The `ssh-copy-id` command is used to install your SSH public key on a remote server, allowing for passwordless authentication when connecting via SSH.
ssh-copy-id user@remote_host
Understanding SSH Key Authentication
What are SSH Keys?
SSH keys are a pair of cryptographic keys used to authenticate a secure connection between a client and a server. Each pair consists of a public key and a private key. The public key is stored on the server in a special file, while the private key remains with the client. This method enhances security by allowing users to connect to remote servers without needing to transmit passwords over the network.
Benefits of Key-Based Authentication
Using SSH keys provides numerous advantages:
- Enhanced Security: The encryption provided by SSH keys makes unauthorized access considerably more difficult compared to traditional password authentication.
- Convenience: Once set up, key-based authentication allows for seamless logins without entering passwords. This is particularly beneficial for automated scripts and routines.
- Prevention of Brute Force Attacks: Since SSH keys are virtually impossible to guess or crack, they effectively fend off brute force attacks aimed at password-protected logins.
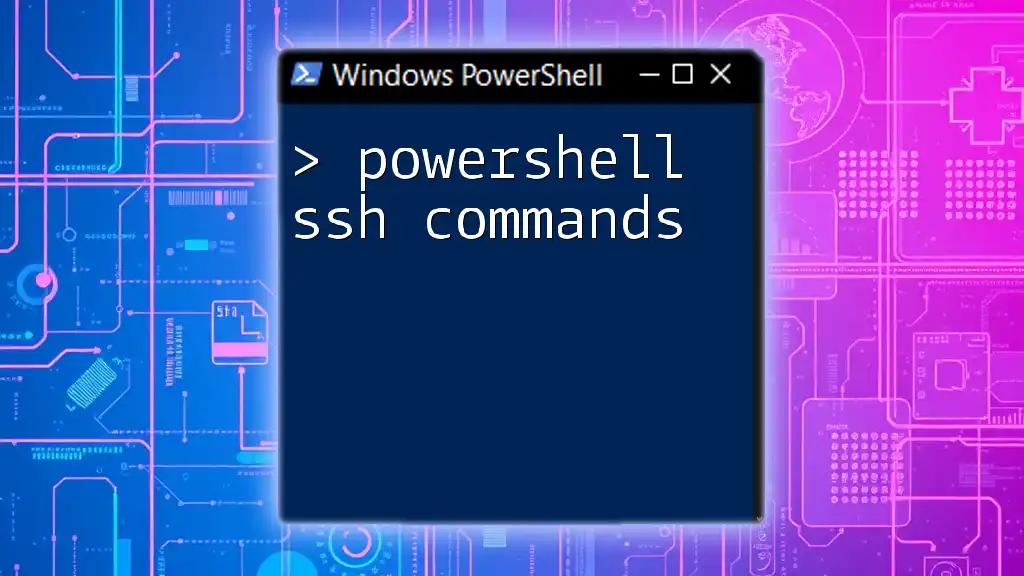
Setting Up PowerShell for SSH
Installing OpenSSH
To utilize the `powershell ssh-copy-id` functionality, you need to ensure that OpenSSH is installed on your Windows system. You can do this through the Settings app:
- Open Settings > Apps > Optional features.
- Look for OpenSSH Client. If it's not listed, click on Add a feature, search for OpenSSH Client, and install it.
After installation, verify it by executing the following command in PowerShell:
ssh -V
This command will display the version of SSH installed, confirming a successful installation.
Generating SSH Keys
The first step in setting up key-based authentication is to generate SSH keys. You can do this by using the `ssh-keygen` command in PowerShell. Follow these steps:
- Open PowerShell.
- Enter the following command to generate a new SSH key pair:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
This command will generate two files in the default location (`%USERPROFILE%\.ssh\`):
- `id_rsa`: Your private key (keep this secure and do not share it).
- `id_rsa.pub`: Your public key (this is what you'll send to servers for authentication).
Where to Find SSH Keys
You can locate your SSH keys in the `.ssh` directory within your user profile:
C:\Users\YourUsername\.ssh\
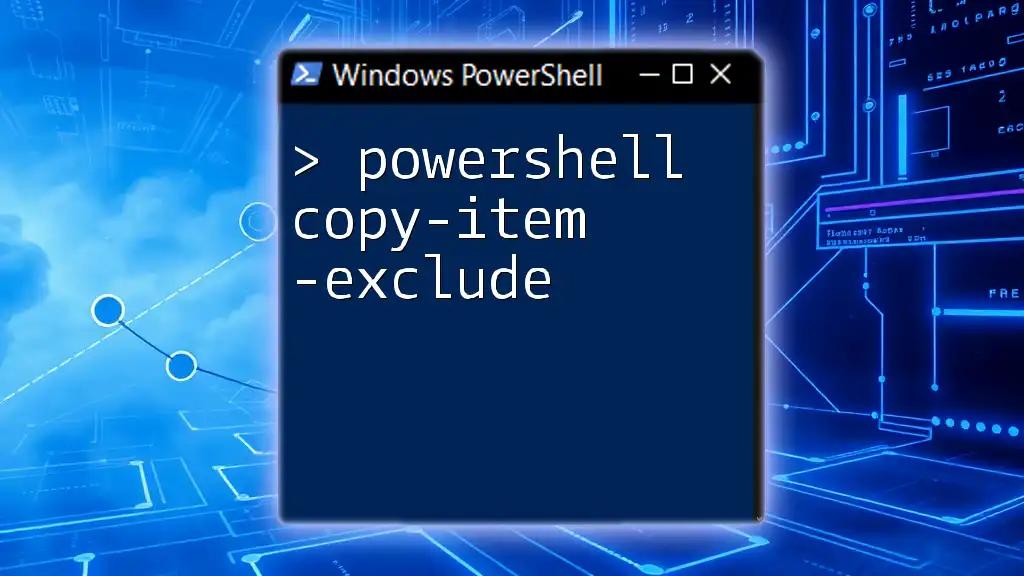
Introduction to `ssh-copy-id`
What is `ssh-copy-id`?
The `ssh-copy-id` command is a convenient utility to install your public key onto a remote server’s authorized keys. By doing so, you can establish a password-less SSH connection to that server.
Limitations of `ssh-copy-id` in PowerShell
While `ssh-copy-id` is a native command in Unix-like systems, PowerShell does not support it directly. However, you can simulate its functionality through a manual approach in PowerShell, which we'll explore next.
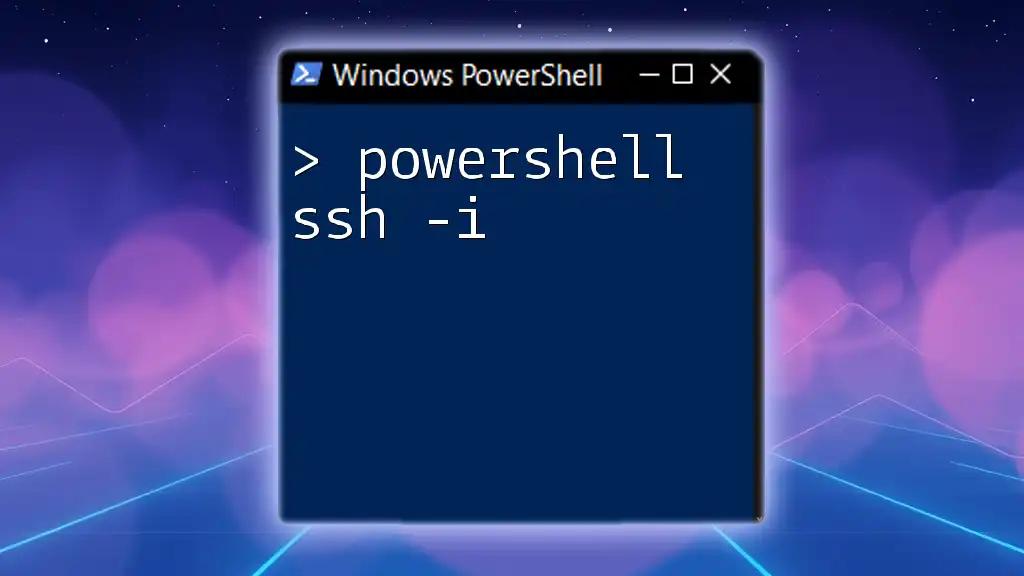
Using `ssh-copy-id` in PowerShell
Simulating `ssh-copy-id` Functionality
To implement the functionality of `ssh-copy-id` in PowerShell, you need to manually copy the public key to the remote server. The command below demonstrates this process:
cat ~/.ssh/id_rsa.pub | ssh user@remote_host "mkdir -p ~/.ssh && cat >> ~/.ssh/authorized_keys"
Explanation of Each Command in the Snippet:
- `cat ~/.ssh/id_rsa.pub`: Reads your public key file.
- `|`: Pipes the output of the previous command into the next one.
- `ssh user@remote_host`: Initiates an SSH session to the specified user and host.
- `"mkdir -p ~/.ssh"`: Creates the `.ssh` directory on the remote server (if it does not exist).
- `cat >> ~/.ssh/authorized_keys`: Appends the public key to the `authorized_keys` file, allowing for key-based authentication.
Verifying Key-Based Authentication
After copying the SSH key, test the connection to ensure that it works without a password prompt. You can do this by executing:
ssh user@remote_host
If everything is set up correctly, you should be logged in without requiring a password. If you encounter issues, check for common mistakes such as incorrect permissions or misconfiguration.
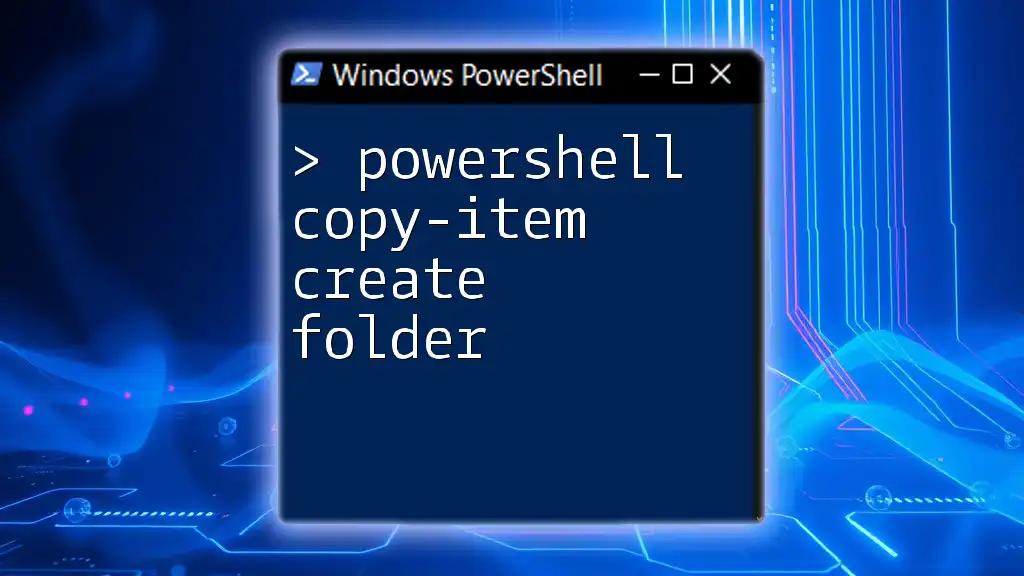
Practical Examples
Example Scenario: Copying Keys to a Remote Server
Assuming you have a remote server (`remote_host`) and a user (`user`), use the provided command to transfer your key:
cat ~/.ssh/id_rsa.pub | ssh user@remote_host "mkdir -p ~/.ssh && cat >> ~/.ssh/authorized_keys"
Once executed, you'll be prompted for your password for the last time. Upon successful connection, verifying key-based authentication should grant you password-less access.
Example Scenario: Automating Key Copy with PowerShell Scripts
To further streamline the process, you can automate the key copying with a PowerShell script. Below is a sample script:
# Save as Copy-SSHKeys.ps1
param (
[string]$User,
[string]$Host
)
$keyPath = "$HOME\.ssh\id_rsa.pub"
if (Test-Path $keyPath) {
$key = Get-Content $keyPath
ssh $User@$Host "mkdir -p ~/.ssh && echo $key >> ~/.ssh/authorized_keys"
} else {
Write-Error "SSH public key not found!"
}
Explanation of the Script:
- `param`: Accepts parameters for the username and host to which the key is being copied.
- `Test-Path`: Checks if the public key file exists.
- `Get-Content`: Reads the public key from the specified file.
- `ssh $User@$Host`: Executes the SSH command to append the key.
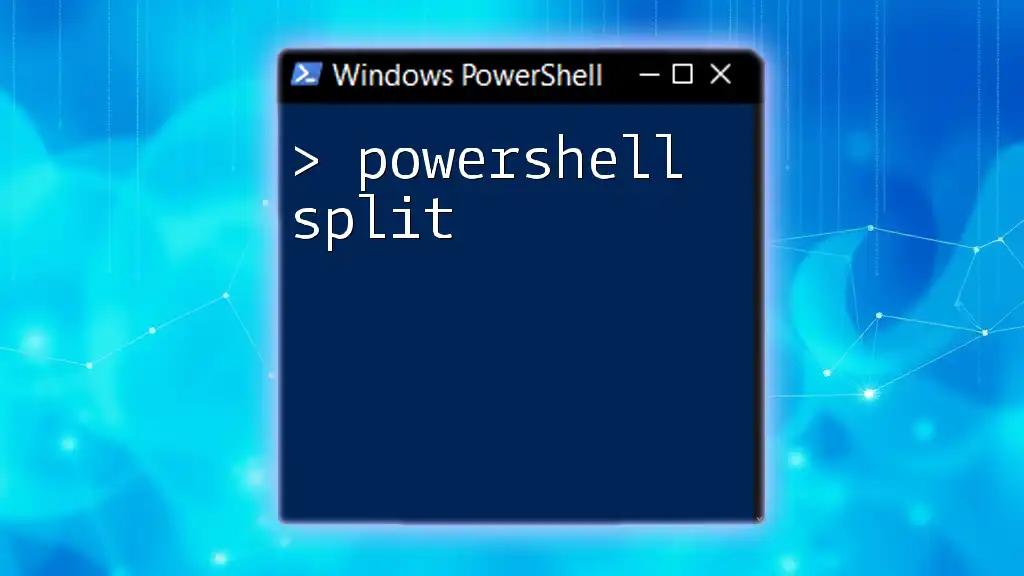
Troubleshooting Common Issues
Common Errors with SSH Key Authentication
While setting up SSH key authentication, you may encounter various errors, such as:
- Permission denied errors: Often occurs due to incorrect permissions on the `.ssh` directory or the `authorized_keys` file.
- SSH key not recognized: This can happen if the public key isn’t properly added or has incorrect formatting.
Fixing Permissions of `.ssh` Directory
To ensure proper permissions, you can set the correct permissions directly on the remote server using:
ssh user@remote_host "chmod 700 ~/.ssh; chmod 600 ~/.ssh/authorized_keys"
This command makes the `.ssh` directory accessible only to the user and secures the `authorized_keys` file.
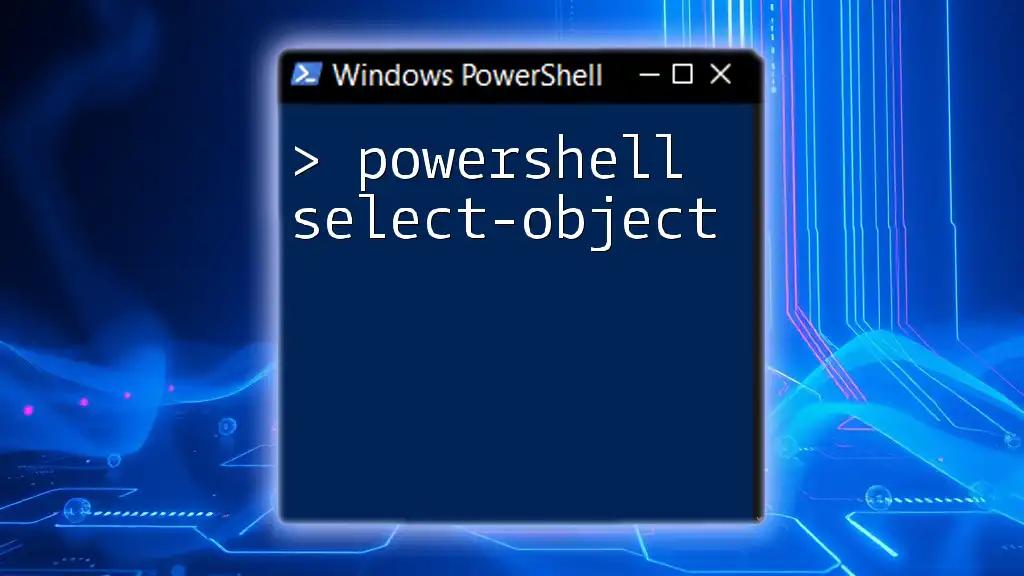
Tips and Best Practices
Protecting Your SSH Keys
Managing your SSH keys securely is crucial. Consider implementing the following practices:
- Use a Passphrase: Protect your private key with a strong passphrase to add an extra security layer.
- Backup Your Keys: Always keep a secure backup of your SSH keys in a safe location.
Rotating SSH Keys Regularly
Regularly updating and rotating your SSH keys is essential to maintaining a robust security posture. Implement this practice as part of your routine system maintenance.
Using SSH Config File for Convenience
You can simplify your SSH connection methods by using an SSH config file (`~/.ssh/config`). Here’s an example entry:
Host myserver
HostName remote_host
User user
IdentityFile ~/.ssh/id_rsa
This configuration allows you to connect using a shorthand command (`ssh myserver`), improving efficiency.
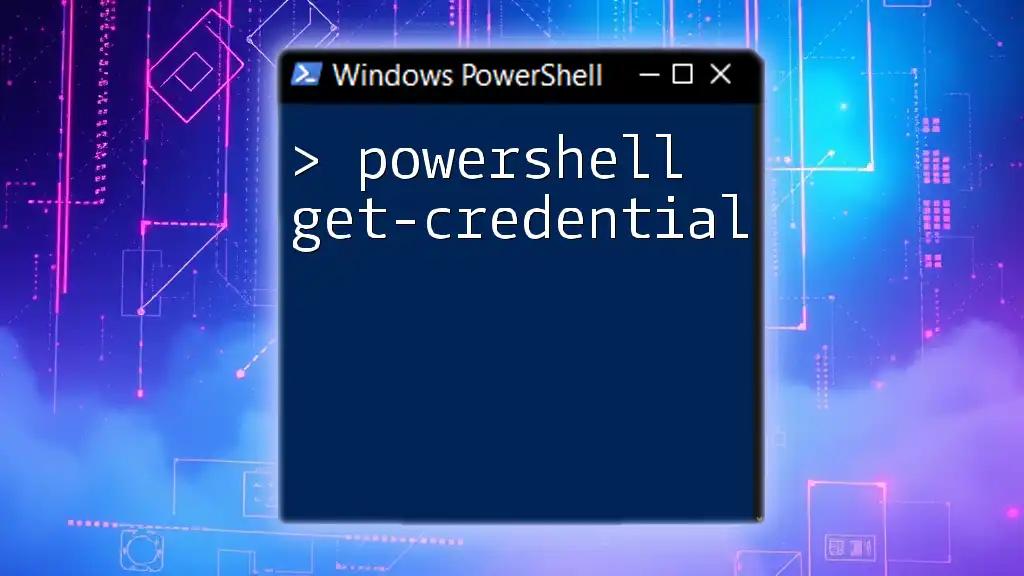
Conclusion
In this article, we explored how to implement `powershell ssh-copy-id` functionality. By leveraging the PowerShell commands and understanding SSH key authentication mechanisms, you can enhance your network operations and secure your connections effectively. Start applying these principles today for a more secure SSH experience!
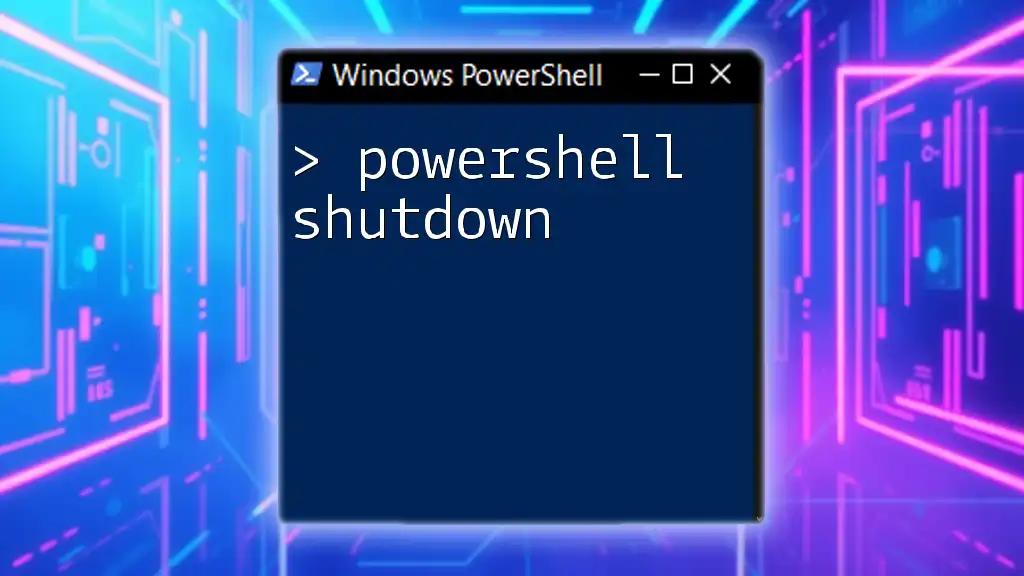
Resources
For further reading, consider checking the official PowerShell and OpenSSH documentation. Engaging with community forums can also provide additional support and insights as you deepen your understanding and skills in using PowerShell and SSH.