To set the default web browser using PowerShell, you can modify the registry values associated with the desired browser.
Here’s the PowerShell code snippet to set a default browser (for example, Google Chrome):
$browserPath = "C:\Program Files\Google\Chrome\Application\chrome.exe"
Set-ItemProperty -Path "HKCU:\Software\Microsoft\Windows\Shell\Associations\UrlAssociations\http\UserChoice" -Name "ProgId" -Value "ChromeHTML"
Make sure to customize the `$browserPath` variable according to the browser you are setting as default.
Understanding Default Browsers
What is a Default Browser?
A default browser is the web browser that your operating system uses to open web links. When you click on a link in a document, email, or another application, the default browser dictates which application will launch. This setting is vital for enhancing your user experience, as it ensures consistency in how web content is handled across various platforms.
Why Use PowerShell to Set a Default Browser?
Using PowerShell to set a default browser offers several advantages:
- Efficiency: The process can be automated, allowing for quick changes without navigating through graphical settings.
- Scripting Capabilities: You can create scripts to set default browsers across multiple user accounts, making it a powerful tool for system administrators and power users alike.
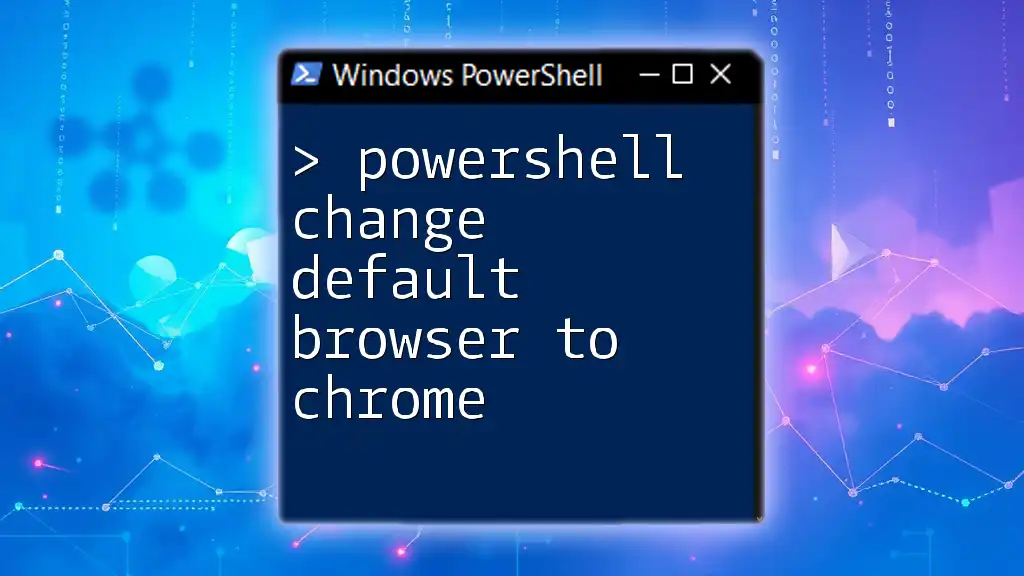
Preparing Your Environment
Checking PowerShell Version
Before diving into setting the default browser, it’s essential to ensure you are using an updated version of PowerShell. To check your PowerShell version, run:
$PSVersionTable.PSVersion
If your version is outdated, consider updating it to benefit from the latest features and security updates.
Administrator Privileges
To change system settings, including default browsers, you need to run PowerShell with administrator privileges. Here’s how you can do that:
- Right-click on the Start button.
- Select “Windows PowerShell (Admin).”
Running PowerShell as an administrator grants the necessary permissions to modify system configurations.
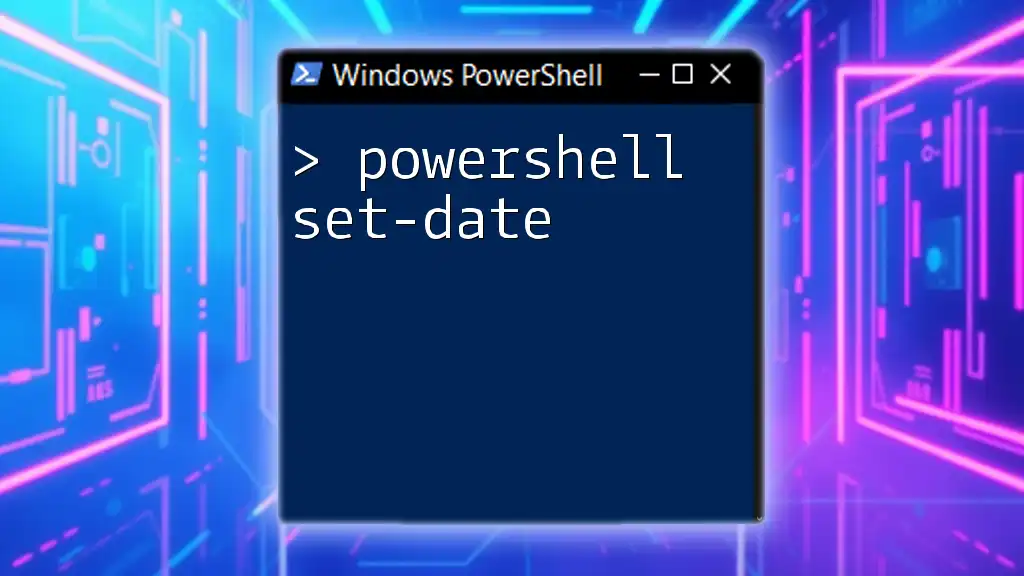
Common Browsers to Set as Default
Overview of Popular Browsers
Here are some of the most commonly used web browsers you might consider setting as default:
- Google Chrome: Known for its speed and extensive extension library.
- Mozilla Firefox: Renowned for its privacy features and customizability.
- Microsoft Edge: Integrated with Windows and offers increasing performance and features.
- Opera: Features a built-in VPN and a unique browser experience.
- Brave: Focused on privacy, it blocks ads and trackers by default.
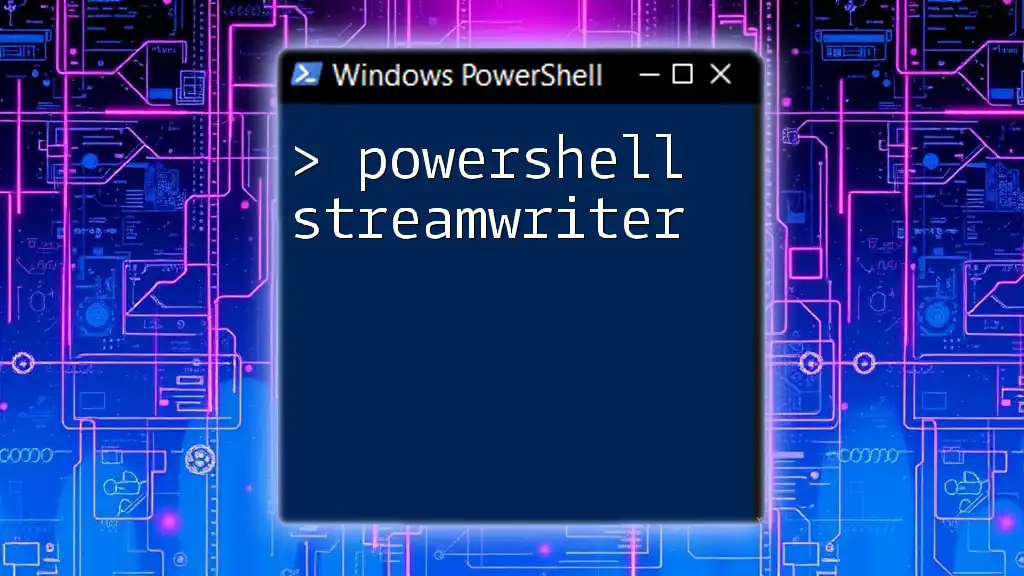
Setting the Default Browser Using PowerShell
Step-by-Step Process
Understanding the Registry
Windows manages default applications using the Windows Registry, particularly through `HKEY_CURRENT_USER` and `HKEY_LOCAL_MACHINE`. PowerShell allows you to modify these registry keys to change default browser settings.
Using PowerShell to Modify Registry Entries
To set Google Chrome as your default browser, you’ll need to modify specific registry entries. Here’s how to do it:
Example Code Snippet:
$chromePath = 'C:\Program Files\Google\Chrome\Application\chrome.exe'
$chromeProgId = 'ChromeHTML'
Set-ItemProperty -Path "HKCU:\Software\Microsoft\Windows\Shell\Associations\UrlAssociations\http\UserChoice" -Name ProgId -Value $chromeProgId
Set-ItemProperty -Path "HKCU:\Software\Microsoft\Windows\Shell\Associations\UrlAssociations\https\UserChoice" -Name ProgId -Value $chromeProgId
This script sets Google Chrome as the default handler for both HTTP and HTTPS links. The `ProgId` corresponds to the browser you wish to set as default.
Automating the Process
If you manage multiple user accounts, you can automate the process of setting the default browser by creating a PowerShell script. Here’s an example of a simple PowerShell script structure:
Example Script:
# Default Browser Update Script
param (
[string]$browserProgId = "ChromeHTML"
)
# Set HTTP and HTTPS associations
Set-ItemProperty -Path "HKCU:\Software\Microsoft\Windows\Shell\Associations\UrlAssociations\http\UserChoice" -Name ProgId -Value $browserProgId
Set-ItemProperty -Path "HKCU:\Software\Microsoft\Windows\Shell\Associations\UrlAssociations\https\UserChoice" -Name ProgId -Value $browserProgId
Write-Host "Default browser set to $browserProgId"
This script allows you to change the default browser by simply passing the desired browser's `ProgId` as a parameter, making it flexible for various browsers.
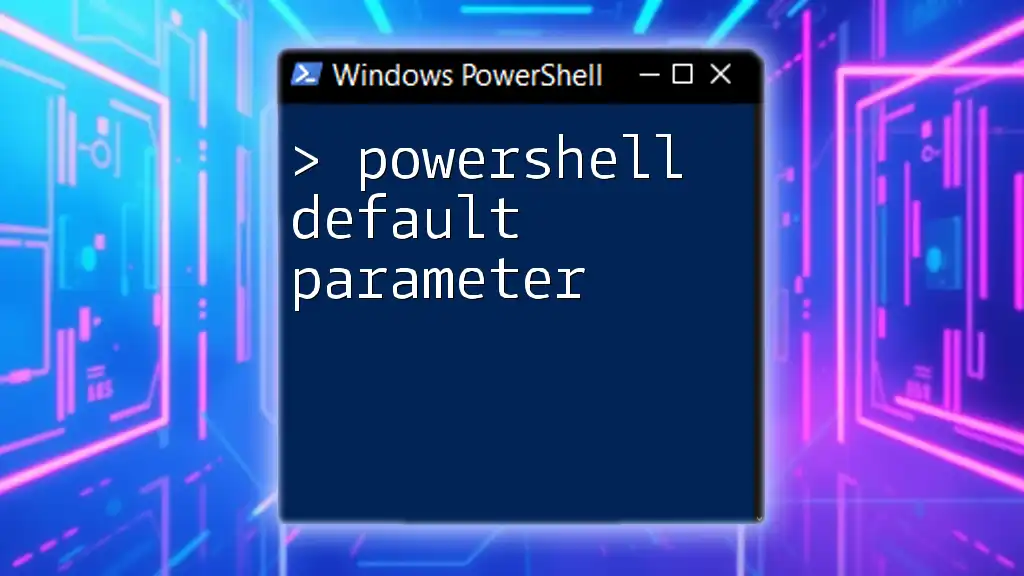
Troubleshooting Common Issues
Permission Errors
One common issue you may encounter is permission errors when attempting to change the default browser settings. To resolve this, ensure that you are running PowerShell with administrative privileges. Moreover, double-check that you have permission to edit the registry on the machine.
Browser Not Changing
If you notice that the default browser hasn’t changed despite running the script, there could be multiple factors at play:
- It's essential to check that the browser is correctly installed and registered in the system.
- Sometimes, group policies or third-party applications can override manual settings. Ensure that no policies are preventing the change.
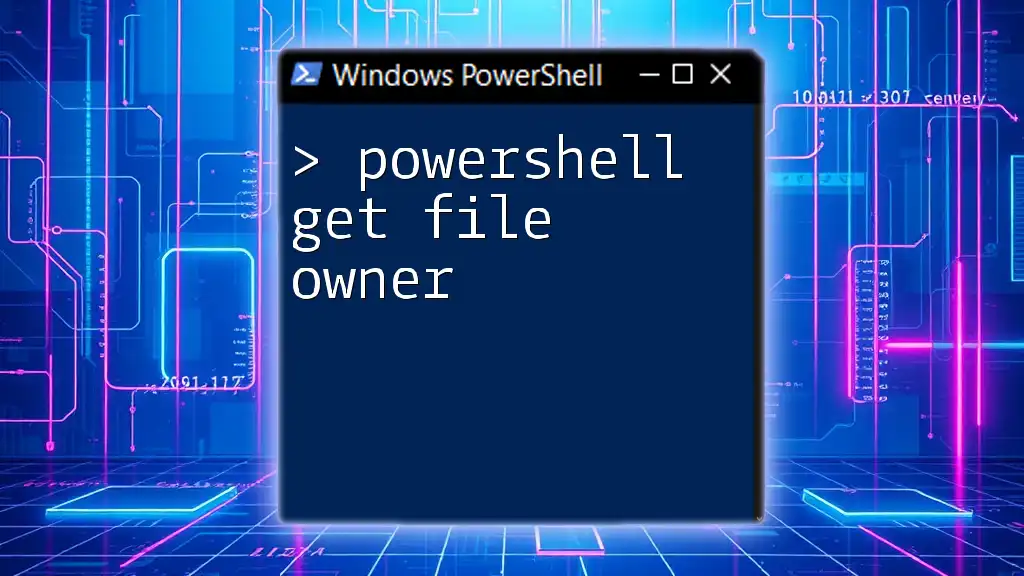
Testing Your Configuration
Verifying the Default Browser
After running your script, it’s crucial to verify that the default browser has been successfully changed. You can do this by executing a simple command to launch a URL. For example:
start http://www.example.com
If the correct browser opens when you run this command, you've successfully set your default browser using PowerShell!
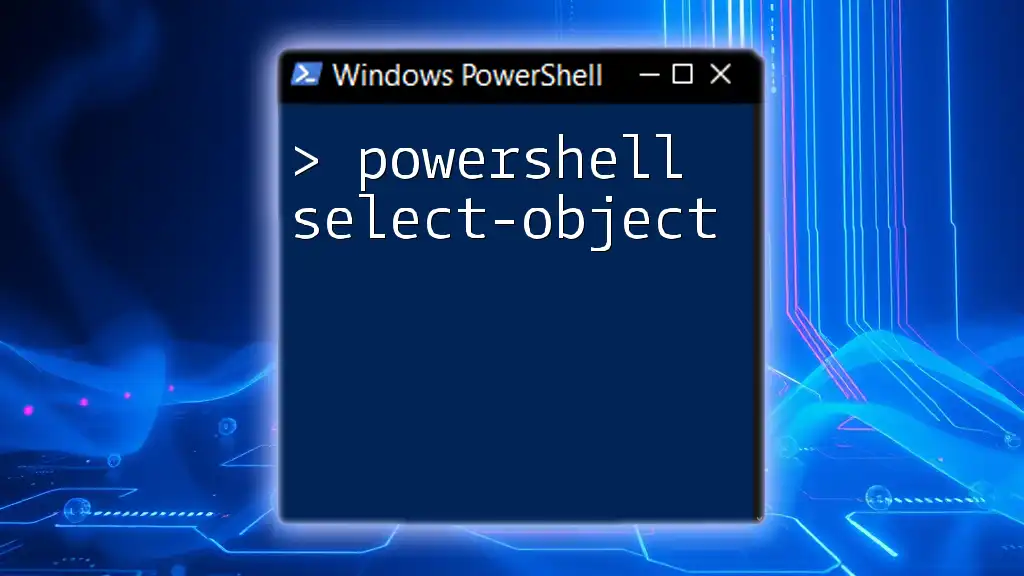
Conclusion
Setting the default browser through PowerShell is an efficient way to manage your system’s web experience. By leveraging scripts, you can automate this process for individual users or across multiple accounts. Whether you’re configuring your personal devices or managing a larger network, using PowerShell to set your default browser can save time and effort.
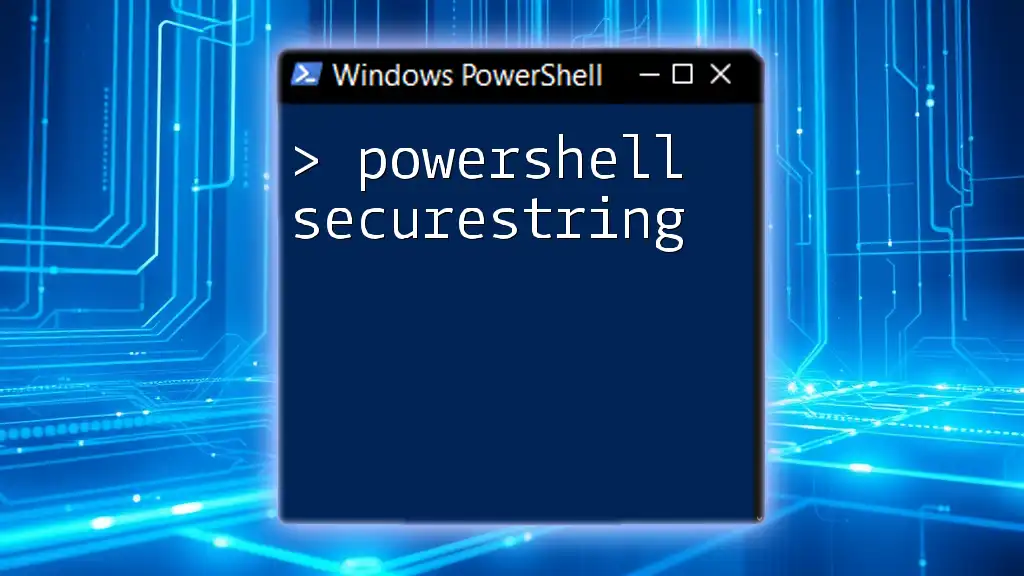
Additional Resources
For more detailed information about PowerShell, consider reviewing the official [PowerShell documentation](https://docs.microsoft.com/en-us/powershell/). You’ll find numerous resources to help expand your knowledge and capabilities within this powerful tool.
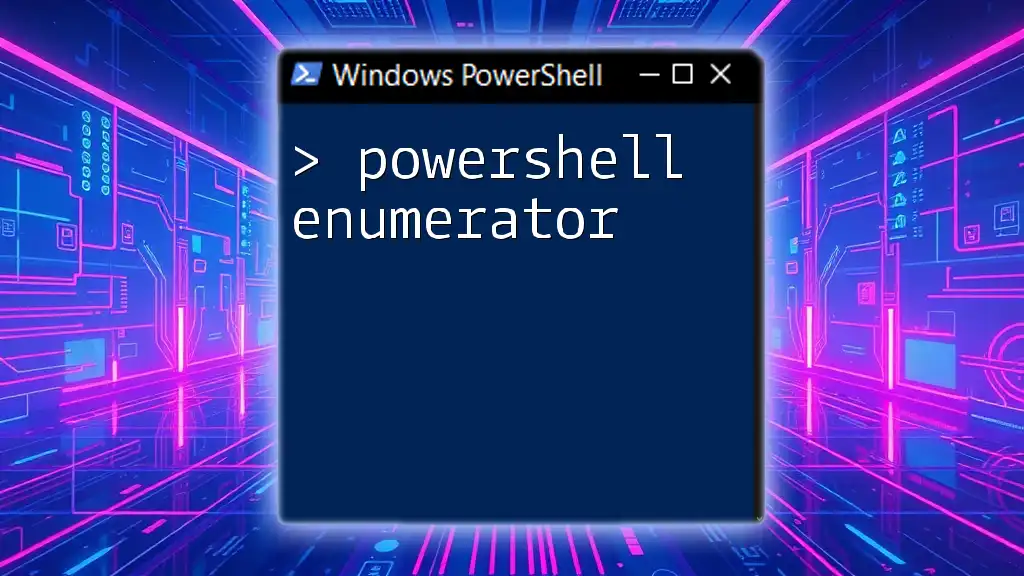
FAQs
Can I set the default browser for all users on a system?
Yes, it is possible to set the default browser for all users, but it requires modifying the registry keys in `HKEY_LOCAL_MACHINE` instead of `HKEY_CURRENT_USER`. This typically necessitates higher privileges and should be done with caution, ensuring that it aligns with the needs of all users.
Is it possible to set the default browser through a group policy?
Yes, setting a default browser through group policy is a widely used method in organizational contexts. Group policies allow administrators to enforce specific settings across all user accounts, including default applications like browsers.