In PowerShell, the equivalent of the Telnet command for testing network connectivity to a specific port can be achieved using the `Test-NetConnection` cmdlet.
Test-NetConnection -ComputerName example.com -Port 23
Understanding Telnet
What is Telnet?
Telnet is a network protocol that allows for communication between two networked devices. Often utilized for remote management of systems, it operates over the Transmission Control Protocol (TCP) and allows users to log into remote systems via a command line interface. Common use cases for Telnet include testing connectivity to a specific port, troubleshooting network services, and managing network devices.
Limitations of Telnet
Despite its utility, Telnet comes with significant security concerns. The protocol transmits data, including usernames and passwords, in plaintext, making it susceptible to interception and unauthorized access. In modern networking practices, these vulnerabilities have led to a decline in its usage, with alternative protocols like SSH (Secure Shell) recommended for secure communication.
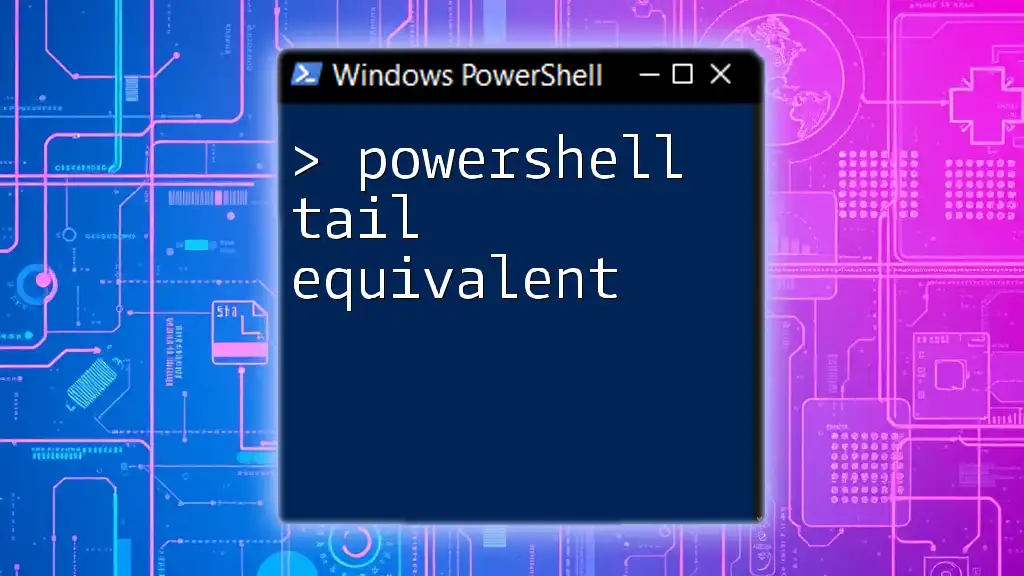
PowerShell Overview
What is PowerShell?
PowerShell is a powerful task automation and configuration management framework from Microsoft. It combines the functionality of a command-line shell with a scripting language, enabling users to interact with and manage system configurations and networks efficiently. Unlike traditional command-line interfaces, PowerShell structures its commands in a way that makes them more intuitive and flexible.
Why Use PowerShell as a Telnet Equivalent?
The power of PowerShell lies in its inherent versatility and robust built-in networking capabilities. It allows users to perform complex networking tasks without the weaknesses associated with Telnet. PowerShell provides a modern replacement for checking network connections and managing networked systems with enhanced flexibility, error handling, and scriptability.
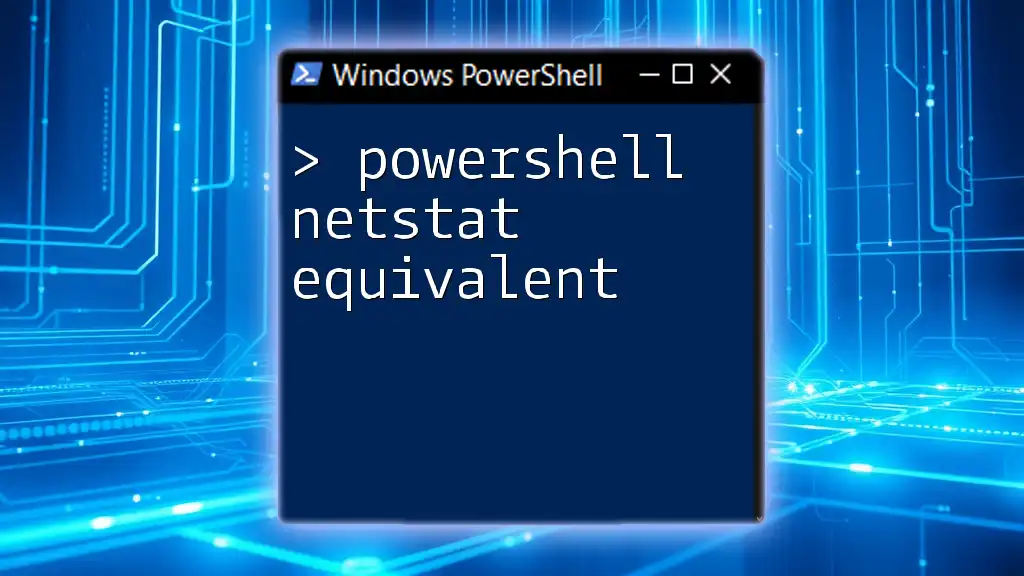
PowerShell Commands to Mimic Telnet Functionality
Using `Test-NetConnection`
Overview
The `Test-NetConnection` cmdlet in PowerShell is a valuable tool for assessing connectivity to specified ports on remote hosts. This cmdlet effectively replaces several Telnet functions by reporting the status of the network connection to a given address and port.
Example Command
Test-NetConnection -ComputerName "example.com" -Port 80
This command checks connectivity to the specified domain (`example.com`) over port 80 (commonly used for HTTP). The output provides feedback about the connection status, including whether it is successful or any errors encountered.
Using `Telnet` Command in PowerShell
Enabling Telnet Client in Windows
While PowerShell itself offers various connectivity tests, the traditional `telnet` command can still be utilized. However, it may not be enabled by default on Windows. To enable it, follow these steps:
- Press the Windows key and type "Turn Windows features on or off."
- Scroll down to Telnet Client, check the box, and click OK to install it.
Example Usage
Once enabled, you can use the `telnet` command within PowerShell:
telnet example.com 80
This command connects to `example.com` on port 80. While it has its vulnerabilities, it can still serve as an operational tool for specific quick checks on legacy systems.
Creating a Simple TCP Client in PowerShell
Explanation of TCP Client
For users looking to create customized networking scripts, PowerShell allows building a simple TCP client to interact with remote services. This method gives you more control than traditional commands, enabling tailored actions and responses.
Example Code Snippet
Here’s a basic example of building a TCP client in PowerShell:
$tcpClient = New-Object System.Net.Sockets.TcpClient
$tcpClient.Connect("example.com", 80)
$stream = $tcpClient.GetStream()
# Send a request
$writer = New-Object System.IO.StreamWriter($stream)
$writer.WriteLine("GET / HTTP/1.1")
$writer.WriteLine("Host: example.com")
$writer.WriteLine("")
$writer.Flush()
# Read response
$reader = New-Object System.IO.StreamReader($stream)
$response = $reader.ReadToEnd()
$response
In this example, we:
- Create a new TCP client.
- Connect it to `example.com` on port 80.
- Use a stream writer to send an HTTP GET request.
- Read and display the server's response using a stream reader.
This approach allows for automated responses and checks, showcasing PowerShell's capability of functioning as a Telnet equivalent through customized scripts.
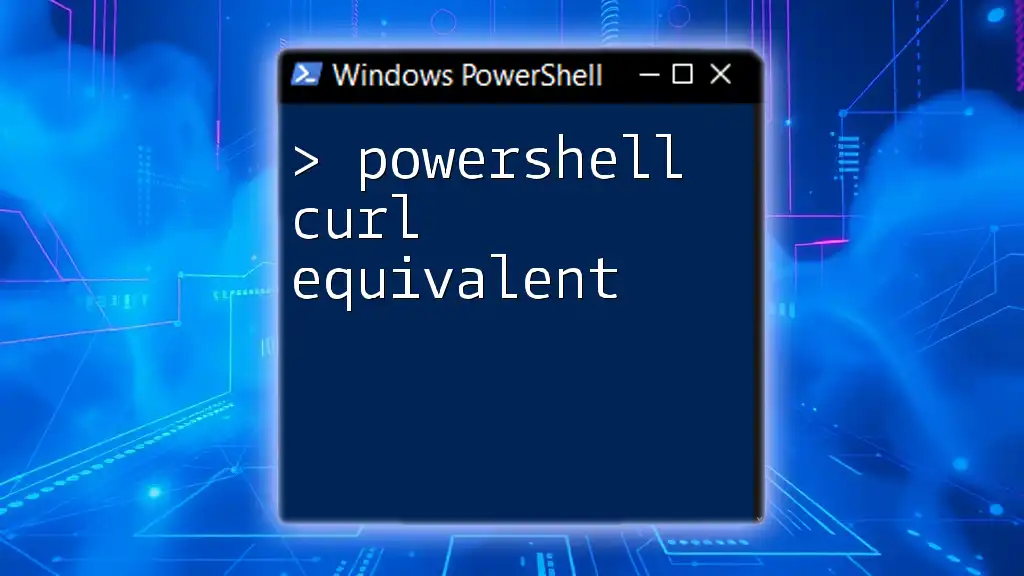
PowerShell Networking Cmdlets
Other Useful Networking Cmdlets
Overview of Networking in PowerShell
PowerShell is equipped with various cmdlets that enhance its networking capabilities beyond the simple Telnet functionalities. Understanding these cmdlets can greatly aid in diagnosing issues and managing network configurations.
Example Cmdlets
-
`Get-NetIPAddress`: This cmdlet retrieves the IP configuration of the local system, offering valuable insights into the networking setup.
Get-NetIPAddress
-
`Get-NetTCPConnection`: This command lists all active TCP connections. It provides immediate visibility into what ports are in use and can help identify any conflicts or unwanted connections.
Get-NetTCPConnection
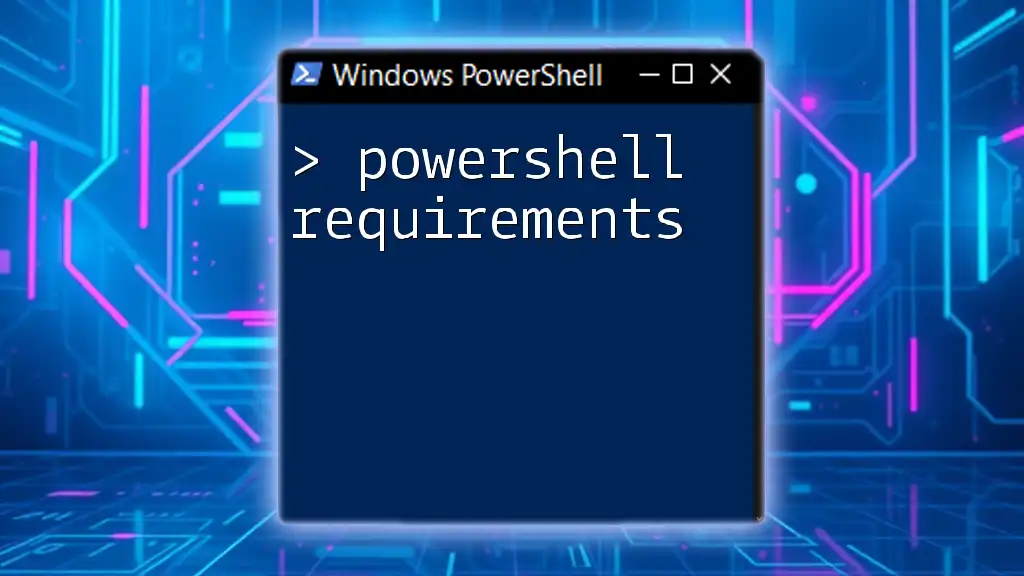
Troubleshooting Connectivity with PowerShell
Common Issues
When testing connectivity with PowerShell tools, users may encounter various issues, such as incorrect host names, port blocks by firewalls, or network misconfigurations. It's crucial to understand these potential pitfalls for effective troubleshooting.
Diagnosing with PowerShell
PowerShell can serve as a valuable ally in diagnosing connectivity issues. Utilize cmdlets like `Get-EventLog` to review network-related events. This cmdlet fetches entries from the event log, helping identify system or application errors that may affect network performance.
Get-EventLog -LogName Application -EntryType Error
This command retrieves errors from the Application log, allowing users to pinpoint issues that may hinder successful connections.
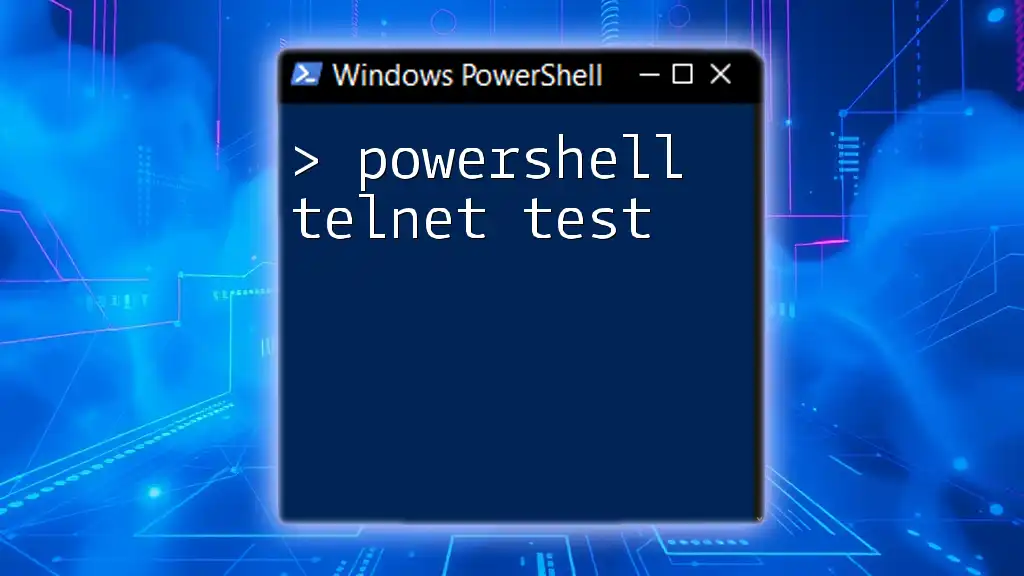
Conclusion
In a world where secure communication is paramount, understanding how to replace the traditional Telnet protocol with PowerShell's more secure and capable command sets is essential. This guide has provided a comprehensive overview of various methods and commands that serve as a PowerShell telnet equivalent, highlighting not just basic functionality but also empowering users to explore further network management options within PowerShell.
By trying out the provided examples and diving deeper into the recommended cmdlets, users can enhance their understanding and effective utilization of PowerShell for networking tasks. Embrace the powerful capabilities of PowerShell, and transform the way you manage network connectivity!
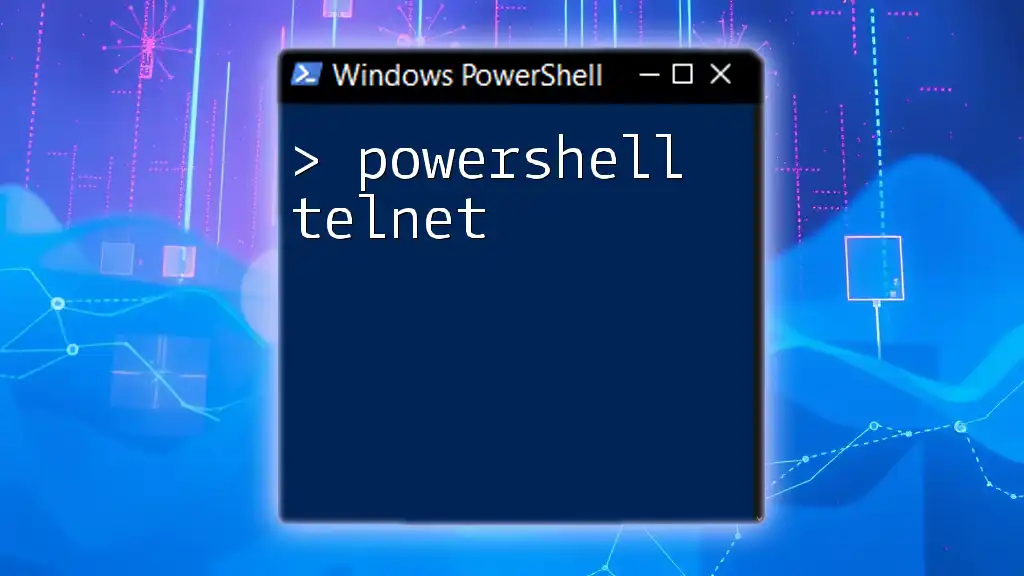
Additional Resources
For further learning, consider visiting the official PowerShell documentation for detailed insights, enrolling in dedicated courses, and engaging with community forums to share knowledge and challenges in mastering PowerShell.