In PowerShell, you can use the `curl` command (which is an alias for `Invoke-WebRequest`) to send a POST request to a specified URL with data, as shown in the following example:
curl -Uri "https://example.com/api" -Method Post -Body @{ param1="value1"; param2="value2" } -ContentType "application/x-www-form-urlencoded"
Understanding cURL in the Context of PowerShell
What is cURL?
cURL is a command-line tool and library for transferring data with URLs. It supports numerous protocols, allowing users to interact with web servers by sending and receiving data over the internet. In particular, cURL is frequently used for making HTTP requests, which are central to web applications and APIs.
Why Use PowerShell for cURL Operations?
PowerShell provides several advantages when working with cURL operations:
- Integration: PowerShell is versatile and can easily integrate with other scripts and applications.
- Object-Oriented: Unlike traditional cURL commands that return raw data, PowerShell's `Invoke-RestMethod` and `Invoke-WebRequest` return .NET objects, allowing for more straightforward manipulation and access to data.
- Ease of Use: PowerShell syntax is often considered more accessible for scripts and administrative tasks in Windows environments.
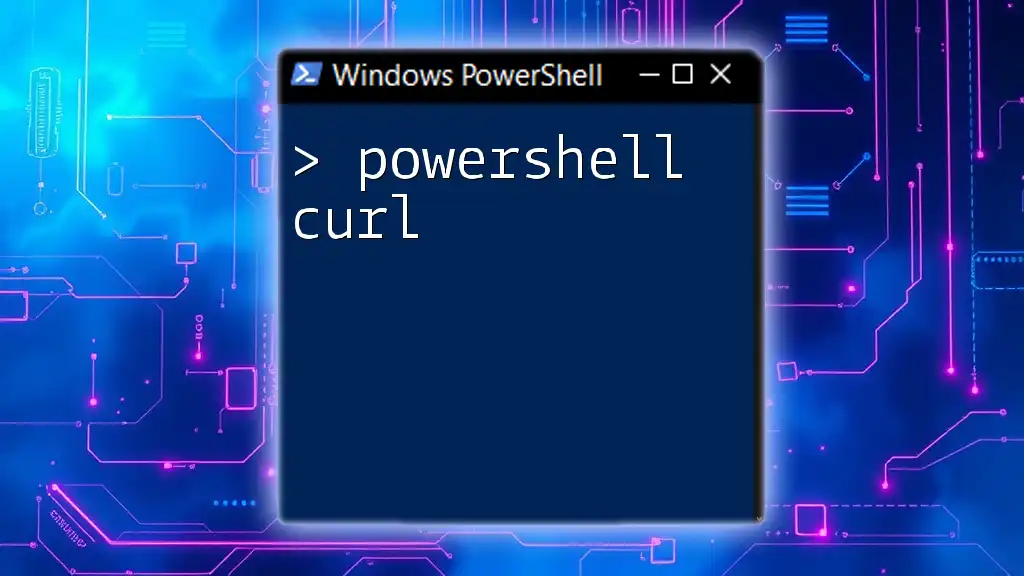
Setting Up Your PowerShell Environment
Prerequisites
Before diving into using PowerShell for cURL operations, ensure you're using a compatible version of PowerShell. PowerShell 3.0 or later is recommended for the best support for web requests. You typically don't need an additional cURL installation on Windows systems; PowerShell's built-in commands are sufficient for most use cases.
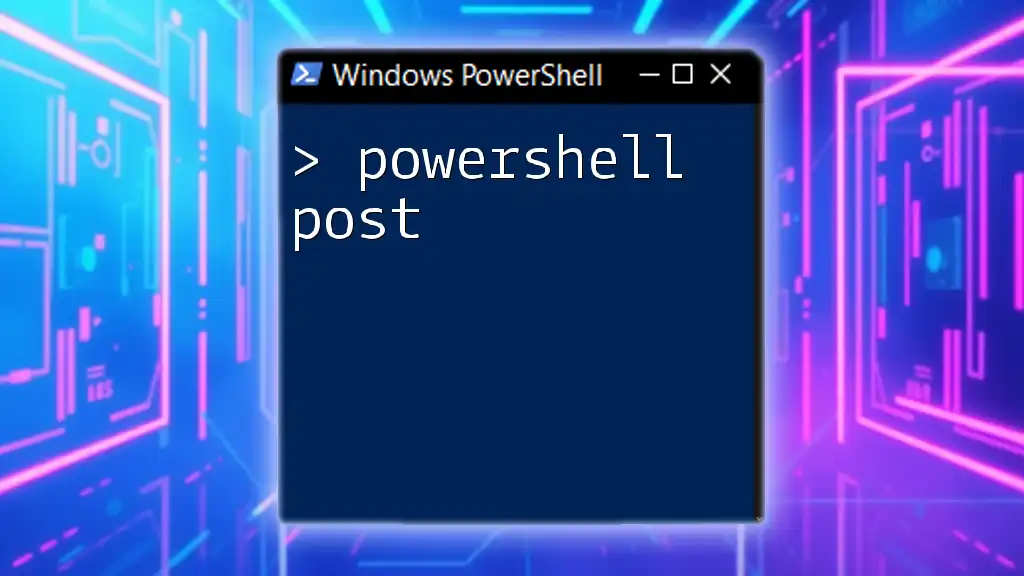
Making a POST Request with PowerShell
Introduction to POST Requests
In HTTP, a POST request is used to send data to a server. This data usually includes form data or JSON, which the server processes and responds to accordingly. Unlike GET requests, which retrieve data from a server, POST requests are commonly used for creating or updating resources.
Using Invoke-RestMethod for POST
Syntax Overview
The `Invoke-RestMethod` cmdlet is a PowerShell command specifically designed for working with REST APIs. Here’s its basic syntax:
Invoke-RestMethod -Uri <Uri> -Method <Method> -Body <Body> -Headers <Headers>
Example: Basic POST Request
To make a straightforward POST request using `Invoke-RestMethod`, you can use the following code:
$Uri = "https://example.com/api"
$Body = @{ key1 = "value1"; key2 = "value2" }
Invoke-RestMethod -Uri $Uri -Method Post -Body $Body
In this example:
- `$Uri` specifies the API endpoint.
- `$Body` holds the data sent to the server. It is defined as a hashtable, which will be converted to form URL-encoded format.
- The response will be outputted directly into the console.
Using Invoke-WebRequest for POST
Syntax Overview
The `Invoke-WebRequest` cmdlet differs slightly from `Invoke-RestMethod`, mainly returning raw response data rather than deserialized objects. Use it when you need detailed response information, such as headers or status codes.
Example: Basic POST Request
Here's how to perform a POST request with `Invoke-WebRequest`:
$Uri = "https://example.com/api"
$Body = @{ key1 = "value1"; key2 = "value2" }
Invoke-WebRequest -Uri $Uri -Method Post -Body $Body
This command works similarly to the previous example, but it is especially useful when you need additional details about the HTTP response.
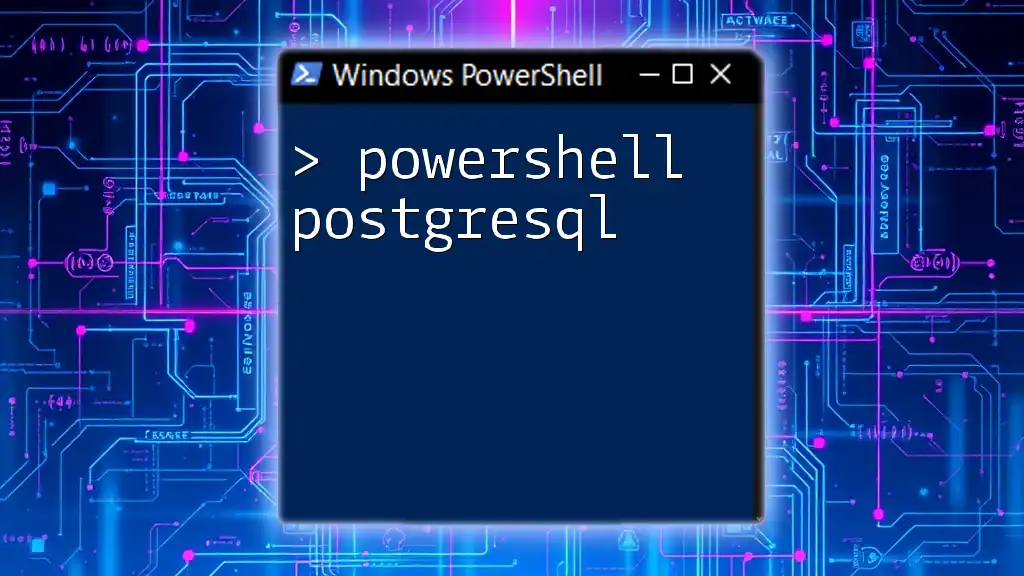
Handling JSON Data
Sending JSON Data in a POST Request
Many APIs expect data in JSON format rather than URL-encoded strings. PowerShell makes it easy to convert data structures to JSON.
Creating and Converting Data to JSON
To create JSON, use the `ConvertTo-Json` cmdlet:
$Body = @{ key1 = "value1"; key2 = "value2" } | ConvertTo-Json
Making a POST request with JSON
Once you have your JSON, making a POST request is straightforward:
$Uri = "https://example.com/api"
Invoke-RestMethod -Uri $Uri -Method Post -Body $Body -ContentType "application/json"
Setting the `ContentType` to `"application/json"` informs the server of the format being sent, which is crucial for proper data handling.
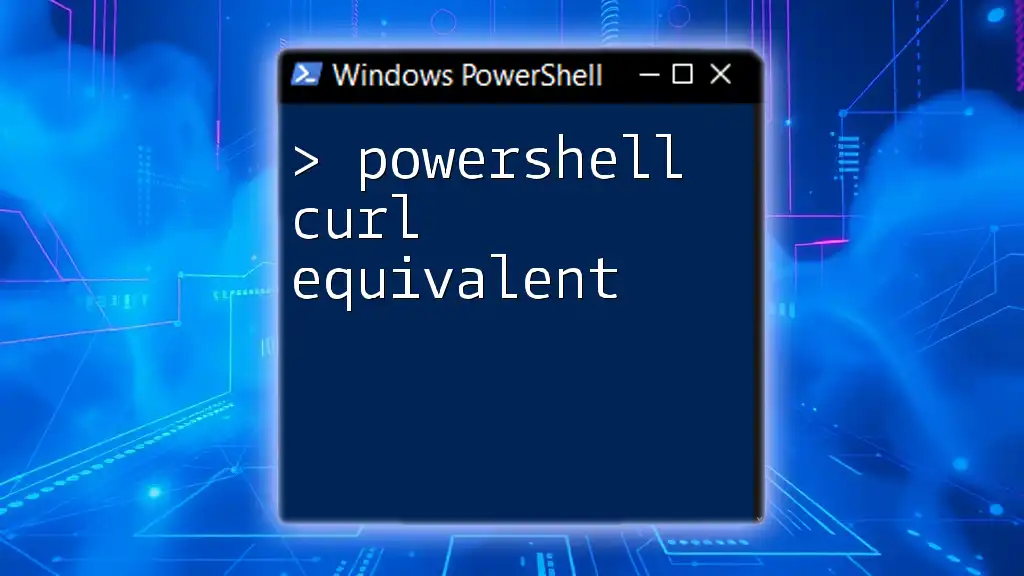
Adding Headers to Your Requests
Custom Headers
In many cases, APIs require headers for authentication, such as an API key or bearer token, or to define the accepted response format.
Example: Adding Headers
To add headers to your POST request, use the `-Headers` parameter:
$Headers = @{ Authorization = "Bearer token"; "Accept" = "application/json" }
Invoke-RestMethod -Uri $Uri -Method Post -Body $Body -Headers $Headers
By customizing headers in this way, you can tailor your requests to meet specific API requirements.
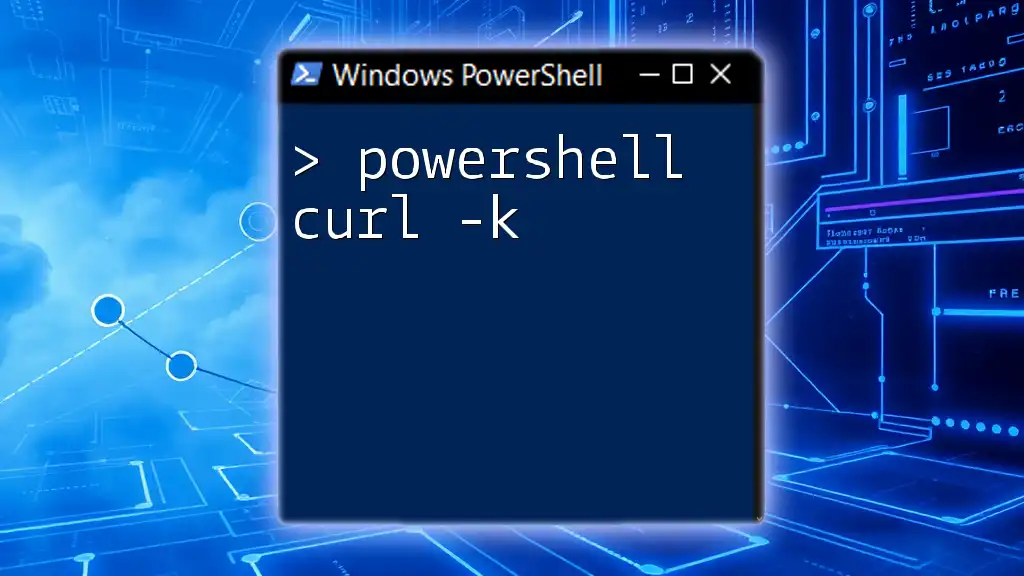
Error Handling
Understanding HTTP Status Codes
When making API calls, it's essential to understand the response status codes. Common codes include:
- 200 OK: The request succeeded.
- 400 Bad Request: There was an error in the request format.
- 401 Unauthorized: Authentication failed or the user doesn't have the necessary permissions.
- 500 Internal Server Error: A generic error occurred on the server side.
Using Try-Catch for Error Management
Using PowerShell’s `try-catch` block is an effective way to catch errors during requests:
try {
$response = Invoke-RestMethod -Uri $Uri -Method Post -Body $Body -Headers $Headers
Write-Output $response
} catch {
Write-Error "Error occurred: $_"
}
This block will handle any exceptions thrown by the command, allowing for more resilient scripts.
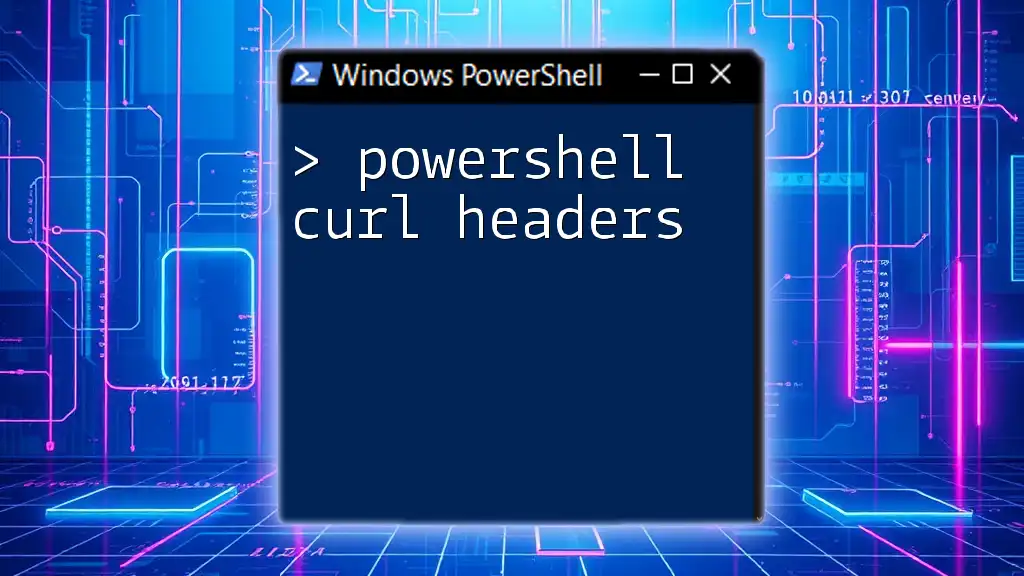
Testing and Debugging Your POST Requests
Using the PowerShell ISE or VS Code
Utilizing environments like PowerShell ISE or Visual Studio Code can simplify debugging with features like syntax highlighting and command suggestions.
How to Debug Your Requests
When testing your requests, inspect the returned variable. You can log the response or check properties directly, using commands like:
$response.StatusCode
$response.Content
These insights can help identify issues with your requests and the server’s responses.
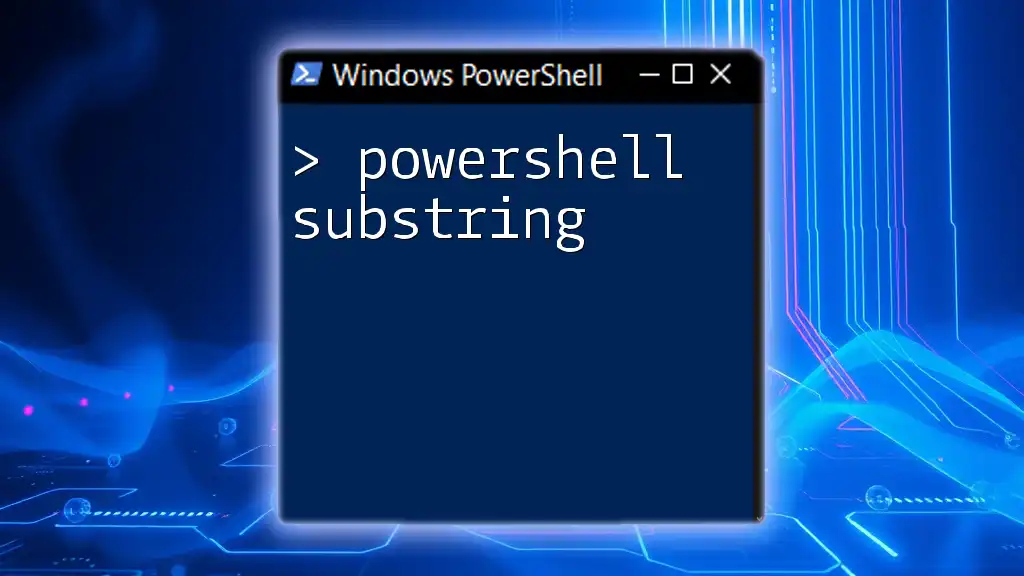
Best Practices for Using PowerShell cURL
Security Considerations
When dealing with sensitive information such as API keys, always prioritize security. Store such data securely, avoiding hardcoding them in scripts. Using natural PowerShell features like `Get-Credential` can help manage these connections securely.
Code Reusability
To unify your approach, create functions for common API interactions. Here’s a sample function for reusable POST requests:
function Invoke-MyPost {
param (
[string]$Uri,
[hashtable]$Body,
[hashtable]$Headers = @{}
)
$BodyJson = $Body | ConvertTo-Json
Invoke-RestMethod -Uri $Uri -Method Post -Body $BodyJson -Headers $Headers -ContentType "application/json"
}
This function simplifies making requests across multiple scripts or sessions, enhancing maintainability and reducing redundancy.
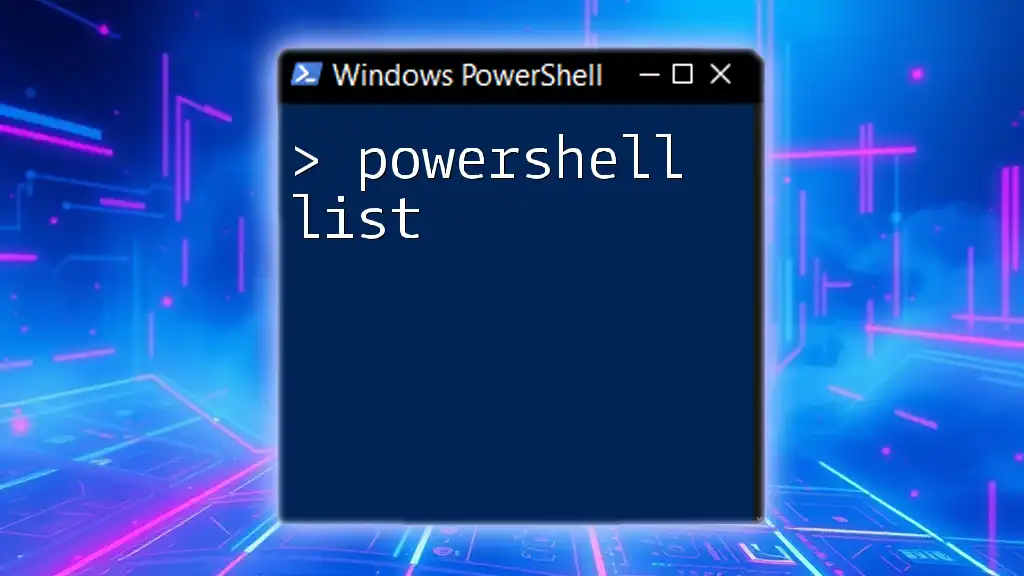
Conclusion
In this guide, we've explored how to handle `PowerShell cURL POST` operations using PowerShell's native cmdlets. By mastering the nuances of making POST requests, working with JSON, handling headers, and managing errors, you can efficiently interact with web APIs and automate various tasks.
For further learning, don’t hesitate to explore the extensive resources available in the PowerShell community. Practice is key to perfecting these skills and enhancing your productivity with PowerShell.