To pause a PowerShell script before it exits, you can use the `Read-Host` cmdlet to wait for user input, as shown in the code snippet below:
Read-Host -Prompt 'Press Enter to exit'
Why Use Pause Before Exit?
Using a pause before exit in PowerShell scripts is essential for a few key reasons. One of the primary motivations for incorporating a pause is ensuring output visibility. When scripts are executed in a command-line interface, the console window often closes immediately after the script finishes running. If there are important outputs or error messages, users may miss them entirely. By adding a pause, you give users the opportunity to see and understand what's happening before the window disappears.
Furthermore, using a pause improves user feedback. It allows for an interactive experience where users can engage with the script, decide if they want to continue or exit, and understand how the script operates. This crucial interaction can enhance the overall user experience and make troubleshooting easier if something goes wrong.
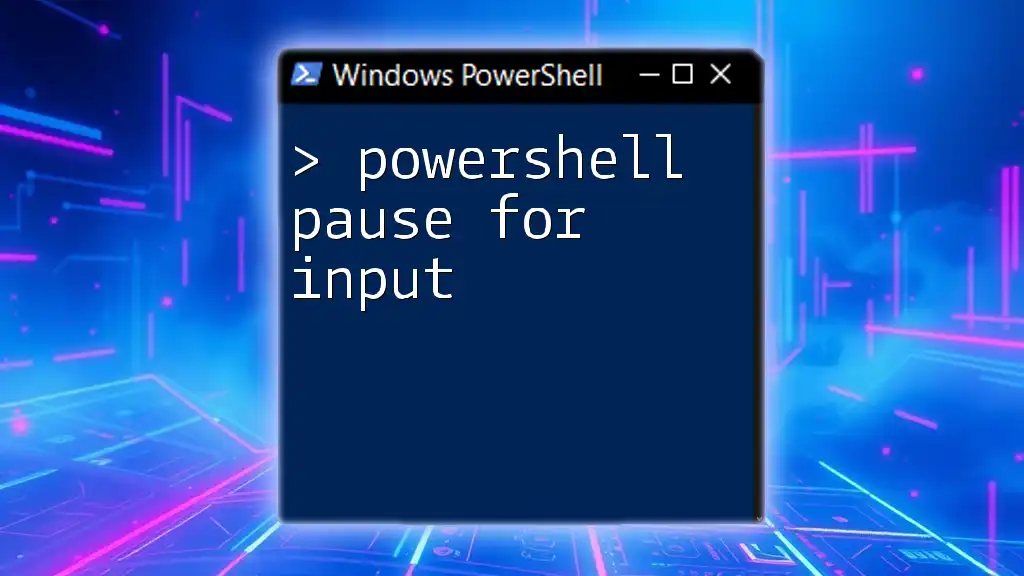
How to Implement Pause in PowerShell
Basic Pause Command
The simplest way to implement a pause in PowerShell is through the `Read-Host` cmdlet. This command can halt script execution until the user provides input, which is typically by pressing the Enter key. Here's a straightforward example:
Write-Host "Press Enter to exit..."
Read-Host
In this snippet, when the script reaches the `Read-Host` command, it will prompt users to press Enter, effectively pausing execution at that point.
More User-Friendly Options
Using a Custom Function
Creating a custom function for pausing can encapsulate this functionality effectively, making your script clearer and more reusable. Here's how you can define a `Pause` function:
function Pause {
Write-Host "Press any key to continue..."
$null = $Host.UI.RawUI.ReadKey("NoEcho,IncludeKeyDown")
}
This function will display a message prompting the user to continue. The `ReadKey` method captures any key press, leading to a user-friendly interface. To use this function in your scripts, simply call `Pause` whenever you want to introduce a break.
Prompting for User Response
Another option is to prompt the user for a specific response before exiting the script, allowing them to make a choice. Here’s how you might implement this:
$response = Read-Host "Do you want to exit? [Y/N]"
if ($response -eq 'Y') { exit }
With this snippet, users can decide whether to end the script based on their input. If they type 'Y', the script will exit. Otherwise, it will continue executing. This approach adds flexibility and control for the user.
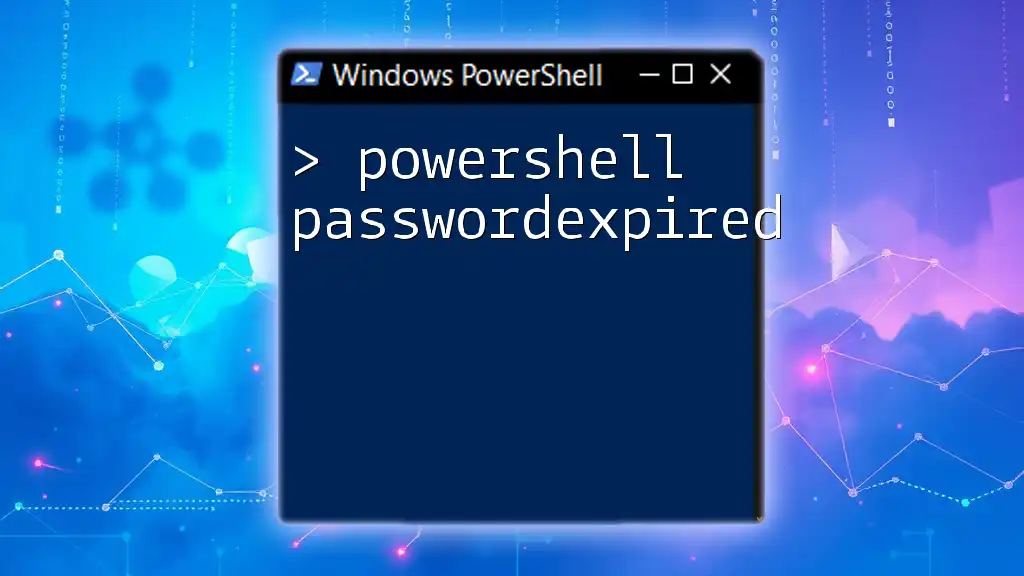
Advanced Techniques for Pausing Execution
Using Sleep for Delayed Exit
Sometimes, a timed pause can be useful, especially in cases where you may want to provide information before the script closes automatically. You can achieve this with the `Start-Sleep` cmdlet:
Write-Host "The script will exit in 10 seconds..."
Start-Sleep -Seconds 10
This example will display a message and then pause for ten seconds before proceeding. This gives users a chance to digest the output before the script finishes.
Error Handling with Pauses
Incorporating pauses during error handling is another effective way to maintain visibility. For instance, if a script encounters an exception, you can pause execution to allow the user to see what went wrong:
try {
# Code that may throw an error
} catch {
Write-Host "An error occurred: $_"
Read-Host "Press Enter to exit..."
}
In this snippet, if an error occurs, the except block runs, displaying the error message and pausing for user input. This design helps in understanding issues and can make debugging significantly easier.
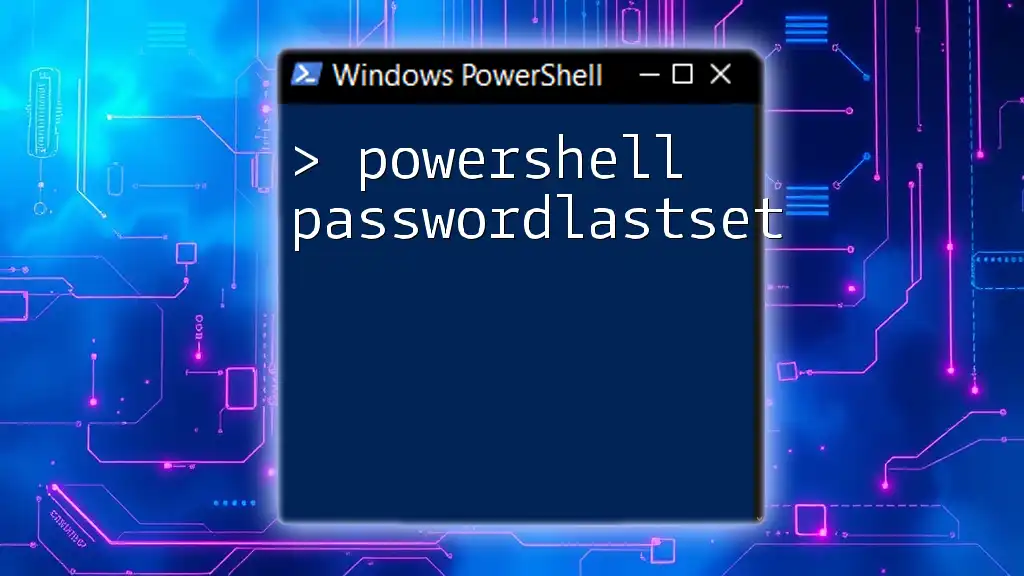
Best Practices
Consistency in Script Design
Maintaining a consistent approach to pauses across different scripts is vital. A coherent scripting style helps users feel comfortable and familiar with your scripts. Consistency ensures that users will know where and when they can expect pauses, allowing for a smoother interaction.
Providing Clear Instructions to Users
When prompting users, clarity is key. Ensure your prompts are concise and informative. This can minimize confusion and improve user experience. Using clear and straightforward language like "Press Enter to continue..." or "Do you want to exit? [Y/N]" can directly enhance the effectiveness of your script.
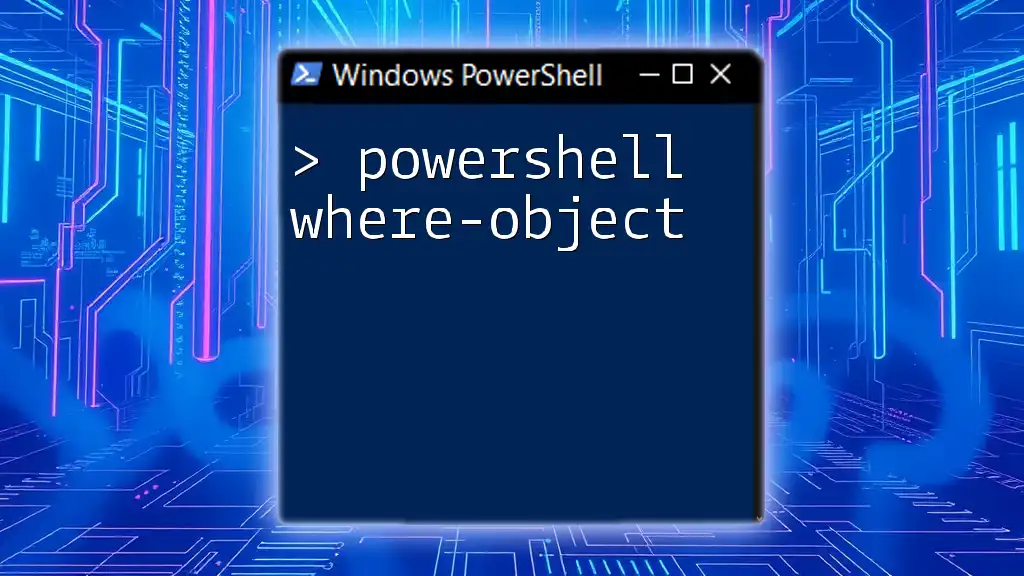
Troubleshooting Common Issues
Script Not Pausing
Occasionally, users might find that their script does not pause as expected. Common reasons might include missing the pause command or misconfigurations in the coding blocks. If `Read-Host` is not functioning, double-check that the script is executing properly.
User Confusion with Input Options
Another issue may occur if the prompts are unclear. If users are unsure about what input is necessary, this can lead to frustration. It's crucial to provide unambiguous options and feedback to guide them through their choices.
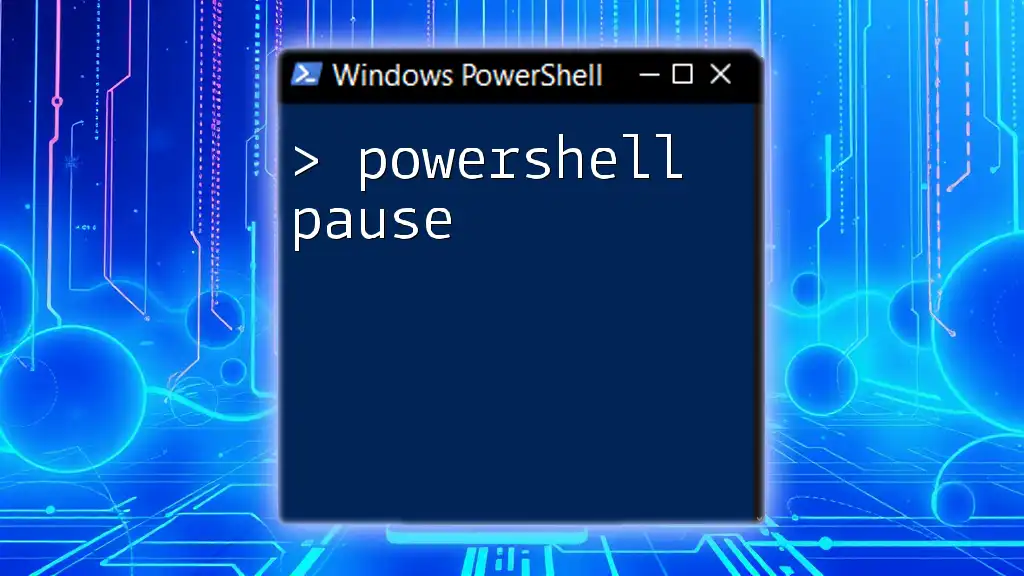
Conclusion
In summary, understanding how to implement a PowerShell pause before exit is vital for improving the usability and functionality of your scripts. By utilizing commands like `Read-Host`, creating custom functions, and effectively managing user prompts, you can enhance interaction and prevent window closures that might obscure valuable information. Encouraging practice with these techniques will empower you to build more user-friendly PowerShell scripts that cater to both novice and experienced users.
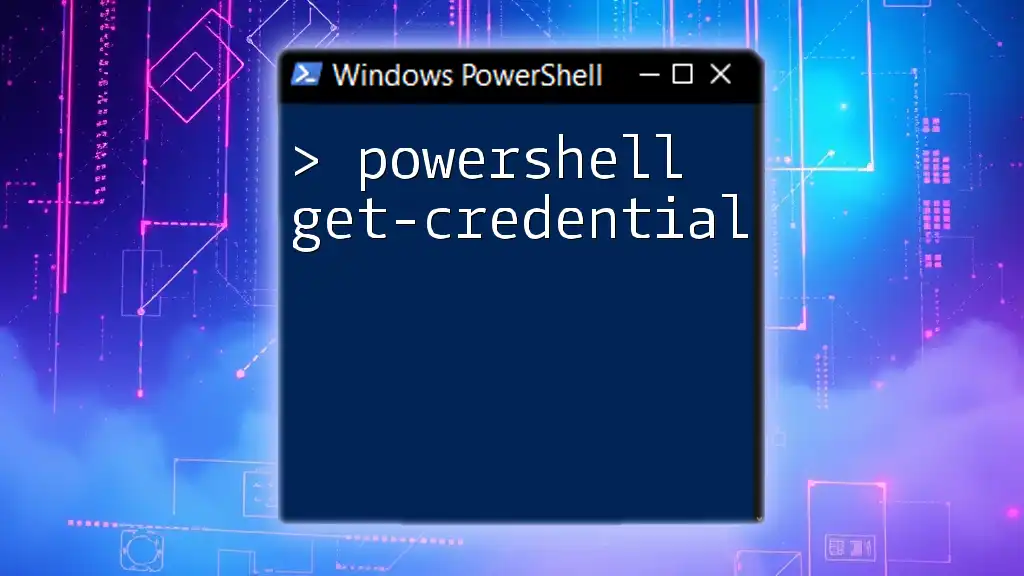
Additional Resources
To further your knowledge, exploring the PowerShell documentation can provide deeper insights into automation techniques and best practices. Various online learning platforms also offer excellent tutorials emphasizing practical uses of pausing commands in PowerShell, equipping you with the skills needed for real-world applications.