In PowerShell, you can use the `curl` command (alias for `Invoke-WebRequest`) to send an HTTP request and view the headers using a simple one-liner.
Here’s an example code snippet to retrieve the headers from a URL:
curl -Uri "https://example.com" -Method Head | Select-Object -ExpandProperty Headers
What Are HTTP Headers?
HTTP headers are a fundamental component of the Hypertext Transfer Protocol (HTTP) communication between clients and servers. They carry essential metadata about the request being sent or the response being received. Understanding HTTP headers is crucial when working with any web-based application, including those executed in PowerShell.
Request Headers
Request headers are sent by the client to provide context about the request. They define the properties of the HTTP request and can influence how the server processes it. Some common request headers include:
- User-Agent: Identifies the client making the request, which can affect how the server responds.
- Accept: Specifies the content types that the client is willing to accept.
- Authorization: Used to provide credentials for authenticating with the server.
Response Headers
Response headers are sent back by the server and provide information regarding the server's response. They are crucial for understanding the state of the requested resource or the nature of the response. Examples include:
- Content-Type: Indicates the media type of the resource (e.g., application/json).
- Set-Cookie: Used to send cookies from the server to the client.
- Cache-Control: Directs how caching mechanisms should be managed.
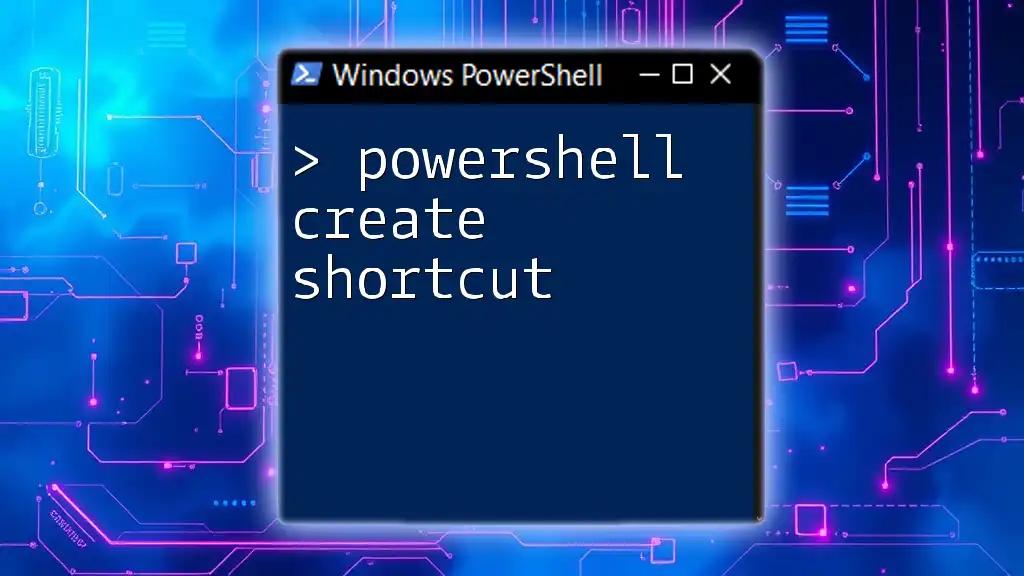
Understanding the PowerShell `curl` Command
In PowerShell, the `curl` command is an alias for `Invoke-WebRequest`, which is a powerful cmdlet designed for making web requests. The basic syntax for the command is as follows:
curl -Uri "<URL>" -Method <METHOD> -Headers <HEADERS>
Example: A simple GET request to a website may look like this:
curl -Uri "http://example.com"
Using `curl` allows you to interact with APIs, download files, or test web services directly from the PowerShell console.
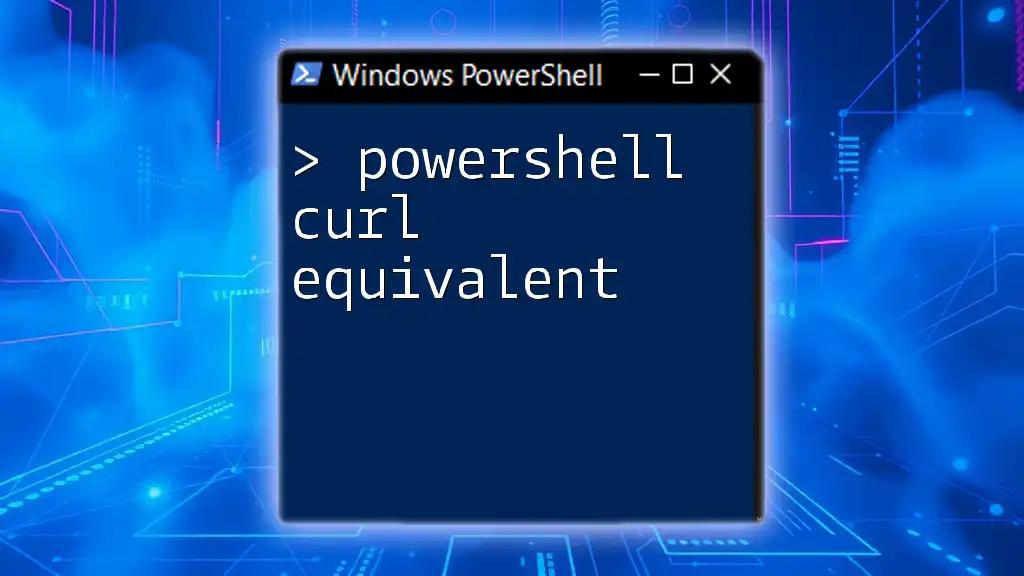
How to Set Headers Using PowerShell Curl
Setting headers in PowerShell's `curl` command is essential for customizing the request and ensuring proper communication with the server. The syntax for adding headers is straightforward:
curl -Uri "<URL>" -Headers @{ "Header-Name" = "Value" }
Example: Basic Header Setup
To illustrate the process, consider a scenario where you want to set a `User-Agent` header. Here's how you do it:
curl -Uri "http://example.com" -Headers @{ "User-Agent" = "MyPowerShellTool" }
In this example, we are informing the server that the request is coming from "MyPowerShellTool," which might influence the response received or how the server handles the request.
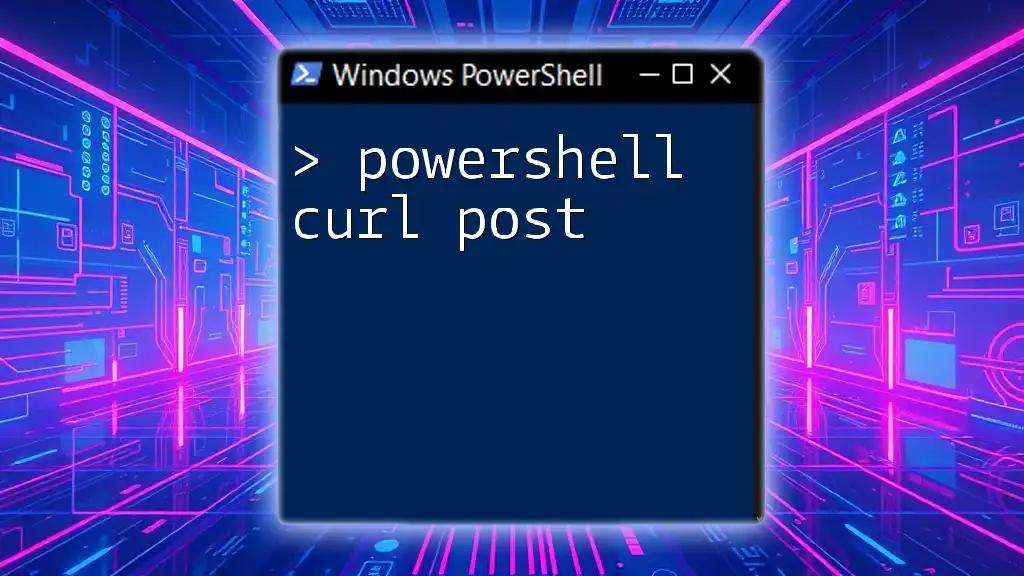
Adding Multiple Headers
You can include multiple headers in a single command by separating them with semicolons within the hashtable. This is particularly useful when dealing with APIs or servers that have specific requirements regarding multiple headers.
Example: Multi-Header Setup
Here’s how to set multiple headers in one command:
curl -Uri "http://example.com" -Headers @{
"User-Agent" = "MyPowerShellTool";
"Authorization" = "Bearer TOKEN"
}
In this example, we are not only setting the `User-Agent` header but also providing an `Authorization` token. By including essential headers, we increase the likelihood of a successful request.
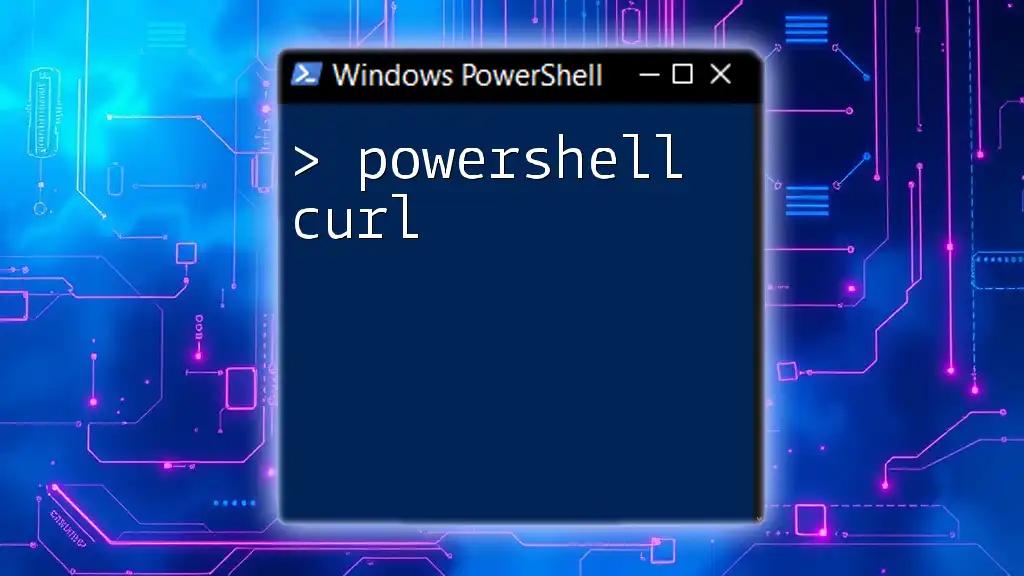
Viewing Response Headers
After sending a request, it is often necessary to inspect the response headers to understand what information the server has returned. PowerShell allows you to easily access these headers.
Example: Capturing Response Headers
You can store the server’s response in a variable and then view the headers like this:
$response = curl -Uri "http://example.com" -Headers @{ "User-Agent" = "MyPowerShellTool" }
$response.Headers
This command captures the response into the `$response` variable, allowing us to explore its properties, including headers. This provides valuable insights into the server's response behavior.
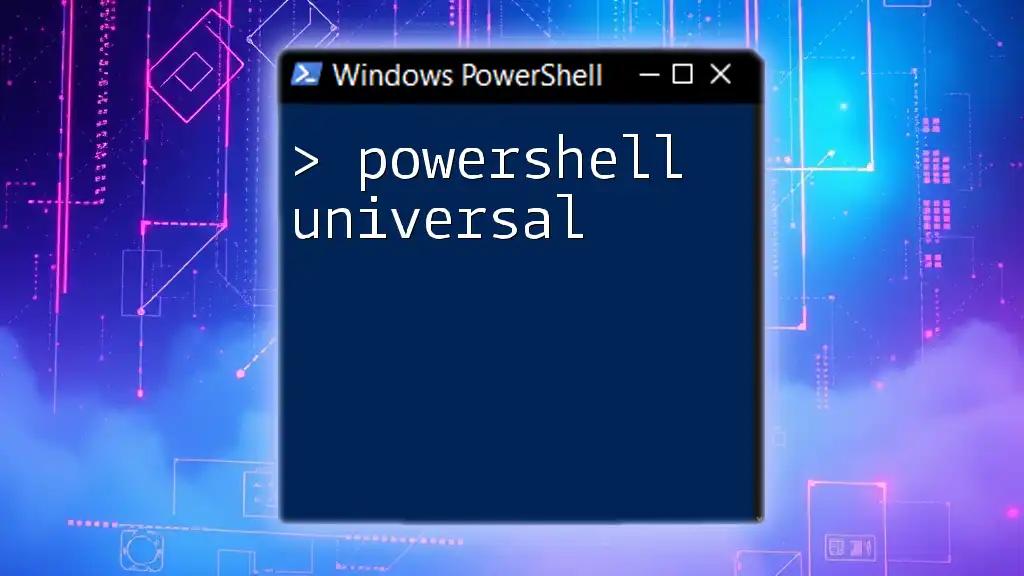
Common Use Cases for Setting Headers
Understanding `powershell curl headers` becomes particularly important in various real-world scenarios. Some common use cases include:
- REST APIs: Many APIs require specific headers, including authentication tokens or content type specifications. Customizing headers can allow you to seamlessly interact with these services.
- OAuth Authentication: When dealing with OAuth, headers play a crucial role in authorizing requests. Providing the correct authorization header is essential for gaining access.

Troubleshooting Common Issues
While using `curl` in PowerShell, users may encounter several common errors, often related to headers. Here are some common headaches and how to mitigate them:
- Incorrectly formatted headers can lead to 401 Authorization errors.
- Missing required headers can result in 400 Bad Request errors.
Example: Debugging a Failed Request
To debug ineffective requests, leverage PowerShell's verbose output feature. It provides detailed information about the request and response, which is extremely helpful for troubleshooting.
curl -Uri "http://example.com" -Headers @{ "User-Agent" = "MyPowerShellTool" } -Verbose
This command gives you a more comprehensive view of what's happening behind the scenes during the request, helping you identify where things went wrong.
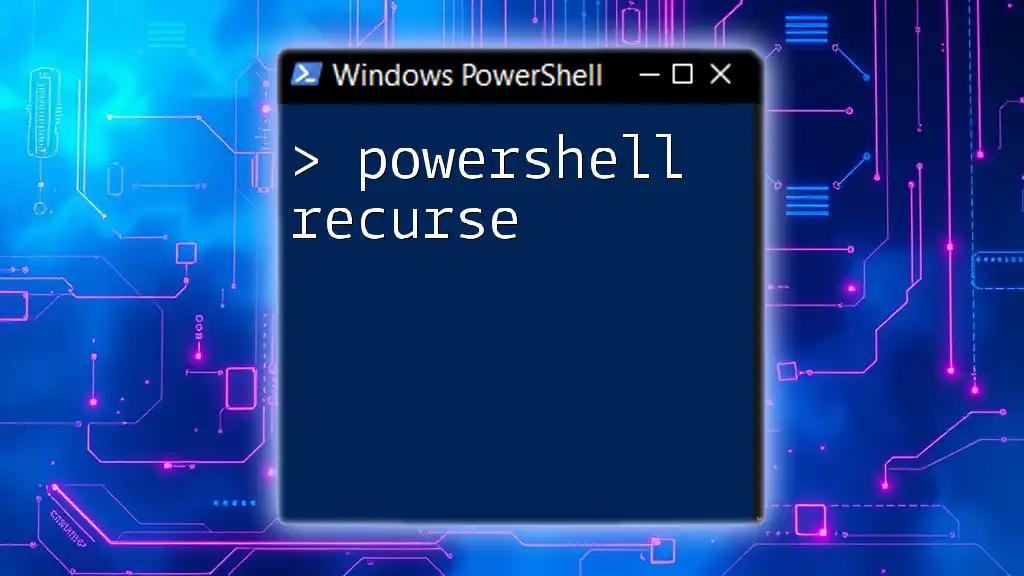
Conclusion
Understanding `powershell curl headers` is critical for anyone looking to harness the full power of web requests in PowerShell. By diving into what headers are, how to set them, and common practices when dealing with APIs, you empower yourself with the tools to navigate and manage HTTP communications effectively. Now is the time to experiment further with more advanced headers and commands to expand your PowerShell capabilities.
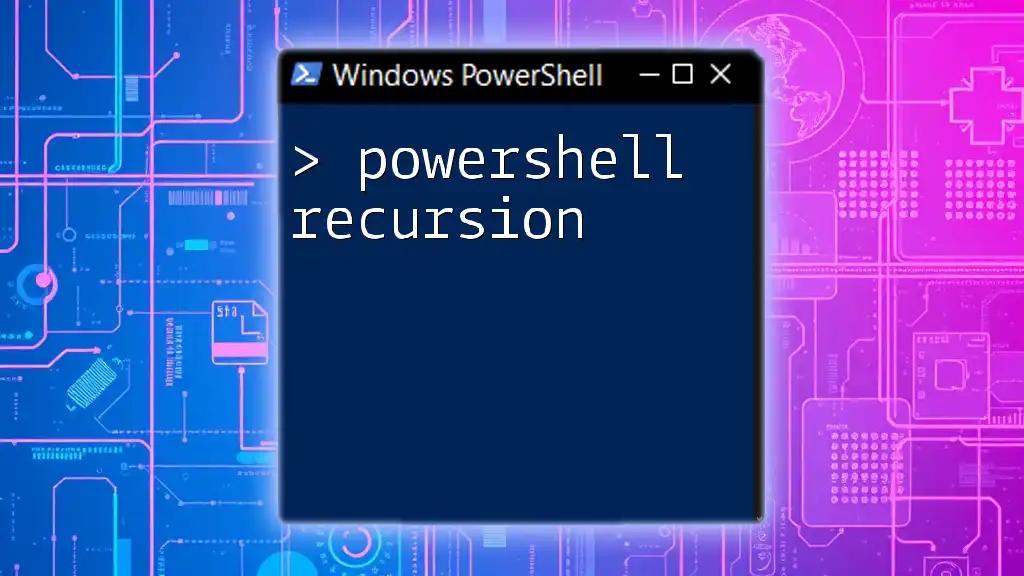
Additional Resources
For further learning, explore the official documentation on `Invoke-WebRequest` and consider reading about HTTP protocols to deepen your understanding of web communications. With practice, you will become proficient in using headers to refine and enhance your PowerShell scripting skills.