In PowerShell, the equivalent of the `curl` command to fetch content from a URL is achieved using the `Invoke-WebRequest` cmdlet, as shown in the following example:
Invoke-WebRequest -Uri "http://example.com" -OutFile "output.html"
Understanding CURL Commands
What is CURL?
CURL is a command-line tool used for transferring data with URL syntax. It supports a variety of protocols, including HTTP, HTTPS, FTP, and more. One of its primary strengths is its ability to connect and communicate with different types of servers, making it essential for developers and system administrators when testing APIs or downloading resources over the web.
Common CURL Usage Scenarios
CURL is commonly used for several tasks:
- Making GET requests: Retrieve data from a server.
- Submitting POST requests: Send data to a server to create or update resources.
- Downloading files: Easily pull files from remote servers.
- Authenticating against APIs: Handle token-based and basic authentication seamlessly.
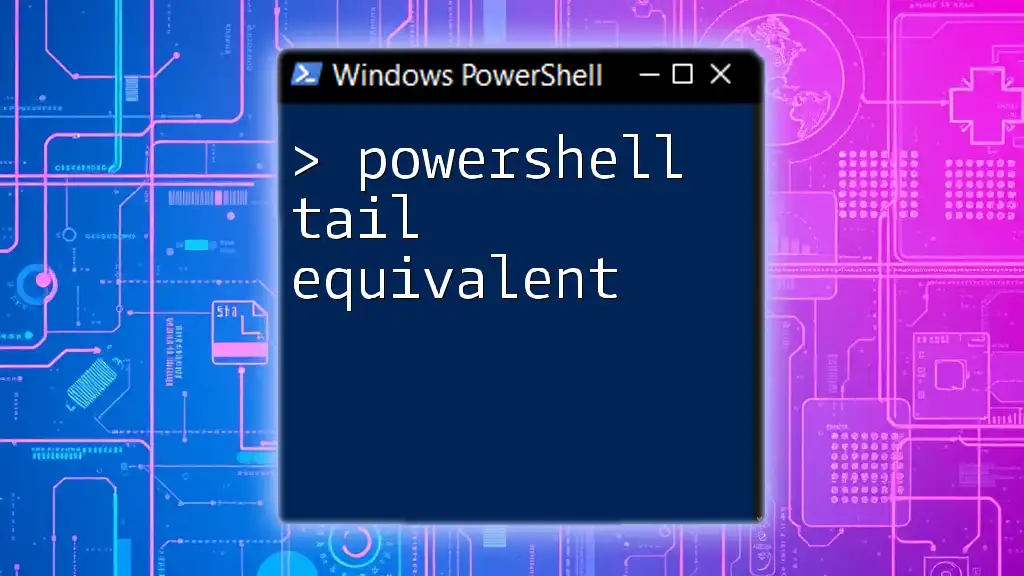
PowerShell: The Basics
Introduction to PowerShell Cmdlets
PowerShell cmdlets are built-in functions that enable users to perform specific functions efficiently. Unlike traditional command-line interfaces, PowerShell is built on the .NET framework and allows for easy handling of complex tasks, like HTTP requests, through easily understandable commands.
PowerShell's Web Cmdlets
PowerShell provides powerful cmdlets for working with web requests: `Invoke-RestMethod` and `Invoke-WebRequest`. These cmdlets are designed for API interaction and web page retrieval, making PowerShell a strong alternative to CURL.
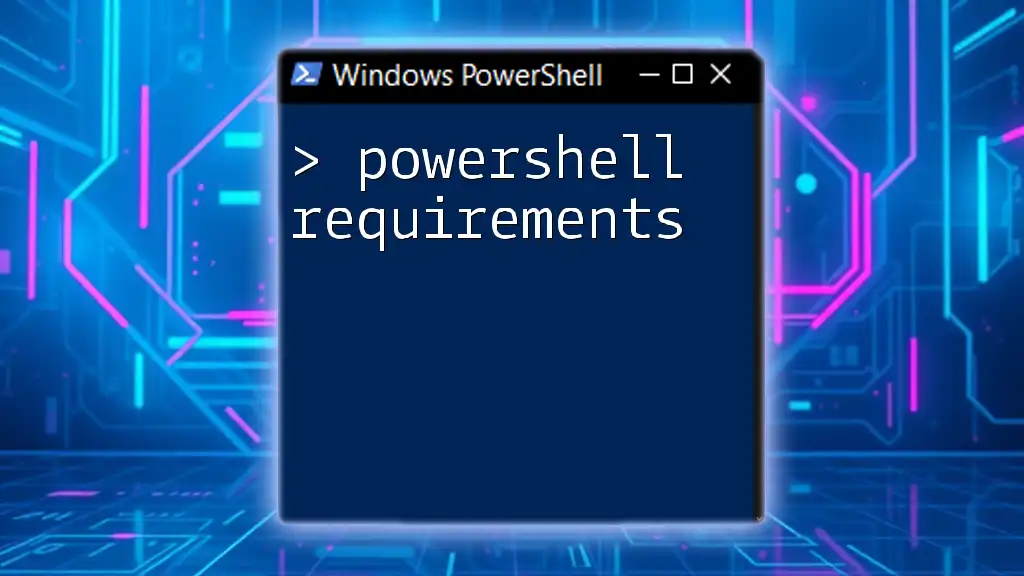
PowerShell Curl Equivalent: Getting Started
Making GET Requests
To perform a GET request in PowerShell, you can use the `Invoke-RestMethod` cmdlet. This cmdlet automatically converts the response to JSON, which makes it easier to work with.
Here’s the syntax for a basic GET request:
Invoke-RestMethod -Uri 'https://api.example.com/data' -Method Get
Example:
$result = Invoke-RestMethod -Uri 'https://jsonplaceholder.typicode.com/posts'
$result | Format-Table
In this example, we retrieve a list of posts from a sample JSON placeholder API and format the result in a readable table format.
Submitting POST Requests
When it comes to sending data to a server, PowerShell uses the following syntax for a POST request with `Invoke-RestMethod`:
Invoke-RestMethod -Uri 'https://api.example.com/data' -Method Post -Body $body
Example:
$body = @{ title = 'New Post'; body = 'This is the body'; userId = 1 }
$response = Invoke-RestMethod -Uri 'https://jsonplaceholder.typicode.com/posts' -Method Post -Body $body
$response
In this example, we are creating a new post with a title, body, and user ID in our request payload. PowerShell makes it simple to format this data using a hashtable.
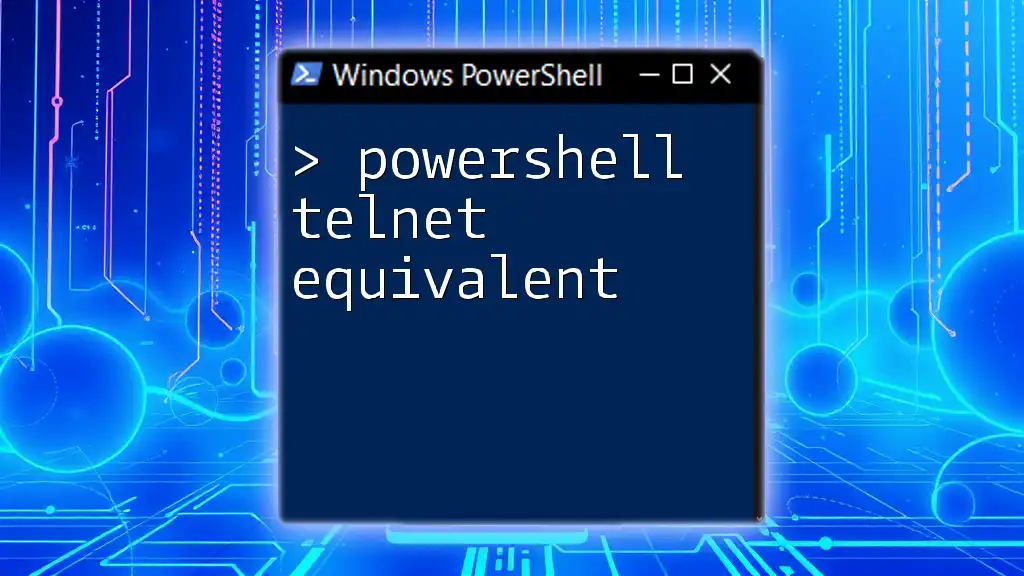
Additional PowerShell Features for HTTP Requests
Headers and Authentication
Sometimes you may need to include headers, such as authorization tokens, with your requests. Here's how to add headers in PowerShell:
$headers = @{ Authorization = 'Bearer your_token' }
$response = Invoke-RestMethod -Uri 'https://api.example.com/protected' -Headers $headers
Basic Authentication Example:
If you are connecting to an API that requires basic authentication, here's a straightforward example:
$username = 'your_username'
$password = 'your_password'
$pair = "$username:$password"
$base64 = [Convert]::ToBase64String([Text.Encoding]::ASCII.GetBytes($pair))
$headers = @{ Authorization = "Basic $base64" }
$response = Invoke-RestMethod -Uri 'https://api.example.com/secure-endpoint' -Headers $headers
This snippet encodes your username and password into a base64 string to be sent as an authentication header.
Handling Responses
Working with JSON responses in PowerShell is simplified due to its automatic conversion. You can easily parse the response data:
$jsonResponse = Invoke-RestMethod -Uri 'https://jsonplaceholder.typicode.com/posts' -Method Get
$jsonResponse[0].title
In this case, we are accessing the title of the first post received from the API.
Downloading Files
Just as CURL has command-line options for downloading, PowerShell also provides a straightforward method using `Invoke-WebRequest`:
Invoke-WebRequest -Uri 'https://example.com/file.zip' -OutFile 'C:\path\to\file.zip'
Example:
Invoke-WebRequest -Uri 'https://httpbin.org/image/png' -OutFile 'C:\path\to\image.png'
In this example, we download an image and store it in a specified path on our local filesystem.
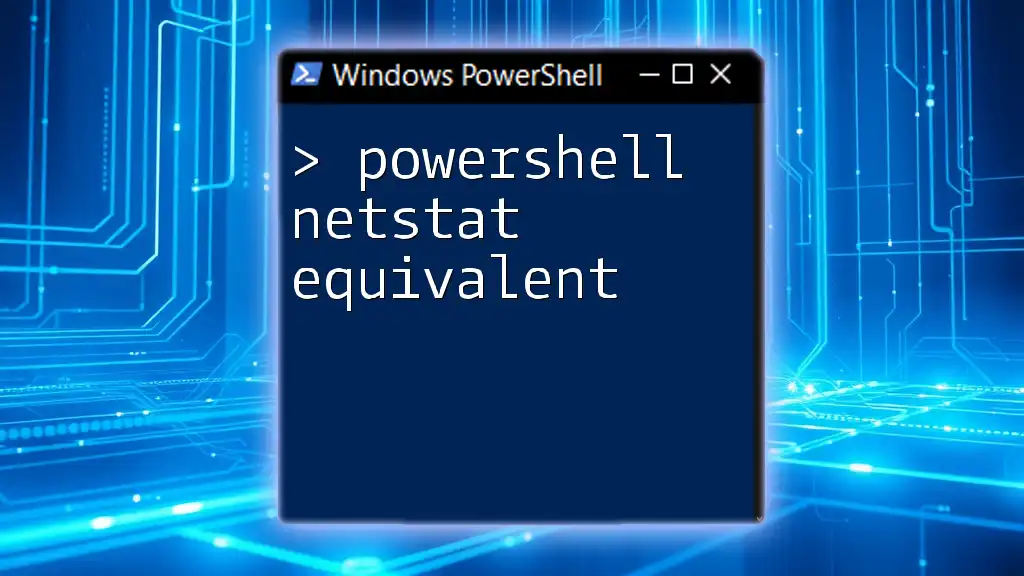
Troubleshooting Common Issues
Common Errors
When working with APIs, errors can occur. You can use error handling in PowerShell with `Try/Catch` blocks:
try {
$response = Invoke-RestMethod -Uri 'https://api.example.com/data' -Method Get
} catch {
Write-Host "Error occurred: $_"
}
This approach will catch any errors encountered during the API call and print a message to the console.
Network Issues
To check for connectivity issues, you can use `Test-NetConnection`:
Test-NetConnection -ComputerName 'api.example.com'
This cmdlet determines if a connection can be established to the given server, helping to troubleshoot connectivity problems.
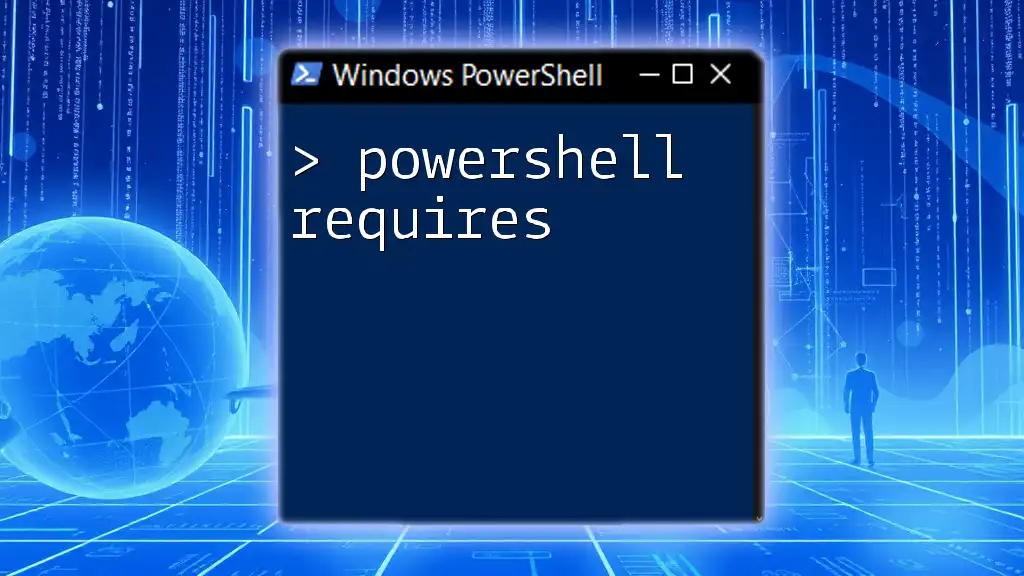
Conclusion
PowerShell serves as a robust alternative to CURL, allowing for efficient web requests through a powerful scripting language. By utilizing cmdlets like `Invoke-RestMethod` and `Invoke-WebRequest`, you can easily make GET and POST requests, handle authentication, and interact with APIs effectively. Each command is designed to prioritize ease of use and flexibility, catering to both novices and seasoned developers.
With this knowledge, we encourage you to practice the examples provided and explore the full potential of PowerShell for your scripting and automation needs.