just like with users. Here’s how to create a new group and then add a user to that group:
$group = $adsi.Create("group", "CN=New Group")
$group.SetInfo()
$group.Add("LDAP://CN=UserName,CN=Users,DC=yourdomain,DC=com")
This creates "New Group" and adds an existing user to it.
Checking Group Membership
It's possible to verify a user's group membership with ADSI. For example, to check if a user is part of a specific group:
$group = [ADSI]"LDAP://CN=Example Group,CN=Users,DC=yourdomain,DC=com"
$isMember = $group.IsMember("LDAP://CN=Username,CN=Users,DC=yourdomain,DC=com")
This line checks the user’s membership status and returns `True` or `False`.
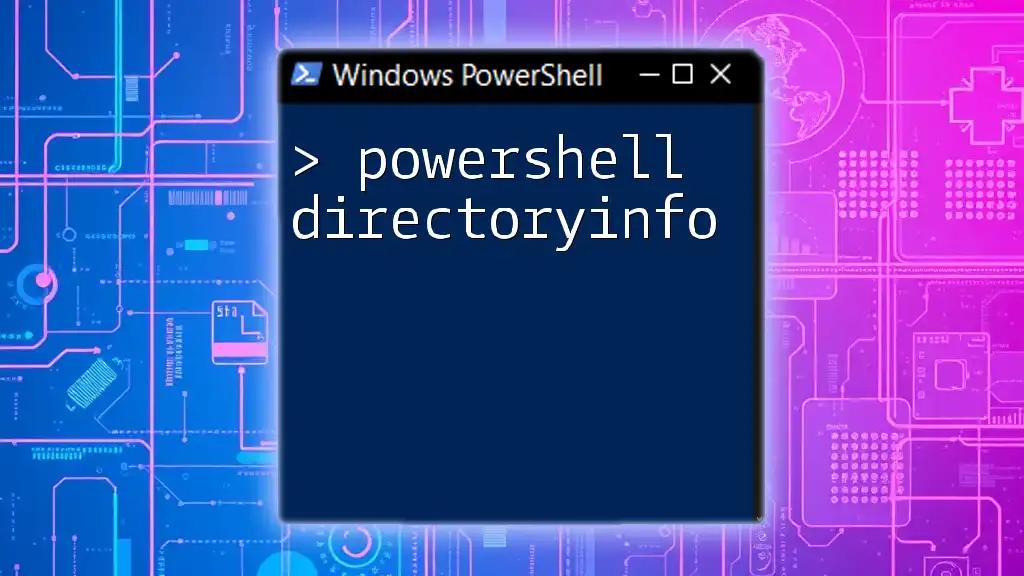
Best Practices for Using ADSI
Efficient Scripting Techniques
When using PowerShell ADSI, it’s best to encapsulate repetitive tasks within functions. This not only enhances readability but also maintains your code neat and manageable.
Error Handling in PowerShell ADSI Scripts
Adding error handling is crucial to ensure that your scripts run smoothly, especially when interacting with Active Directory. You can employ `try/catch` blocks to handle any exceptions gracefully:
try {
$user = [ADSI]"LDAP://CN=NonExistent,CN=Users,DC=yourdomain,DC=com"
} catch {
Write-Host "Failed to retrieve user: $_"
}
This example attempts to access a user, and if it fails, it informs the operator of the issue without crashing the script.

Conclusion
Summary of Key Points
In this comprehensive guide, we've explored the fundamentals of using PowerShell with ADSI for managing Active Directory objects. Whether you're creating, updating, or querying objects, PowerShell provides a powerful and flexible interface to work with.
Encouragement for Further Learning
The world of PowerShell and ADSI is vast and rich with possibilities. For those interested in deepening their knowledge, numerous resources exist, including official Microsoft documentation, online forums, and community discussions that provide insights and assistance in mastering PowerShell ADSI.
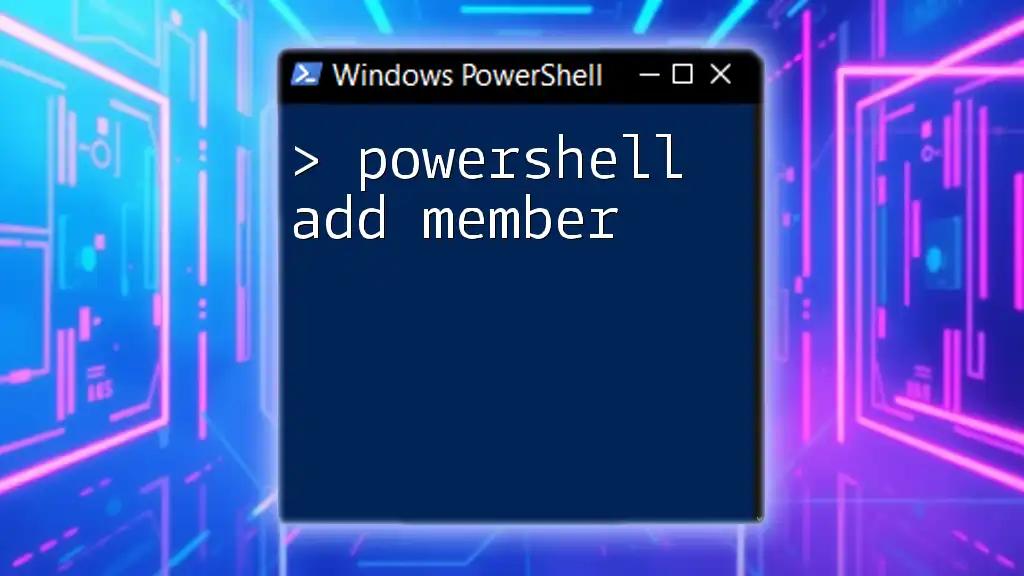
Additional Resources
For a further exploration of this topic, consider checking out recommended books, online tutorials, and engaging with PowerShell communities. The official Microsoft documentation is also an invaluable resource for keeping updated with the latest developments in PowerShell and ADSI.