Certainly! In PowerShell, you can sort objects by a specific property using the `Sort-Object` cmdlet, allowing you to easily organize your data for better readability and analysis.
Here's a code snippet demonstrating how to sort an array of objects by a property named `Name`:
$users = @(
[PSCustomObject]@{ Name = 'Alice'; Age = 30 },
[PSCustomObject]@{ Name = 'Bob'; Age = 25 },
[PSCustomObject]@{ Name = 'Charlie'; Age = 35 }
)
$sortedUsers = $users | Sort-Object -Property Name
$sortedUsers
Understanding `Sort-Object` in PowerShell
What is `Sort-Object`?
`Sort-Object` is a powerful cmdlet in PowerShell that is used to arrange objects in a specified order, either ascending or descending. This functionality is essential for efficiently managing data output, especially when you're dealing with large sets of information where the ability to find, compare, and analyze data quickly is paramount.
How to Use `Sort-Object`
The basic syntax for using `Sort-Object` is straightforward:
Sort-Object -Property <PropertyNames> [-Descending]
The `-Property` parameter specifies which property of the objects to sort by, while the `-Descending` switch allows you to sort the results in reverse order. Here’s a simple example to illustrate its basic use:
Get-Process | Sort-Object Name
In this command, `Get-Process` retrieves all running processes, and `Sort-Object Name` sorts them alphabetically by their names.

Sorting Objects with PowerShell
PowerShell Sort Objects
When sorting objects, it’s crucial to understand the properties available within those objects. For instance, each element in a collection can have numerous attributes, and choosing the right one to sort by can significantly affect your results.
Sorting by Property
To sort objects based on a specific property, simply specify the desired property in the `-Property` parameter. For example:
Get-Service | Sort-Object Status
In this example, the command retrieves all Windows services and sorts them by their current status (running, stopped, etc.). This kind of sorting can help you quickly identify services that need attention.
Sorting Alphabetically
Sorting strings alphabetically is a common requirement, particularly when working with text data. Here’s how to do it:
"apple", "banana", "orange" | Sort-Object
This command will return the items in alphabetical order as follows: apple, banana, orange.

Advanced Sorting Techniques
Using `Sort-Object` with Multiple Properties
You can also sort by more than one property. For instance, you may want to sort running processes first by CPU usage and then by Process ID. Here’s how:
Get-Process | Sort-Object CPU, Id
This command sorts the processes primarily by CPU usage, and if there’s a tie, it further sorts those by their IDs.
Descending Sort Order
When you need to sort in the opposite order, simply include the `-Descending` switch. An example is shown below:
Get-Process | Sort-Object CPU -Descending
This will list the processes using the highest amount of CPU first, which is often useful for performance troubleshooting.
Sorting Using Expressions
PowerShell allows you to use script blocks to define custom sorting logic. This is particularly useful for more complex scenarios. For instance, if you want to sort processes by their working set (memory usage):
Get-Process | Sort-Object { $_.WorkingSet }
Here, `$_` represents the current object in the pipeline, allowing for dynamic sorting based on your criteria.
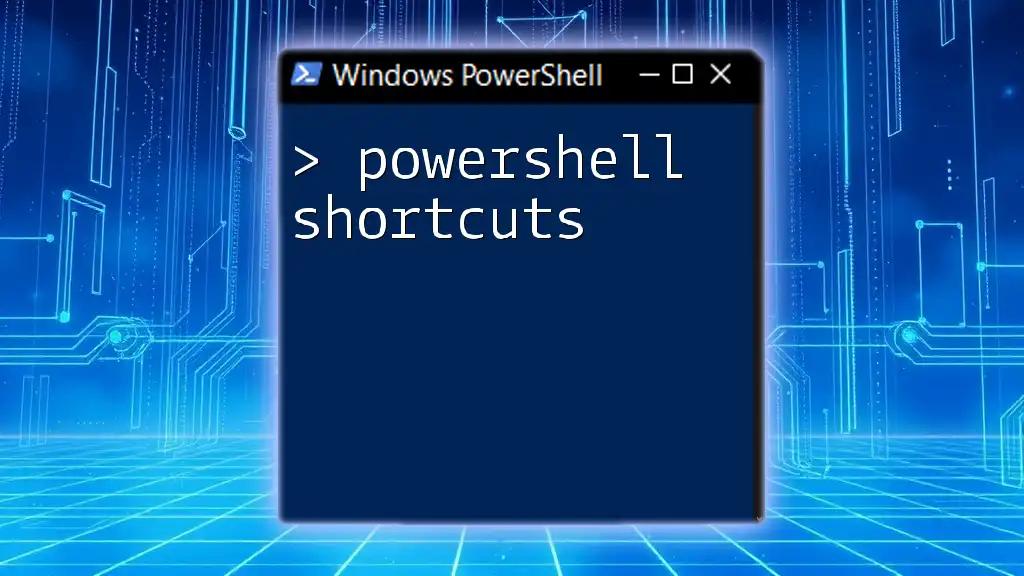
Common Scenarios for Sorting in PowerShell
Sorting User Accounts
Sorting user accounts by name can be particularly useful in administrative tasks. You can achieve this with the following command:
Get-LocalUser | Sort-Object Name
This will list all local user accounts in alphabetical order, making it easier to find specific users.
Sorting Files in a Directory
When managing files, sorting them based on size or modification date can save time. You can use:
Get-ChildItem | Sort-Object Length
This command sorts files in the current directory by their size, enabling you to quickly identify large files that may need attention.

Leveraging `Order By` in PowerShell
What is `Order By`?
The term `Order By` typically refers to a concept borrowed from SQL and used in various programming contexts. In PowerShell, it is not explicitly a command but describes the behavior of sorting data using `Sort-Object`.
Using `Order By` in PowerShell
To sort data effectively using `Order By`, leverage the capabilities of `Sort-Object`. Here’s an example of how to sort a set of user accounts effectively:
$users = Get-LocalUser
$sortedUsers = $users | Sort-Object -Property Name
Here, the scripts first gather all local users and then create a sorted list by name.
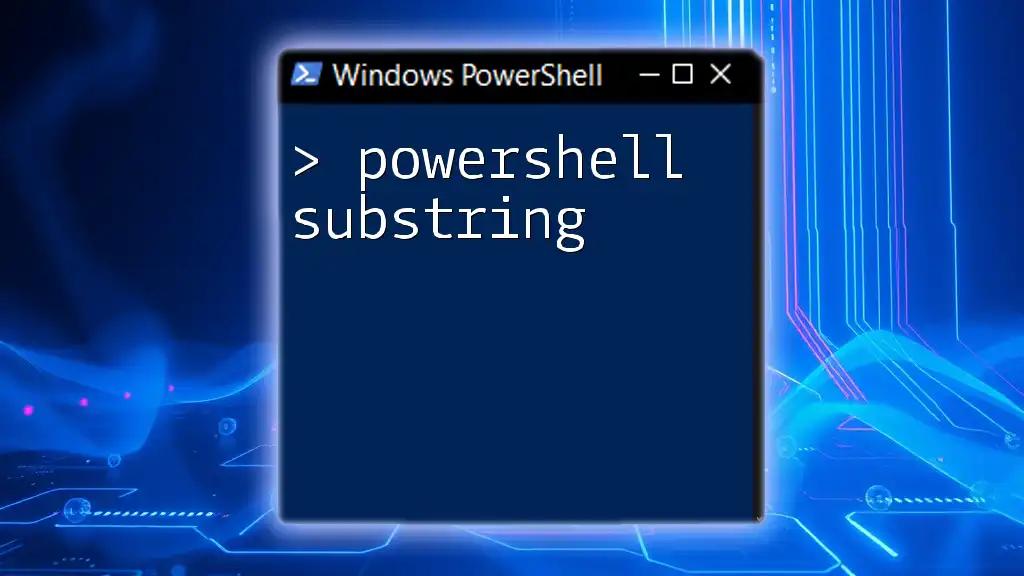
Practical Examples and Best Practices
Combining Sorting with Other Cmdlets
Sorting goes hand-in-hand with other cmdlets in PowerShell. For instance, when you want to analyze system logs, this command can come in handy:
Get-EventLog -LogName System | Sort-Object TimeGenerated -Descending
This retrieves system events ordered by their generated time, with the latest at the top—crucial for diagnosing recent issues.
Best Practices for Sorting in PowerShell
To optimize your sorting operations, consider the following best practices:
- Be selective about the properties you sort on to improve performance.
- Utilize `-Descending` judiciously, as excessive sorting can slow down your scripts.
- Experiment with different sorting methods, including custom expressions, for more complex datasets.
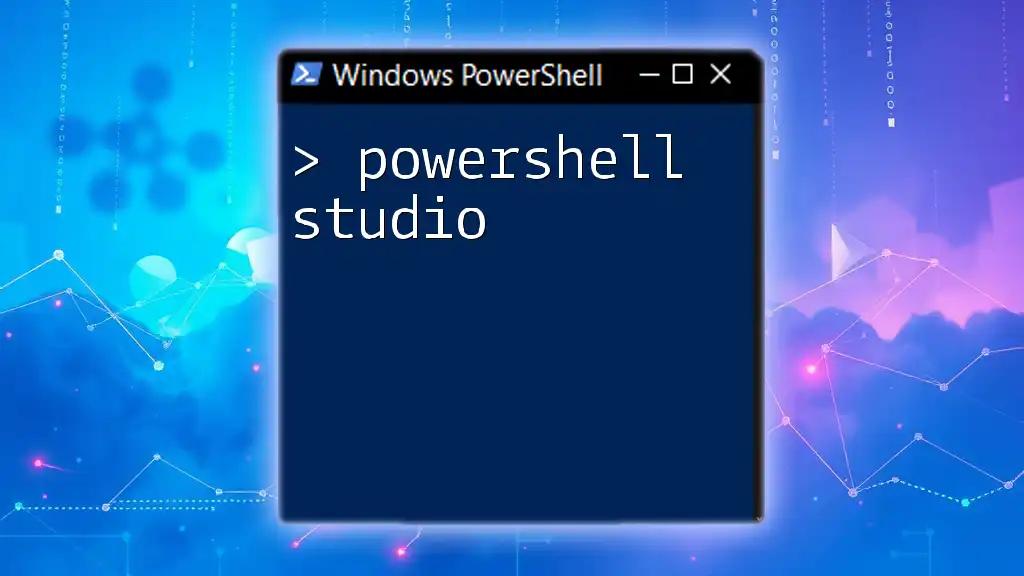
Conclusion
Sorting in PowerShell is not just a basic task; it's an essential skill required for any PowerShell user working with data. From simple alphabetic sorts to complex multi-parameter sorting, mastering these techniques will significantly enhance your productivity and efficiency in script writing.
Practice using the various sorting commands discussed to bolster your skills—whether you're a beginner or an experienced user looking to refine your commands. Happy scripting!