The "PowerShell hide" functionality allows you to run scripts or commands without displaying the console window, providing a discreet execution method. Here's an example of how to run a PowerShell script hidden:
$Hidden = New-Object -ComObject WScript.Shell
$Hidden.Run("powershell.exe -Command ""& {Your-Command-Here}""", 0, $false)
Replace `Your-Command-Here` with the command you want to execute.
Understanding PowerShell Hiding Techniques
What is Hiding in PowerShell?
In PowerShell, hiding typically refers to the ability to conceal certain elements, outputs, or processes from the user. This may include hiding the PowerShell console window itself, masking sensitive data inputs like passwords, or suppressing output from commands that are not necessary for the user to see. Understanding these hiding techniques can greatly improve how scripts are perceived and improve overall security practices.
Why Use Hiding Techniques?
Employing hiding techniques in PowerShell offers several crucial benefits:
- Protection of Sensitive Data: Hiding allows you to mask information, such as passwords or personally identifiable information (PII), which is vital for security.
- Improved User Experience: By minimizing unnecessary output, users can focus on the crucial parts of what a script does, leading to a smoother experience.
- Enhanced Script Functionality: Some scripts may require certain outputs to be suppressed to function correctly without cluttering the console.
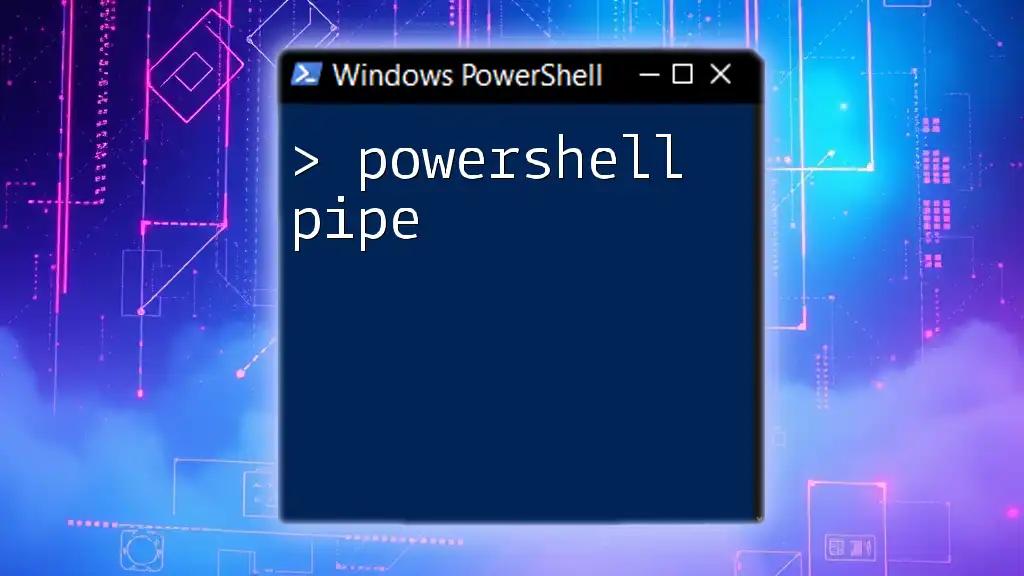
Common Use Cases for Hiding in PowerShell
Hiding Command Window and UI Elements
Hiding the PowerShell Window
One common scenario for hiding in PowerShell involves running scripts in a way that users cannot see the PowerShell window. This is particularly useful for GUI applications or scheduled tasks. You can achieve this by starting a new PowerShell process with a hidden window style.
Example Code Snippet:
$process = Start-Process PowerShell -ArgumentList "-NoProfile -ExecutionPolicy Bypass -File `"path\to\script.ps1`"" -WindowStyle Hidden
This command starts a new PowerShell process that runs your specified script without displaying the console window. It enhances application aesthetics and helps keep automated processes discreet.
Hiding Sensitive Information
Masking Passwords
When requesting user credentials, it's vital to ensure that sensitive information like passwords is not visible. PowerShell offers the `Read-Host` cmdlet with the `-AsSecureString` option that allows you to safely capture user input without displaying it on the screen.
Example Code Snippet:
$password = Read-Host -AsSecureString "Enter your password"
The input captured will be converted into a secure string, ensuring that it remains concealed and is encrypted in memory, thus adding a critical layer of security.
Hiding Output from the Console
Sometimes, it is beneficial to suppress the output of commands that may not be relevant to the user, thereby keeping the console clean. PowerShell allows the use of `Out-Null` to direct output away from the console.
Example Code Snippet:
Get-Service | Out-Null
This snippet runs the `Get-Service` command but does not display the results in the console. This technique is useful in scripts where the command's output is not needed or when logging output is handled differently.

Advanced Techniques for Hiding in PowerShell
Hiding Variables and Functions
Scoping Rules in PowerShell
PowerShell uses variable scoping rules to help manage the visibility of variables. Through these rules, you can limit a variable's scope to a function or script, effectively making it "hidden" from other parts of the script.
Example Code Snippet:
$privateVar = "This is a private variable"
function Hide-Variable {
$privateVar = "I'm hidden!"
Write-Output $privateVar
}
In this example, the `$privateVar` defined inside the `Hide-Variable` function cannot be accessed outside of it, making it hidden from the global scope. This encapsulation promotes better script organization and reduces the chances of unintended interactions.
Concealing Scripts and Commands
Obfuscating PowerShell Scripts
Obfuscation is another method that can be used to conceal the actual code of a script. It makes it harder for an observer to understand what the script does, providing a layer of protection for proprietary code.
Example Code Snippet:
# This is a simple form of obfuscation
$command = "Get-Process"
Invoke-Expression $command
Here, the command to retrieve processes is stored in a variable and executed later, making it less apparent during inspection. However, keep in mind that while obfuscation can deter casual observers, it is not foolproof against determined individuals.
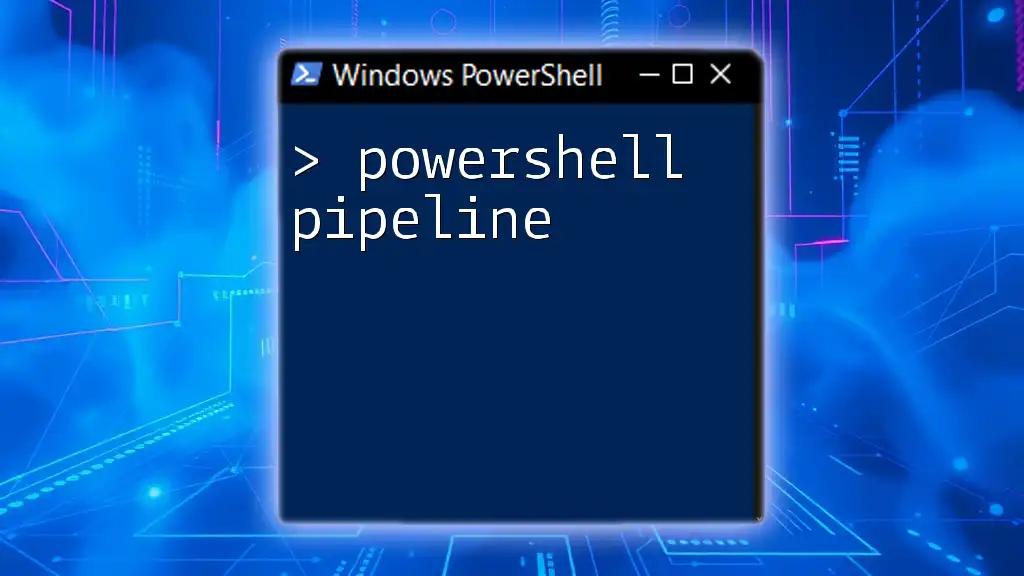
Tips and Best Practices for Hiding in PowerShell
Always Keep Security in Mind
When utilizing hiding techniques, always prioritize security. When handling sensitive information, ensure best practices like using `SecureString`, encrypting data, and employing controlled access. Never hard-code credentials directly into scripts, as this exposes them to anyone with access.
Maintain Code Readability
While hiding can be necessary, it is crucial to strike a balance between necessary obfuscation and code readability. Well-documented scripts can help other developers (or nawet your future self) understand why certain elements are hidden. This is essential for maintaining collaboration and future updates to the script.
Testing and Debugging Hidden Elements
Testing and debugging can become challenging when elements are hidden. When troubleshooting issues in your scripts, consider temporarily revealing certain hidden outputs by commenting out the hiding techniques or logging outputs for review. This approach allows easier diagnostics while still maintaining the intended hide functionalities.

Conclusion
The ability to effectively utilize hiding techniques in PowerShell can significantly enhance both the functionality of your scripts and the security of your data. By understanding and applying concepts like window hiding, output suppression, and variable scoping, you can create robust and user-friendly PowerShell scripts. Remember to practice these techniques and continually refine them as you grow in your PowerShell proficiency.
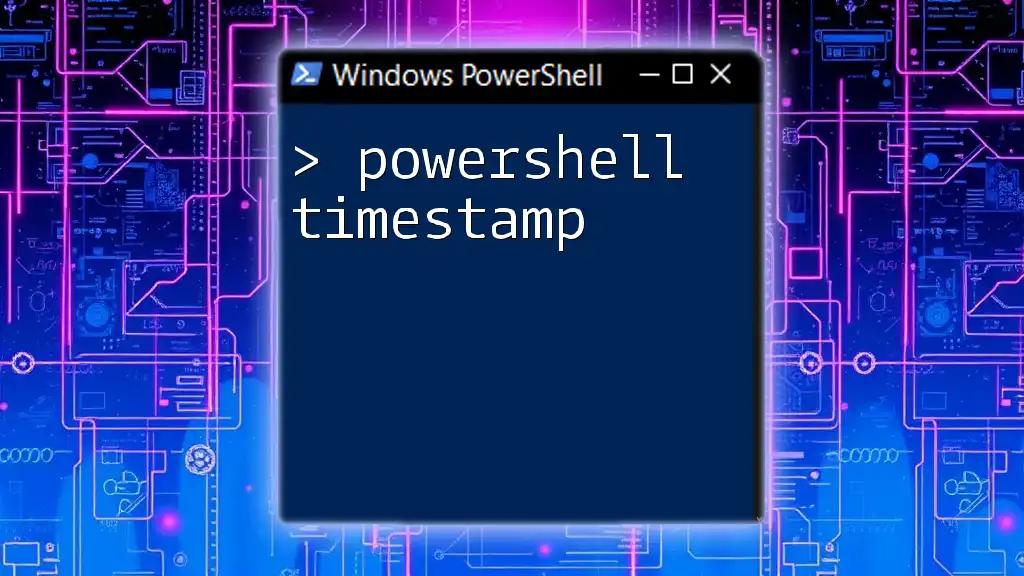
Additional Resources
- Visit the official PowerShell documentation for in-depth guidance on cmdlets and best practices.
- Consider online forums and communities to exchange knowledge with fellow PowerShell users, enriching your understanding and approach to hiding techniques.
Call-to-Action
If you're interested in mastering PowerShell commands and exploring even more advanced functionalities, be sure to sign up for our courses and resources tailored to help you become proficient in PowerShell!